目录
内容小结
String类
String对象的创建
字符串的特性判断
拼接
String类的常用方法
String和char[]的相互转换
实现代码
StringBuffer
StringBuffer常用方法
StringBuilder
JDK8之前日期时间API
Date类
Calendar日历类
常用方法的使用:
JDK8中新日期时间API
Instant类
格式化与解析日期或时间
DateTimeFormatter类
实例化的三种方式
Java比较器
Comparable接口
Comparator排序
实际在写算法题时用到的排序方法
System类
Math类
BigInteger类
BigDecimal类
?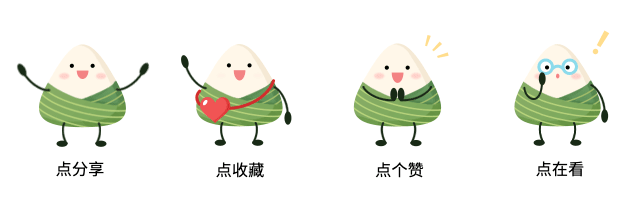
内容小结
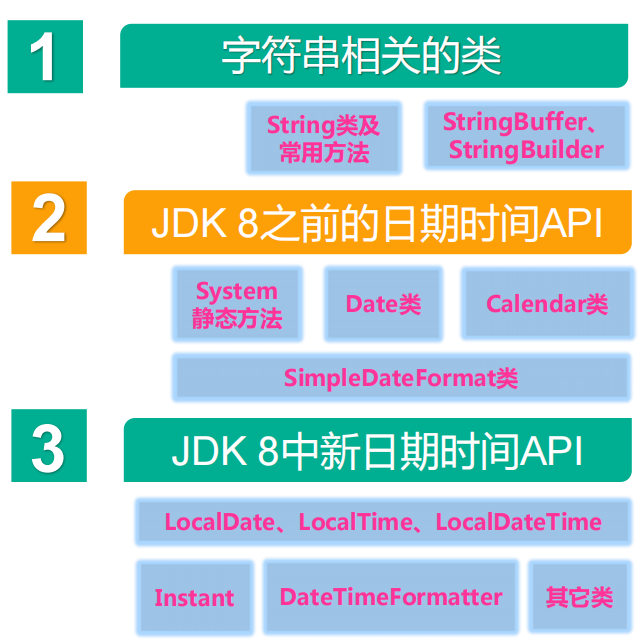
?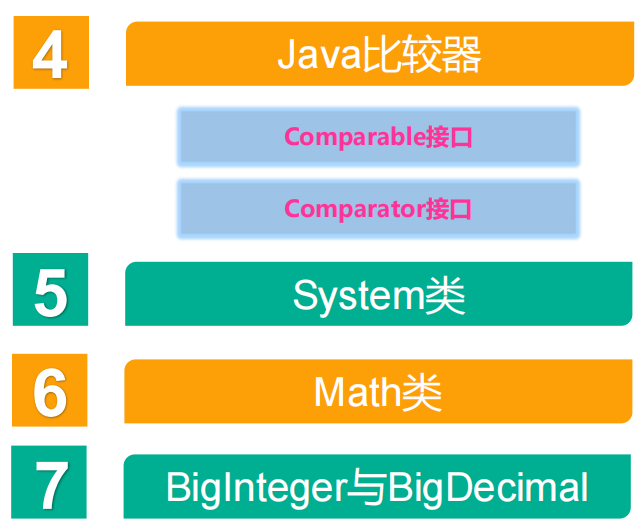
?
String类
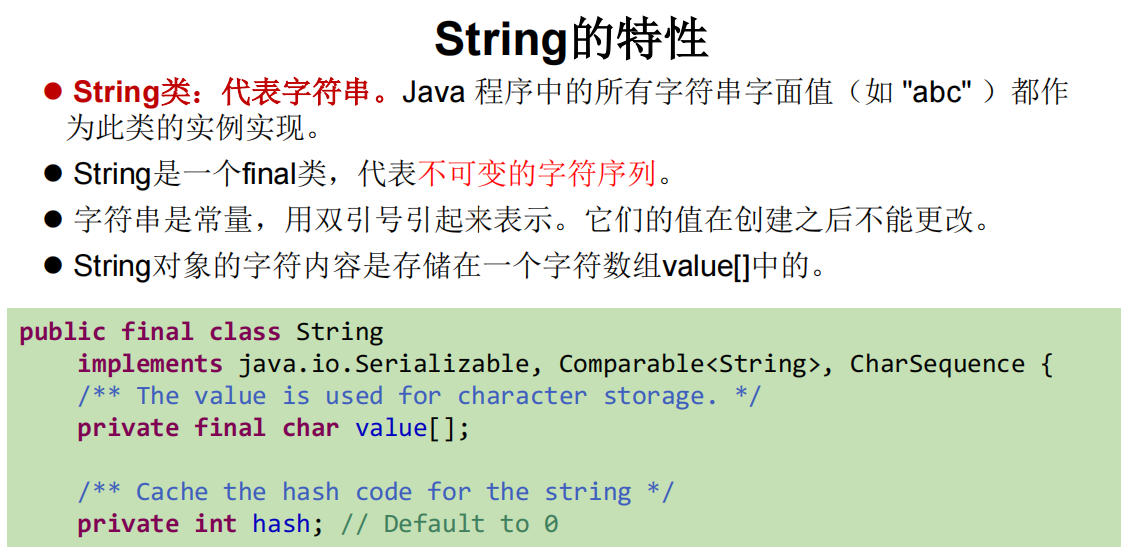 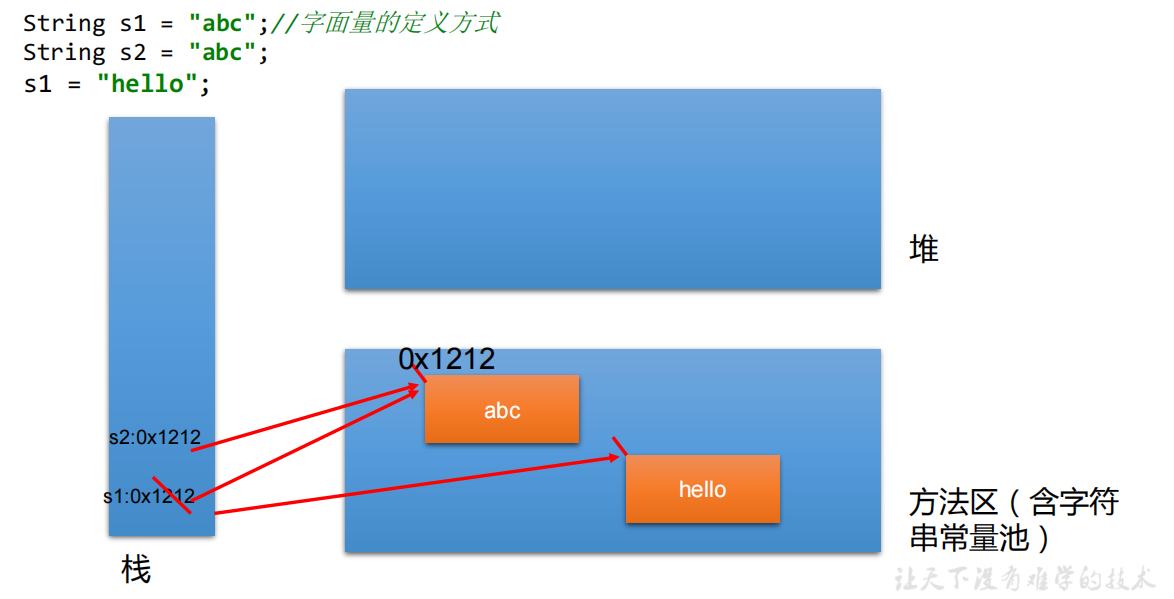
?
