力扣算法学习day03-3
19-删除链表的倒数第N个结点
题目
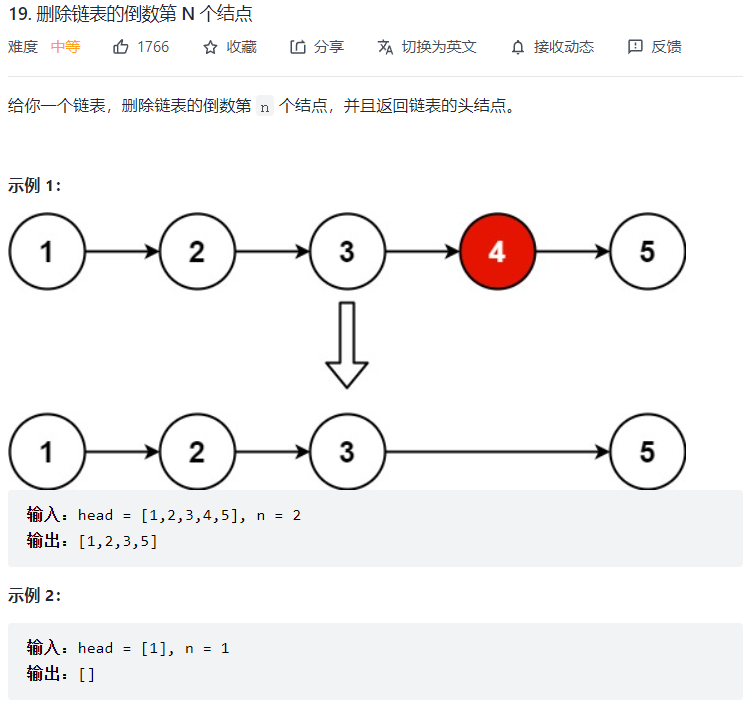
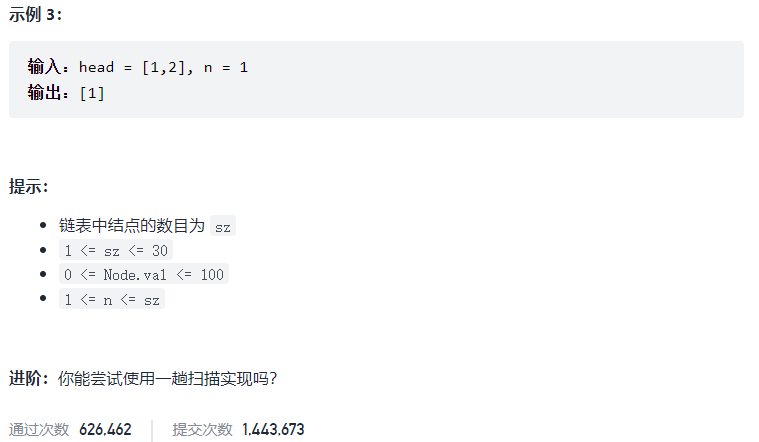
代码实现
class Solution {
public ListNode removeNthFromEnd(ListNode head, int n) {
ListNode node = new ListNode(0,head);
ListNode pre = node;
ListNode cur = node;
while(n-- >= 0){
cur = cur.next;
}
while(cur != null){
pre = pre.next;
cur = cur.next;
}
pre.next = pre.next.next;
return node.next;
}
}
面试题02.07.-链表相交
题目
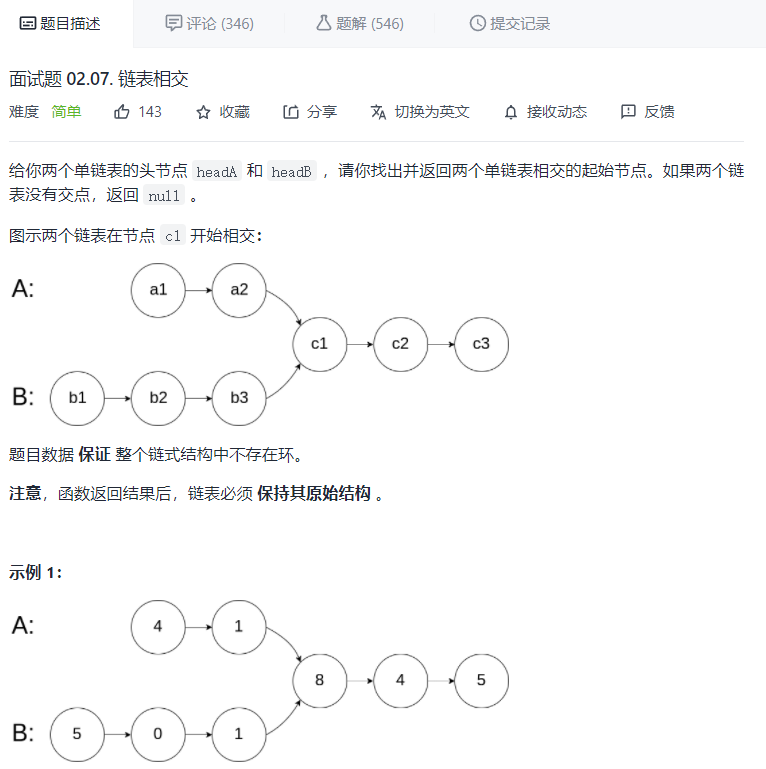
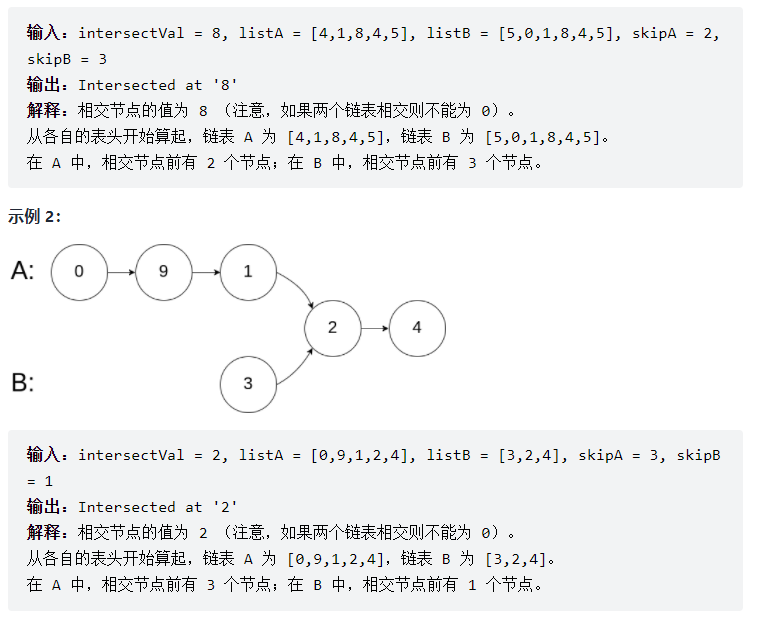
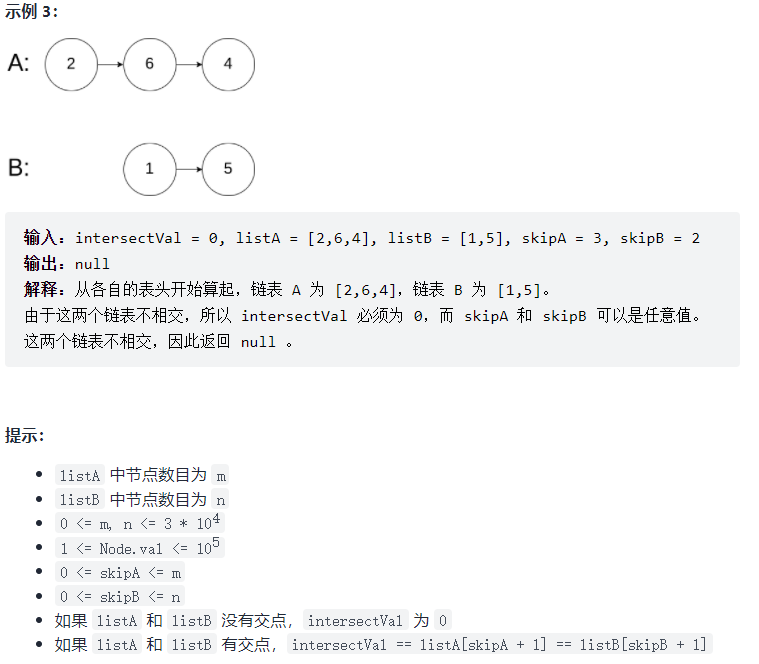
代码实现
