平衡二叉树(AVL树)
1)介绍
-
案例分析: 给一个数列{1, 2, 3, 4, 5, 6},要求创建一颗二叉排序树(BST),存在一些问题: 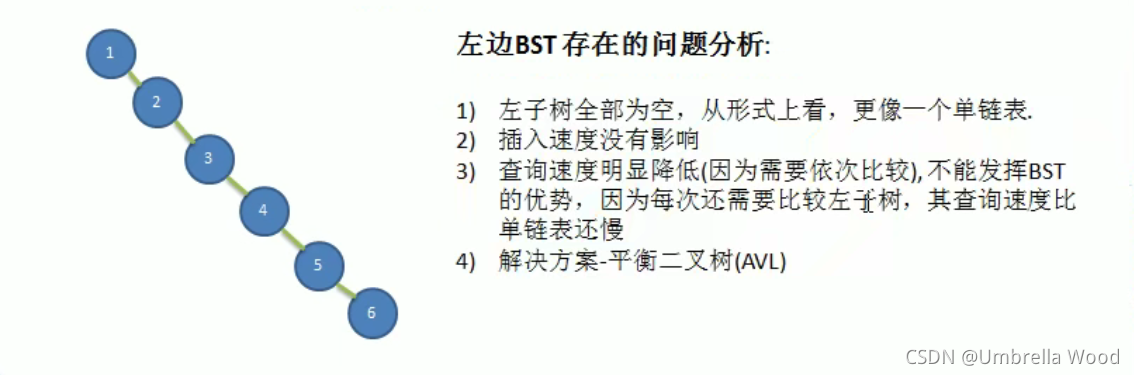 -
基本介绍:
-
平衡二叉树也叫平衡二叉搜索树(Self-balancing binary search tree)又被称为AVL树,可以保证查询效率为较高水平 -
具有以下特点:它是一颗空树或它的左右两个子树的高度差的绝对值不超过1,并且左右两个子树都是一颗平衡二叉树。平衡二叉树的常用实现方法有红黑树、AVL(算法)、替罪羊树、Treap、伸展树 等。 -
判断是否是AVL树
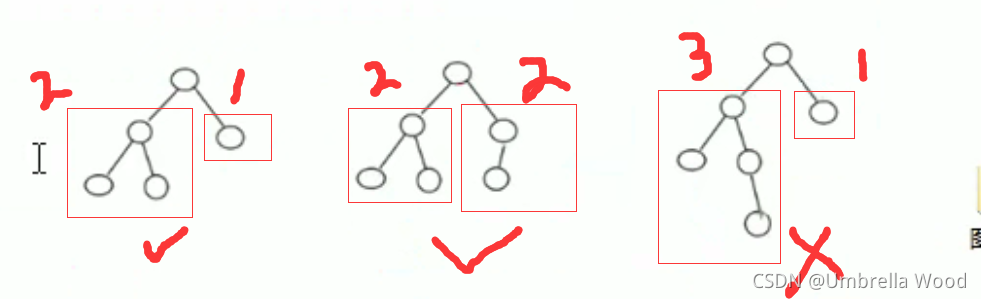
2)创建AVL树
-
应用案例—单旋转(左旋转) 左旋转的目的就是为了降低右子树的高度 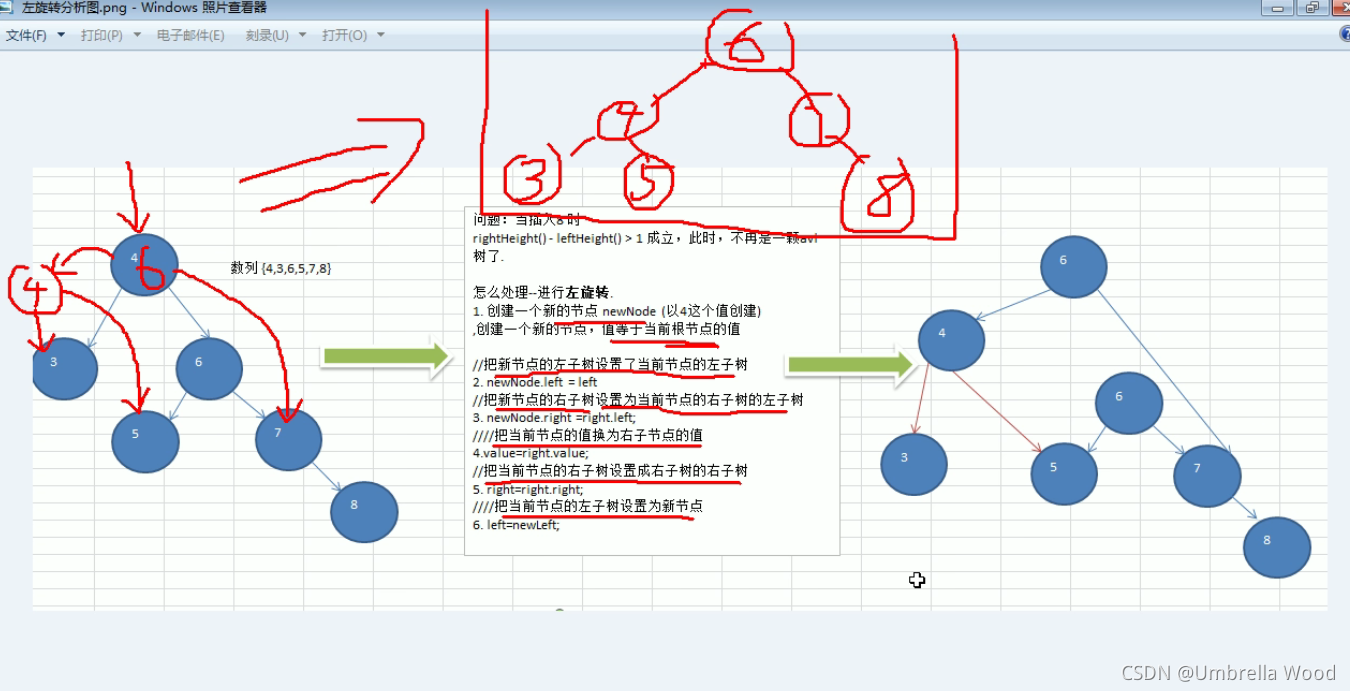 -
应用案例—单旋转(右旋转) 右旋转的目的就是为了降低左子树的高度
Ps:雷同左旋转
-
应用案例—双旋转 在某些情况下。单旋转不能完成平衡二叉树的转换,比如数列
int arr[] = {10, 11, 7, 6, 8, 9}
int arr[] = {2, 1, 6, 5, 7, 3}
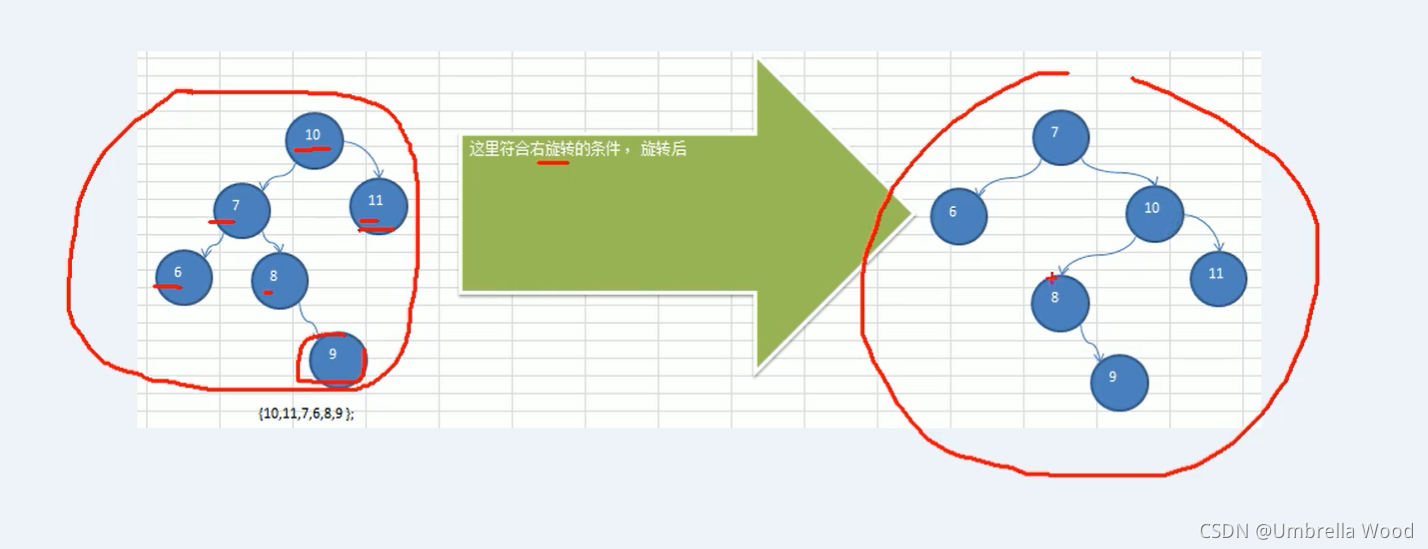
? 问题分析:
- 当符合右旋转的条件时<-> (leftHight() - rightHight() > 1)
- 当符合左旋转的条件时<-> (rightHight() - leftHight() > 1)
3)代码实现——单循环+双循环
package DataStructures.AVL;
public class AVLTreeDemo {
public static void main(String[] args) {
int[] arr = {10, 11, 7, 6, 8, 9};
AVLTree avlTree = new AVLTree();
for (int i = 0; i < arr.length; i++) {
avlTree.add(new Node(arr[i]));
}
System.out.println("中序遍历");
avlTree.infixOreder();
System.out.println("做平衡处理之后");
System.out.println("树的高度=" + avlTree.getRoot().height());
System.out.println("左子树的高度=" + avlTree.getRoot().leftHight());
System.out.println("右子树的高度=" + avlTree.getRoot().rightHight());
}
}
class AVLTree {
private Node root;
public Node getRoot() {
return root;
}
public Node search(int value) {
if (root == null) {
return null;
} else {
return root.search(value);
}
}
public Node searchParent(int value) {
if (root == null) {
return null;
} else {
return root.searchParent(value);
}
}
public int delRightTreeMin(Node node) {
Node target = node;
while (target.left != null) {
target = target.left;
}
delNode(target.value);
return target.value;
}
public int delLetfTreeMin(Node node) {
Node target = node;
