数据结构
??数据结构-第一章 ??抽象数据类型案例 ??数据结构-第二章(1)-线性结构 ??数据结构-第二章(2)-线性表的顺序表示和实现
一、前言
二、顺序表的基本操作
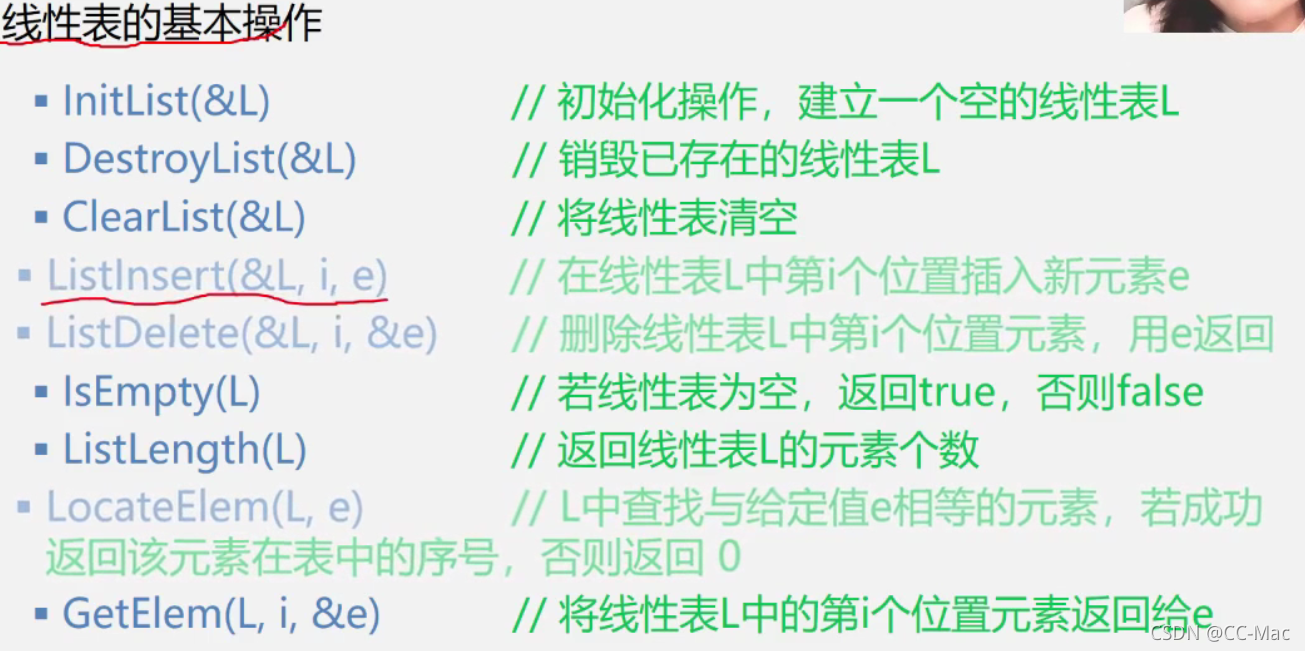 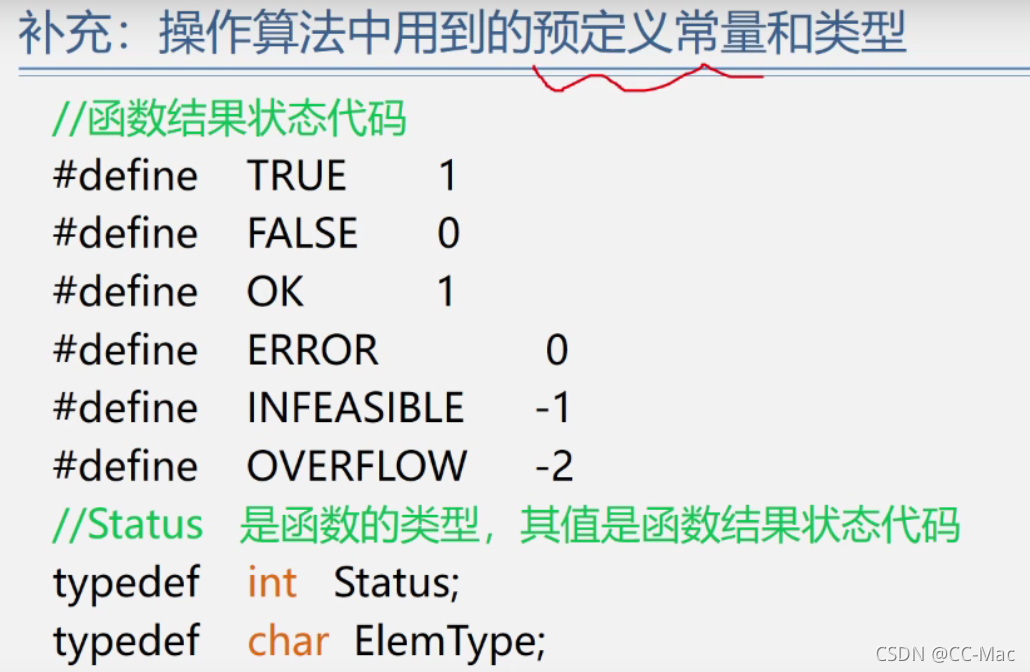 1?? 创建顺序表结构类型
静态分配 | 动态分配 |
---|
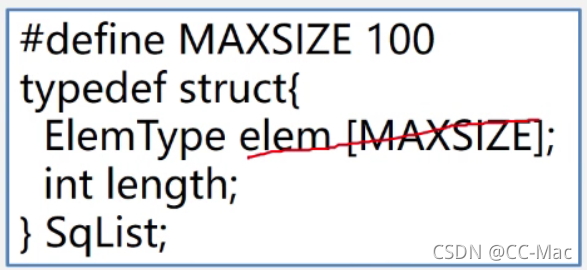 | 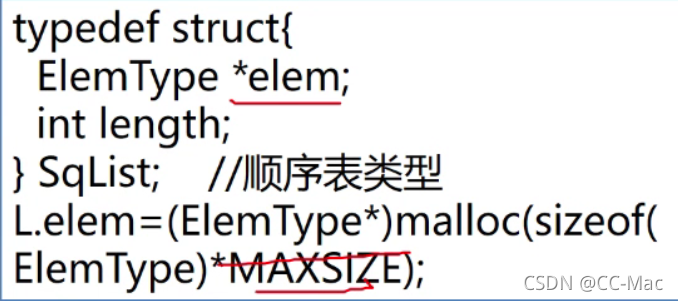 | | |
2?? 线性表初始化
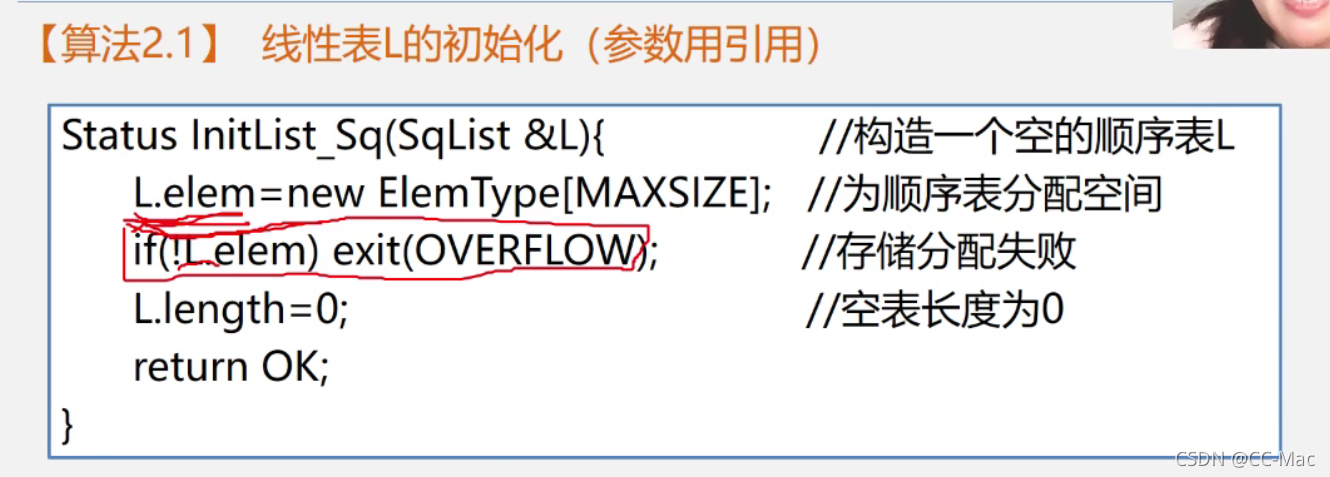 C++不支持默认的int,所以在初始化赋值时,使其返回类型为空。
void InitList(SqList &L)
{
int max_size;
cout << "请输入顺序表的最大长度:" << endl;
