148. 排序链表
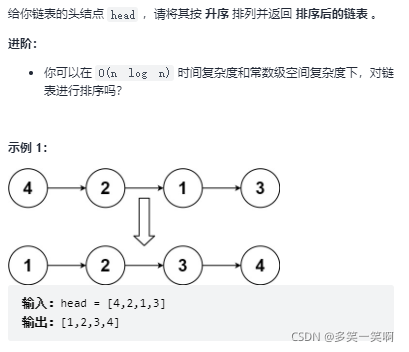
思路:递归实现链表归并排序,具体步骤见代码 注意:对于递归的理解还是不够透彻,可以将递归函数一直推算到极限,比如本题最终每个子链表分割到只有一个结点的时候,此时结合代码去思考。递归是一个套娃!递归到最小单位再思考怎么写代码,还要注意跳出递归的条件。 在合并队列的时候,要秉承由小到大的原则,所以每循环一次只加一个节点,另一个节点还要继续参与比较。 代码:
class Solution:
def sortList(self, head: Optional[ListNode]) -> Optional[ListNode]:
if not head or not head.next:
return head
fast=head.next
slow=head
while fast and fast.next:
slow=slow.next
fast=fast.next.next
mid=slow.next
slow.next=None
left=self.sortList(head)
right=self.sortList(mid)
h=res=ListNode(0)
while left and right:
if left.val < right.val:
h.next=left
left= left.next
else:
h.next=right
right=right.next
h=h.next
h.next=left if left else right
return res.next
206. 反转链表
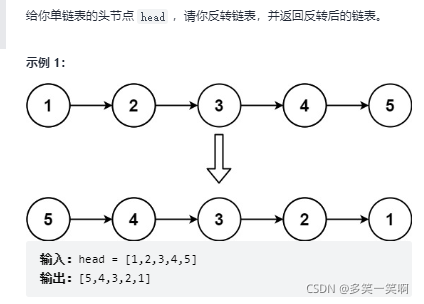
思路:迭代方法已经没问题,重点理解递归 注意:在迭代中,第一个节点的next一定要为None(设pre=None即可)。在递归中,每迭代一次都要将 head.next=None。 代码:
class Solution:
def reverseList(self, head: ListNode) -> ListNode:
pre=None
cur=head
while cur:
next=cur.next
cur.next=pre
pre=cur
cur=next
return pre
if not head or not head.next:
return head
newHead= reverseList(head.next)
head.next.next=head
head.next=None
return newHead
|