什么是队列
队列是一种特殊的线性表,特殊之处在于它只允许在表的前端(front)进行删除操作,而在表的后端(rear)进行插入操作,和栈一样,队列是一种操作受限制的线性表。进行插入操作的端称为队尾,进行删除操作的端称为队头。队列中没有元素时,称为空队列。 队列的数据元素又称为队列元素。在队列中插入一个队列元素称为入队,从队列中删除一个队列元素称为出队。因为队列只允许在一端插入,在另一端删除,所以只有最早进入队列的元素才能最先从队列中删除,故队列又称为先进先出(FIFO—first in first out)线性表。 ——form baidu
队列的结构
队列是一种先进先出的结构,由队首指针和队尾指针构成 这里用数组进行模拟 同时我也加上了一个currentLength 来对队列的长度进行动态统计 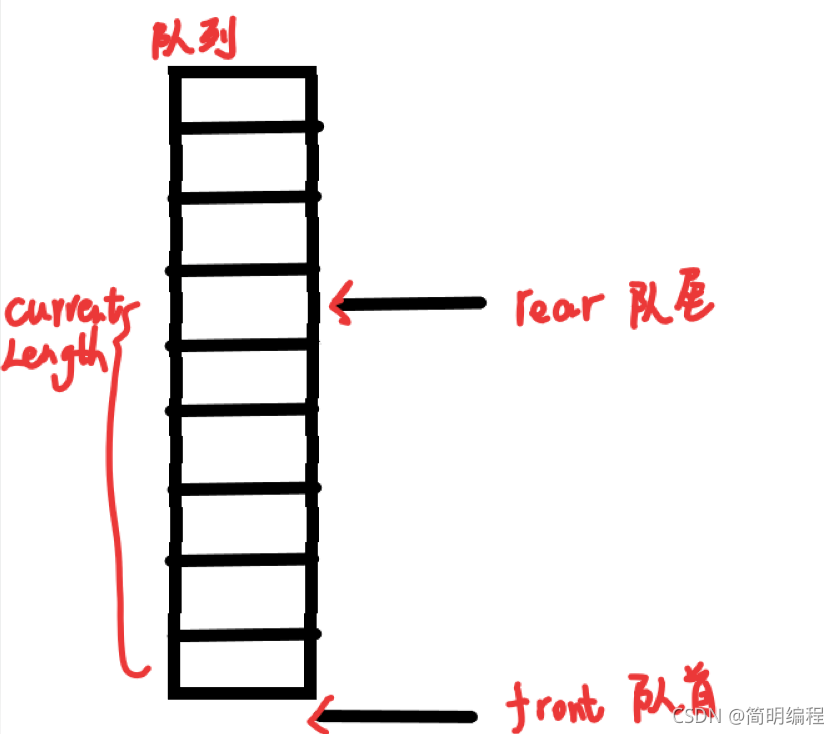
应用
实际生活中,队列的应用随处可见,比如排队买 XXX、医院的挂号系统等,采用的都是队列的结构
数组模拟队列代码
实体类:
package queue;
public class ArrQueueDemo {
private int maxSize;
private int front;
private int rear;
private int[] arr;
private int currentLength;
public ArrQueueDemo(int arrMaxSize) {
this.maxSize = arrMaxSize;
this.arr = new int[maxSize];
this.front = -1;
this.rear = -1;
}
public void initArrQueue() {
System.out.println("当前队列已初始化成功");
System.out.println("队列最大长度为:" + maxSize);
this.showQueue();
this.currentLength = 0;
System.out.println("队列当前长度为:" + currentLength);
}
public void getCurrentLength() {
if (isEmpty()) {
System.out.println("队列为空");
}
if (isFull()) {
System.out.println("队列已满");