cin >> max_size;
if (max_size == 0 || max_size > MAXSIZE)
{
max_size = MAXSIZE;
cout << "顺序表最大长度为:" << max_size << endl;
}
L.elem = new ElemType[MAXSIZE];
if (!L.elem)
{
cout << "内存分配失败" << endl;
}
cout << "请输入当前顺序表的长度:" << endl;
cin >> L.length;
L.listsize = max_size;
cout << "请输入当前顺序表的元素: ";
for (int i = 0; i < L.length; i++)
{
cin >> L.elem[i];
}
system("pause");
}
3?? 销毁和清除线性表 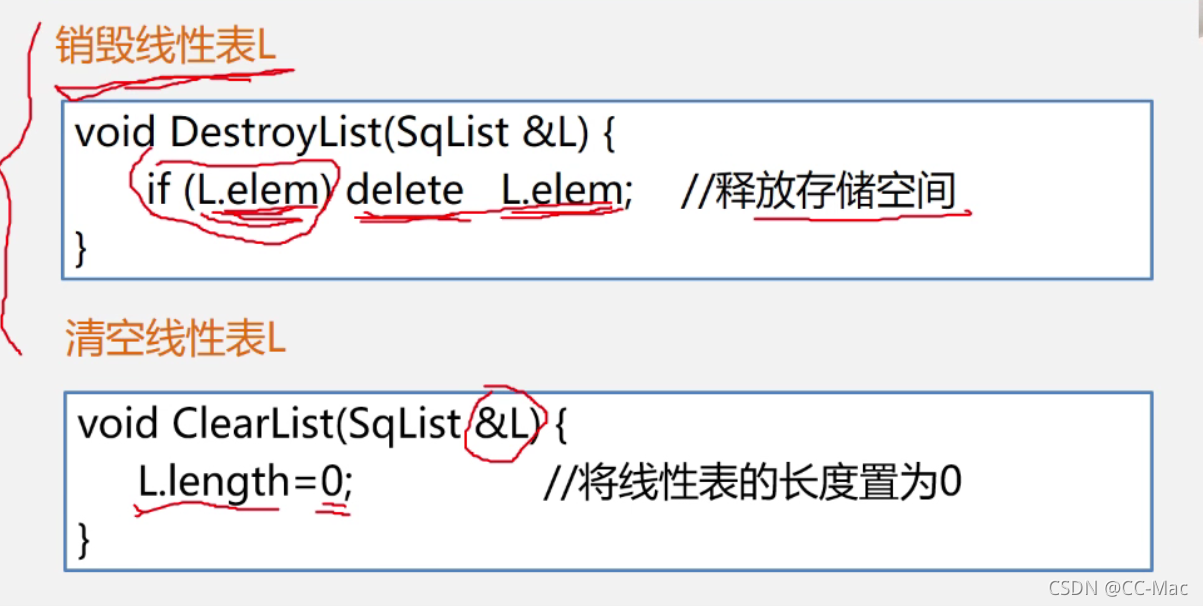
