1.介绍
Java 集合框架 Java Collection Framework ,又被称为容器 container ,是定义在 java.util 包下的一组接口 interfaces 和其实现类 classes 。
1.1类和接口总览
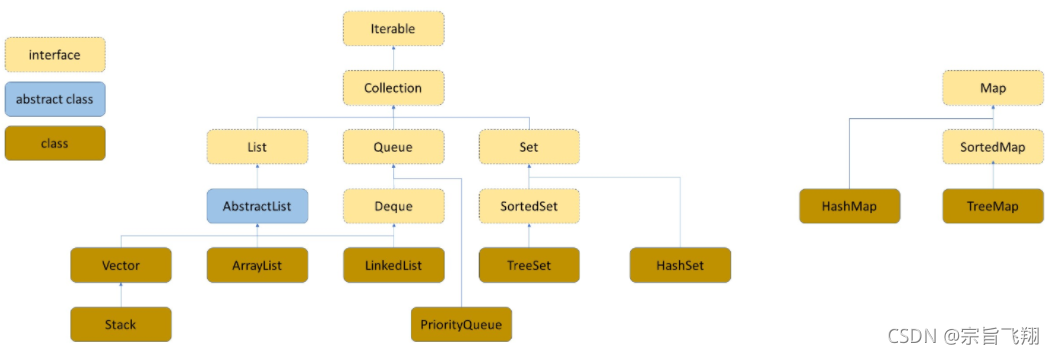
2.接口 interfaces
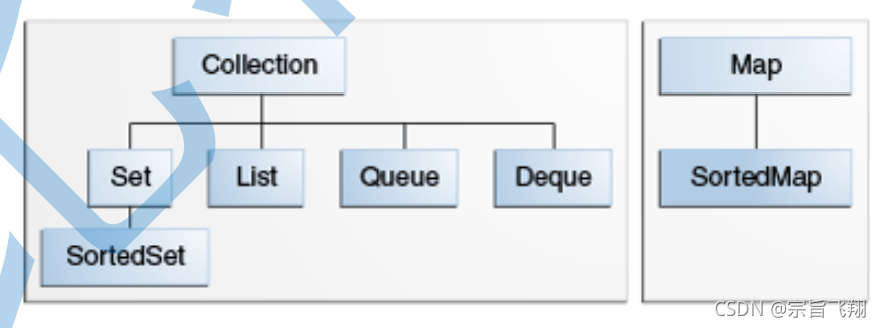
Collection :用来存储管理一组对象 objects ,这些对象一般被成为元素 elements
- Set: 元素不能重复,背后隐含着查找/搜索的语义
- SortedSet : 一组有序的不能重复的元素
- List : 线性结构
- Queue : 队列
- Deque : 双端队列
Map : 键值对 Key-Value-Pair ,背后隐含着查找/搜索 的语义
- SortedMap : 一组有序的键值对
2.1Collection 常用方法说明
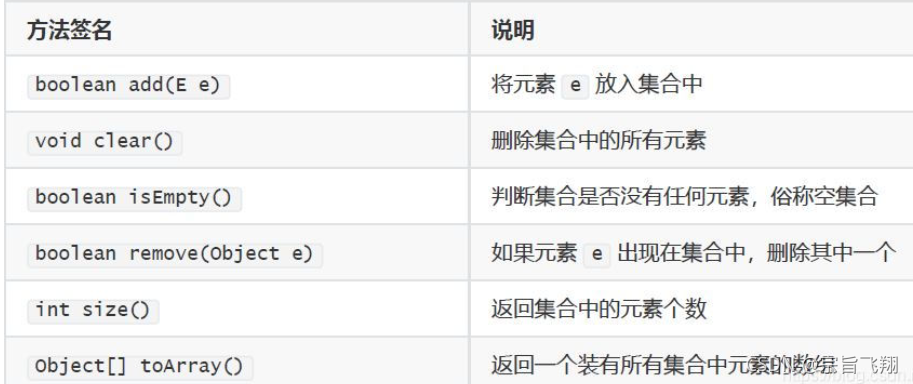
public class TestDemo {
public static void main(String[] args) {
Collection<String> collection = new ArrayList<>();
collection.add("hello");
collection.add("hello2");
Object[] objects = collection.toArray();
System.out.println(Arrays.toString(objects));
}
public static void main1(String[] args) {
Collection<String> collection = new ArrayList<>();
collection.add("hello");
collection.add("hello2");
Collection<Integer> collection1 = new ArrayList<>();
collection1.add(1);
collection1.add(2);
collection1.add(3);
}
}
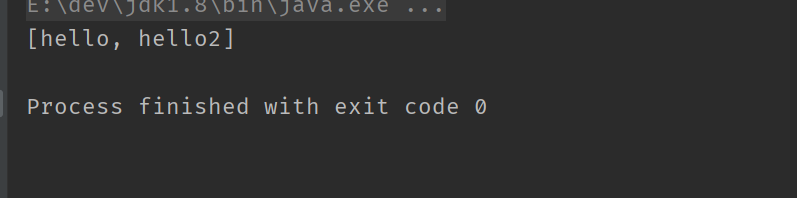
2.2Map 常用方法说明
