链表实现栈
一、项目结构
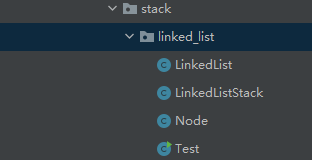
二、链表
1、节点Node
public class Node {
private int n;
private Node next;
public Node(int n) {
this.n = n;
}
public int getN() {
return n;
}
public void setN(int n) {
this.n = n;
}
public Node getNext() {
return next;
}
public void setNext(Node next) {
this.next = next;
}
}
2、链表
public class LinkedList {
private Node bottom = null;
public void add(int n){
if (bottom == null){
bottom = new Node(n);
return;
}
Node temp = bottom;
while (true){
if (temp.getNext() == null){
break;
}
temp = temp.getNext();
}
temp.setNext(new Node(n));
}
public int delete(){
if (bottom.getNext() == null){
int value = bottom.getN();
bottom = null;
return value;
}
Node temp = bottom;
while (true){
if (temp.getNext().getNext() == null){
break;
}
temp = temp.getNext();
}
int value = temp.getNext().getN();
temp.setNext(null);
return value;
}
public Node getBottom() {
return bottom;
}
public int size(){
Node temp = bottom;
int size = 0;
while (true){
if (temp == null){
break;
}
size++;
temp = temp.getNext();
}
return size;
}
public void print(){
Node temp = bottom;
int size = 0;
while (true){
if (temp == null){
break;
}
System.out.println(temp.getN());
temp = temp.getNext();
}
}
}
三、栈
public class LinkedListStack {
private LinkedList linkedList;
public LinkedListStack() {
this.linkedList = new LinkedList();
}
public boolean isEmpty(){
return linkedList.getBottom() == null;
}
public void push(int n){
linkedList.add(n);
}
public int pop(){
if (isEmpty()){
System.out.println("栈为空");
return -1;
}
return linkedList.delete();
}
public int size(){
return linkedList.size();
}
public void print(){
linkedList.print();
}
}
四、测试
1、测试类
public class Test {
public static void main(String[] args) {
LinkedListStack stack = new LinkedListStack();
for (int i = 0; i < 10; i++) {
stack.push(i);
}
stack.print();
System.out.println("----------");
for (int i = 0; i < 10; i++) {
System.out.println(stack.pop());
}
System.out.println("-----------");
stack.pop();
}
}
2、运行结果
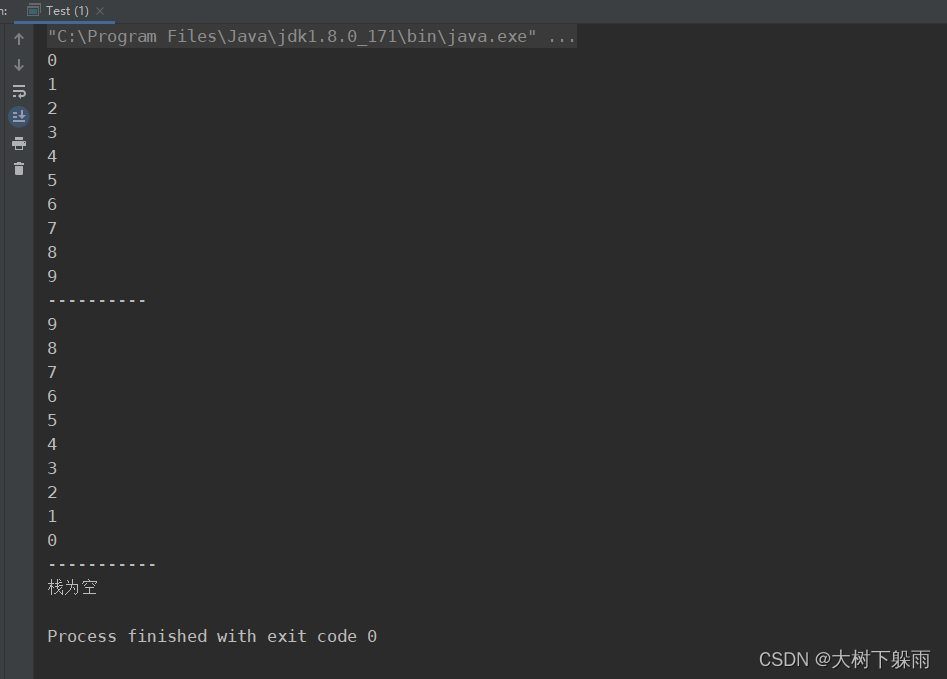
|