while (target.right != null) {
target = target.right;
}
delNode(target.value);
return target.value;
}
public void delNode(int value) {
if (root == null) {
return;
} else {
Node targetNode = search(value);
if (targetNode == null) {
return;
}
if (root.left == null && root.right == null) {
root = null;
return;
}
Node parent = searchParent(value);
if (targetNode.left == null && targetNode.right == null) {
if (parent.left != null && parent.left.value == value) {
parent.left = null;
} else if (parent.right != null && parent.right.value == value) {
parent.right = null;
}
} else if (targetNode.left != null && targetNode.right != null) {
int maxVal = delLetfTreeMin(targetNode.left);
targetNode.value = maxVal;
} else {
if (targetNode.left != null) {
if (parent != null) {
if (parent.left.value == value) {
parent.left = targetNode.left;
} else {
parent.right = targetNode.left;
}
} else {
root = targetNode.left;
}
} else {
if (parent != null) {
if (parent.left.value == value) {
parent.left = targetNode.right;
} else {
parent.right = targetNode.right;
}
} else {
root = targetNode.right;
}
}
}
}
}
public void add(Node node) {
if (root == null) {
root = node;
} else {
root.add(node);
}
}
public void infixOreder() {
if (root != null) {
root.infixOrder();
} else {
System.out.println("当前二叉排序树为空");
}
}
}
class Node {
int value;
Node left;
Node right;
public Node(int value) {
super();
this.value = value;
}
public int leftHight() {
if (left == null) {
return 0;
} else {
return left.height();
}
}
public int rightHight() {
if (right == null) {
return 0;
} else {
return right.height();
}
}
public int height() {
return Math.max(left == null ? 0 : left.height(), right == null ? 0 : right.height()) + 1;
}
private void leftRotate() {
Node newNode = new Node(value);
