前言
学校JAVA实验13
一、要求是什么?
学生成绩保存在score3.txt中,输入学生姓名,输出各科成绩和总成绩, 以及各科成绩排名和总成绩的排名。
要求:
1) 使用LinkedList<E>或ArrayList<E>泛型类和Iterator<E>迭代器类来
查找成绩和确定成绩排名。参照课本15.2节的内容。
2) 如果没有该学生的成绩,则给出提示信息.
二、思路
- 先构建一个结构体学生,它包含名字成绩和排名
- 然后构建四个比较器,方便之后将链表分别按成绩和总分排序( 不懂比较器的可以点击这里参考我的另外一篇博客)当然下面的代码我也写了注释
- 然后建立文件,判断文件是否可读写
- 接着建立结构体链表
- 将第一行读入舍去,然后接着读入每一行,每读一行建立一个结构体,将名字,成绩读入。然后加入链表
- 然后根据建立的四个比较器,排序四次,每一次排序,都便利一遍链表,将遍历的下标也就是排名,记录下来
- 基本就完成了,然后输入一个名字,遍历一次链表就行,如果存在就输出,不存在就输出对应的话
三代码实现
代码如下
import java.util.*;
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
import java.io.Reader;
import java.io.Writer;
import java.util.Scanner;
public class exex {
public static class Comparator2 implements Comparator<student>{
@Override
public int compare(student b,student a) {
return (int)(a.shu - b.shu);
}
}
public static class Comparator1 implements Comparator<student>{
@Override
public int compare(student b,student a) {
return (int)(a.yu - b.yu);
}
}
public static class Comparator3 implements Comparator<student>{
@Override
public int compare(student b,student a) {
return (int)(a.wai - b.wai);
}
}
public static class Comparator4 implements Comparator<student>{
@Override
public int compare(student b,student a) {
return (int)(a.zong - b.zong);
}
}
public static class student{
String name;
double yu,shu,wai,zong;
int ryu,rshu,rwai,rzong;
student(){}
}
public static void main(String[] args) {
File f = new File("D:\\学习\\Java实验\\Java实验要求\\13 实验十三 成绩统计4-链表\\13 实验十三 成绩统计4-链表","score3.txt");;
String str= f.getName();
System.out.println("文件的名字:"+str);
if(f.exists()){
System.out.println("文件是存在的");
}
else{
System.out.println("文件是不存在的");
}
if(f.canRead()){
System.out.println("文件是可读的");
}
else{
System.out.println("文件是不可读的");
}
if(f.canWrite()){
System.out.println("文件是可写的");
}
else{
System.out.println("文件是不可写的");
}
System.out.println("文件的长度:"+f.length());
System.out.println("文件的父路径"+f.getAbsolutePath());
try {
LinkedList<student> stu = new LinkedList<student>();
