基本数据类型
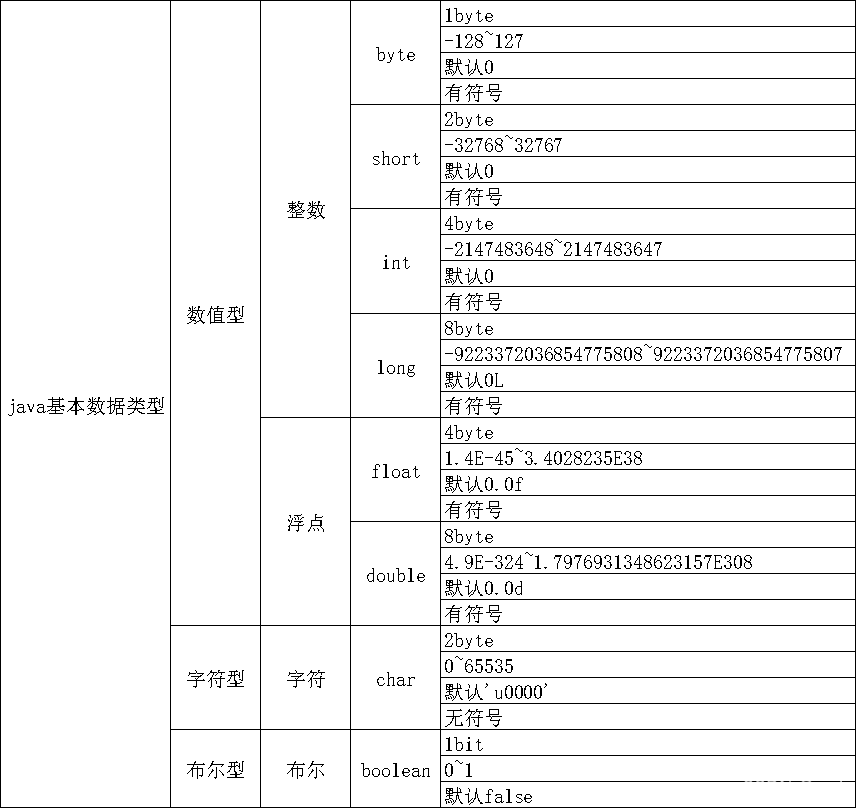
注意
- 只要不超过byte、short、char的表示范围字面量int会自动进行转换
- 因为整型和浮点型存储结构不同,整型存储为浮点型时会产生精度问题
- 浮点型转化为整型,整型会截取浮点型的整数部分
- long占8个byte能够直接转为float占4byte,long转float不需要强制转换,因为float的表示范围更大
- 类型提升,避免溢出。类型提升,以式子靠左的高类型作为提升类型
字面量
- 书写的整数字面量默认int类型
- 书写的小数字面量默认double类型
类型提升-避免溢出
long longValue=70*2100000000;
long longValue=70L*2100000000;
long longValue=2*2100000000*70L;
字面量int->long
long longValue=10L;
字面量double->float
float floatValue=10.1f;
long->低字节float
long longValue=10L;
float floatValue=longValue;
long->double
long longValue=10L;
double doubleValue=longValue;
int->byte
int intValue=10;
byte byteValue=(byte)intValue;
byte->int
byte byteValue=10;
int intValue=byteValue;
int->short
int intValue=10;
short shortValue=(short)intValue;
short->int
short shortValue=10;
int intValue=shortValue;
int->long
int intValue=10;
long longValue=intValue;
long->int
long longValue=10L;
int intValue=(int)longValue;
float->double
float floatValue=10.1f;
double doubleValue=floatValue;
double->float
double doubleValue=10.1;
float floatValue=(float)doubleValue;
int->float
int intValue=10;
float floatValue=(float)intValue;
float->int
float floatValue=10.1f;
int intValue=(int)floatValue;
int->double
int intValue=10;
double doubleValue=(double)intValue;
double->int
double doubleValue=10.1;
int intValue=(int)doubleValue;
包装类
包装类型 | 基本类型 |
---|
Byte | byte | Short | short | Integer | int | Long | long | Float | float | Double | double |
注意
Byte value=-128~127 时,java并不会产生包装类Short value=-128~127 时,java并不会产生包装类Integer value=-128~127 时,java并不会产生包装类Long value=-128~127 时,java并不会产生包装类- 包装类之间可以通过
xxxValue() 方法相互转换
优化-Byte、Short、Integer、Long
Byte aByte = 127;
Byte bByte = 127;
boolean flagByte = aByte == bByte ? true : false;
Byte aByte2 = new Byte("127");
Byte bByte2 = new Byte("127");
boolean flagByte2 = aByte2 == bByte2 ? true : false;
Short aShort = 127;
Short bShort = 127;
boolean flagShort = aShort == bShort ? true : false;
Short aShort2 = new Short("127");
Short bShort2 = new Short("127");
boolean flagShort2 = aShort2 == bShort2 ? true : false;
Integer aInteger = 127;
Integer bInteger = 127;
boolean flagInteger = aInteger == bInteger ? true : false;
Integer aInteger2 = new Integer(127);
Integer bInteger2 = new Integer(127);
boolean flagInteger2 = aInteger2 == bInteger2 ? true : false;
Long aLong = 127L;
Long bLong = 127L;
boolean flagLong = aLong == bLong ? true : false;
Long aLong2 = new Long(127L);
Long bLong2 = new Long(127L);
boolean flagLong2 = aLong2 == bLong2 ? true : false;
Byte->byte
Byte value = 10;
byte value1= value.byteValue();
Short->short
Short value = 10;
short value1= value.shortValue();
Integer->int
Integer value = 10;