void ClearList(SqList &L)
{
L.length = 0;
cout << "线性表的长度为:" << L.length << endl;
cout << "线性表已清空!" << endl;
}
void DestoryList(SqList &L)
{
if (L.elem != NULL)
{
delete[] L.elem;
cout << "顺序表已销毁" << '\n';
}
system("pause");
}
4?? 求线性表L的长度
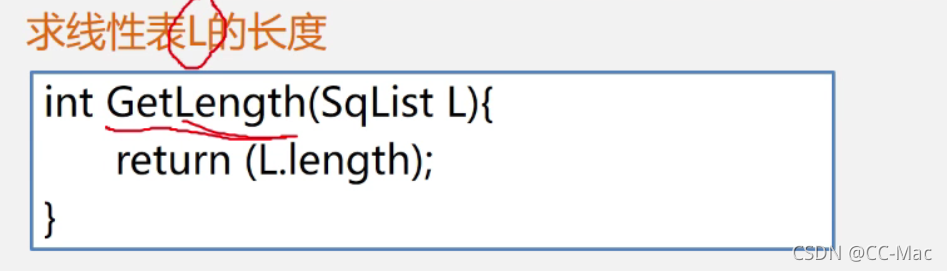
int GetLength(SqList &L)
{
return (L.length);
}
5?? 判断线性表是否为空 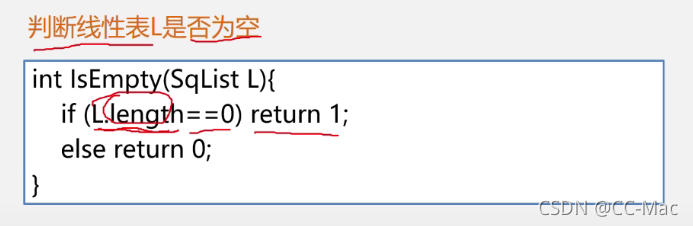
void IsEmpty(SqList &L)
{
if (L.length == 0)
{
