九、弗洛伊德算法
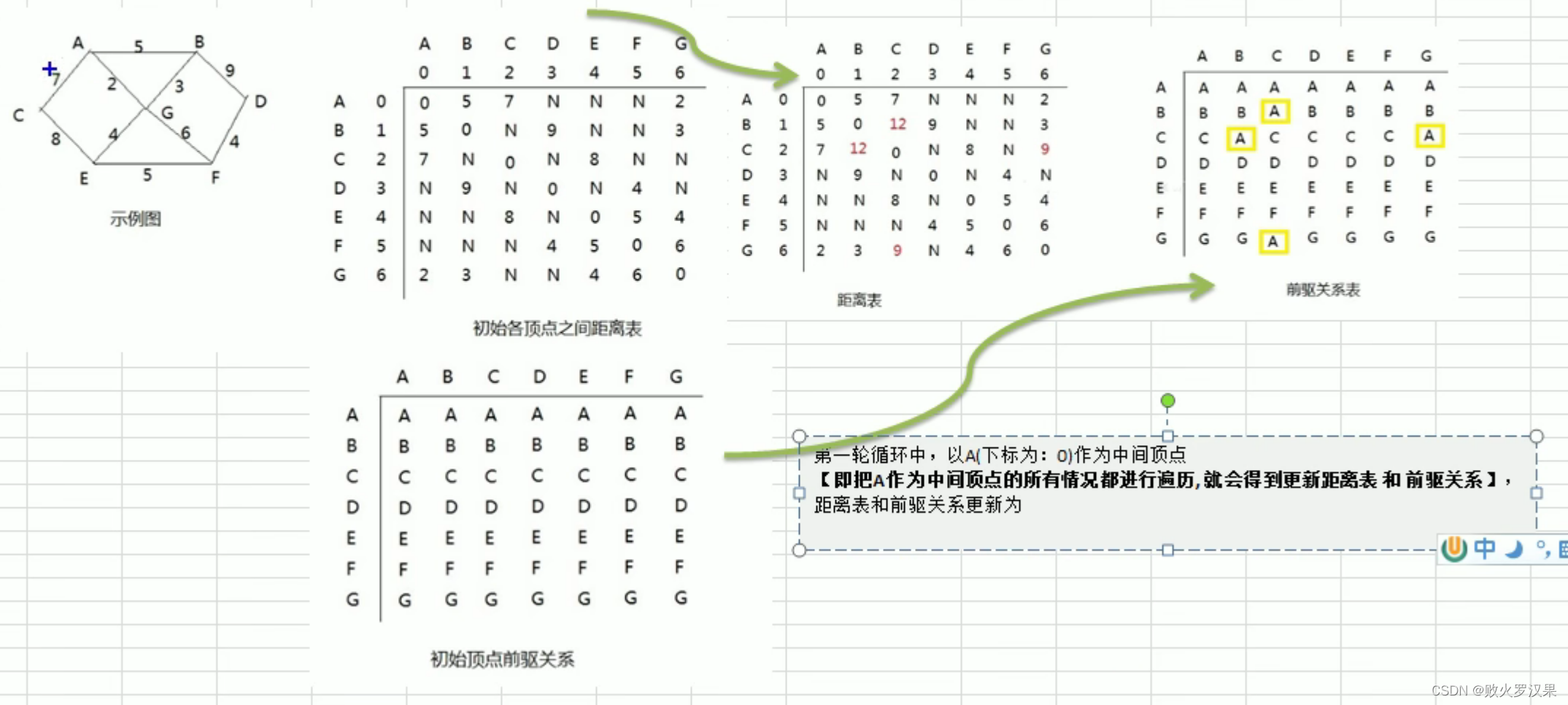 代码实现:
package Algorithm.Floyd;
public class Floyd {
public static void main(String[] args) {
char[] vertex = {'A', 'B', 'C', 'D', 'E', 'F', 'G'};
int[][] matrix = new int[vertex.length][vertex.length];
final int N = 65535;
matrix[0] = new int[]{0, 5, 7, N, N, N, 2};
matrix[1] = new int[]{5, 0, N, 9, N, N, 3};
matrix[2] = new int[]{7, N, 0, N, 8, N, N};
matrix[3] = new int[]{N, 9, N, 0, N, 4, N};
matrix[4] = new int[]{N, N, 8, N, 0, 5, 4};
matrix[5] = new int[]{N, N, N, 4, 5, 0, 6};
matrix[6] = new int[]{2, 3, N, N, 4, 6, 0};
Graph graph = new Graph(vertex.length, matrix, vertex);
graph.floyd();
graph.show();
}
}
class Graph {
char[] vertex;
int[][] pre;
int[][] dis;
public Graph(int length, int[][] matrix, char[] vertex) {
this.vertex = vertex;
this.dis = matrix;
this.pre = new int[length][length];
for (int i = 0; i < length; i++) {
for (int j = 0; j < length; j++) {
pre[i][j] = i;
}
}
}
public void show() {
for (int i = 0; i < dis.length; i++) {
for (int j = 0; j < dis.length; j++) {
System.out.print(vertex[pre[i][j]]+" ");
}
System.out.println();
for (int j = 0; j < dis.length; j++) {
System.out.print(dis[i][j]+" ");