String对象的创建
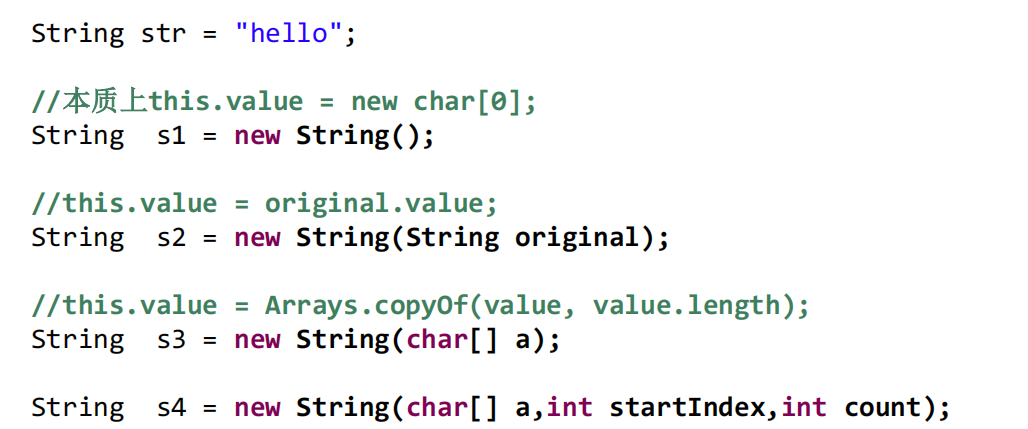
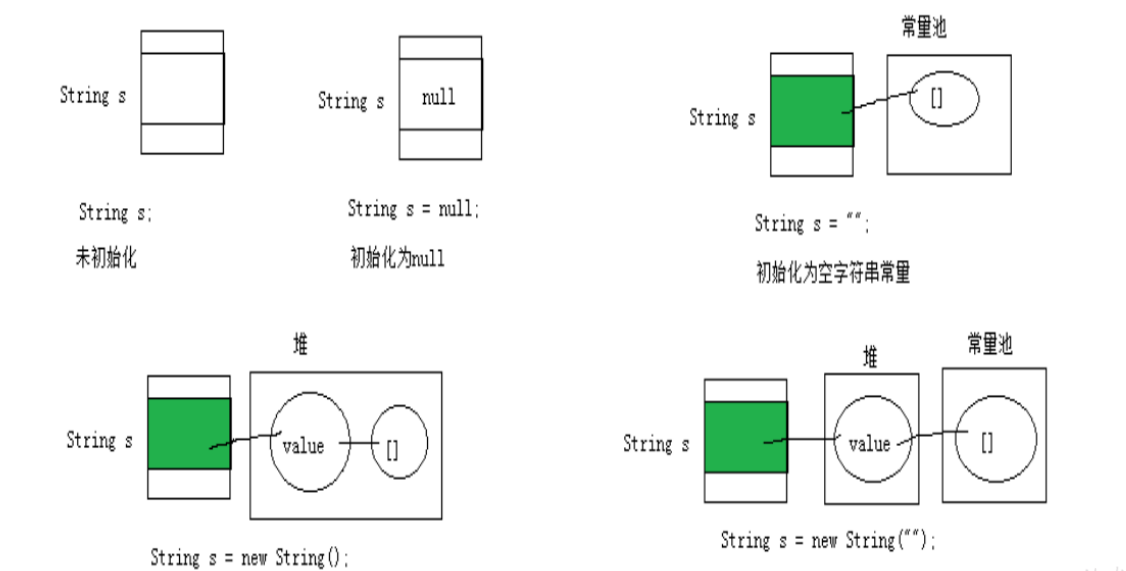
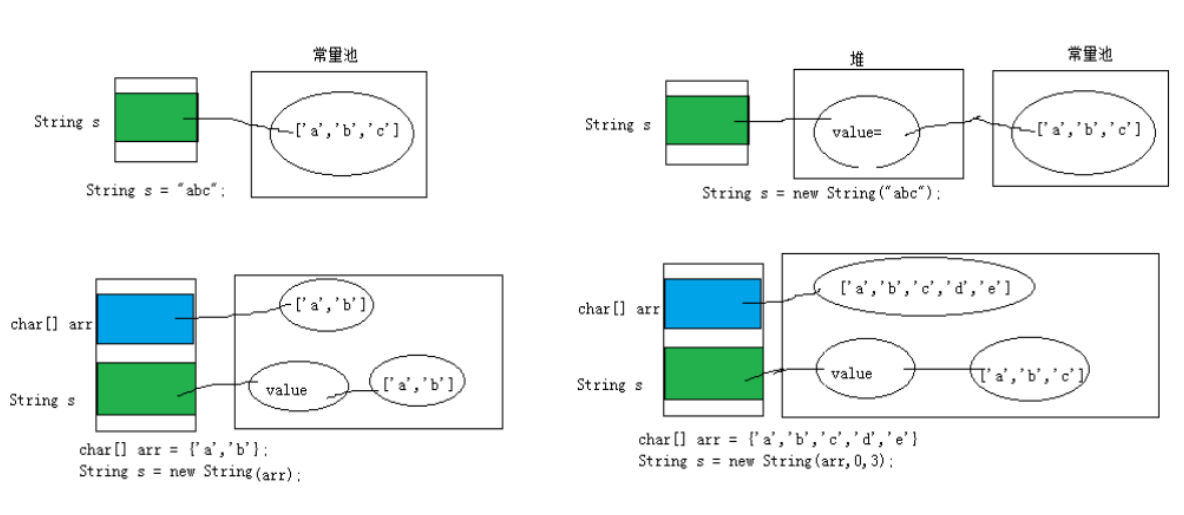
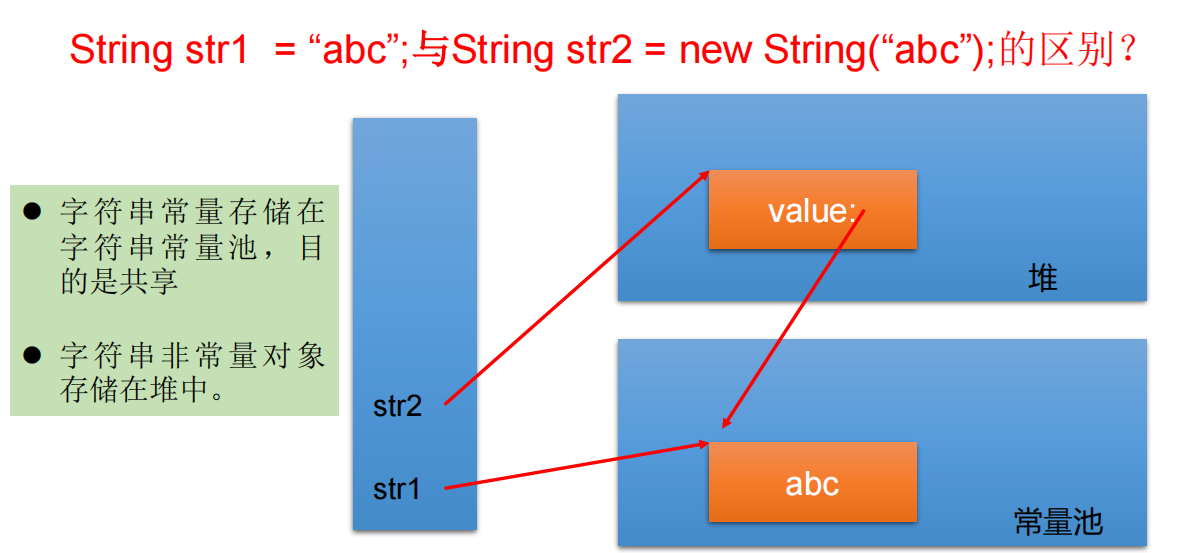
?
字符串的特性判断
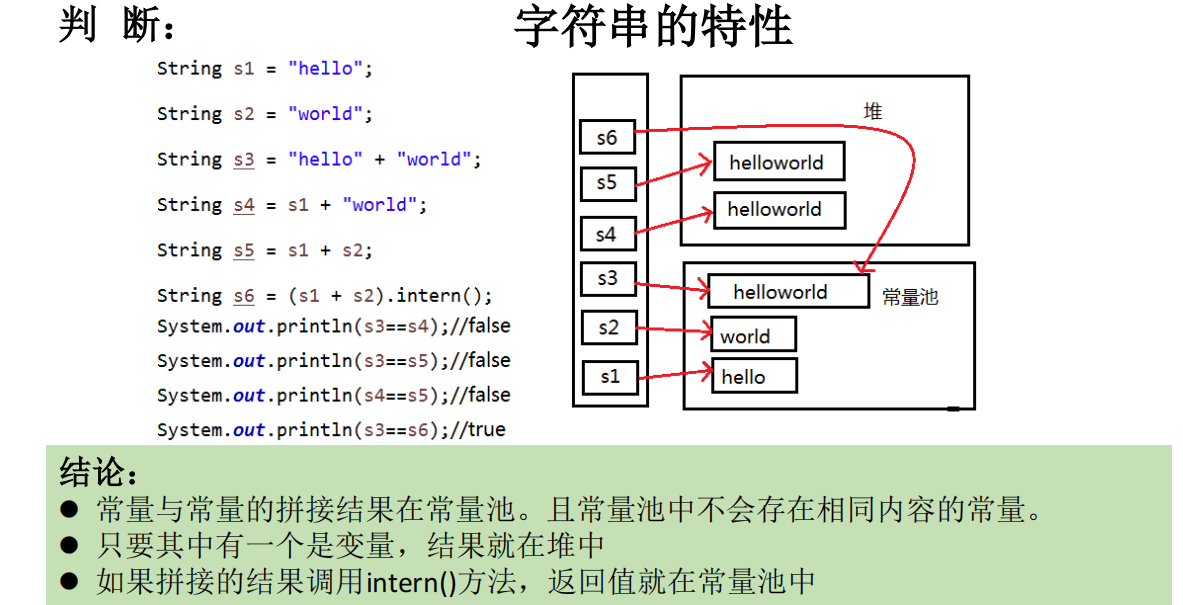
?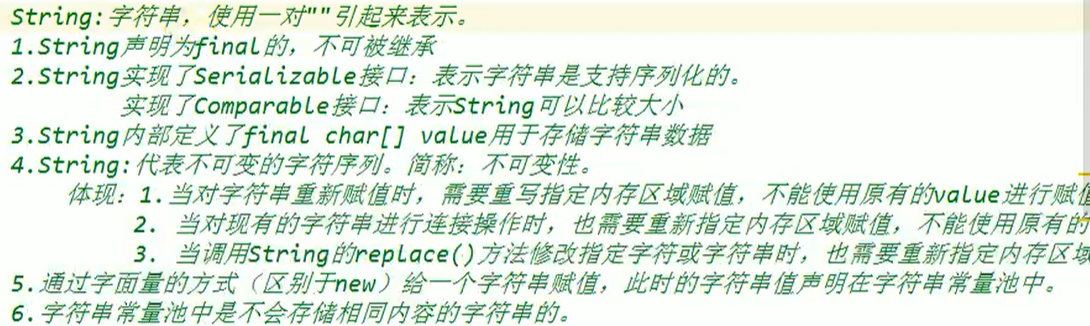
?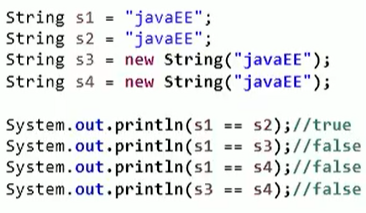
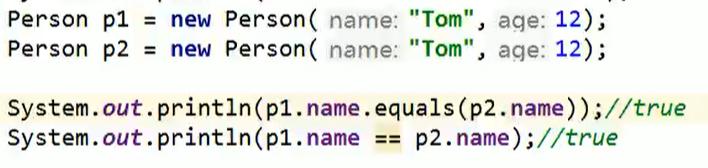
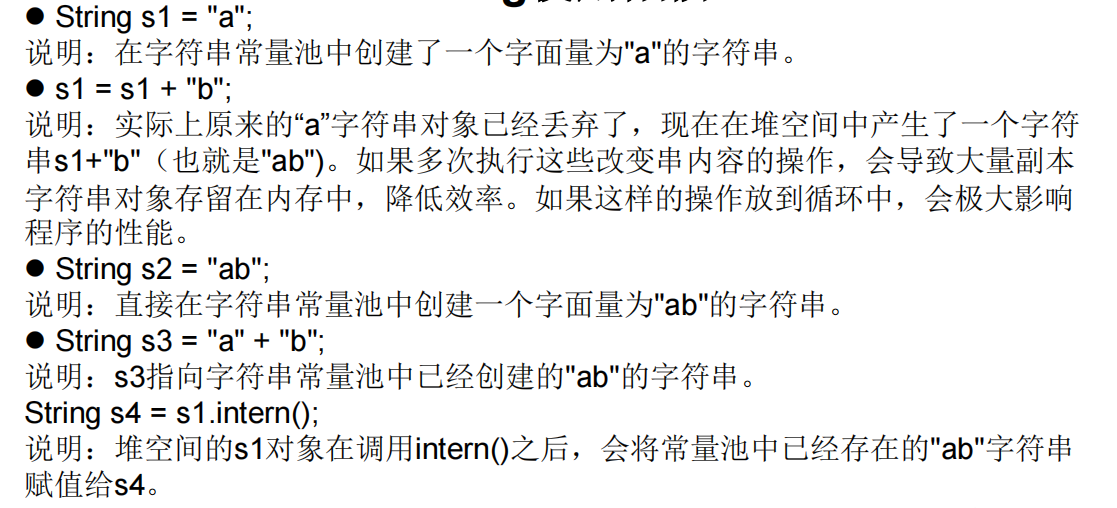
?