cout << "当前线性表为空" << endl;
}
else
{
cout << "当前线性表不为空" << endl;
}
}
6?? 顺序表的取值
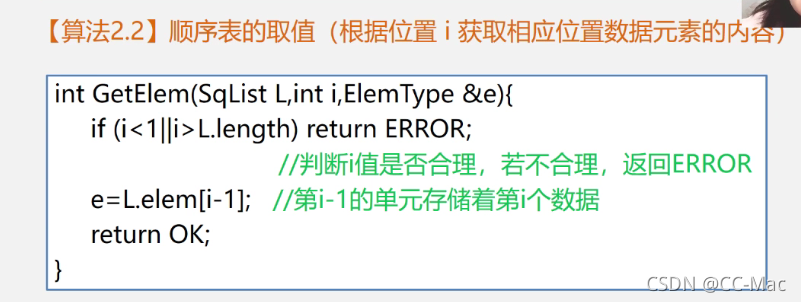
void GetElem(SqList &L)
{
int i = 0;
cout << "请输入要取出的元素位置:";
cin >> i;
if (i<1 || i>L.length)
{
cout << "取址位置有误" << endl;
}
else
{
int e = L.elem[i - 1];
cout << "取出的元素为:" << e << endl;
}
}
7?? 顺序表的查找
查找算法的基本操作:将记录的关键字同给定值进行比较(L.elem==e)

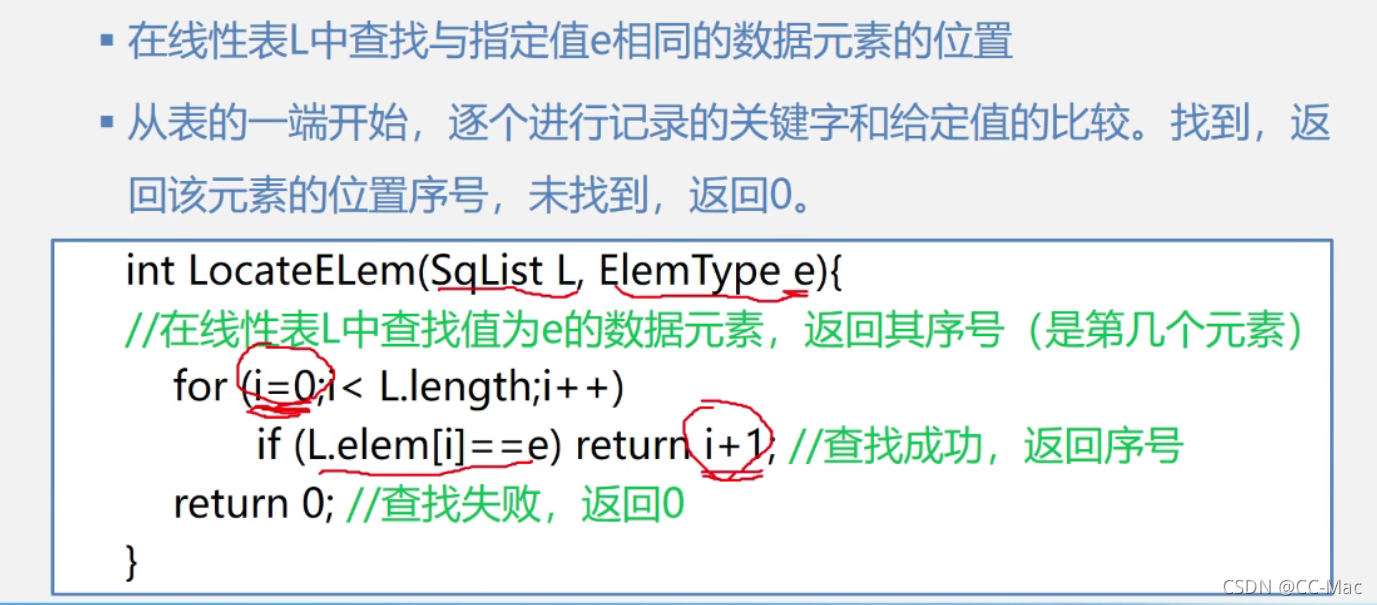