}
System.out.println();
}
}
public void floyd(){
int len = 0;
for (int k = 0; k < dis.length; k++) {
for (int i = 0; i < dis.length; i++) {
for (int j = 0; j < dis.length; j++) {
len = dis[i][k]+dis[k][j];
if (len<dis[i][j]){
dis[i][j] = len;
pre[i][j] = pre[k][j];
}
}
}
}
}
}
十、骑士周游问题
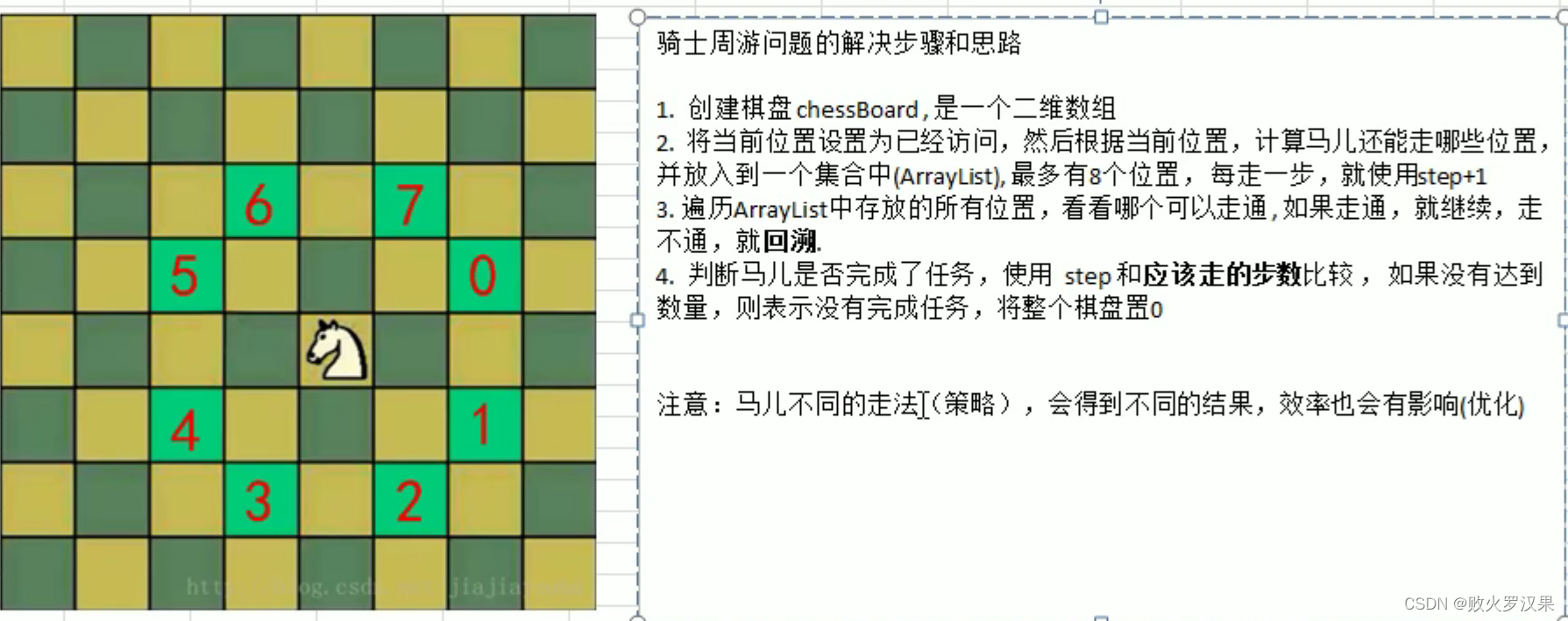 其他思路:不在同行同列同对角线的点 策略优化:选择下一步的下一步选择越少的,效率越高 代码实现:
package Algorithm.Horse;
import java.awt.*;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Comparator;
public class Horse {
public static int X;
public static int Y;
public static boolean[] visited;
public static boolean finished;
public static void main(String[] args) {
Y = 8;
X = 8;
int row = 1;
int column = 1;
int[][] board = new int[Y][X];
visited = new boolean[Y * X];
travel(board, row - 1, column - 1, 1);
for (int[] ints : board) {
System.out.println(Arrays.toString(ints));
}
}
public static void travel(int[][] board, int row, int column, int step) {
board[row][column] = step;
visited[row * X + column] = true;
ArrayList<Point> ps = next(new Point(column, row));
sort(ps);
while (!ps.isEmpty()) {
Point p = ps.remove(0);
if (!visited[p.y * X + p.x]) {
travel(board, p.y, p.x, step + 1);