拼接
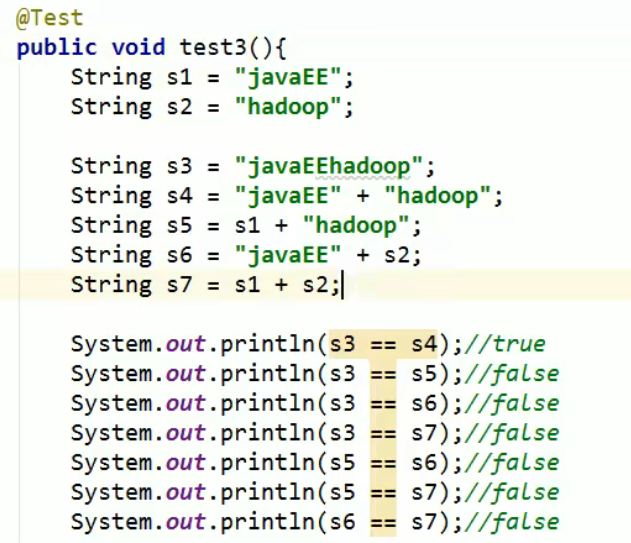
?
?
String类的常用方法
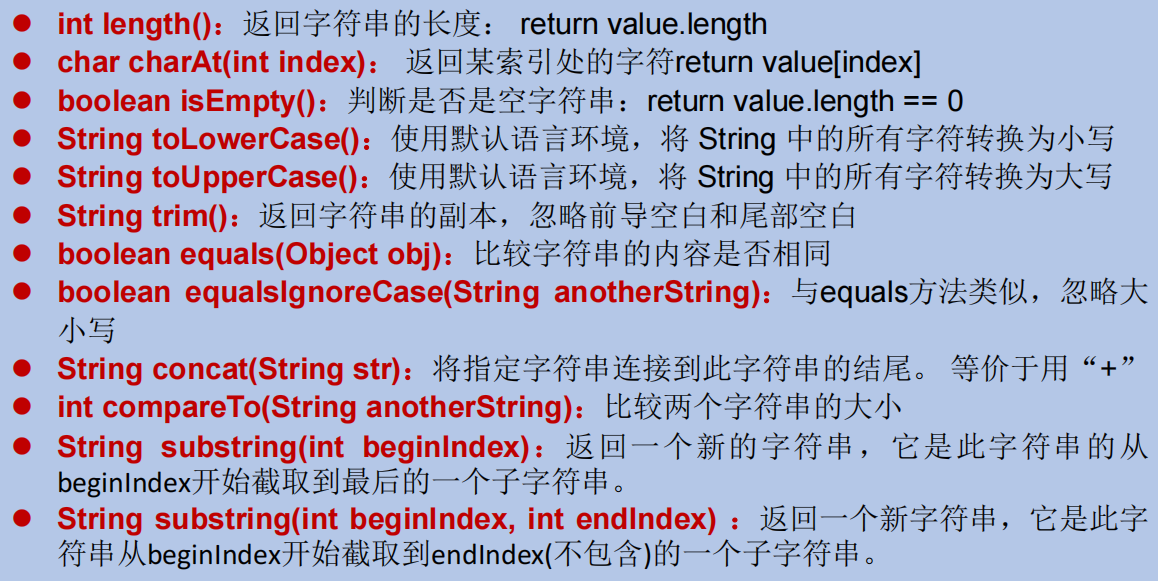
?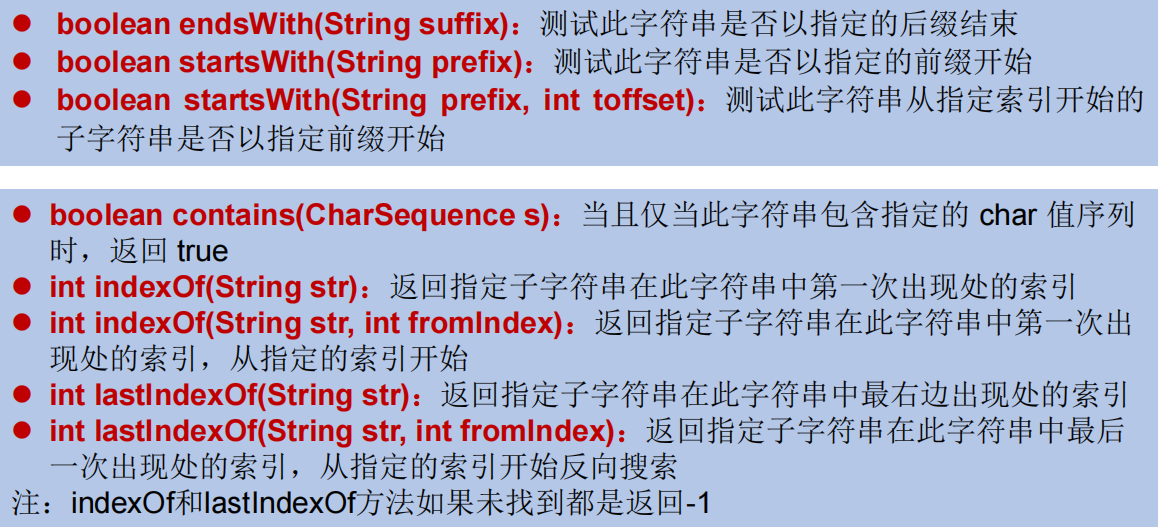
?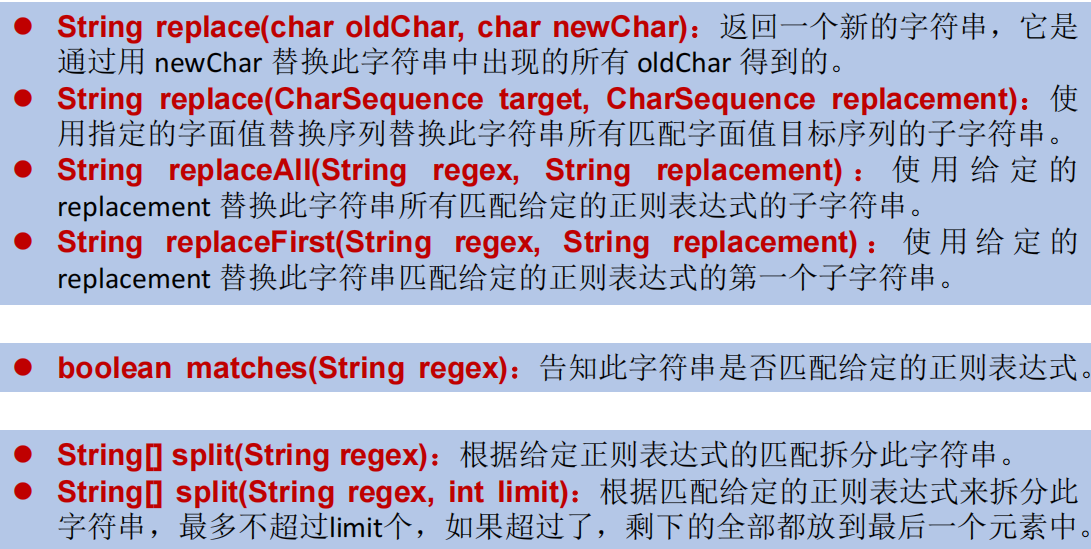
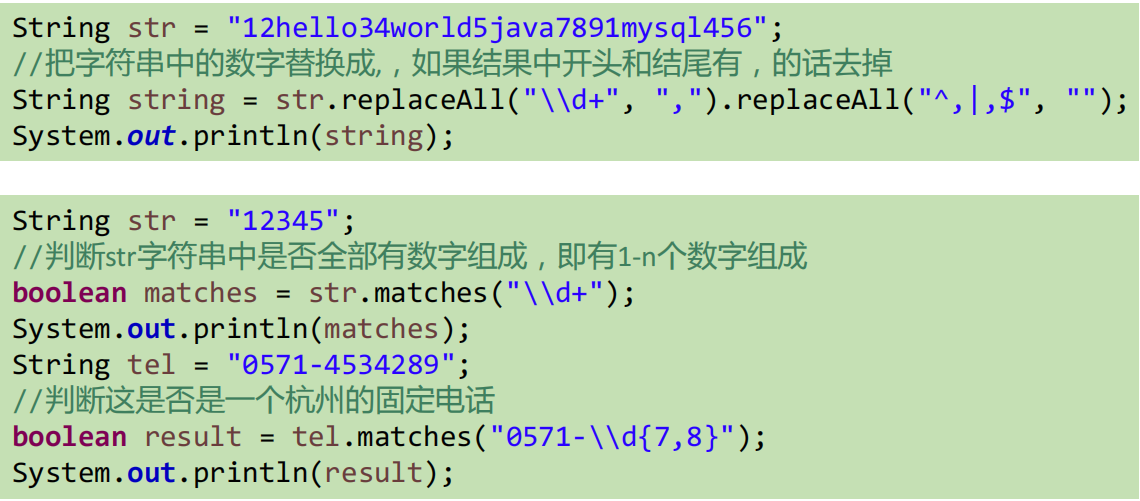
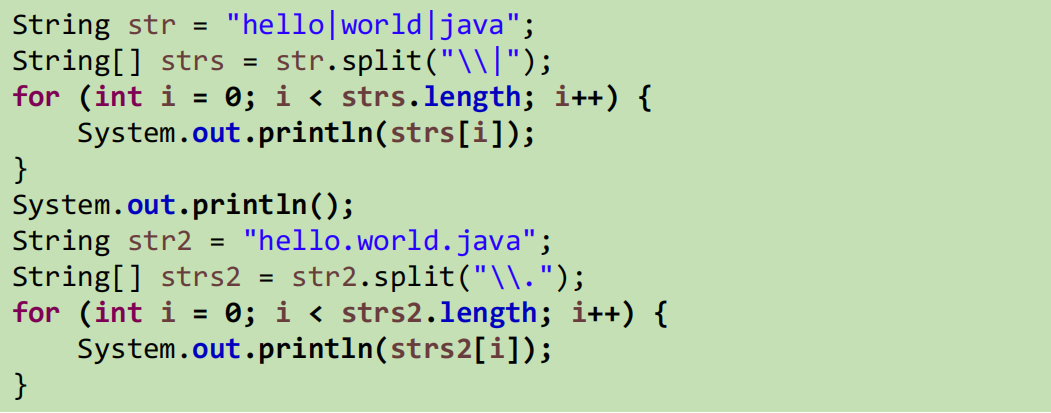
?
