1. 介绍
1)front含义:arr[front]就是队列的一个元素,初始值为0。 2)rear含义:rear指向队列的最后一个元素的最后一个位置,空出一个空间作为约定,初始值为0。 3)表示队列为空:rear == front
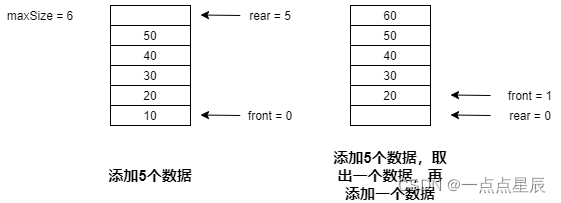
4)表示队列为满:(rear + 1) % maxSize == front
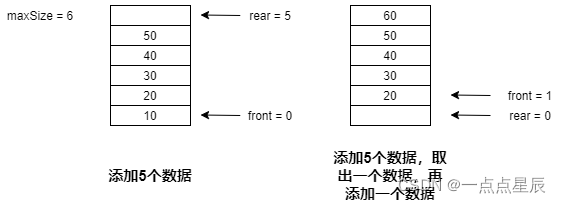 5)表示队列有效数据的个数:(rear + maxSize - front) % maxSize
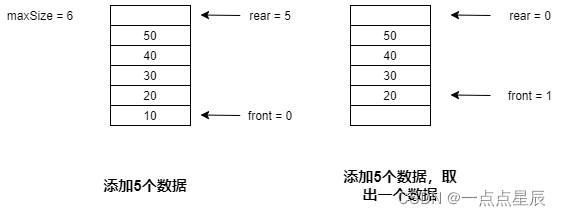 6)代码实现
import java.util.Scanner;
public class CircleArrayQueue {
public static void main(String[] args) {
Scanner scan = new Scanner(System.in);
Queue1 q1 = new Queue1(6);
System.out.println("1.显示队列");
System.out.println("2.添加数据");
System.out.println("3.取出数据");
System.out.println("4.显示队首");
System.out.println("5.退出");
boolean bool = true;
while(bool) {
System.out.println("请输入操作:");
int cin = scan.nextInt();
if(cin != 1 && cin != 2 && cin != 3 && cin != 4 && cin != 5){
System.out.println("输入有误,请重新输入!");
}else{
switch (cin) {
case 1:
try{
q1.showQueue();
}catch(Exception e){
System.out.println(e.getMessage());
}
break;
case 2:
System.out.println("请输入一个数字:");
int value = scan.nextInt();
q1.addQueue(value);
break;
case 3:
System.out.println("取出的数据为:" + q1.removeQueue());
break;
case 4:
try{
System.out.println("队首元素是:" + q1.showHeadQueue());
}catch(Exception e){
System.out.println(e.getMessage());
}
break;
case 5:
scan.close();
bool = false;
}
}
}
System.out.println("程序退出~~");
}
}
class Queue1{
private int maxSize;
private int front;
private int rear;
private int arr[];
public Queue1(int size) {
maxSize = size;
front = 0;
rear = 0;
arr = new int[maxSize];
}
public boolean empty(){
return front == rear;
}
public boolean full(){
return (rear + 1) % maxSize == front;
}
public int dataNumber(){
return (rear + maxSize - front) % maxSize;
}
public void showQueue(){
if(empty()){
throw new RuntimeException("队列为空~~");
}
for(int i = front; i < front + dataNumber();i++){
int j = i % maxSize;
System.out.println("arr[" + j + "] = " + arr[j]);
}
}
public void addQueue(int data){
if(full()){
System.out.println("队列已满~~");
return;
}
arr[rear] = data;
rear = (rear + 1) % maxSize;
}
public int removeQueue(){
if(empty()){
throw new RuntimeException("队列为空~~");
}
int value = arr[front];
front = (front + 1) % maxSize;
return value;
}
public int showHeadQueue(){
if(empty()){
throw new RuntimeException("队列为空~~");
}
return arr[front];
}
}
|