int value1= value.intValue();
Long->long
Long value = 10L;
long value1= value.longValue();
Byte->Integer
Byte value = 10;
Integer value1 = value.intValue();
Integer->Byte
Integer value = 10;
Byte value1 = value.byteValue();
Short->Integer
Short value=10;
Integer value1=value.intValue();
Integer->Short
Integer value=10;
Short value1=value.shortValue();
Integer->Long
Integer value=10;
Long value1=value.longValue();
Long->Integer
Long value=10L;
Integer value1=value.intValue();
Integer->Float
Integer value=10;
Float value1=value.floatValue();
Float->Integer
Float value=10.1f;
Integer value1=value.intValue();
Integer->Double
Integer value=10;
Double value1=value.doubleValue();
Double->Integer
Double value=10.1;
Long value1=value.longValue();
String
String->byte
String str="10";
byte value=new Byte(str);
String->short
String str="10";
short value=new Short(str);
String->integer
String str="10";
int value=new Integer(str);
String->long
String str="10";
long value=new Long(str);
String->float
String str="10.1";
float value=new Float(str);
String->double
String str="10.1";
double value=new Double(str);
String->Byte
String str="10";
Byte value=new Byte(str);
Byte value2=Byte.parseByte(str);
String->Short
String str="10";
Short value=new Short(str);
Short value2=Short.parseShort(str);
String->Integer
String str="10";
Integer value=new Integer(str);
Integer value2=Integer.parseInt(str);
String->Long
String str="10";
Long value=new Long(str);
Long value2=Long.parseLong(str);
String->Float
String str="10.1";
Float value=new Float(str);
Float value2=Float.parseFloat(str);
String->Double
String str="10.1";
Double value=new Double(str);
Double value2=Double.parseDouble(str);
byte->String
byte value=10;
String str=new Byte(value).toString();
String str2=String.valueOf(value);
short->String
short value=10;
String str=new Short(value).toString();
String str2=String.valueOf(value);
int->String
int value=10;
String str=new Integer(value).toString();
String str2=String.valueOf(value);
long->String
long value=10L;
String str=new Long(value).toString();
String str2=String.valueOf(value);
float->String
float value=10.1f;
String str=new Float(value).toString();
String str2=String.valueOf(value);
double->String
double value=10.1;
String str=new Double(value).toString();
String str2=String.valueOf(value);
Byte->String
Byte value=10;
String str=value.toString();
Short->String
Short value=10;
String str=value.toString();
Integer->String
Integer value=10;
String str=value.toString();
Long->String
Long value=10L;
String str=value.toString();
Float->String
Float value=10.1f;
String str=value.toString();
Double->String
Double value=10.1;
String str=value.toString();
byte数组
todo(xcrj)
作者声明
|