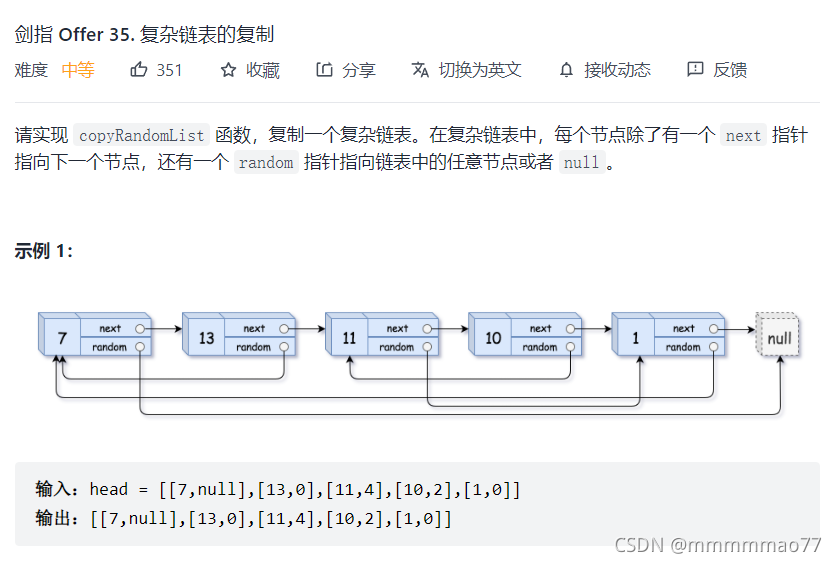
?
思路:这一题最开始没想出来,想了很久都觉得很复杂。看了答案有两个方法:
1.使用递归和哈希表,创建一个哈希表,key为原链表中的元素,value为复制链表中的元素。递归判断,是否存在key。
如果存在key 就说明已经创建了该复制节点,拿到复制节点。
如果不存在key,说明没有创建该复制节点,应该new一个节点,next 和random都调用递归方法拿到。最终拿到的headcopy就是满足题意的。
2.第二个方法使用复制双节点的方法,很巧妙。需要遍历三次:
首先第一次遍历,把链表每一个节点后面都插入一个复制节点,它的value与上一个节点相同。第二次遍历把复制节点random 是? 它上一个节点random的下一个。此时要注意random为 null的情况。第三次遍历把两个链表分开,分成两个。因为是复制,注意要还原原链表。
方法2
/*
// Definition for a Node.
class Node {
int val;
Node next;
Node random;
public Node(int val) {
this.val = val;
this.next = null;
this.random = null;
}
}
*/
class Solution {
public Node copyRandomList(Node head) {
if(head==null){
return null;
}
Node node =head;
while(head!=null){
Node temp = new Node(head.val);
temp.next=head.next;
head.next=temp;
head=head.next.next;
}
head=node;
while(head!=null){
if(head.random!=null){
head.next.random=head.random.next;
}else{
head.next.random=null;
}
head= head.next.next;
}
head=node;
Node nodehead=node;
node=node.next;
Node headcopy=node;
while(node.next!=null){
nodehead.next=nodehead.next.next;
node.next=node.next.next;
node=node.next;
nodehead=nodehead.next;
}
nodehead.next=nodehead.next.next;
return headcopy;
}
}
|