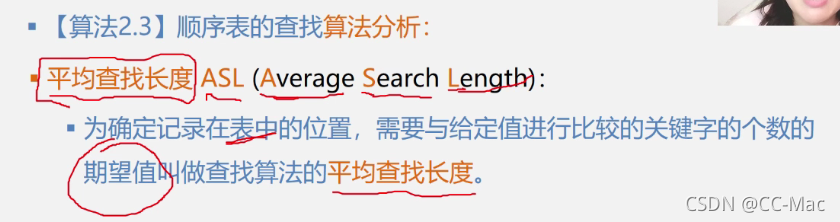 比较的次数与输入的定值e有关(假设7个数字出现的概率均为1/7) 当e=a,1次;当e=b,2次;当e=c,3次;…e=g,7次 平均比较次数(1+2+3+…+7)/7=4
在查找时,为确定元素在顺序表中的位置,需和给定值进行比较的数据元素个数的期望值称为查找算法在查找成功时的平均查找长度(AverageSearch Length, ASL)。 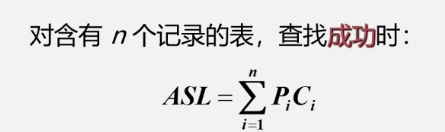 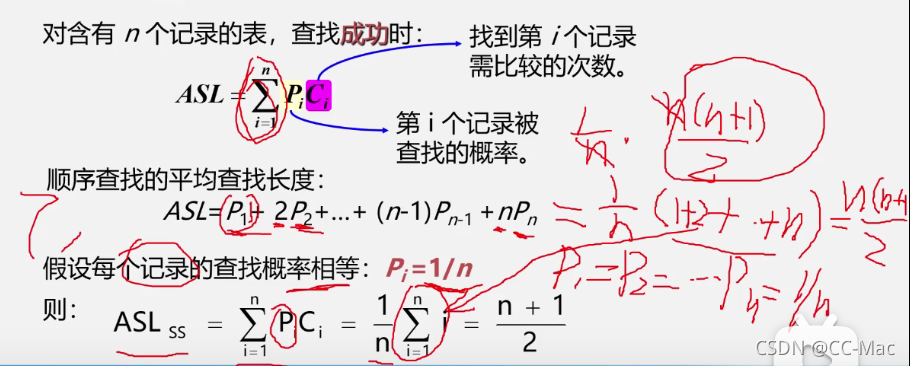
void LocateElem(SqList &L)
{
int e = 0;
int j = 0;
cout << "请输入需要查找的元素" << endl;
cin >> e;
while (j < L.length && L.elem[j] != e)
{
++j;
}
if (j < L.length)
{
cout << "该顺序表存在此元素,所找元素的位置为:" << j + 1 << "处";
