概述
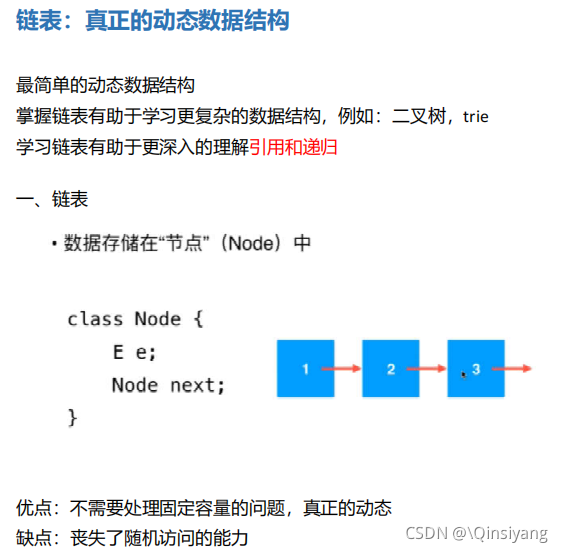
MyLink
package link;
public class MyLink<T> {
private class Node {
private T val;
private Node next;
Node(T val) {
this.val = val;
this.next = null;
}
@Override
public String toString() {
return val.toString();
}
}
private Node head;
private int size;
public MyLink() {
head = null;
}
public boolean isEmpty() {
return this.size == 0;
}
public int getSize() {
return this.size;
}
public void addTail(T val) {
Node node = new Node(val);
if (head == null) {
head = node;
} else {
Node curNode = head;
while (curNode.next != null) {
curNode = curNode.next;
}
curNode.next = node;
}
this.size++;
}
public void addHead(T val) {
Node node = new Node(val);
node.next = head;
head = node;
this.size++;
}
public void add0(T val, int index) {
Node node = new Node(val);
if (index < 0 || index > size) {
throw new IllegalArgumentException("index is error");
}
if (head == null) {
head = node;
this.size++;
return;
}
if (index == 0) {
this.addHead(val);
return;
}
Node preNode = head;
for (int i = 0; i < index - 1; i++) {
preNode = preNode.next;
}
node.next = preNode.next;
preNode.next = node;
this.size++;
}
public void add(T val, int index) {
if (index > this.size || index < 0) {
throw new IllegalArgumentException("index is error");
}
Node dummyHead = new Node(null);
Node node = new Node(val);
dummyHead.next = head;
Node preNode = dummyHead;
for (int i = 0; i < index; i++) {
preNode = preNode.next;
}
node.next = preNode.next;
preNode.next = node;
this.size++;
head = dummyHead.next;
}
public T getFirst() {
return get(0);
}
public T getLast() {
return get(this.size - 1);
}
public T get(int index) {
if (index < 0 || index > this.size) {
throw new IllegalArgumentException("index is error");
}
Node curNode = head;
for (int i = 0; i < index; i++) {
curNode = curNode.next;
}
return curNode.val;
}
public boolean isContains(T val) {
Node curNode = head;
while (curNode != null && !curNode.val.equals(val)) {
curNode = curNode.next;
}
return curNode != null;
}
public void set(int index, T val) {
if (index < 0 || index >= this.size) {
throw new IllegalArgumentException("index is error");
}
Node curNode = head;
for (int i = 0; i < index; i++) {
curNode = curNode.next;
}
curNode.val = val;
}
public T removeFirst() {
return removeByIndex(0);
}
public T removeLast() {
return removeByIndex(this.size - 1);
}
public T removeByIndex(int index) {
if (index >= this.size || index < 0) {
throw new IllegalArgumentException("index is error");
}
Node dummyHead = new Node(null);
dummyHead.next = head;
Node preNode = dummyHead;
for (int i = 0; i < index; i++) {
preNode = preNode.next;
}
Node delNode = preNode.next;
T result = delNode.val;
preNode.next = delNode.next;
delNode.next = null;
this.size--;
head = dummyHead.next;
return result;
}
public Node removeByVal(T val) {
Node dummyHead = new Node(null);
dummyHead.next = head;
Node preNode = dummyHead;
while (preNode.next != null) {
T result = preNode.next.val;
if (result.equals(val)) {
Node delNode = preNode.next;
preNode.next = delNode.next;
delNode.next = null;
this.size--;
} else {
preNode = preNode.next;
}
}
return dummyHead.next;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
Node curNode = head;
while (curNode != null) {
sb.append(curNode + "-->");
curNode = curNode.next;
}
return sb.toString();
}
}
|