String和char[]的相互转换
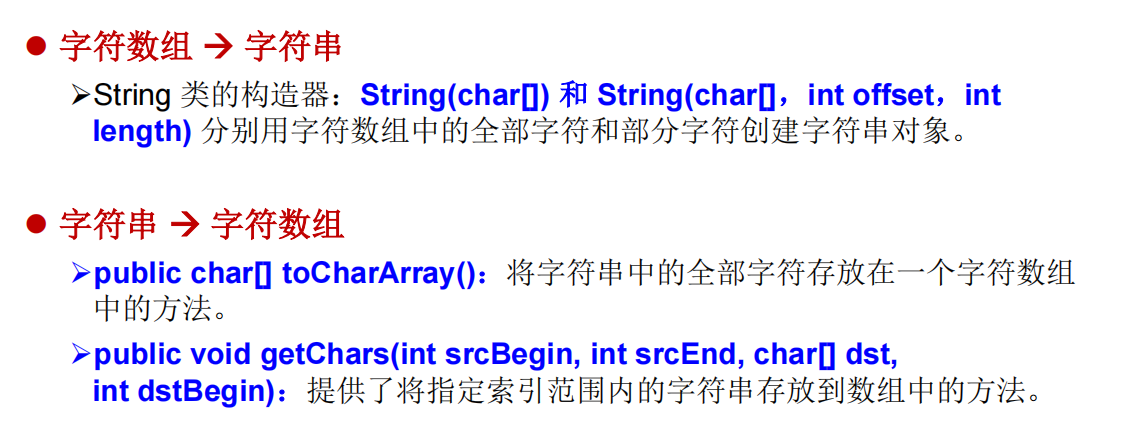
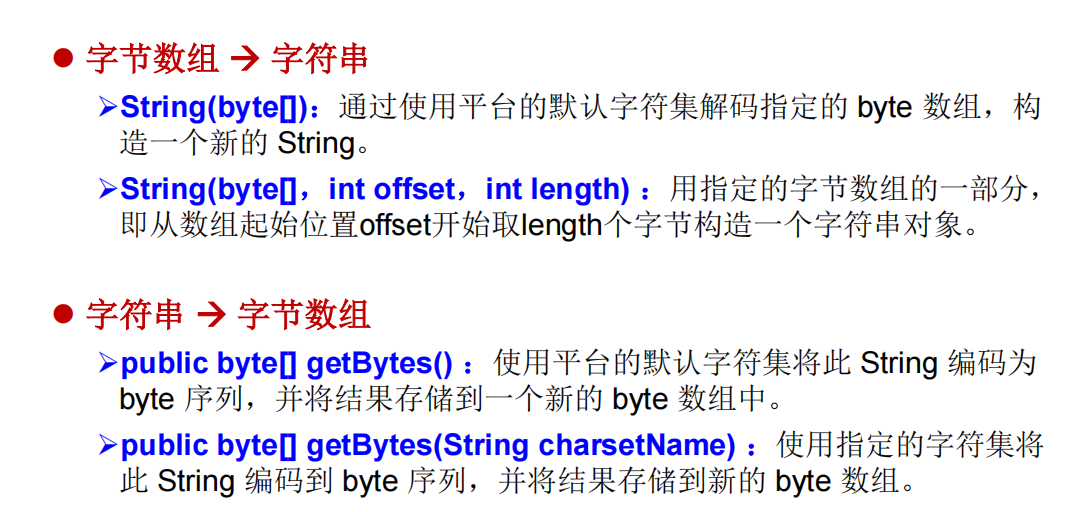
?
实现代码
package com.String;
import org.junit.Test;
// String与其他类型的转换
public class StringDemo {
// String --> 基本数据类型、包装类:调用包装类的静态方法:parseXxx(str)
// 基本数据类型、包装类 --> String:调用String重载的valueOf(xxx)
@Test
public void test1() {
String s1 = "100";
int i = Integer.parseInt(s1);
String s2 = String.valueOf(i);
}
@Test
public void test2() {
// String --> char[]:调用String的toCharArray()
// char[] --> String:调用String的构造器
String s1 = "hello world";
char[] chars = s1.toCharArray();
for (char i:chars) {
System.out.println(i);
}
char[] char1 = new char[]{'a','b','c'};
String str = new String(char1);
System.out.println(str);
}
@Test
public void tets3(){
// 编码:String --> byte[]:调用String的getBytes()
// 解码:byte[] --> String:调用String的构造器
String s1 = "hello world";
byte[] bytes = s1.getBytes();
for (byte a : bytes) {
System.out.println(a);
}
byte[] b = new byte[]{'h','e','l','l','o'};
String s = new String(b);
System.out.println(s);
}
}
String s = "169";
byte b = Byte.parseByte( s );
short t = Short.parseShort( s );
int i = Integer.parseInt( s );
long l = Long.parseLong( s );
Float f = Float.parseFloat( s );
Double d = Double.parseDouble( s );
StringBuffer
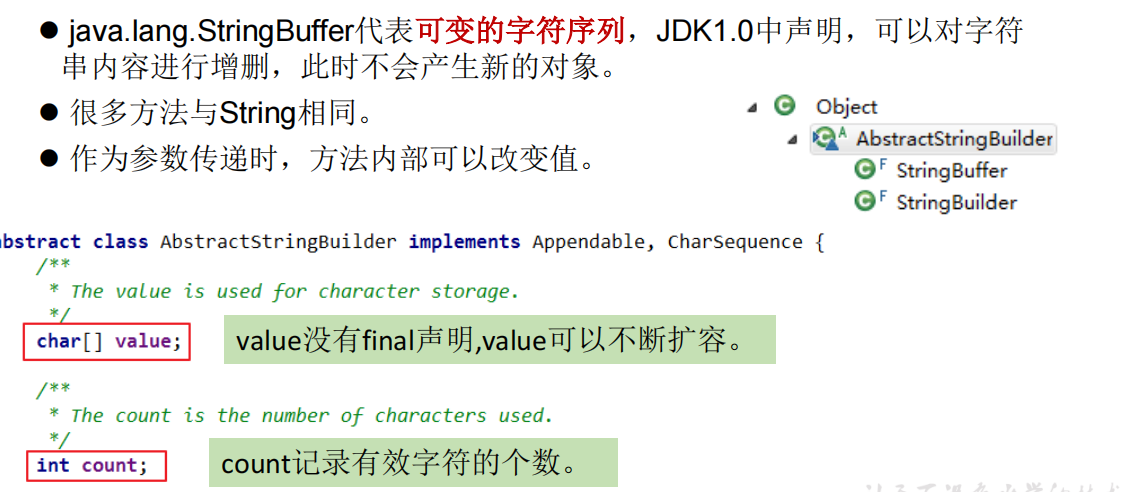
?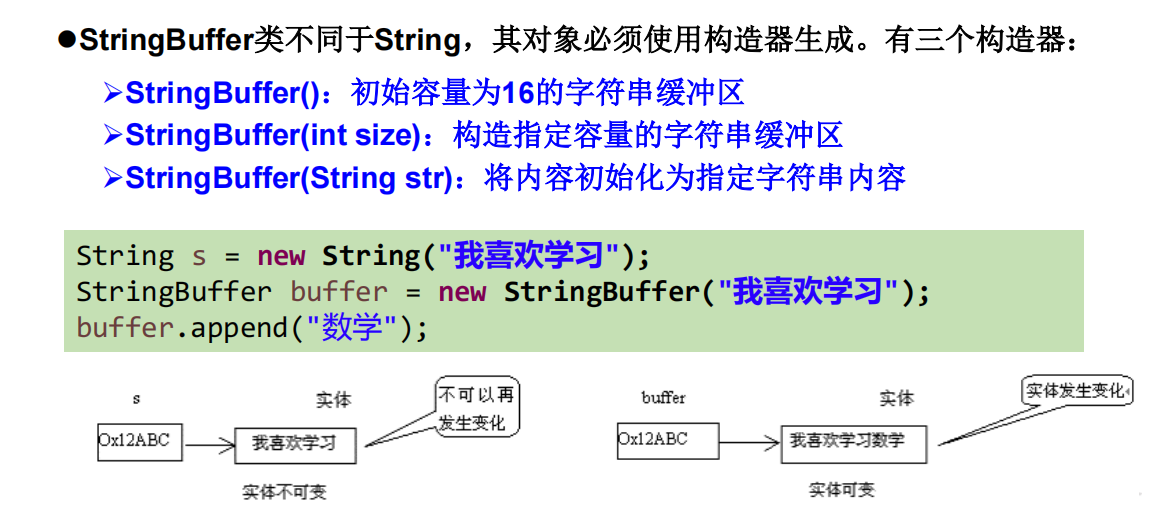
?
