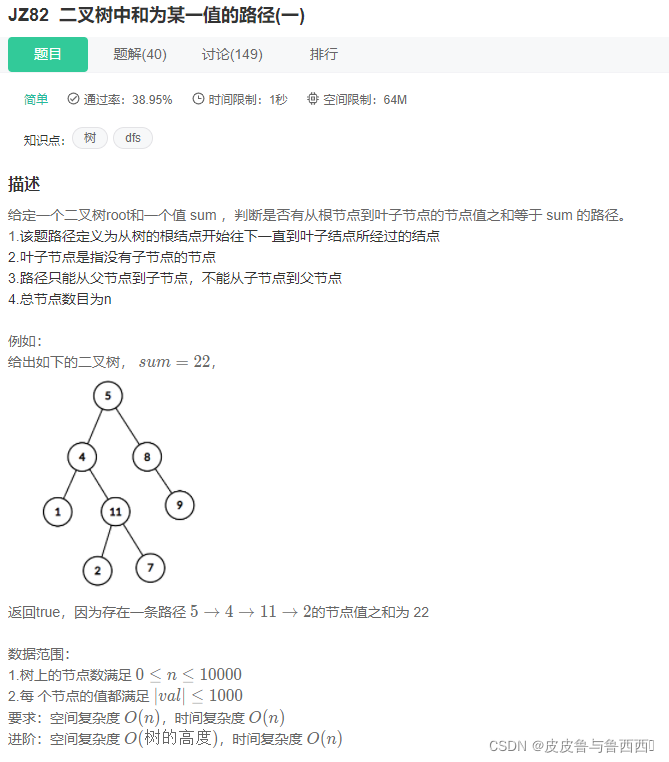
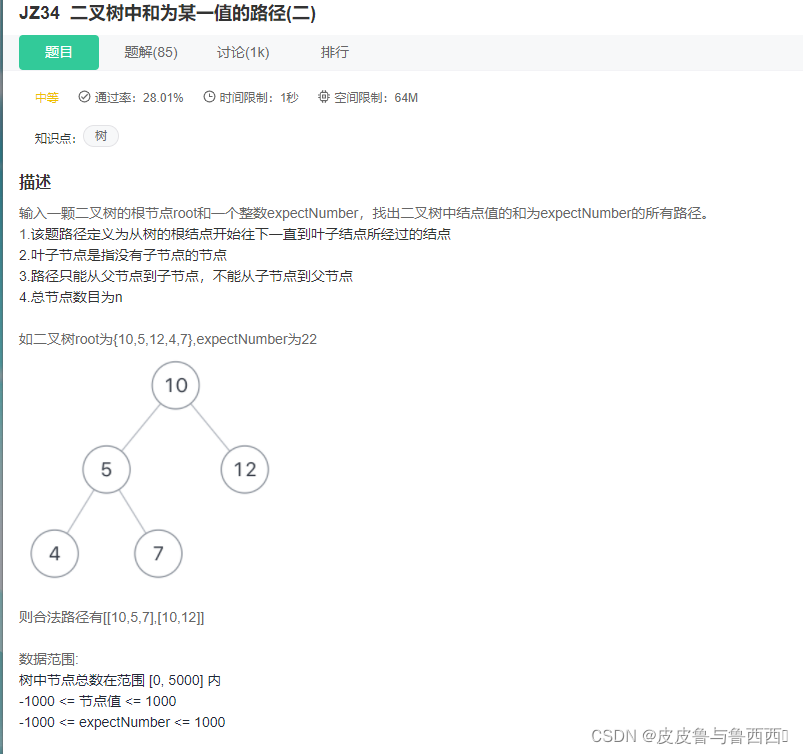
class Solution:
def hasPathSum(self , root: TreeNode, sum: int) -> bool:
if not root: return False
sum -= root.val
if not root.left and not root.right and sum==0: return True
return self.hasPathSum(root.left, sum) or self.hasPathSum(root.right, sum)
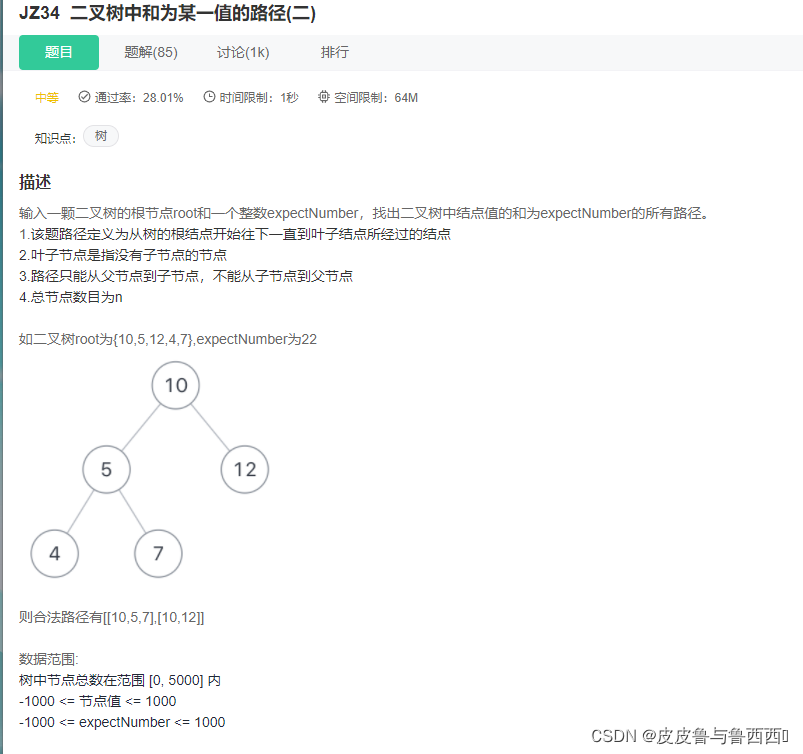 注意:在函数参数传递时,对于列表,传递的是引用的对象,所以会对原列表修改,最后奇奇怪怪。在递归时,特别要注意,不要直接传原始列表,而是copy一下,传一个新列表。用list[:]或list.copy()生成一个新的对象。
class Solution:
def FindPath(self , root: TreeNode, target: int) -> List[List[int]]:
res = []
def dfs(root, sum, path):
if not root: return
sum -= root.val
path.append(root.val)
if not root.left and not root.right and sum == 0:
res.append(path)
dfs(root.left, sum, path[:])
dfs(root.right, sum, path[:])
dfs(root, target, [])
return res
———————————————————————————————————————————— 在python中递归定义一个列表,列表中的所有内容都被最后一个项目替换 python递归时遇到的关于list的问题
|