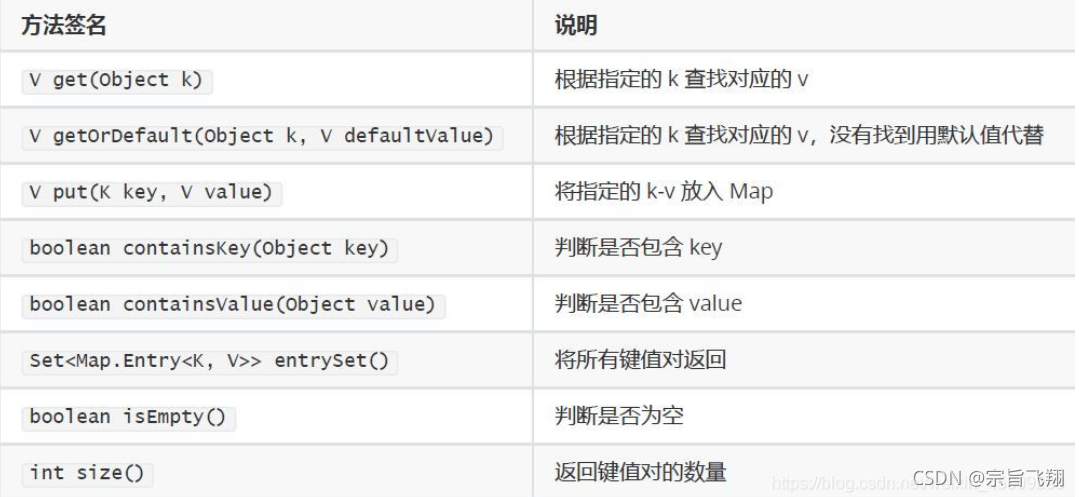
public class TestDemo2 {
public static void main(String[] args) {
Map<String, String> map2 = new TreeMap<>();
map2.put("国民女神", "高圆圆");
map2.put("及时雨", "宋江");
System.out.println(map2);
System.out.println("=======================");
}
public static void main2(String[] args) {
Map<String, String> map = new HashMap<>();
map.put("国民女神", "高圆圆");
map.put("及时雨", "宋江");
System.out.println(map);
System.out.println("=======================");
Set<Map.Entry<String, String>> entrySet = map.entrySet();
for (Map.Entry<String, String> entry : entrySet) {
System.out.println("key: " + entry.getKey() + "value: " + entry.getValue());
}
}
public static void main1(String[] args) {
Map<String, String> map = new HashMap<>();
map.put("国民女神", "高圆圆");
map.put("及时雨", "宋江");
System.out.println(map);
String ret = map.getOrDefault("及时雨", "xulin");
System.out.println(ret);
boolean flg = map.containsKey("国民女神");
System.out.println(flg);
Map<String, String> map2 = new TreeMap<>();
}
}
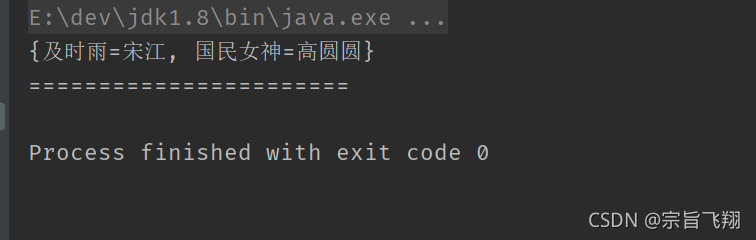
3.实现 classes

|