Reader in = new FileReader("D:\\\\学习\\\\Java实验\\\\Java实验要求\\\\13 实验十三 成绩统计4-链表\\\\13 实验十三 成绩统计4-链表\\score3.txt");
BufferedReader in1 = new BufferedReader(in);
String str1 = in1.readLine();
String str2 = null;
boolean f3 = false;
while((str2=in1.readLine())!=null) {
int num=0;
student sd;
sd= new student();
Scanner cin = new Scanner(str2);
if(cin.hasNext()) {
String str8 = cin.next();
if(str8.equals("平均")) {break;}
System.out.println(str8);
sd.name=str8;
}
cin.useDelimiter("[^0123456789.]+");
while(cin.hasNextInt()) {
double price = cin.nextInt();
System.out.println(price);
if(num==0) {
sd.yu=price;
}else if(num==1) {
sd.shu=price;
}else if(num==2) {
sd.wai=price;
}else if(num==3) {
sd.zong=price;
}
num++;
}
stu.add(sd);
num=0;
}
in1.close();
in.close();
Iterator<student> iter = stu.iterator();
Collections.sort(stu, new Comparator1());
iter = stu.iterator();
int nn=1;
while(iter.hasNext()) {
student st=iter.next();
st.ryu=nn;
nn++;
}
Collections.sort(stu, new Comparator2());
iter = stu.iterator();
nn=1;
while(iter.hasNext()) {
student st=iter.next();
st.rshu=nn;
nn++;
}
Collections.sort(stu, new Comparator3());
iter = stu.iterator();
nn=1;
while(iter.hasNext()) {
student st=iter.next();
st.rwai=nn;
nn++;
}
Collections.sort(stu, new Comparator4());
iter = stu.iterator();
nn=1;
while(iter.hasNext()) {
student st=iter.next();
st.rzong=nn;
nn++;
}
while(true) {
Scanner c = new Scanner(System.in);
String s = c.next();
iter = stu.iterator();
boolean f1 = false;
while(iter.hasNext()) {
student st=new student();
st=iter.next();
if(s.equals(st.name)){
f1=true;
System.out.println(s+"的语文,数学,外语,总分成绩为:"+st.yu+","+st.shu+","+st.wai+","+st.zong);
System.out.println(s+"的语文,数学,外语, 总成绩的排名为:"+st.ryu+","+st.rshu+","+st.rwai+","+st.rzong);
}
}
if(!f1) {
System.out.println(s+"同学不存在");
}
}
}catch(IOException e){
e.printStackTrace();
System.out.println("不能打开文件");
}
}
}
结果截图
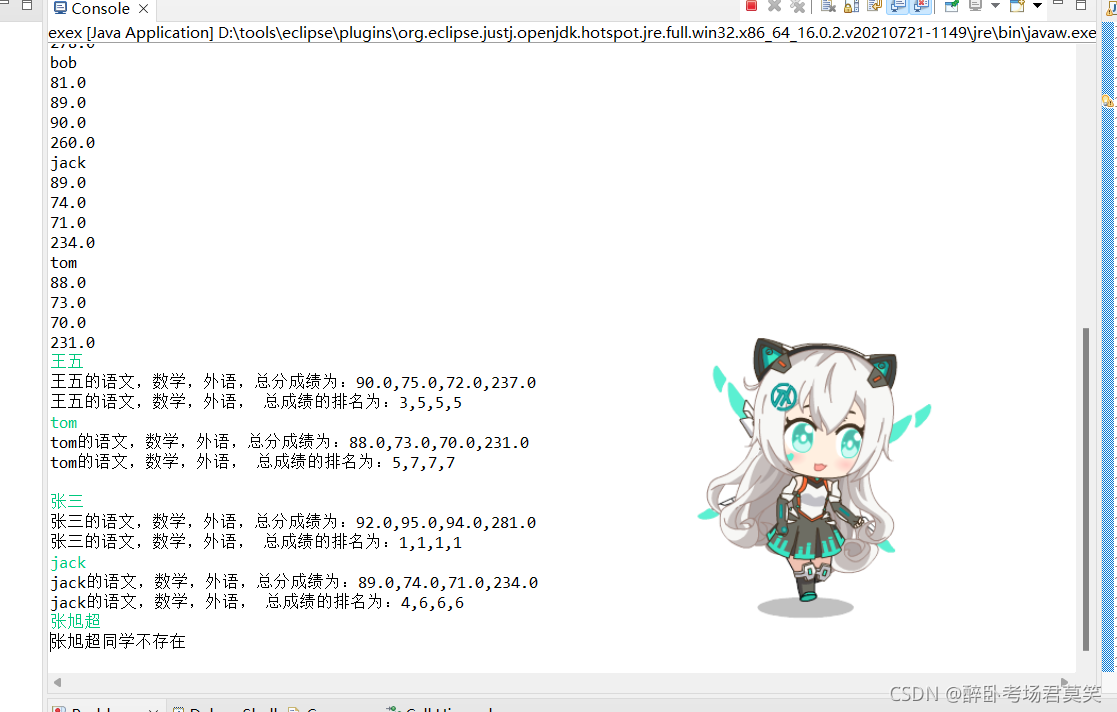
总结
有一些小细节还是要注意的,像退出循环的条件啊 排序后记录排名啊重载运算符啊。
|