思想
- 存储:国家之间只有相邻和不相邻两种关系。把国家看作顶点,用边表示两个国家相邻。考虑到每个国家都要考虑相邻关系,用邻接矩阵存储。
- 四种色号编号为1,2,3,4;国家序号用 0…n 表示;
- 用色号循环方式,给国家涂色。
- 在涂色前,先判断与当前国家相邻的国家的涂色情况:若与之相邻的国家的色号与当前国家色号相同,则用下一个色号涂色;
- 核心函数:graphColor(int k);isSafe(int k,int color); 掌握这两个函数就可以了!
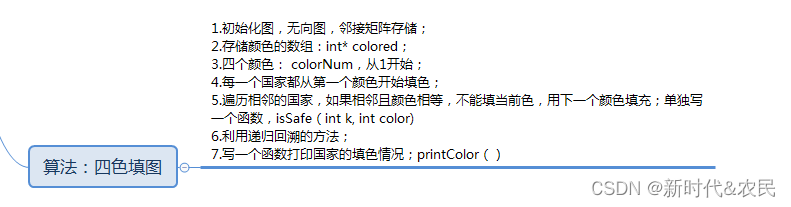 以这几个省份为例: 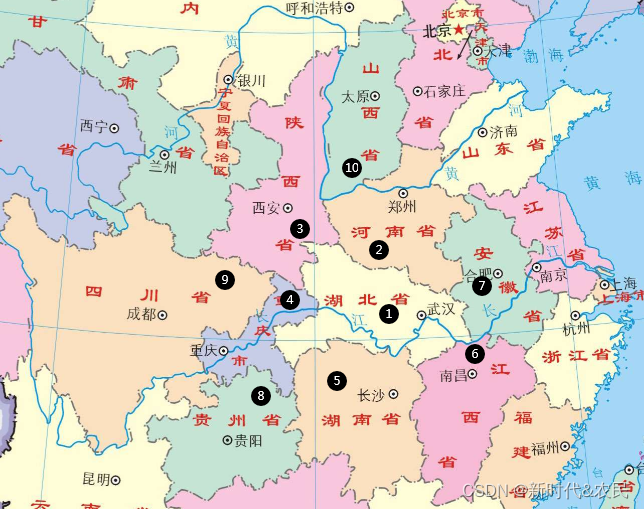 抽象成顶点与边的关系: 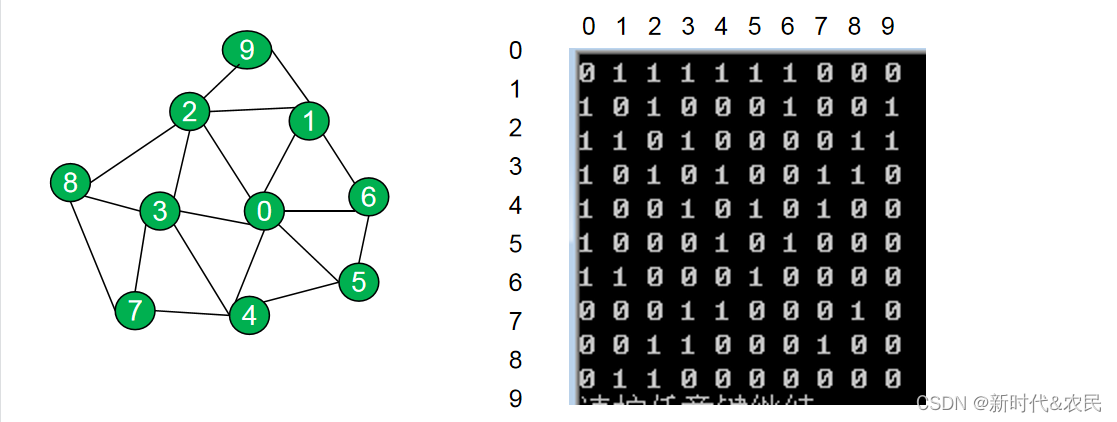
代码
#pragma once
#include <iostream>
#include <cassert>
#include "DenseGraph.h"
using namespace std;
class colorMap {
private:
int colorNum = 4;
int* colored;
DenseGraph& G;
vector<vector<bool>> vec;
public:
colorMap(DenseGraph& graph):G(graph) {
colored = new int[G.V()];
this->vec = G.getMatrix();
for (int i = 0; i <G.V(); ++i) {
colored[i] = 0;
}
graphColor(0);
}
~colorMap() {
delete[] colored;
}
void graphColor(int k) {
