前言
随风潜入夜,润物细无声。—《春夜喜雨》 (每天一道数据结构,Day 1)
关于队列
队列是有序列表,可以用数组或链表来实现。 队列的特点:先入先出。今天我们采用数组实现队列。 比较关键的是要弄明白rear、front、maxSize之间的关系,最好自己先推一遍,这样理解地更加深刻。 图解如下: 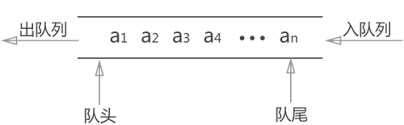
必须理解的地方
- front、rear、maxSize
使 front=0;rear=0; 实际队列比maxSize小1。 空出一个空间做约定。 出队列:front(队头)增加。 入队列:rear(队尾)增加。 a[ ] :用来存放数据。 front指向队列的第一个元素,rear指向队列的最后一个元素的后一个位置。 —————————————————————————————————— 当队列满时:(rear+1)%maxSize==front 当队列为空:rear==front 有效数据个数:(rear+maxSize-front)%maxSize 遍历数据:
for (int i = front; i< front + size(); i++) {
System.out.printf("a[%d]=%d\n", i%maxSize, a[i%maxSize]);
}
}
- CircleQueue方法
isFull :判断队列是否已满。(注意计算方法) isEmpty :判断队列是否为空。 size :返回有效的数据的个数。 addDate :增加数据。 getDate :取出数据。 showHead :显示头部数据。 listDate :显示所有数据。
Java代码实现
import java.util.Scanner;
public class CircleArrayQueue {
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
Queue q = new Queue(4);
Boolean loop = true;
while (loop) {
System.out.println("a(增加数据)");
System.out.println("g(取出数据)");
System.out.println("s(显示数据)");
System.out.println("h(显示头部数据)");
System.out.println("e(退出程序)");
char key = in.next().charAt(0);
switch (key) {
case 'a':
System.out.println("请输入数据:");
try {
q.addDate(in.nextInt());
} catch (Exception e) {
System.out.println(e.getMessage());
}
break;
case 'g':
try {
System.out.println(q.getDate());
} catch (Exception e) {
System.out.println(e.getMessage());
}
break;
case 's':
try {
q.listDate();
} catch (Exception e) {
System.out.println(e.getMessage());
}
break;
case 'h':
try {
q.showHead();
} catch (Exception e) {
System.out.println(e.getMessage());
}
break;
case 'e':
loop = false;
break;
}
}
System.out.println("程序结束_by—Sivan_Xin");
}
}
class Queue{
private int maxSize;
private int front=0;
private int rear=0;
private int []a;
public Queue(int maxSize){
this.maxSize=maxSize;
a=new int [maxSize];
}
public boolean isFull() {
return (rear+1)%maxSize==front;
}
public boolean isEmpty(){
return front==rear;
}
public void addDate(int date){
if(isFull()){
throw new RuntimeException("队列满,不能存入数据");
}
a[rear]=date;
rear=(rear+1)%maxSize;
}
public int getDate(){
if(isEmpty()){
throw new RuntimeException("队列为空,不能取数据");
}
int value=a[front];
front=(front+1)%maxSize;
return value;
}
public void showHead(){
if(isEmpty()){
throw new RuntimeException("队列为空,无头部数据");
}
System.out.println("头部数据为:"+a[front]);
}
public void listDate() {
if (isEmpty()) {
throw new RuntimeException("队列为空,没有数据");
}
for (int i = front; i< front + size(); i++) {
System.out.printf("a[%d]=%d\n", i%maxSize, a[i%maxSize]);
}
}
public int size(){
return (rear+maxSize-front)%maxSize;
}
}
最后,如果觉得写的还不错的话,欢迎关注哦!
|