}
else
{
cout << "所找的元素不存在";
}
cout << endl;
}
8?? 顺序表的插入 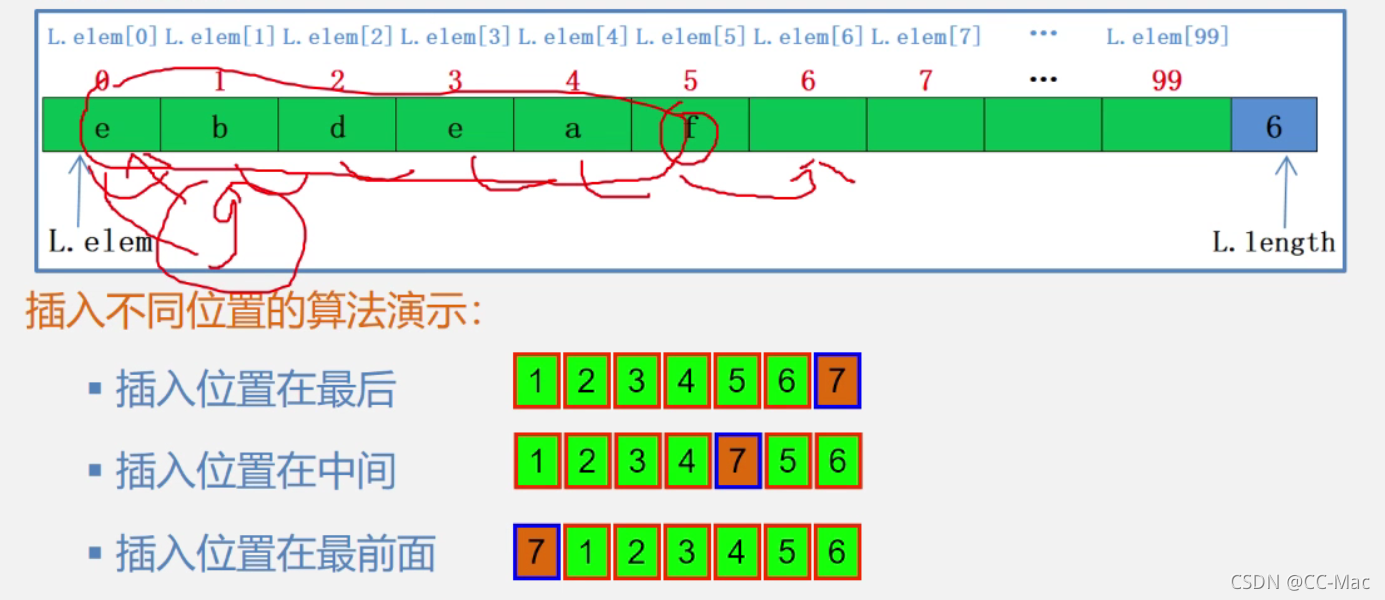 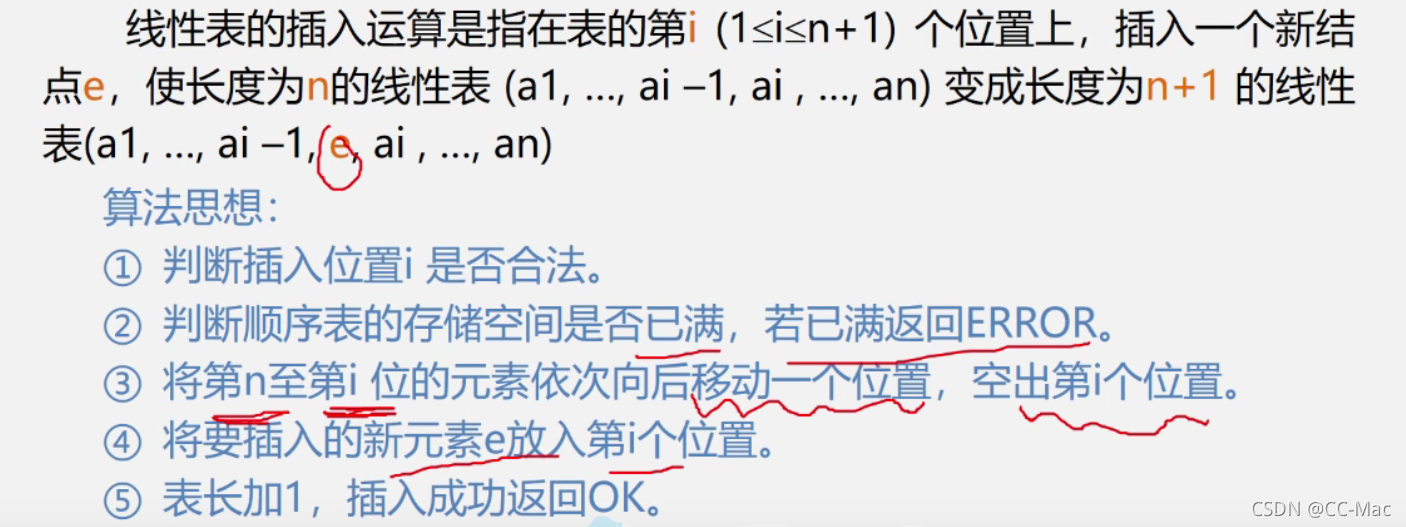
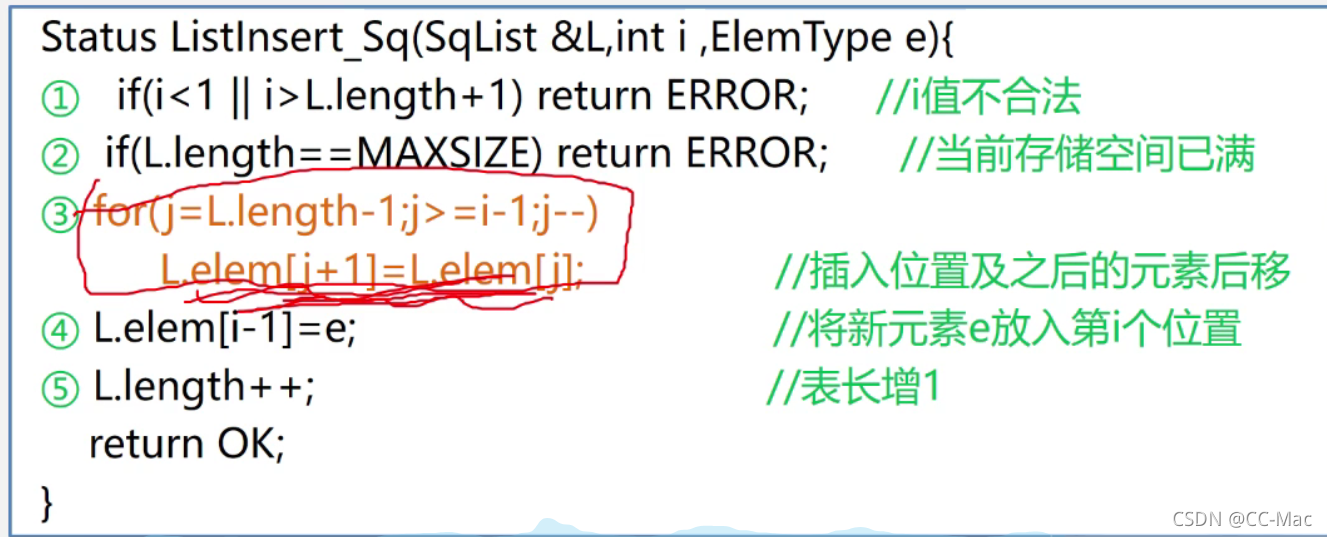 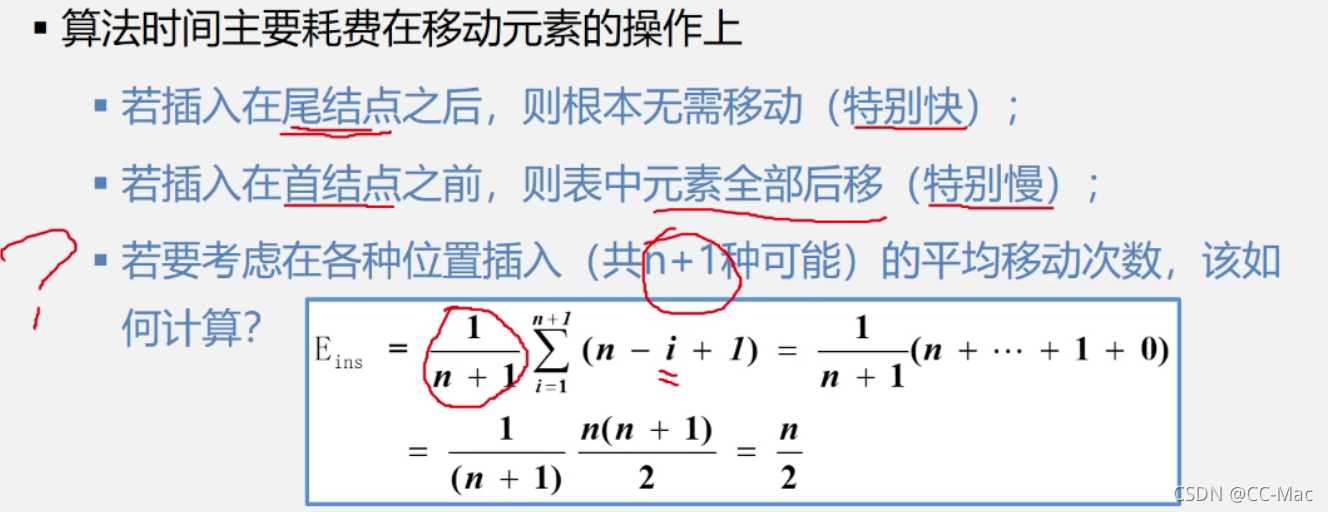
void ListInsert_Sq(SqList &L)
{
int pos = 0;
ElemType e = 0;
cout << "请输入要插入的位置:";
cin >> pos;
cout << "请输入插入的元素:";
cin >> e;
if (pos<1 || pos>L.length + 1)
{
cout << "插入不合法" << endl;
}
if (L.length == MAXSIZE)