newNode.left = left;
newNode.right = right.left;
value = right.value;
right = right.right;
left = newNode;
}
private void rightRotate() {
Node newNode = new Node(value);
newNode.right = right;
newNode.left = left.right;
value = left.value;
left = left.left;
right = newNode;
}
@Override
public String toString() {
return "Node{" + "value=" + value + '}';
}
public void add(Node node) {
if (node == null) {
return;
}
if (node.value < this.value) {
if (this.left == null) {
this.left = node;
} else {
this.left.add(node);
}
} else {
if (this.right == null) {
this.right = node;
} else {
this.right.add(node);
}
}
if (rightHight() - leftHight() > 1) {
if (right != null && right.leftHight() > right.rightHight()) {
right.rightHight();
leftRotate();
} else {
leftRotate();
}
return;
}
if (leftHight() - rightHight() > 1) {
if (left != null && left.rightHight() > left.leftHight()) {
left.leftRotate();
rightRotate();
} else {
rightRotate();
}
}
}
public void infixOrder() {
if (this.left != null) {
this.left.infixOrder();
}
System.out.println(this);
if (this.right != null) {
this.right.infixOrder();
}
}
public Node search(int value) {
if (value == this.value) {
return this;
} else if (value < this.value) {
if (this.left == null) {
return null;
}
return this.left.search(value);
} else {
if (this.right == null) {
return null;
}
return this.right.search(value);
}
}
public Node searchParent(int value) {
if ((this.left != null && this.left.value == value) || (this.right != null && this.right.value == value)) {
return this;
} else {
if (value < this.value && this.left != null) {
return this.left.searchParent(value);
} else if (value >= this.value && this.right != null) {
return this.right.searchParent(value);
} else {
return null;
}
}
}
}
|