题目:
给定一个长度为 n 的数组 num 和滑动窗口的大小 size ,找出所有滑动窗口里数值的最大值。
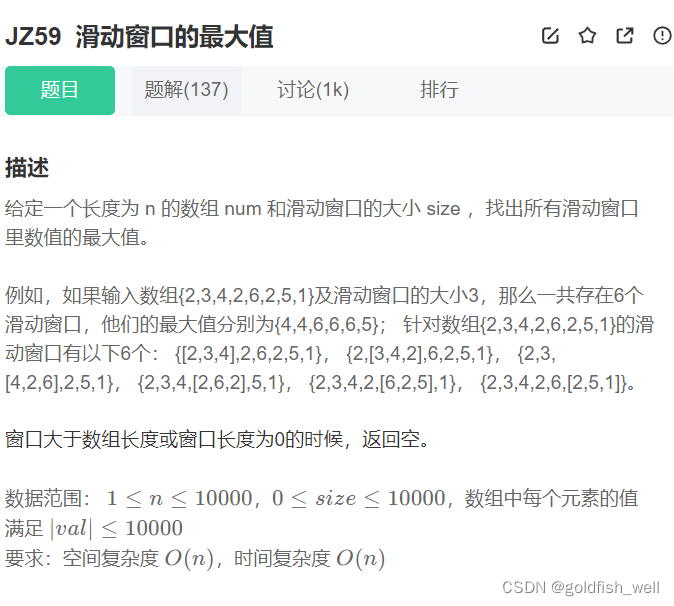
示例:
输入:[2,3,4,2,6,2,5,1],3
返回值:[4,4,6,6,6,5]
思路:
这道题之前写过,这里使用栈是行不通的,因为我们要对双端进行操作。
所以这里要借助链表来完成双向队列 再用双指针完成滑动数组
复杂度:
时间复杂度:遍历O(n)
空间复杂度:队列O(n)
代码:
public ArrayList<Integer> maxInWindows(int [] num, int size) {
//建立双端的递减队列
Deque<Integer> queue = new LinkedList<>();
ArrayList<Integer> res = new ArrayList<>();
int len = num.length;
//特殊情况
if(num==null || size==0 || size>len) return res;
for(int i=1-size,j=0;j<len;++j,++i){
//左指针已在界内,并且队列中的最大值刚好是左指针指向的值,就删除
if(i>0 && queue.peekFirst() == num[i-1]){
queue.removeFirst();
}
//将队列维持递减队列
while(!queue.isEmpty() && queue.peekLast()<num[j]){
queue.removeLast();
}
//队列添加
queue.addLast(num[j]);
//结果添加
if(i>=0){
res.add(queue.peekFirst());
}
}
return res;
|