循环队列
1、当队列数据存储到队列的尾时,如果前面有空的位置,队列尾可以继续到队列开始的地方继续存储。
2、如果此时存储过程中,又遇到了Head = Tail的情况,说明队列存储满了。但是,开始时用来判断队列是否为空也是这个条件。所以改进的方法是:浪费一个存储空间用来判断,当Tail + 1 == Head,说明队列存储满了。
在之前的代码基础上添加判断队列是否满了的判断函数。
int is_full(void)
{
return tail + 1 == head;
}
-
环形队列的提出
1、
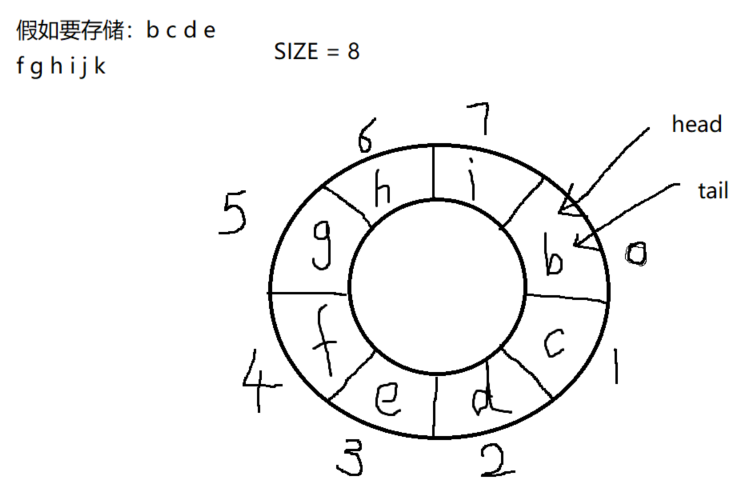
如果,当我的tail到了最后一个存储空间,标号为:7,此时,tail + 1 = 8,tail != head(此时head == 0),如何解决这个问题,这里直接拿出解决方法。一个不大于最大值的数,其+1后对最大值取余运算后还是其本身 。比如这里的最大值就是存储空间的最大容量为8,(tail + 1)% 8 = 0。问题解决!
更新后的程序如下:
#include <stdio.h>
#define SIZE 512
char queue[SIZE];
int head, tail = 0;
void enqueue(char c);
char dequeue(void);
int is_empty(void);
int is_full(void);
int main(void)
{
int i;
char temp = 'A';
if(!is_full())
{
for(i = 0; i < 5; i++)
{
enqueue(temp);
temp++;
}
}
while(!is_empty())
{
printf("%c\n", dequeue());
}
return 0;
}
void enqueue(char c)
{
if(!is_full())
queue[tail++] = c;
tail = (tail + 1) % SIZE;
}
char dequeue(void)
{
char ch;
if(!is_empty())
ch = queue[head++];
return ch;
}
int is_empty(void)
{
return head == tail;
}
int is_full(void)
{
return ((tail + 1) % SIZE) == head;
}
运行结果:
sgk@sgk-VirtualBox:~/C_Study/DataStructure$ gcc queue.c && ./a.out
A
B
C
D
E