}
}
if (step < X * Y && !finished) {
board[row][column] = 0;
visited[row * X + column] = false;
} else {
finished = true;
}
}
public static ArrayList<Point> next(Point curPoint) {
ArrayList<Point> ps = new ArrayList<>();
Point p1 = new Point();
if ((p1.x = curPoint.x - 2) >= 0 && (p1.y = curPoint.y - 1) >= 0) {
ps.add(new Point(p1));
}
if ((p1.x = curPoint.x - 1) >= 0 && (p1.y = curPoint.y - 2) >= 0) {
ps.add(new Point(p1));
}
if ((p1.x = curPoint.x + 1) < X && (p1.y = curPoint.y - 2) >= 0) {
ps.add(new Point(p1));
}
if ((p1.x = curPoint.x + 2) < X && (p1.y = curPoint.y - 1) >= 0) {
ps.add(new Point(p1));
}
if ((p1.x = curPoint.x + 2) < X && (p1.y = curPoint.y + 1) < Y) {
ps.add(new Point(p1));
}
if ((p1.x = curPoint.x + 1) < X && (p1.y = curPoint.y + 2) < Y) {
ps.add(new Point(p1));
}
if ((p1.x = curPoint.x - 1) >= 0 && (p1.y = curPoint.y + 2) < Y) {
ps.add(new Point(p1));
}
if ((p1.x = curPoint.x - 2) >= 0 && (p1.y = curPoint.y + 1) < Y) {
ps.add(new Point(p1));
}
return ps;
}
public static void sort(ArrayList<Point> ps){
ps.sort(new Comparator<Point>() {
@Override
public int compare(Point o1, Point o2) {
return next(o1).size()-next(o2).size();
}
});
}
}
|