StringBuffer常用方法
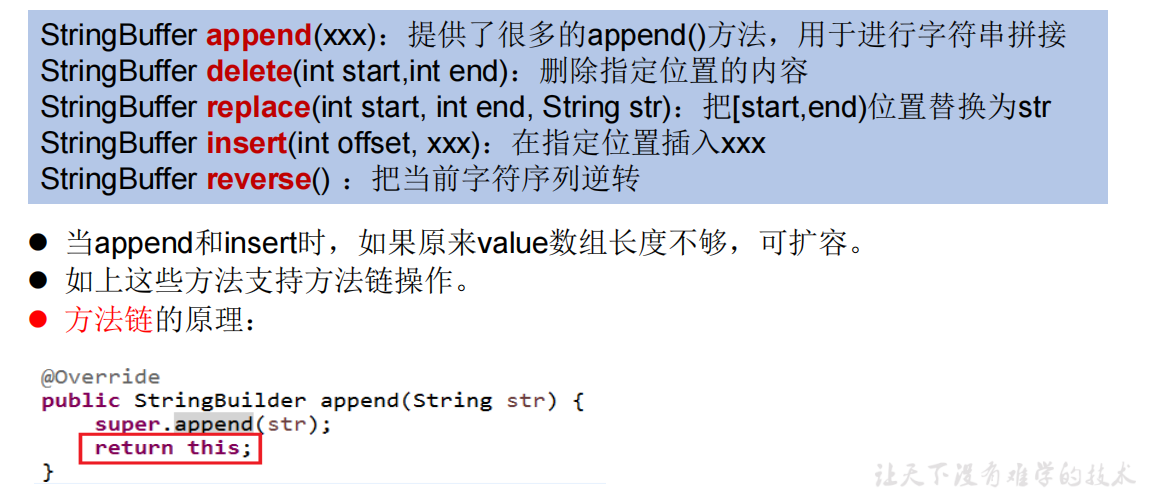
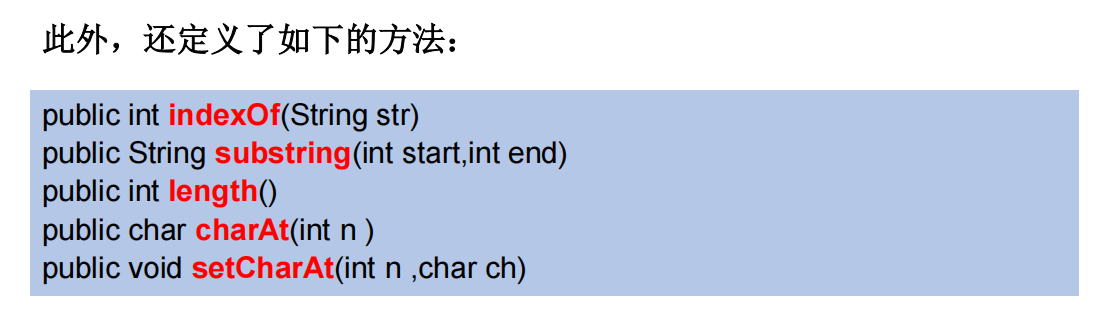
?
StringBuilder
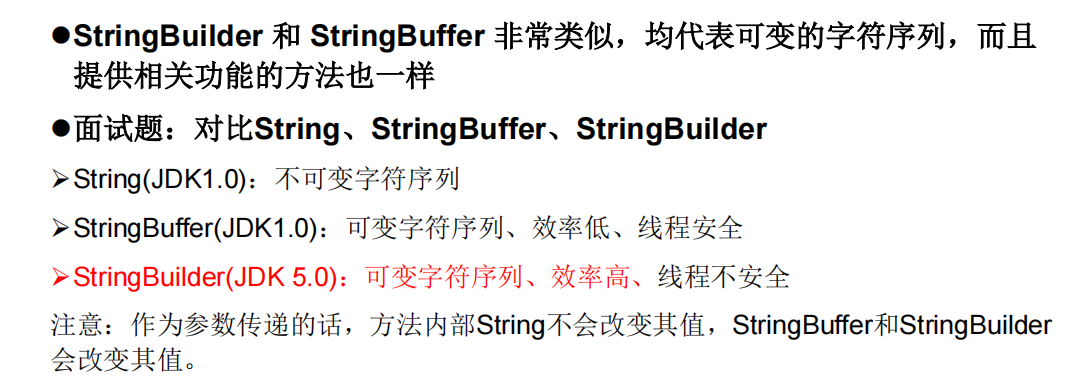

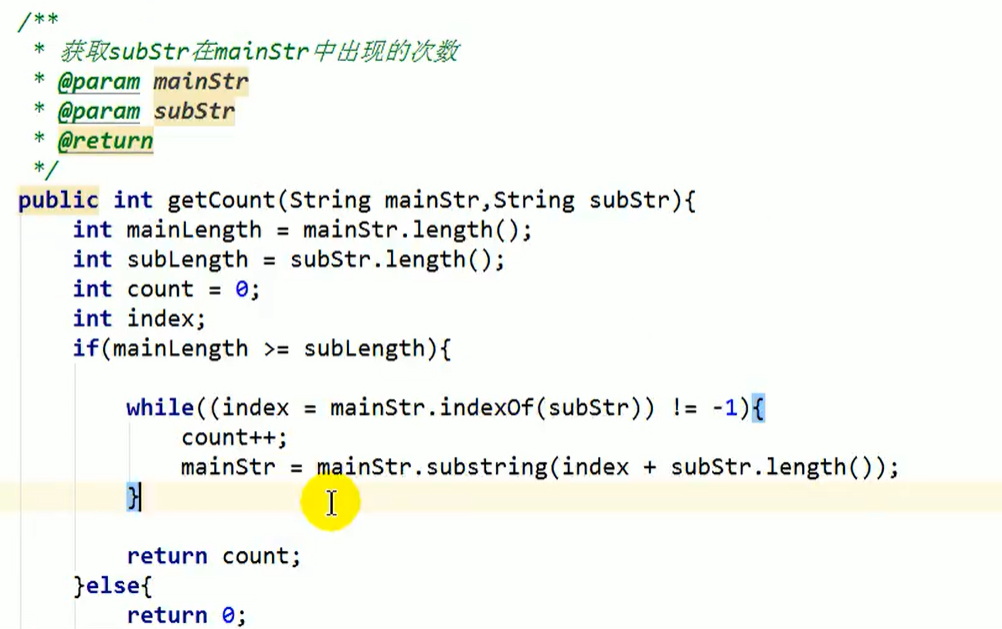 
JDK8之前日期时间API
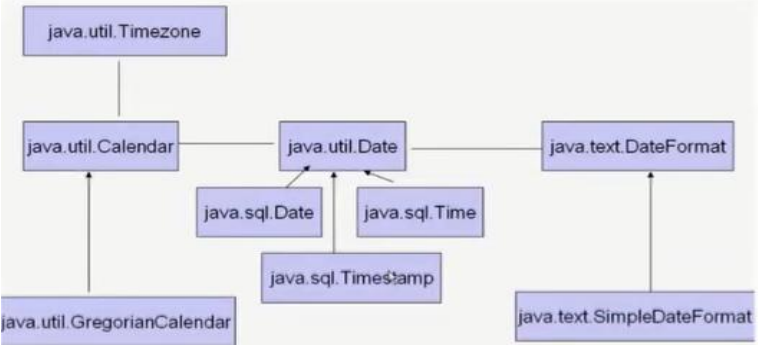
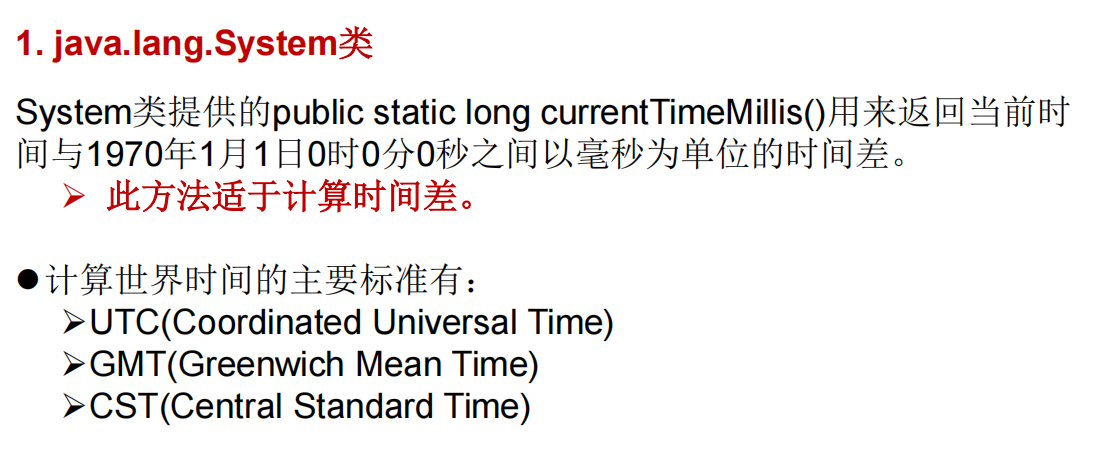
