题目的大致意思:
????????未知一个原矩阵A,给两个矩阵rows和columns,其中rows 表示A按照行重排,但是列的顺序,即列顺序不变,colums 表示A按照列重排,即行顺序不变,请恢复出原矩阵A。
????????示例:给出rows和colums,恢复出A矩阵
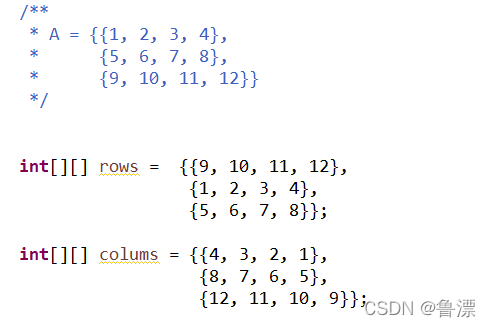
?面试时,没写出来,现在给出暴力破解的思路:
? ? ? ? 使用哈希表记录每个值的位置(rowIndex, columnIndex)
????????Java的具体实现:
public class LeetCodeTest {
public static void main(String[] args) {
/**
* A = {{1, 2, 3, 4},
* {5, 6, 7, 8},
* {9, 10, 11, 12}}
*/
int[][] rows = {{9, 10, 11, 12},
{1, 2, 3, 4},
{5, 6, 7, 8}};
int[][] colums = {{4, 3, 2, 1},
{8, 7, 6, 5},
{12, 11, 10, 9}};
Solution solution = new Solution();
solution.reverseWords(rows, colums);
}
}
class Solution {
public int[][] reverseWords(int[][] rows, int[][] colums) {
if(rows[0].length == 0 || colums[0].length == 0) {
return null;
}
HashMap<Integer, int[]> valAndPosIndex = new HashMap<Integer, int[]>();
for (int i = 0; i < rows.length; i++) {
for (int j = 0; j < rows[0].length; j++) {
int[] pos = new int[2];
pos[1] = j; //(rowIndex, columnIndex)
valAndPosIndex.put(rows[i][j], pos);
}
}
for (int i = 0; i < colums.length; i++) {
for (int j = 0; j < colums[0].length; j++) {
int[] pos = valAndPosIndex.get(colums[i][j]);
pos[0] = i;
valAndPosIndex.put(colums[i][j], pos);
}
}
int[][] result = new int[rows.length][rows[0].length];
for (int i = 0; i < rows.length; i++) {
for (int j = 0; j < rows[0].length; j++) {
int[] pos = valAndPosIndex.get(rows[i][j]);
result[pos[0]][pos[1]] = rows[i][j];
}
}
show(result);
return result;
}
public void show(int[][] nums) {
for (int[] is : nums) {
for (int num : is) {
System.out.print(num + " ");
}
System.out.println();
}
}
}
时间复杂度:O(m*n)
空间复杂度:O(m*n)
|