{
cout << "当前存储空间已满,无法插入,请重新选择顺序表功能" << endl;
}
for (int i = L.length - 1; i >= pos - 1; i--)
{
L.elem[i + 1] = L.elem[i];
}
L.elem[pos - 1] = e;
++L.length;
cout << "插入后的顺序表长为:" << L.length << endl;
cout << "插入后的顺序表为:";
for (int i = 0; i < L.length; i++)
{
cout << L.elem[i] << " ";
}
cout << endl;
}
9?? 顺序表的删除 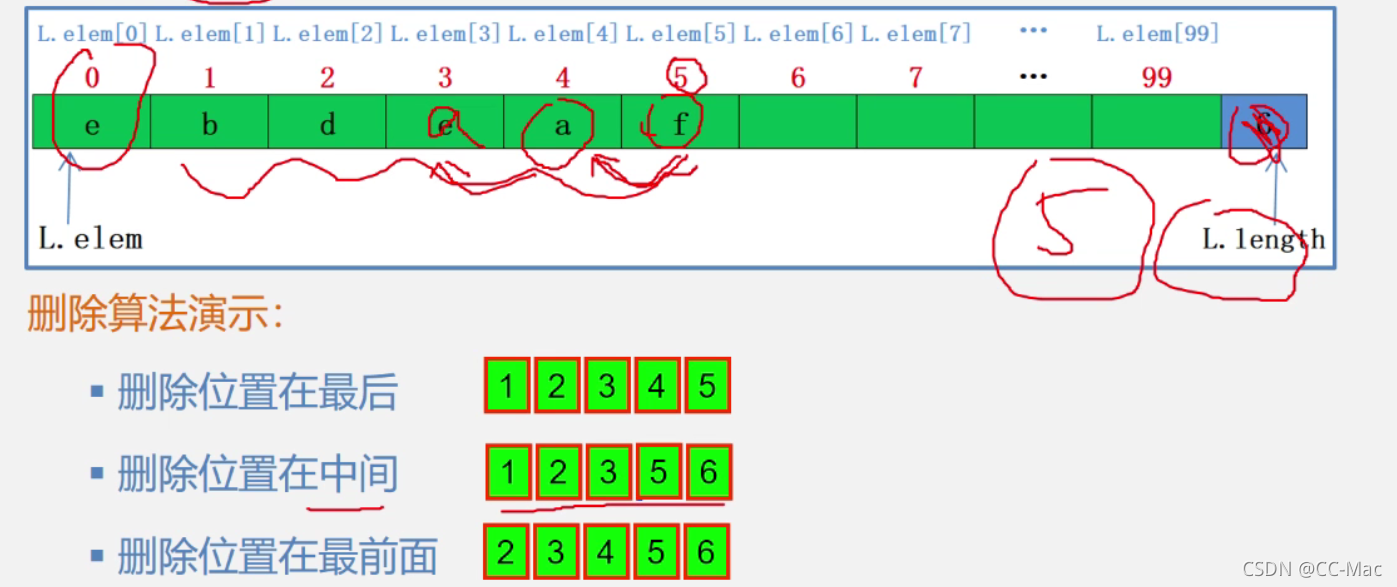 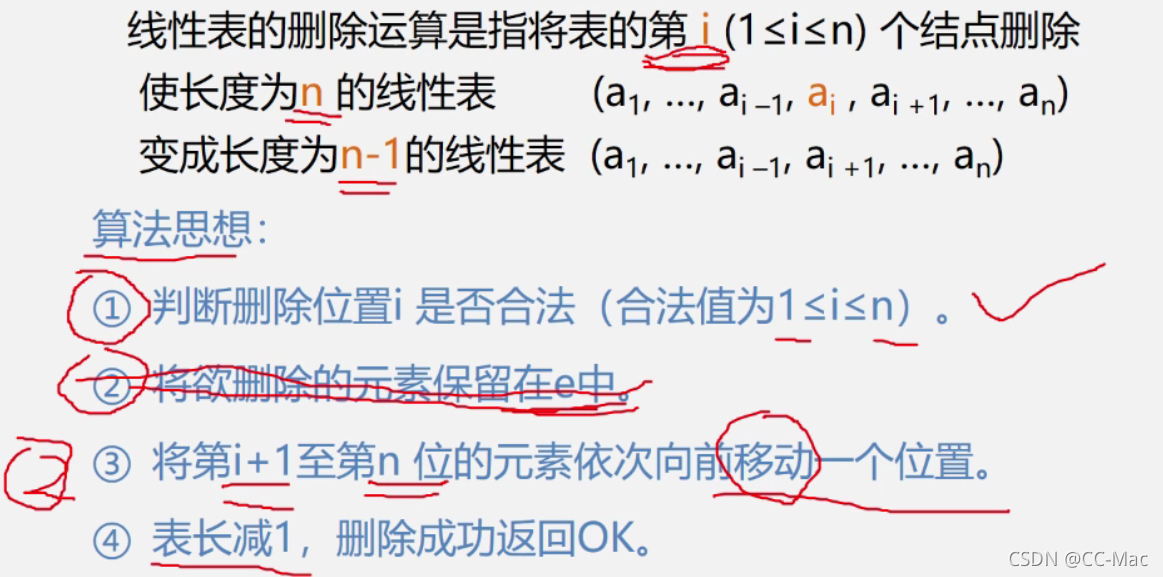 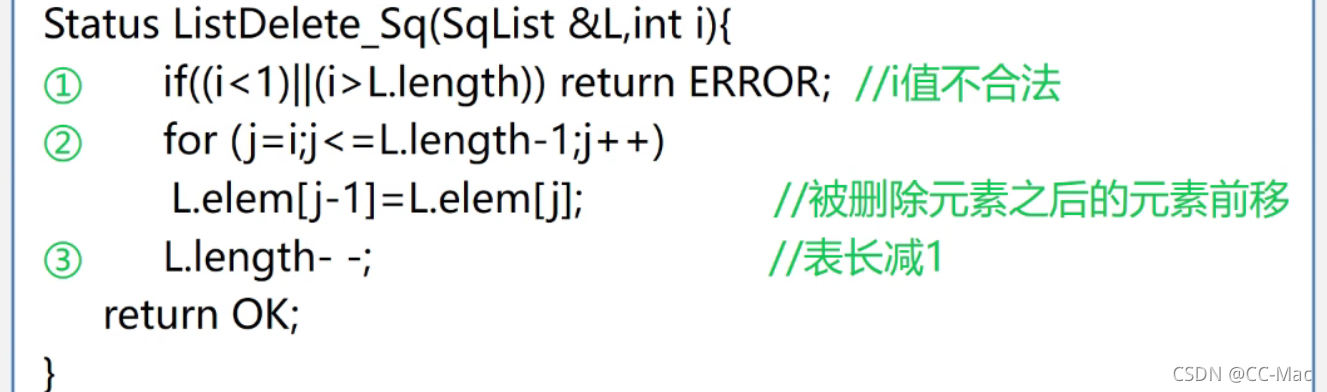 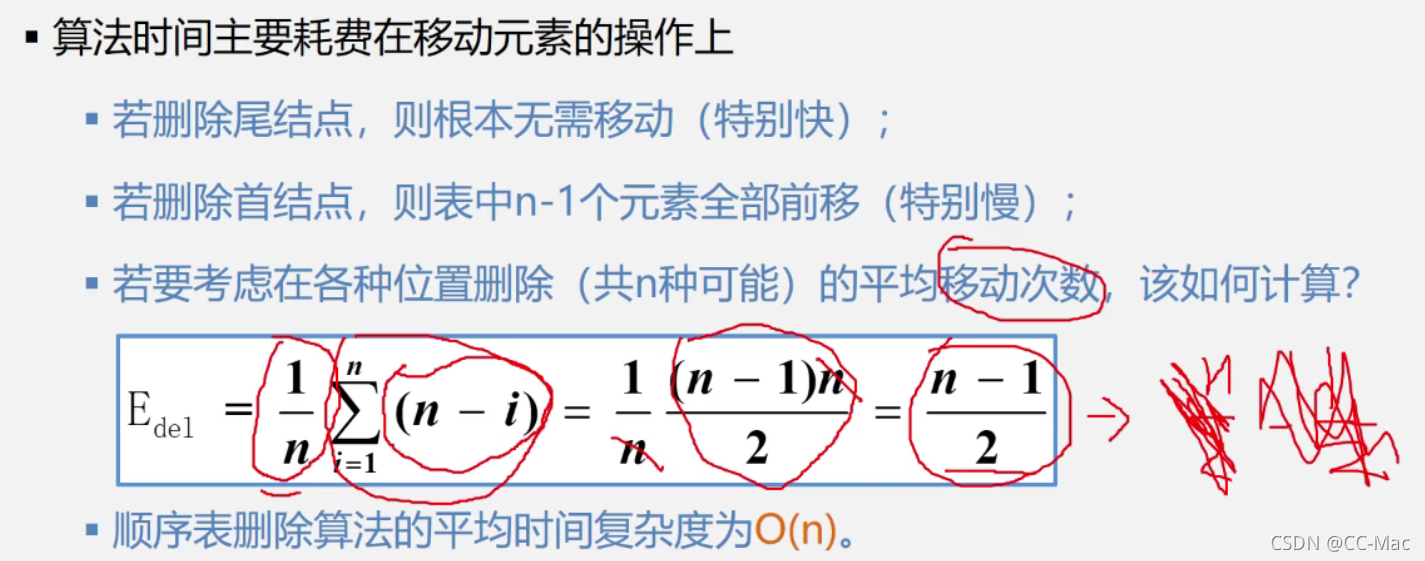
void ListDelete_Sq(SqList &L)
{
int pos = 0;
cout << "请输入要删除元素的位置:";
cin >> pos;
if (pos < 1 || pos>L.length)
{
cout << "删除位置不合法" << endl;
}
for (int i = pos; i <= L.length - 1; i++)
{
L.elem[i - 1] = L.elem[i];
}
L.length--;
cout << "插入后的顺序表长为:" << L.length << endl;
cout << "插入后的顺序表为:";
for (int i = 0; i < L.length; i++)
{
cout << L.elem[i] << " ";
}
cout << endl;
}
总结
期待大家和我交流,留言或者私信,一起学习,一起进步!
|