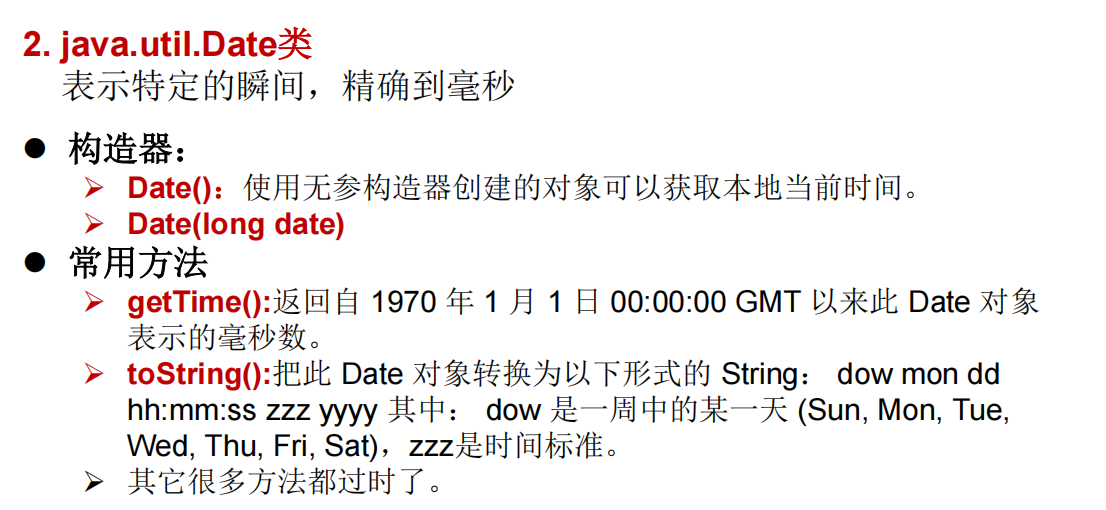
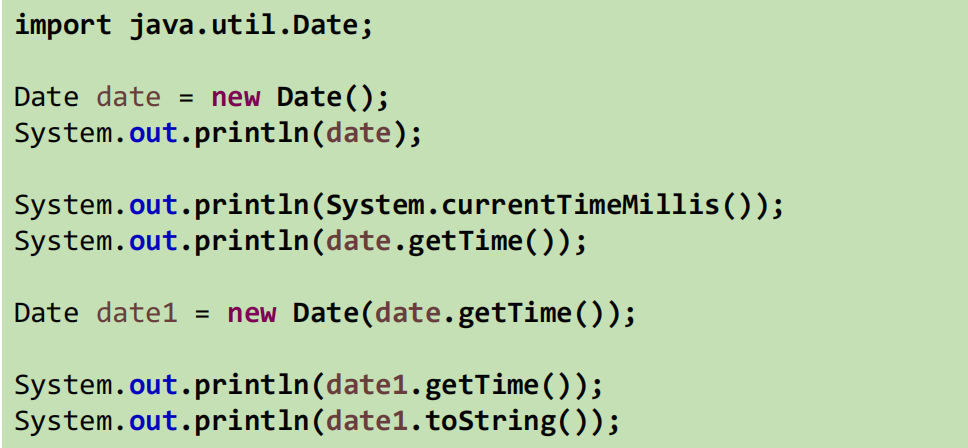
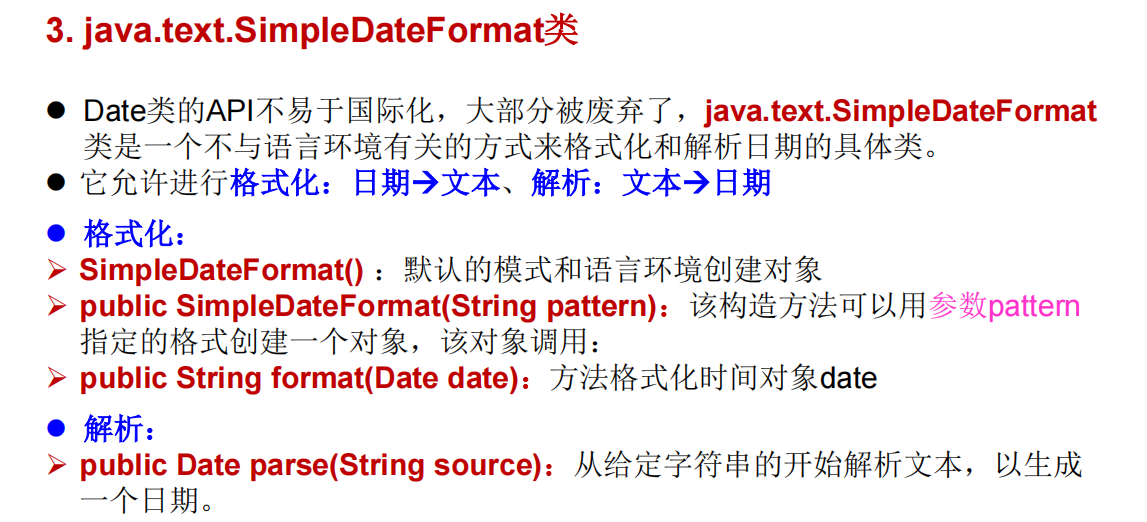
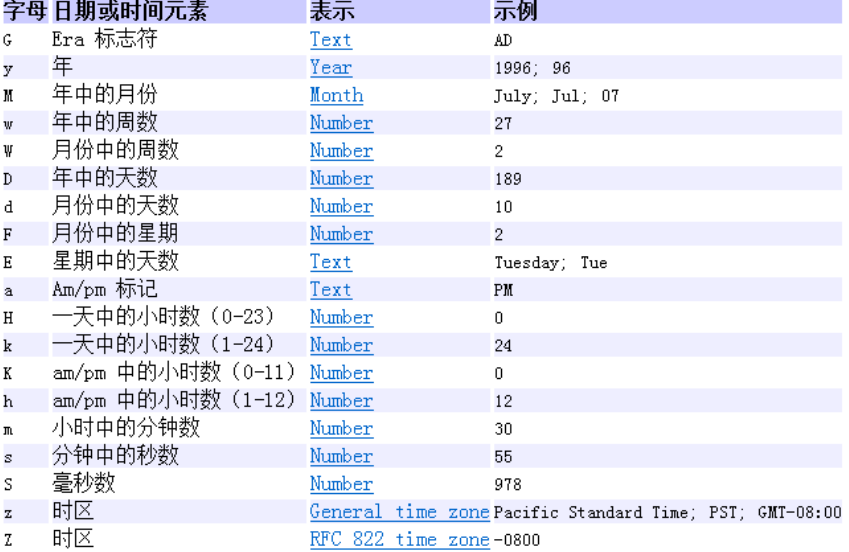
?
Date类
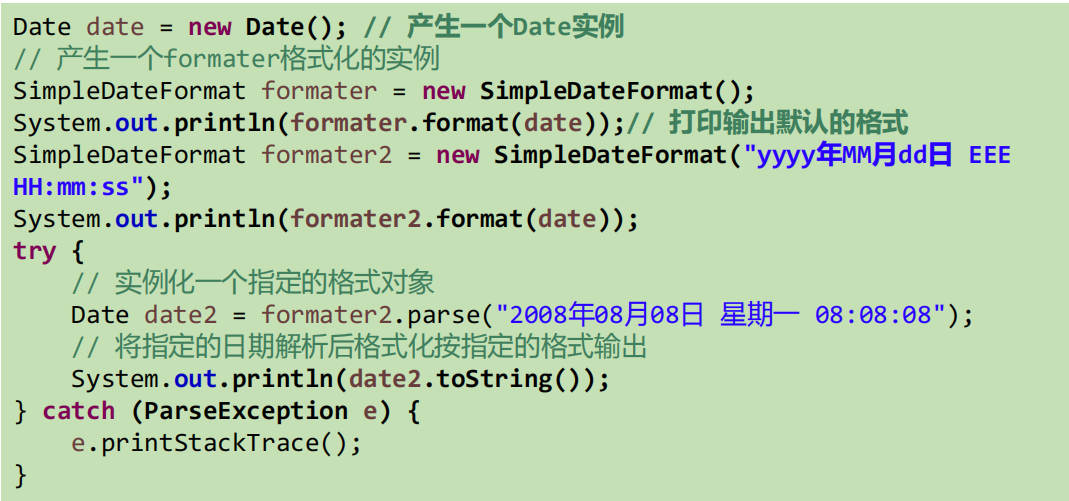
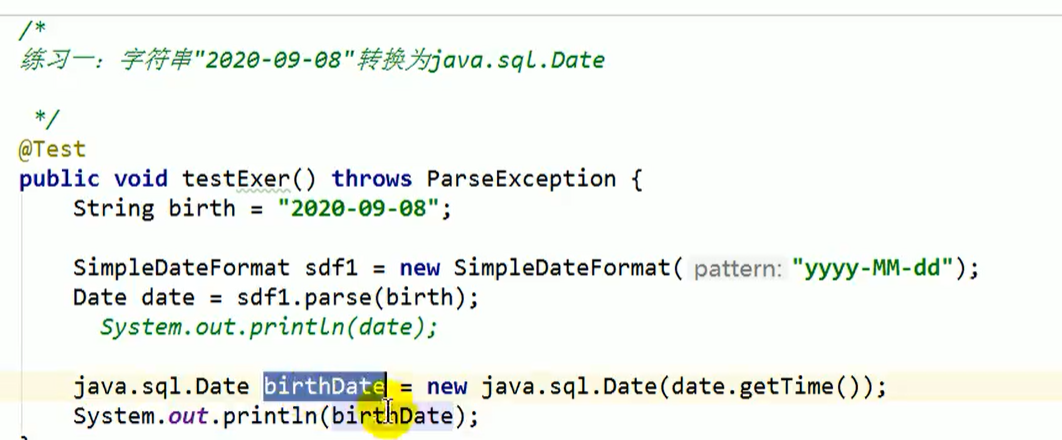
?
