1. 输入三角形的三边a,b,c,计算三角形的面积的公式是area=

s=(a+b+c)/2构成三角形的条件是: a+b>c, b+c>a, c+a>b
编写程序,输入a,b,c,检查a,b,c是否满足以上条件,如不满足,由cerr输出有关出错信息。
?
#include <iostream>
using namespace std;
class Triangle {
public:
double a, b, c, s, area;
void SetTriangle();
void IsTriangle();
};
void Triangle::SetTriangle()
{
cin >> a >> b >> c;
}
void Triangle::IsTriangle()
{
if ((a + b) <= c || (b + c) <= a || (c + a) <= b) {
cerr << "输入不合法" << endl;
}
else
{
s = (a + b + c) / 2;
area = sqrt(s * (s - a) * (s - b) * (s - c));
cout << "area=" << area << endl;
}
}
int main()
{
Triangle t;
t.SetTriangle();
t.IsTriangle();
}
2. 从键盘输入一批数值,要求保留3位小数,在输出时上下行小数点对齐。
#include <iostream>
#include <iomanip>
using namespace std;
int main()
{
float a[5];
int i = 0;
for (i = 0; i < 5; i++) {
cin >> a[i];
}
cout << setiosflags(ios::fixed) << setprecision(3);
for (i = 0; i < 5; i++) {
cout << setw(10) << a[i] << endl;
}
}
3.编程序,在显示屏上显示一个由字母B组成的三角形。
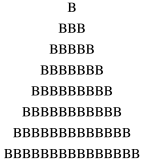
?
#include <iostream>
#include<iomanip>
#pragma warning(disable:26451)
using namespace std;
int main()
{
int size = 0;
cin >> size;
for (int i = 1; i < size; i++) {
cout << setw(10 - i) << setfill(' ') << " " << setw(2 * i - 1) << setfill('B') << "B" << endl;
}
}
4.?(简答题)
建立两个磁盘文件f1.dat和f2.dat,编程序实现以下工作:?
(1) 从键盘输入20个整数,分别存放在两个磁盘文件中(每个文件中放10个整数);
(2) 从f1.dat读入10个数,然后存放到f2.dat文件原有数据的后面;
(3) 从f2.dat中读入20个整数,将它们按从小到大的顺序存放到f2.dat(不保留原来的数据)。
#include <iostream>
#include <fstream>
using namespace std;
void Set1()
{
int a[10];
ofstream outfile1("f1.dat");
ofstream outfile2("f2.dat");
cout << "请输入10个整形数字";
for (int i = 0; i < 10; i++){
cin >> a[i];
outfile1 << a[i] << " ";
}
cout << "请输入10个整形数字";
for (int i = 0; i < 10; i++){
cin >> a[i];
outfile2 << a[i] << " ";
}
outfile1.close();
outfile2.close();
}
void Set2()
{
ifstream infile("f1.dat");
ofstream outfile("f2.dat", ios::app);
int a;
for (int i = 0; i < 10; i++)
{
infile >> a;
outfile << a << " ";
}
infile.close();
outfile.close();
}
void Set3()
{
ifstream infile("f2.dat");
int a[20];
int num = sizeof(a) / sizeof(a[0]);
int temp = 0;
for (int i = 0; i < num; i++) {
infile >> a[i];
}
for (int i = 0; i < num-1; i++) {
for (int j = 0; j < num -1 - i; j++) {
if (a[j] > a[j + 1])
{
temp = a[j];
a[j] = a[j + 1];
a[j + 1] = temp;
}
}
}
infile.close();
ofstream outfile("f2.dat", ios::out);
for (int i = 0; i < 20; i++){
outfile << a[i] << " ";
cout << a[i] << " ";
}
outfile.close();
}
int main()
{
Set1();
Set2();
Set3();
return 0;
}
5.?(简答题)
编程序实现以下功能:?