for (int c = 1; c <= colorNum; ++c) {
if (isSafe(k, c)) {
colored[k] = c;
if (k + 1 < G.V())
graphColor(k + 1);
else
printColored(); return;
}
}
}
bool isSafe(int k, int c) {
for (int i = 0; i < G.V(); ++i) {
if (vec[k][i] == true && c == colored[i])
return false;
}
return true;
}
void printColored() {
for (int i = 0; i < G.V();++i) {
cout << i << ": " << colored[i] << endl;
}
}
};
测试
- 顶点与边的信息存储在 map.txt 中;
- 初始化无向无权图;
- 引入着色头文件,涂色;
#include <iostream>
#include <fstream>
#include <string>
#include "DenseGraph.h"
#include "colorMap.h"
using namespace std;
int main() {
string filename1 = "map.txt";
DenseGraph DG(10, false);
readGraph<DenseGraph> readDenseGraph(DG, filename1);
DG.show();
colorMap CM(DG);
system("pause");
return 0;
}
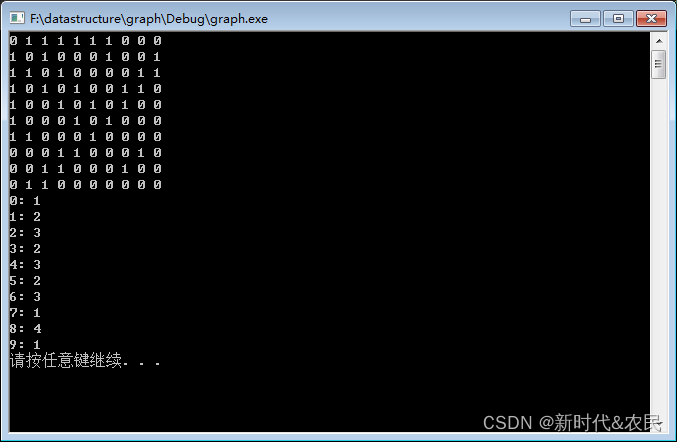 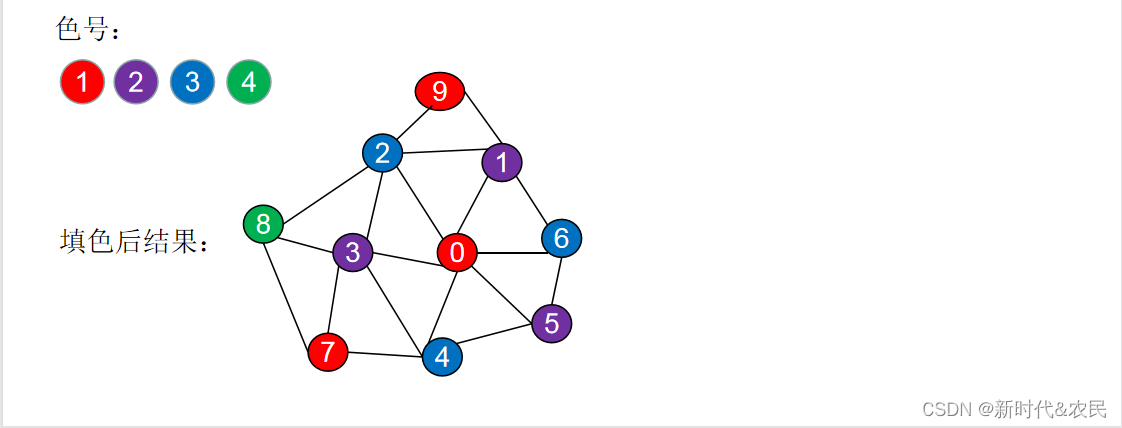
|