Calendar日历类
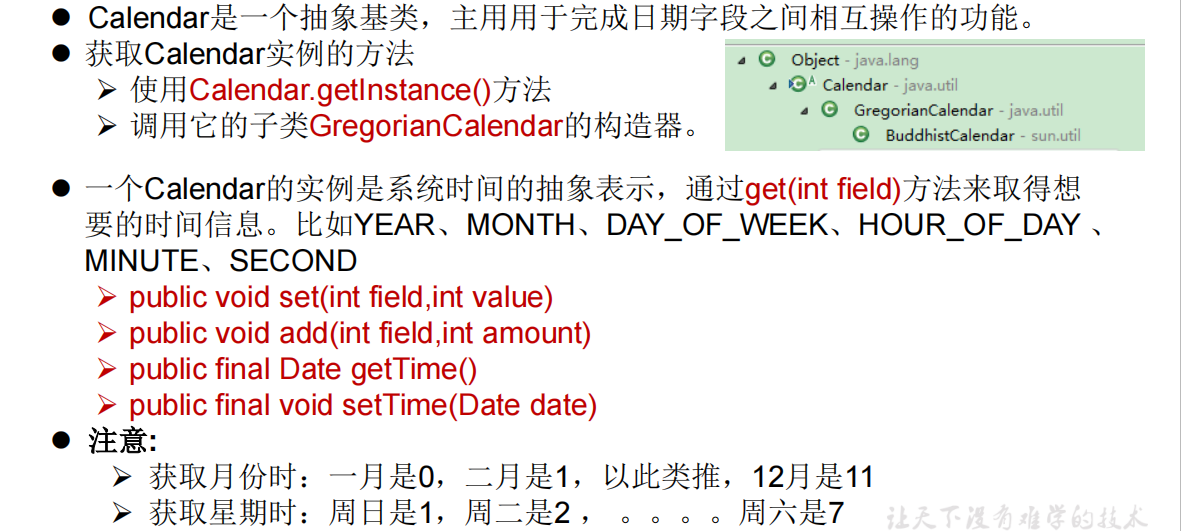
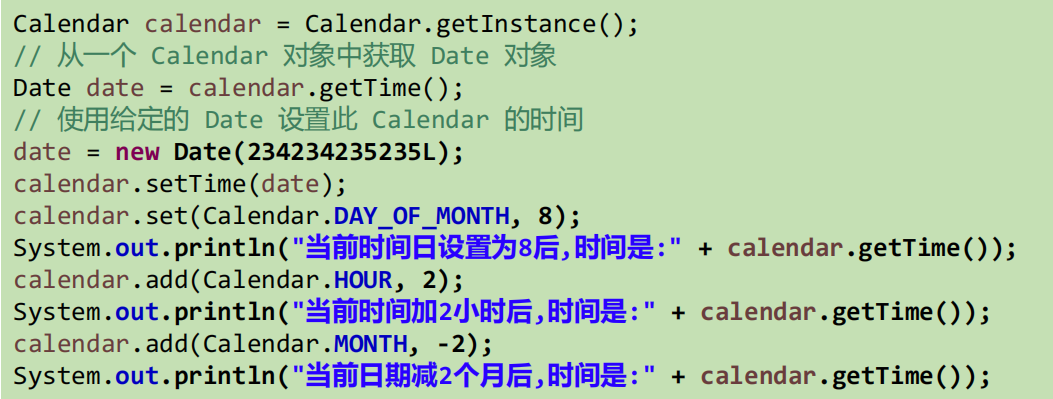
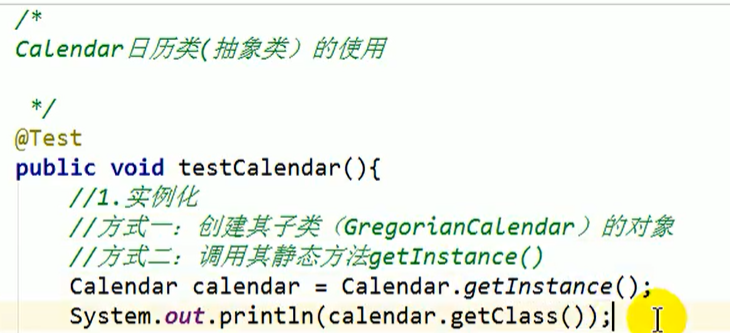
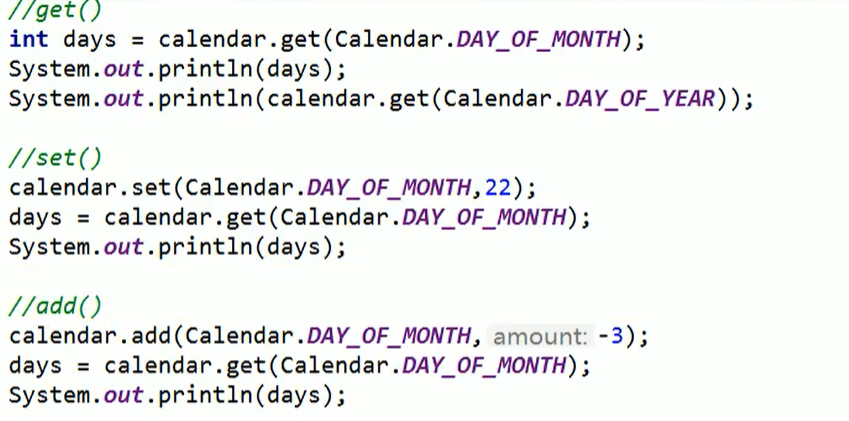
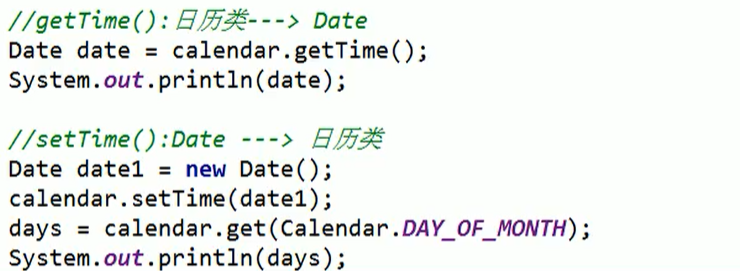
常用方法的使用:
package com.ykx.java;
import org.junit.Test;
import java.util.Calendar;
import java.util.Date;
/**
* @author: yangkx
* @Title: DateTest
* @ProjectName: JavaSenior
* @Description:
* @date: 2022/2/9 11:35
* Calendar日历类的使用
*/
public class DateTest {
@Test
public void test(){
Calendar calendar = Calendar.getInstance();
System.out.println(calendar.getClass());
System.out.println("常用方法举例:");
//get()
System.out.println("====== get():======");
int days = calendar.get(Calendar.DAY_OF_MONTH);
System.out.println(days);
System.out.println(calendar.get(Calendar.DAY_OF_YEAR));
//set()
System.out.println("====== set():======");
calendar.set(Calendar.DAY_OF_MONTH, 22);
System.out.println(calendar.get(Calendar.DAY_OF_MONTH));
//add()
System.out.println("====== addt():======");
calendar.add(Calendar.DAY_OF_MONTH, 5);
System.out.println(calendar.get(Calendar.DAY_OF_MONTH));
//getTime(): 日历类 --> Date
System.out.println("====== getTime():======");