public class Solution {
public ListNode getIntersectionNode(ListNode headA, ListNode headB) {
int lengthA = 0;
int lengthB = 0;
ListNode nodeA = headA;
ListNode nodeB = headB;
while(nodeA != null){
nodeA = nodeA.next;
lengthA++;
}
while(nodeB != null){
nodeB = nodeB.next;
lengthB++;
}
int length = 0;
if(Math.max(lengthA,lengthB) == lengthA){
nodeA = headA;
nodeB = headB;
length = lengthA - lengthB;
} else {
nodeA = headB;
nodeB = headA;
length = lengthB - lengthA;
}
while(length > 0){
nodeA = nodeA.next;
length--;
}
while(nodeA != null){
if(nodeA == nodeB){
return nodeA;
}
nodeA = nodeA.next;
nodeB = nodeB.next;
}
return null;
}
}
142-环形链表II
题目
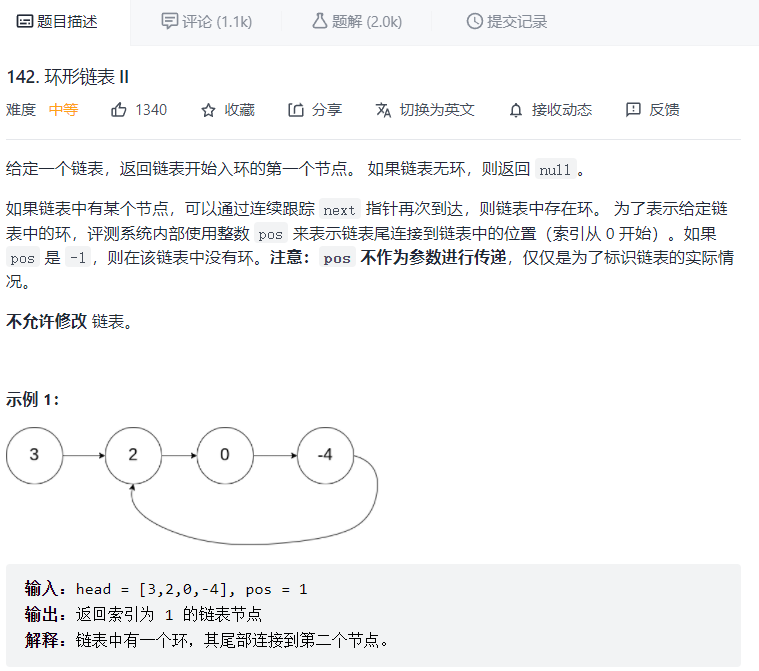
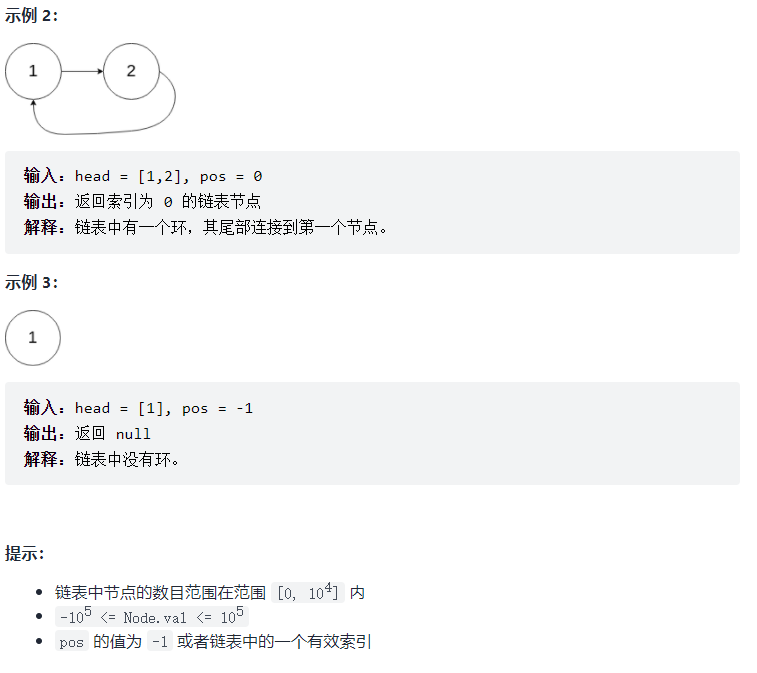
代码实现
public class Solution {
public ListNode detectCycle(ListNode head) {
if(head == null){
return null;
}
ListNode fast = head;
ListNode slow = head;
while(true){
if(fast.next == null || fast.next.next == null){
return null;
}
slow = slow.next;
fast = fast.next.next;
if(fast == slow){
break;
}
}
slow = head;
while(true){
if(fast == slow){
return fast;
}
slow = slow.next;
fast = fast.next;
}
}
}
|