System.out.println(maxSize);
}
System.out.println(currentLength);
}
public boolean isFull() {
return rear == maxSize - 1;
}
public boolean isEmpty() {
return rear == front;
}
public void push(int n) {
if (isFull()) {
System.out.println("队列已满");
return;
}
rear++;
arr[rear] = n;
this.currentLength++;
}
public void pop() {
int flag = 0;
if (isEmpty()) {
throw new RuntimeException("队列为空无法出队列");
}
this.front++;
this.currentLength--;
System.out.println(arr[front]);
for (int i = 0; i < currentLength; i++) {
arr[i] = arr[++flag];
}
rear--;
front--;
this.showQueue();
}
public void showQueue() {
System.out.println("当前的队列为");
if (isEmpty()) {
System.out.println("队列为空");
return;
}
for (int i = 0; i < currentLength; i++) {
System.out.printf("%d\t", arr[i]);
}
System.out.println();
}
public void headQueue() {
if (isEmpty()) {
throw new RuntimeException("队列为空");
}
front++;
System.out.println(arr[front]);
front--;
}
public void cleanQueue() {
if (isEmpty()) {
System.out.println("队列已空无法清空!");
}
for (int i = 0; i < currentLength; i++) {
arr[i] = 0;
}
this.showQueue();
}
