一、整体思路
题目要求输出所有的的最长公共子序列,在遍历数组c查找最长公共子序列长度之后再用print函数输出结果,发现并不能输出所有的最长公共子序列。原因是使用dp算法创建数组b时保留的路径并不完整,所以用b数组来查找子序列的方法不可行。 因此,在查找公共子序列时还需要根据字符串s1和s2中的内容进行比较。此处的难点在于递归时如何保留相同的字符串,因此需要在递归函数的参数中加入一个String类型的参数,保留每一层递归时的结果字符串
二、程序代码
package Lcs;
import java.util.HashSet;
import java.util.Scanner;
import java.util.Set;
public class LCS {
private int[][] b;
private int[][] c;
private String str1;
private String str2;
private int len1;
private int len2;
String str="";
private Set<String> set = new HashSet<String>();
public int findLCS(){
char[] s1=str1.toCharArray();
char[] s2=str2.toCharArray();
int i,j;
for(i = 1;i <=len1;i++)
for(j = 1;j <=len2;j++)
{
if(s1[i-1]==s2[j-1])
{
c[i][j] = c[i-1][j-1]+1;
b[i][j] = 1;
}
else
{
if(c[i-1][j]>=c[i][j-1])
{
c[i][j] = c[i-1][j];
b[i][j] = 2;
}
else
{
c[i][j] = c[i][j-1];
b[i][j] = 3;
}
}
}
return c[len1][len2];
}
public void inputData(){
Scanner scanner=new Scanner(System.in);
System.out.println("请输入字符串s1:");
str1=scanner.nextLine();
System.out.println("请输入字符串s2:");
str2=scanner.nextLine();
len1=str1.length();
len2=str2.length();
c=new int[len1+1][len2+1];
b=new int[len1+1][len2+1];
}
public void display(){
int len=findLCS();
System.out.println(str1+"和"+str2+"的最长公共子序列长度是:"+len);
System.out.print("最长公共子序列的数量是:");
print(len1,len2,str);
System.out.println(set.size());
System.out.println("最长公共子序列是:");
for (String str : set) {
System.out.println(str);
}
}
public void print(int i,int j,String s){
char[] s1=str1.toCharArray();
char[] s2=str2.toCharArray();
while(i>0&&j>0){
if(s1[i-1]==s2[j-1]){
s+=s1[i-1];
i--;
j--;
}
else{
if(c[i-1][j]>c[i][j-1]){
i--;
}
else if(c[i-1][j]<c[i][j-1]){
j--;
}
else{
print(i-1,j,s);
print(i,j-1,s);
return;
}
}
}
String res =new StringBuffer(s).reverse().toString();
set.add(res);
}
public static void main(String[] args) {
LCS lcs=new LCS();
lcs.inputData();
lcs.display();
}
}
其中,递归函数print()参考了大佬的代码。传送门
三、运行结果
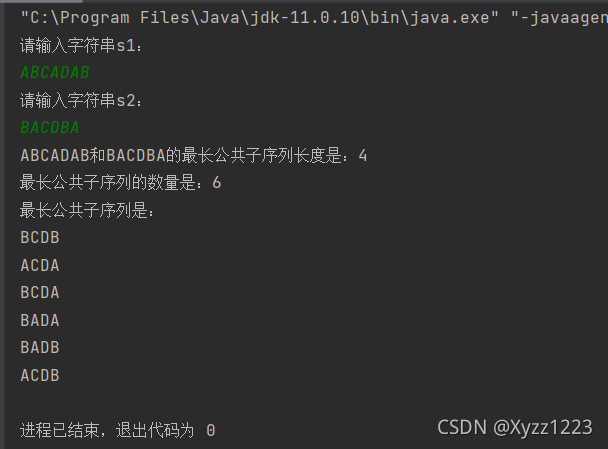
|