92. 反转链表 II(C题解版含VS可运行源程序)
1.题解
- 先设置虚拟头节点便于处理m=1的情况,
- 然后找到第m个节点的前一位,反转m~n之间,
- 注意最后还要把反转之后节点指向剩余的节点。
2.力扣C源码
struct ListNode* reverseBetween(struct ListNode* head, int left, int right){
if (left == right)
return head;
struct ListNode* p = (struct ListNode*)malloc(sizeof(struct ListNode));
p->next = head;
head = p;
right = right - left + 1;
while (left-- > 1) {
p = p->next;
}
struct ListNode* q = NULL;
struct ListNode* cur = p->next;
struct ListNode* next = NULL;
while (right-- > 0 && cur != NULL) {
next = cur->next;
cur->next = q;
q = cur;
cur = next;
}
p->next = q;
while (q->next != NULL) {
q = q->next;
}
q->next = next;
return head->next;
}
3.VS可运行源程序
#include<stdio.h>
#include<stdlib.h>
#include<iostream>
#pragma warning(disable:4996)
using namespace std;
struct ListNode {
int val;
struct ListNode* next;
};
void List_TailInsert(ListNode** head)
{
*head = (ListNode*)malloc(sizeof(ListNode));
ListNode* rear = *head;
ListNode* p;
int num;
scanf("%d", &num);
while (num != 9999) {
p = (ListNode*)malloc(sizeof(ListNode));
p->val = num;
rear->next = p;
rear = p;
scanf("%d", &num);
}
rear->next = NULL;
}
struct ListNode* reverseBetween(struct ListNode* head, int left, int right) {
ListNode* head2 = head->next;
if (left == right) {
return head2;
}
ListNode* p = (ListNode*)malloc(sizeof(ListNode));
p->next = head2;
head2 = p;
right = right - left + 1;
while (left-- > 1) {
p = p->next;
}
ListNode* q = NULL;
ListNode* cur = p->next;
ListNode* next = NULL;
while (right-- > 0 && cur != NULL) {
next = cur->next;
cur->next = q;
q = cur;
cur = next;
}
p->next = q;
while (q->next != NULL) {
q = q->next;
}
q->next = next;
return head2->next;
}
int main()
{
printf("输入链表数据(以9999结束):");
ListNode* head;
List_TailInsert(&head);
printf("输入要反转部分的左右边界:");
int left, right;
scanf("%d %d", &left, &right);
ListNode* head3 = reverseBetween(head, left, right);
ListNode* temp = head3;
printf("反转后的链表:");
while (temp) {
printf("%d ", temp->val);
temp = temp->next;
}
printf("\n");
system("pause");
return 0;
}
4.运行结果截图
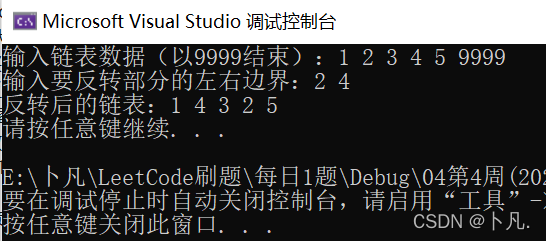
|