王道数据结构实践代码----顺序栈的实现(C语言版)
前言
日期:2021年10月28日 书籍:王道2021年数据结构考研复习指导 代码内容:
实现顺序栈的基本实现,主要功能如下: ? 栈的数据结构 ? 出栈 ? 入栈 ? 判栈空 ? 读栈顶
代码难点
1.关于i++和++i的使用
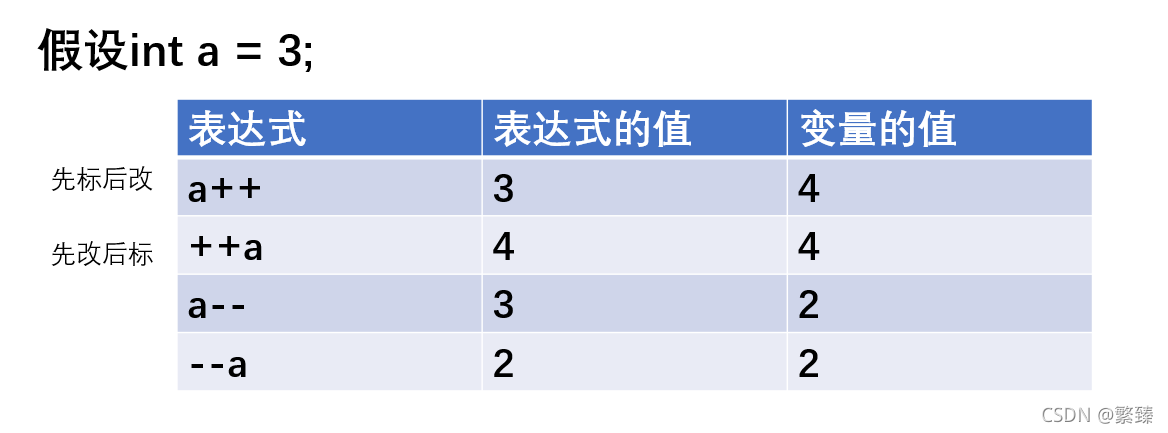
代码展示
1.顺序栈的数据结构
#include <stdio.h>
#include <stdlib.h>
#define bool char
#define true 1
#define false 0
#define MaxSize 50
typedef int Elemtype;
typedef struct SqStack
{
Elemtype data[MaxSize];
int top;
}SqStack;
2.顺序栈的初始化和判空
bool Initstack(SqStack *S)
{
S->top = -1;
}
bool StackEmpty(SqStack S)
{
if(S.top == -1) return true;
else return false;
}
3.顺序栈的进栈和出栈
bool Push(SqStack *S,Elemtype e)
{
if(S->top == MaxSize - 1) return false;
S->data[++S->top] = e;
return true;
}
bool Pop(SqStack *s,Elemtype *e)
{
if(StackEmpty(*s)) return false;
*e = s->data[s->top--];
return true;
}
4.顺序栈的读栈顶元素
bool GetTop(SqStack S,Elemtype *e)
{
if(StackEmpty(S)) return false;
*e = S.data[S.top];
return true;
}
整体代码
#include <stdio.h>
#include <stdlib.h>
#define bool char
#define true 1
#define false 0
#define MaxSize 50
typedef int Elemtype;
typedef struct SqStack
{
Elemtype data[MaxSize];
int top;
}SqStack;
bool Initstack(SqStack *S)
{
S->top = -1;
}
bool StackEmpty(SqStack S)
{
if(S.top == -1) return true;
else return false;
}
bool Push(SqStack *S,Elemtype e)
{
if(S->top == MaxSize - 1) return false;
S->data[++S->top] = e;
return true;
}
bool Pop(SqStack *s,Elemtype *e)
{
if(StackEmpty(*s)) return false;
*e = s->data[s->top--];
return true;
}
bool GetTop(SqStack S,Elemtype *e)
{
if(StackEmpty(S)) return false;
*e = S.data[S.top];
return true;
}
int main()
{
SqStack S;
Initstack(&S);
Push(&S,1);
Push(&S,2);
Push(&S,3);
Push(&S,4);
Push(&S,5);
Push(&S,6);
Elemtype X;
int count = S.top;
for(int i = 0;i <= count;i++)
{
printf("i = %d\n",i);
GetTop(S,&X);
printf("GetTop X = %d\n",X);
Pop(&S,&X);
printf("GetTop X = %d\n",X);
}
return 0;
}
运行效果
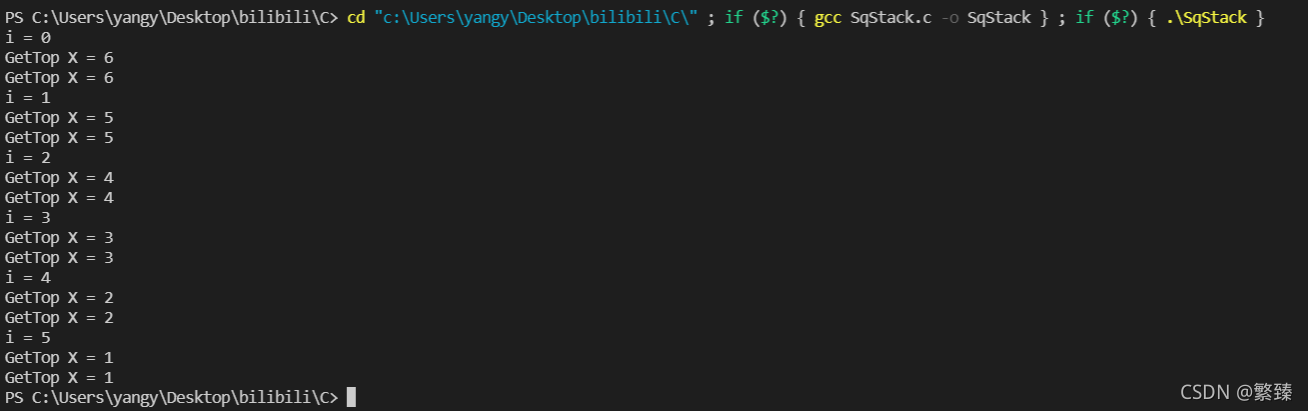
|