public void destoryQueue() {
this.arr = null;
}
public void tailQueue(){
if (isEmpty()) {
throw new RuntimeException("队列为空");
}
System.out.println(arr[rear]);
}
}
实现类:
package queue;
import java.util.Scanner;
public class ArrQueueDemoTest {
public static void main(String[] args) {
int maxSize = 100;
ArrQueueDemo arrQueue = new ArrQueueDemo(maxSize);
int choose = -1;
Scanner sc = new Scanner(System.in);
boolean flag = true;
while (flag) {
System.out.println("============================");
System.out.println("=========1.初始化队列========");
System.out.println("=========2.添加元素=========");
System.out.println("=========3.显示队列=========");
System.out.println("=========4.元素出队列========");
System.out.println("=========5.获取队列头========");
System.out.println("=========6.获取队列长度======");
System.out.println("=========7.清空队列=========");
System.out.println("=========8.销毁队列=========");
System.out.println("=========9.获取队列尾========");
System.out.println("=========0.退出exit=========");
System.out.println("============================");
System.out.println("请输入你的选择:");
choose = sc.nextInt();
if (choose == 0) {
System.out.println("exit...");
break;
}
switch (choose) {
case 1:
arrQueue.initArrQueue();
break;
case 2:
System.out.println("输入你要添加的数据:");
int ele = sc.nextInt();
arrQueue.push(ele);
arrQueue.showQueue();
break;
case 3:
arrQueue.showQueue();
break;
case 4:
arrQueue.pop();
break;
case 5:
arrQueue.headQueue();
break;
case 6:
arrQueue.getCurrentLength();
break;
case 7:
arrQueue.cleanQueue();
break;
case 8:
arrQueue.destoryQueue();
arrQueue = null;
break;
case 9:
arrQueue.tailQueue();
break;
default:
System.out.println("error");
break;
}
}
}
}
演示:
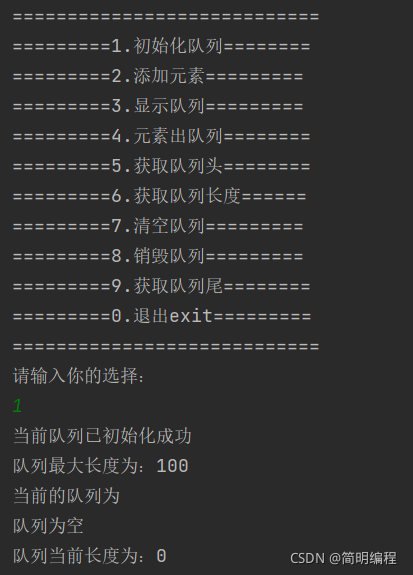 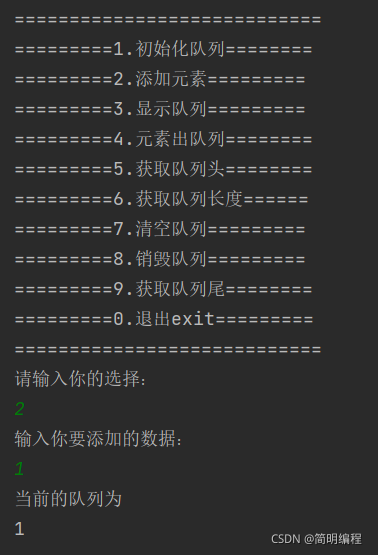 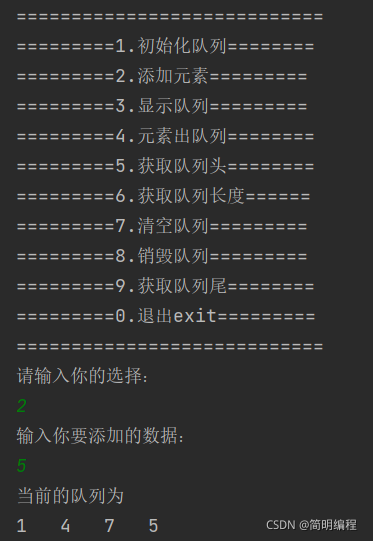 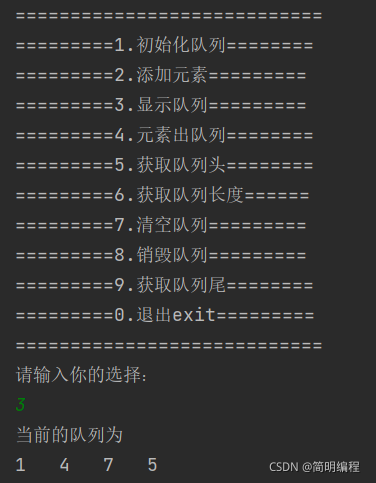 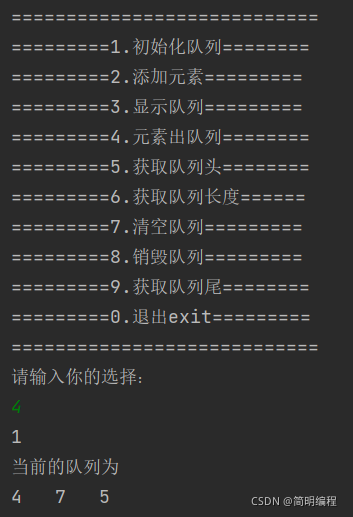 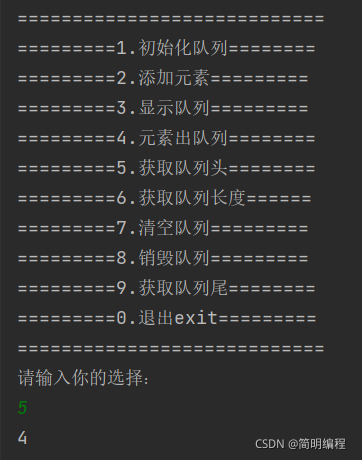 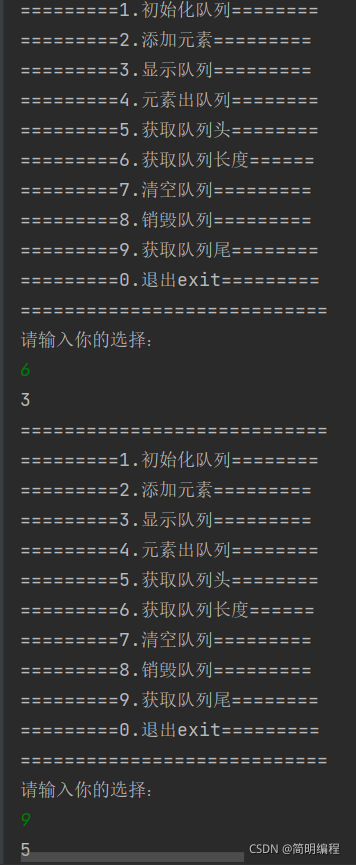 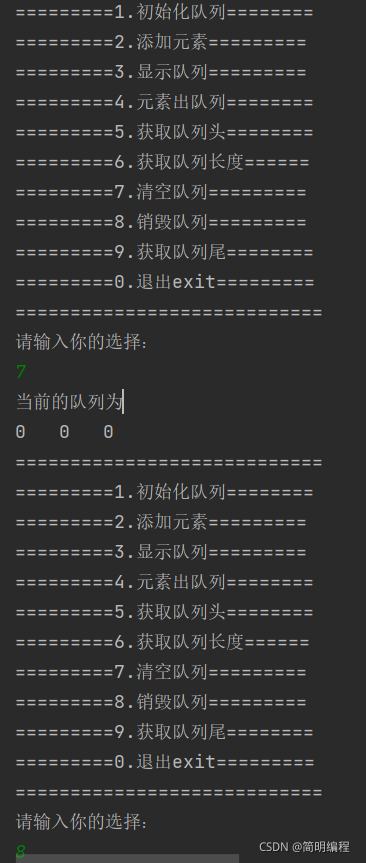 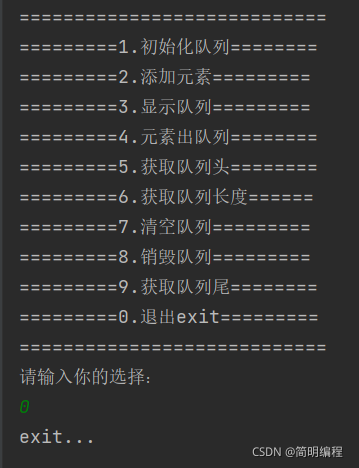
扩展
当然你也可以对代码进行改进 比如多次插入元素,多次删除元素
注意:
当我们在对队列进行销毁之后要重新启动,以为我把整个队列对象和队列里模拟的数组销毁了 否则会报错: 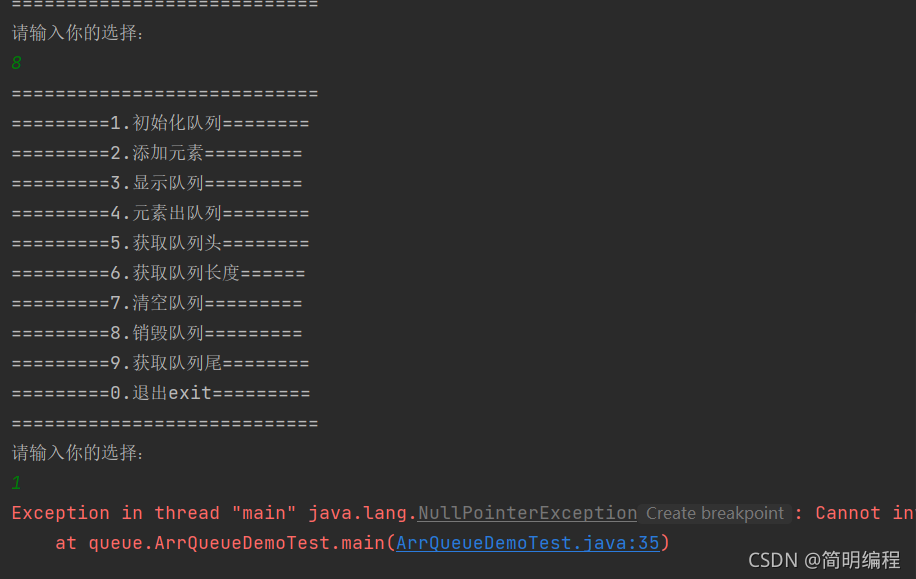
|