"""
1.定义一个生成器函数,生成1-10
使用next(generator)方法获取1-10
使用for循环获取
"""
def get_num():
for num in range(1, 11):
yield num
gen = get_num()
print(type(gen))
for i in gen:
print(i)
print("=" * 80)
"""
2.# 自己定义一个MyIterator, 实现range的功能
# # range(start, stop, step) => MyIterator
# # range(10) # start=0, stop=10, step=1
# # rang(10) # range(start=0, stop, step=1) =>start=10
# # range(0,10)
# # range(0,10,2)
# # MyIterator(10)
# # MyIterator(0,10)
# # MyIterator(0,10,2)
"""
class Myrange(object):
def __init__(self, stop=None, start=None, step=1):
self.start = start
self.stop = stop
self.step = step
print(start)
def __iter__(self):
self.cur_val = self.start
return self
def __next__(self):
self.cur_val += self.step
if self.cur_val < self.stop:
return self.cur_val
else:
raise StopIteration
for i in Myrange(start=-2, stop=13, step=2):
print(i)
print("=" * 80)
"""
3. re中函数的使用(自己写用例来使用):
match
fullmatch
search
findall
finditer
split
sub
subn
complie
"""
import re
pattern = "hello"
string = "hello world"
result = re.match(pattern, string)
print(result, type(result))
print("=" * 80)
pattern = "hello"
string = "hello"
match_obj = re.fullmatch(pattern, string)
print(match_obj)
print("=" * 80)
pattern = "hello"
string1 = "hello world"
string2 = "world hello"
string3 = "hello world hello"
match_obj = re.search(pattern, string1)
print(match_obj)
match_obj = re.search(pattern, string2)
print(match_obj)
match_obj = re.search(pattern, string3)
print(match_obj)
print("=" * 80)
string3 = "hello world hello"
pattern = "hello"
result = re.findall(pattern, string3)
print(result)
print("=" * 80)
string3 = "hello world hello"
pattern = "hello"
result = re.finditer(pattern, string3)
print(result)
for i in result:
print(i)
print("=" * 80)
result = re.split(pattern, string, maxsplit=1)
print(result)
print("=" * 80)
result = re.sub(",", "-", "计算机,软件,网络", 1)
print(result)
print("=" * 80)
result = re.subn(",", "-", "计算机,软件,网络", 1)
print(result)
print("=" * 80)
string3 = "hello world hello"
pattern = "hello"
compile_obj = re.compile(pattern)
print(compile_obj.search(string3))
print(compile_obj.findall(string3))
print(compile_obj.match(string3))
print("=" * 80)
运行结果: 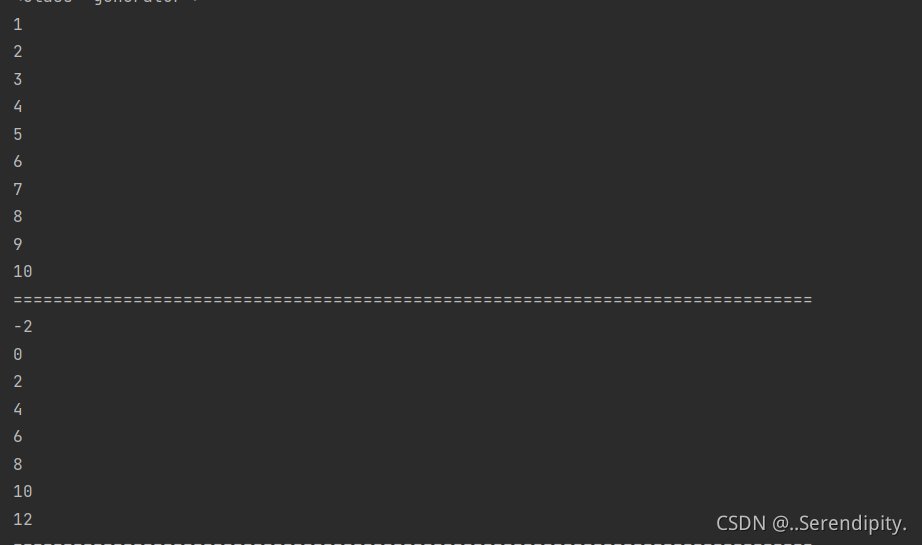
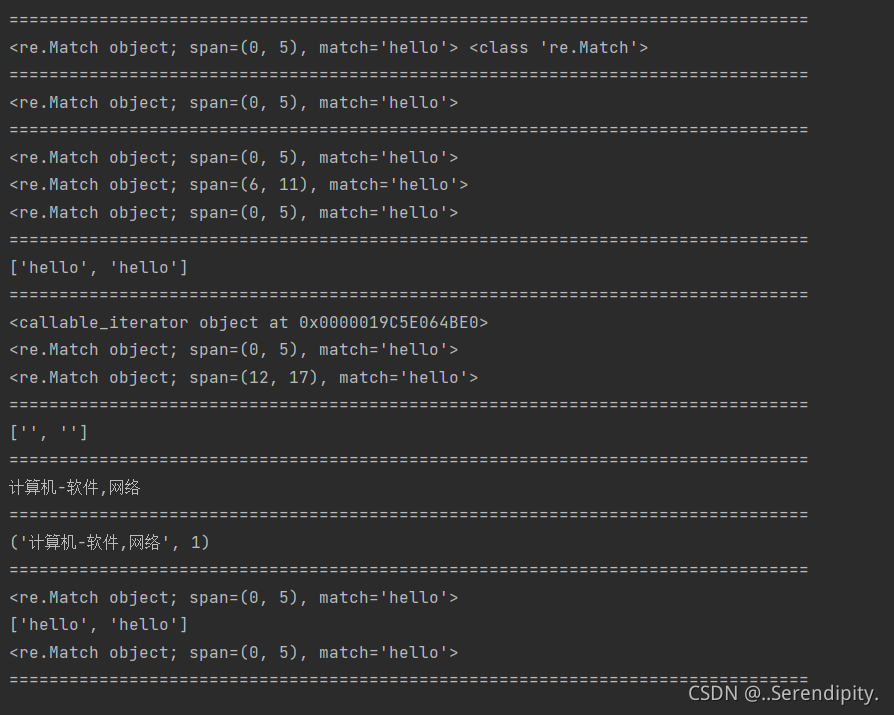
|