(1) 按职工号由小到大的顺序将5个员工的数据(包括号码、姓名、年龄、工资)输出到磁盘文件中保存。
(2) 从键盘输入两个员工的数据(职工号大于已有的职工号),增加到文件的末尾。
(3) 输出文件中全部职工的数据。
(4) 从键盘输入一个号码,从文件中查找有无此职工号,如有则显示此职工是第几个职工,以及此职工的全部数据。如没有,就输出“无此人”。可以反复多次查询,如果输入查找的职工号为0,就结束查询。
#include<iostream>
#include<fstream>
using namespace std;
struct worker
{
int num;
char name[20];
int age;
double pay;
};
int main()
{
worker wk[7] =
{
2020033193, "xi",20,2300,
2020033196, "yang", 21, 1555.5,
2020033191, "wang", 18, 2000.1,
2020033194, "Xu", 17, 3000.1,
2020033192, "zhang", 18, 666.6,
}, wk1;
ofstream outfile1("worker.dat");
ofstream outfile("worker.dat", ios::out);
fstream iofile("worker.dat", ios::in | ios::out | ios::binary);
int i, m, num,temp1,temp2;
double temp3;
char arr[20];
cout << "五个员工" << endl;
for (int i = 0; i < 5 - 1; i++) {
for (int j = 0; j < 5 - i - 1; j++) {
if (wk[j].num > wk[j + 1].num){
temp1 = wk[j + 1].num;
wk[j + 1].num = wk[j].num;
wk[j].num = temp1;
strcpy_s(arr, wk[j + 1].name);
strcpy_s(wk[j + 1].name, wk[j].name);
strcpy_s(wk[j].name, arr);
temp2 = wk[j + 1].age;
wk[j + 1].age = wk[j].age;
wk[j].age = temp2;
temp3 = wk[j + 1].pay;
wk[j + 1].pay = wk[j].pay;
wk[j].pay = temp3;
}
}
}
for (i = 0; i < 5; i++){
cout << wk[i].num << " " << wk[i].name << " " << wk[i].age << " " << wk[i].pay << endl;
iofile.write((char*)&wk[i], sizeof(wk[i]));
}
cout << "请输入您要插入的2个员工数据:" << endl;
iofile.seekp(0, ios::end);
for (i = 0; i < 2; i++){
cin >> wk1.num >> wk1.name >> wk1.age >> wk1.pay;
iofile.write((char*)&wk1, sizeof(wk1));
}
cout << "七个员工" << endl;
iofile.seekg(0, ios::beg);
for (i = 0; i < 7; i++){
iofile.read((char*)&wk[i], sizeof(wk[i]));
cout << wk[i].num << " " << wk[i].name << " " << wk[i].age << " " << wk[i].pay << endl;
}
bool find;
cout << "输入要搜索的员工号,输入0停止。";
cin >> num;
while (num)
{
find = false;
iofile.seekg(0, ios::beg);
for (i = 0; i < 7; i++)
{
iofile.read((char*)&wk[i], sizeof(wk[i]));
if (num == wk[i].num)
{
m = iofile.tellg();
cout << num << " 不是" << m / sizeof(wk1) << endl;
cout << wk[i].num << " " << wk[i].name << " " << wk[i].age << " " << wk[i].pay << endl;
find = true;
break;
}
}
if (!find)
cout << "没找到" << endl;
cout << "输入要搜索的员工号,输入0停止。";
cin >> num;
}
iofile.close();
return 0;
}
6.?(简答题)?在例7.17的基础上,修改程序,将存放在c数组中的数据读入并显示出来。
#include<iostream>
#include<sstream>
#include<cstring>
using namespace std;
struct student
{
int num;
char name[20];
double score;
};
int main()
{
student stud[3] =
{
1001,"Li",78,
1002,"Wang",89.5,
1004,"Fang",90
}, stud1[3];
char c[50] = { 0 };
int i;
ostringstream strout;
for (i = 0; i < 3; i++){
strout << stud[i].num << " " << stud[i].name << " " << stud[i].score << " ";
}
strout << ends;
strcpy_s(c, strout.str().c_str());
cout << "array c:" << endl << c << endl << endl;
istringstream strin(c);
for (i = 0; i < 3; i++){
strin >> stud1[i].num >> stud1[i].name >> stud1[i].score;
}
cout << "data from array c to array stud1:" << endl;
for (i = 0; i < 3; i++){
cout << stud1[i].num << " " << stud1[i].name << " " << stud1[i].score << endl;
}
cout << endl;
return 0;
}
|