题目 给你一个链表,删除链表的倒数第 n 个结点,并且返回链表的头结点。
示例1
输入:head = [1,2,3,4,5], n = 2
输出:[1,2,3,5]
示例2
输入:head = [1], n = 1
输出:[]
示例2
输入:head = [1,2], n = 1
输出:[1]
题解
-
定义两个指针front,back指向头结点 -
第1个指针front从链表的头结点开始遍历k步,第2个指针back保持不动; -
从第k+1步开始指针back也从链表的头结点开始和指针front以相同的速度遍历。 -
当指针p1指向链表的尾节点时指针p2正好指向倒数第k+1个节点(两个指针的距离始终保持为k) -
把倒数第k+1个节点的next指针指向倒数第k-1个节点
代码实现
解类如下,类里包含两个方法,一个是遍历链表的,一个是删除链表的倒数第 N 个结点的
public class Solution {
public static ListNode removeNthFromEnd(ListNode head, int n) {
ListNode<Integer> dummy = new ListNode<>(0);
dummy.next = head;
ListNode front = dummy;
ListNode back = dummy;
for (int i = 0; i < n; i++) {
front = front.next;
}
while (front.next != null) {
front = front.next;
back = back.next;
}
back.next = back.next.next;
return dummy.next;
}
public static void printList(ListNode head) {
System.out.print("[");
while (head != null) {
if (head.next == null) {
System.out.print(head.val);
} else {
System.out.print(head.val + ",");
}
head = head.next;
}
System.out.print("]");
}
}
结点类
public class ListNode<T> {
T val;
ListNode next;
public ListNode() {
}
public ListNode(T val) {
this.val = val;
}
public ListNode(T val, ListNode next) {
this.next = next;
}
}
测试类
public class Test {
@org.junit.Test
public void test1() {
ListNode<Integer> head = new ListNode<>(1);
ListNode<Integer> listNode = new ListNode<>(2);
ListNode<Integer> listNode1 = new ListNode<>(3);
ListNode<Integer> listNode2 = new ListNode<>(4);
ListNode<Integer> listNode3 = new ListNode<>(5);
head.next = listNode;
listNode.next = listNode1;
listNode1.next = listNode2;
listNode2.next = listNode3;
System.out.print("输入:");
Solution.printList(head);
System.out.println(" 2");
ListNode head2 = Solution.removeNthFromEnd(head, 2);
System.out.print("输出:");
Solution.printList(head2);
}
@org.junit.Test
public void test2() {
ListNode<Integer> head = new ListNode<>(1);
System.out.print("输入:");
Solution.printList(head);
System.out.println(" 1");
ListNode head2 = Solution.removeNthFromEnd(head, 1);
System.out.print("输出:");
Solution.printList(head2);
}
@org.junit.Test
public void test3() {
ListNode<Integer> head = new ListNode<>(1);
ListNode<Integer> listNode = new ListNode<>(2);
head.next = listNode;
System.out.print("输入:");
Solution.printList(head);
System.out.println(" 2");
ListNode head2 = Solution.removeNthFromEnd(head, 1);
System.out.print("输出:");
Solution.printList(head2);
}
}
测试结果 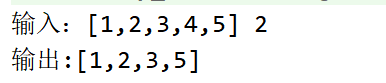 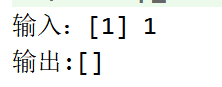 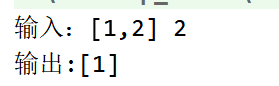
|