Date date = calendar.getTime();
System.out.println(date);
//setTime(): Date --> 日历类
System.out.println("====== setTime():======");
Date date1 = new Date();
calendar.setTime(date1);
System.out.println(calendar.get(Calendar.DAY_OF_MONTH));
}
}
JDK8中新日期时间API
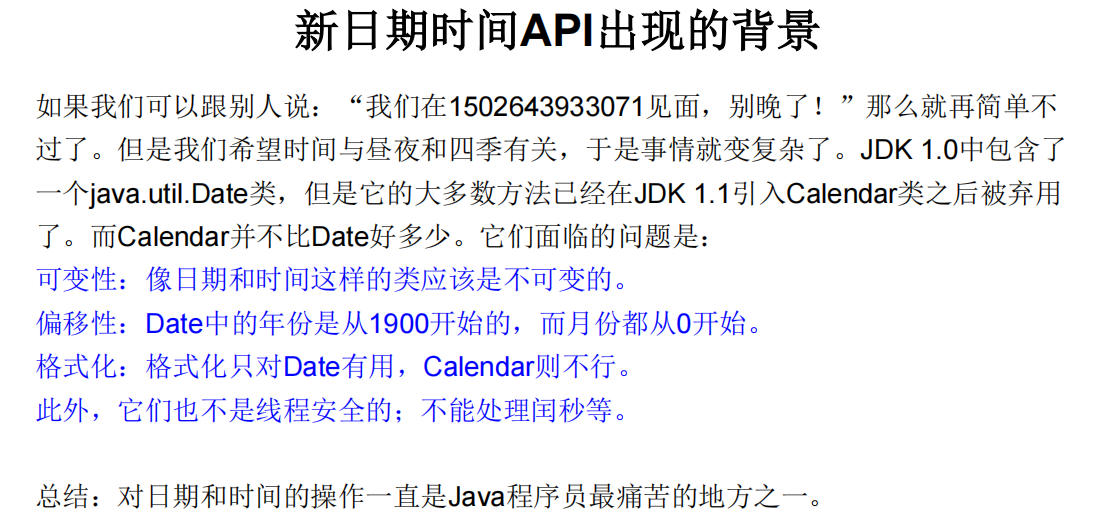
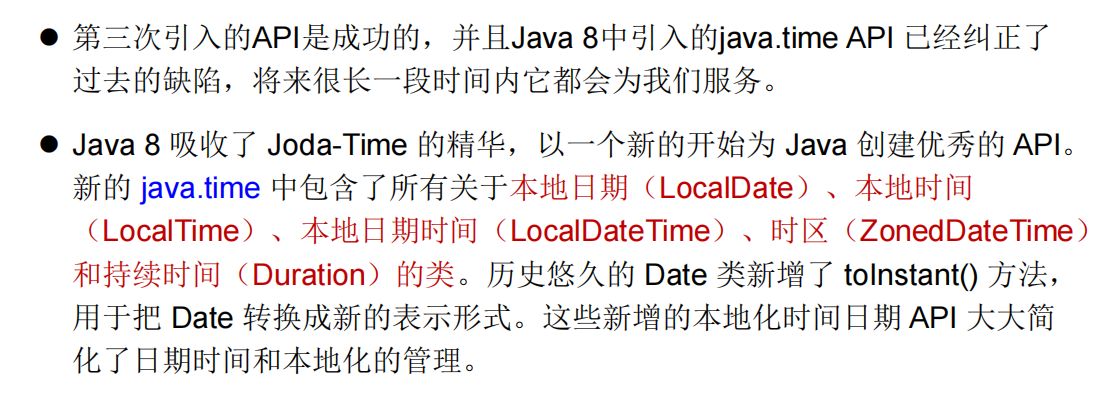 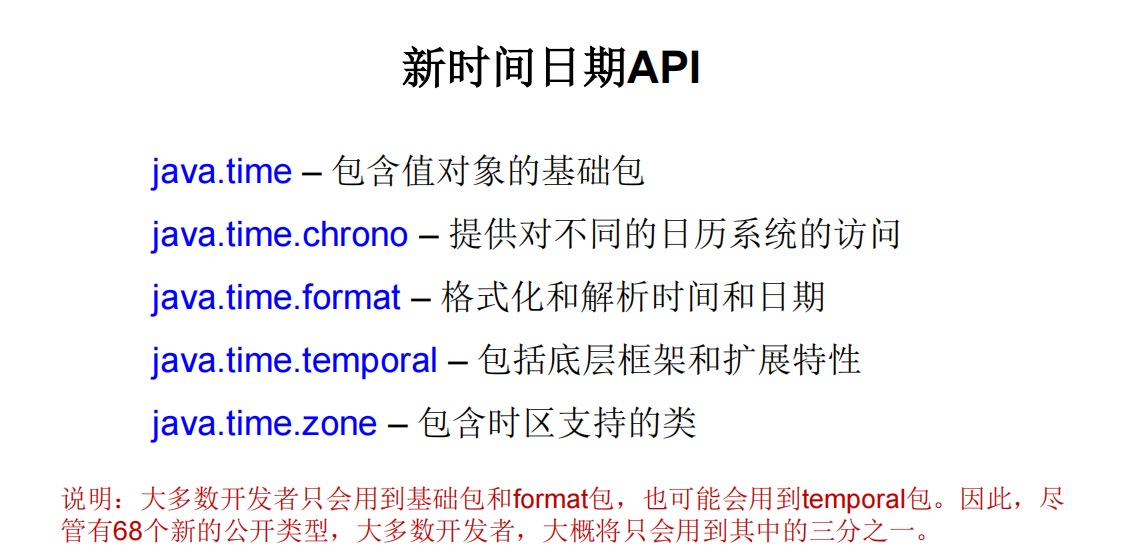
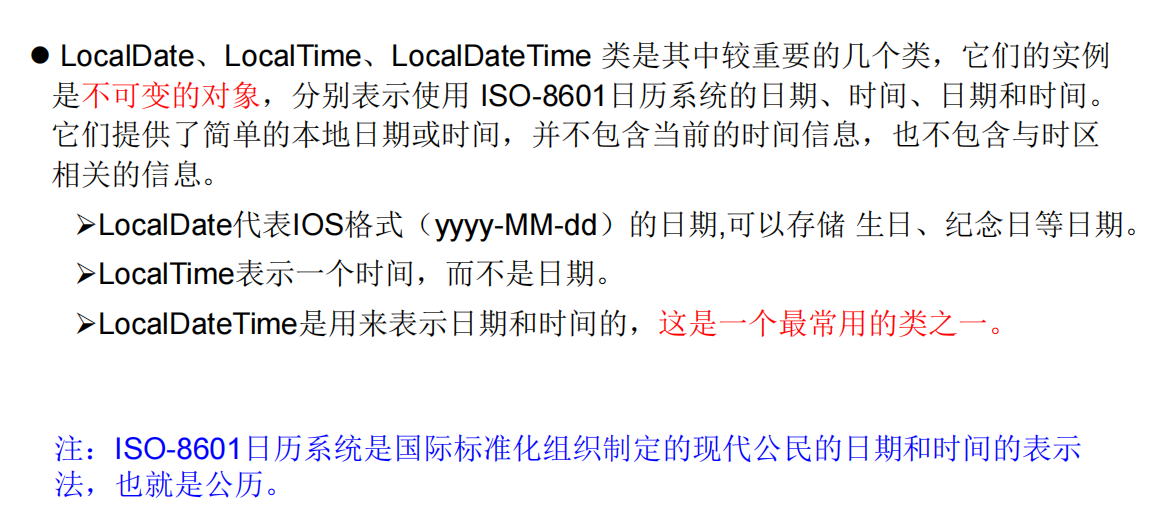
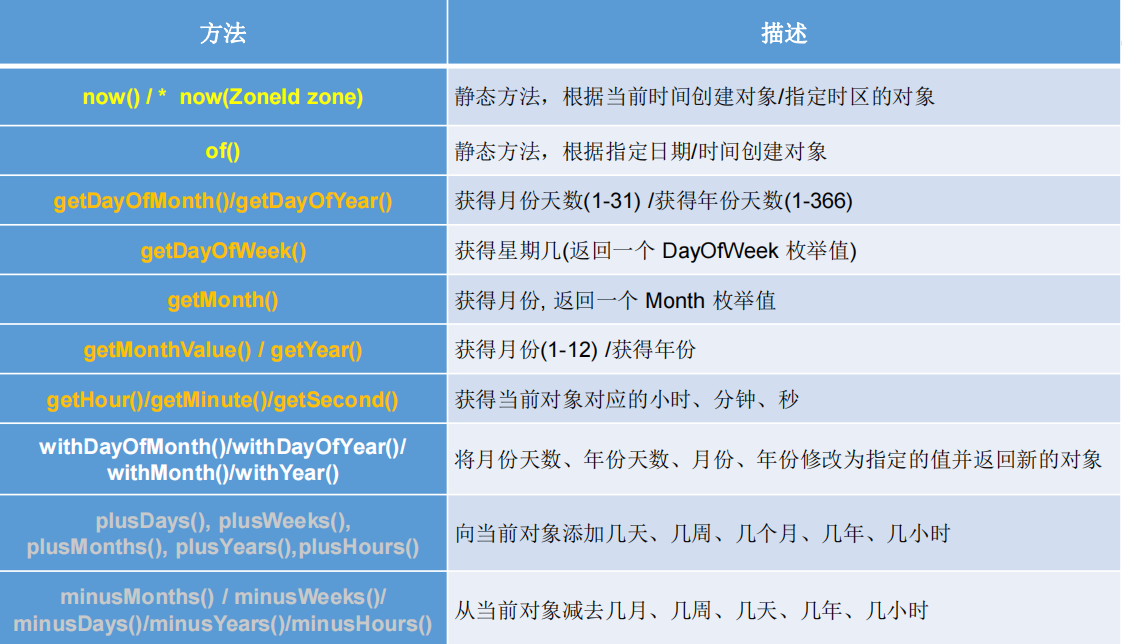
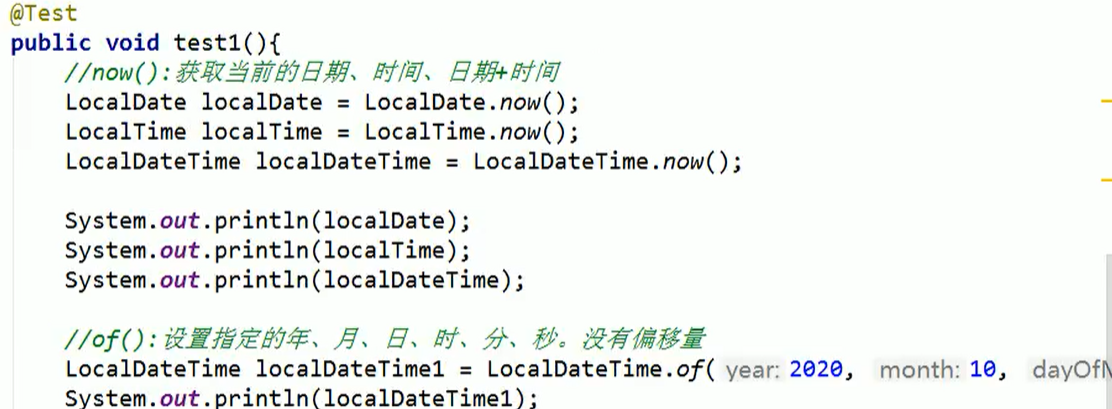
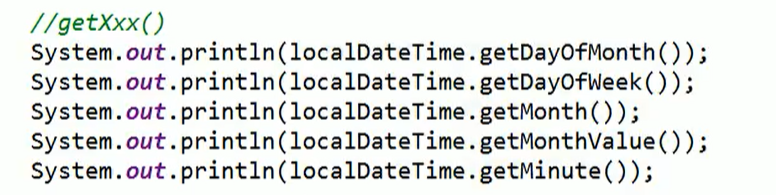
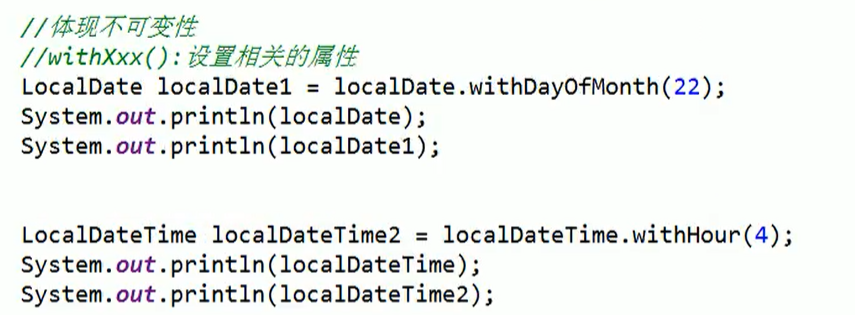
?
package com.ykx.java;
import org.junit.Test;
import java.time.LocalDateTime;
/**
* @author: yangkx
* @Title: NewDateTest
* @ProjectName: JavaSenior
* @Description:
* @date: 2022/2/9 12:08
* JDK8中新的日期时间类使用
*/
public class NewDateTest {
@Test
public void test(){
LocalDateTime localDateTime = LocalDateTime.now();
System.out.println(localDateTime);
System.out.println(localDateTime.getMonthValue());
LocalDateTime localDateTime1 = localDateTime.withMonth(9);
System.out.println(localDateTime1);
}
}
?
Instant类
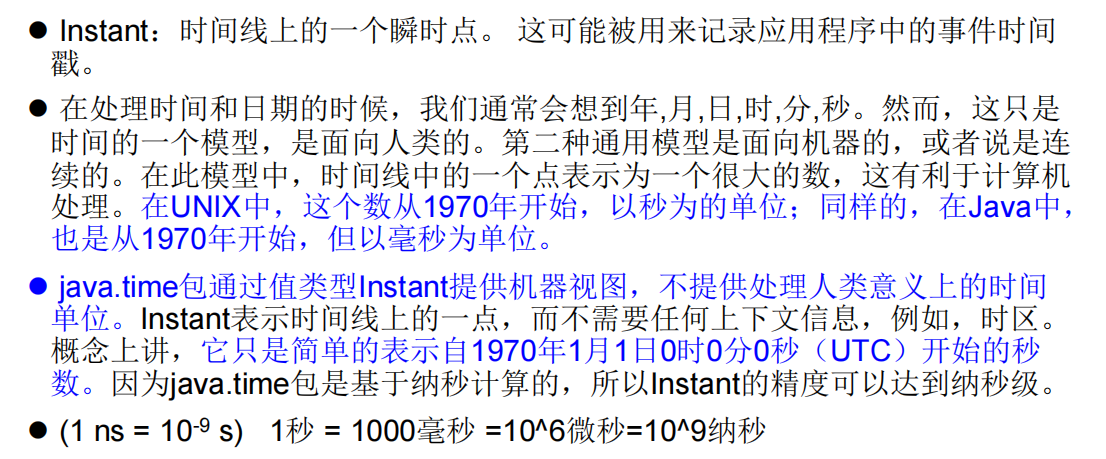
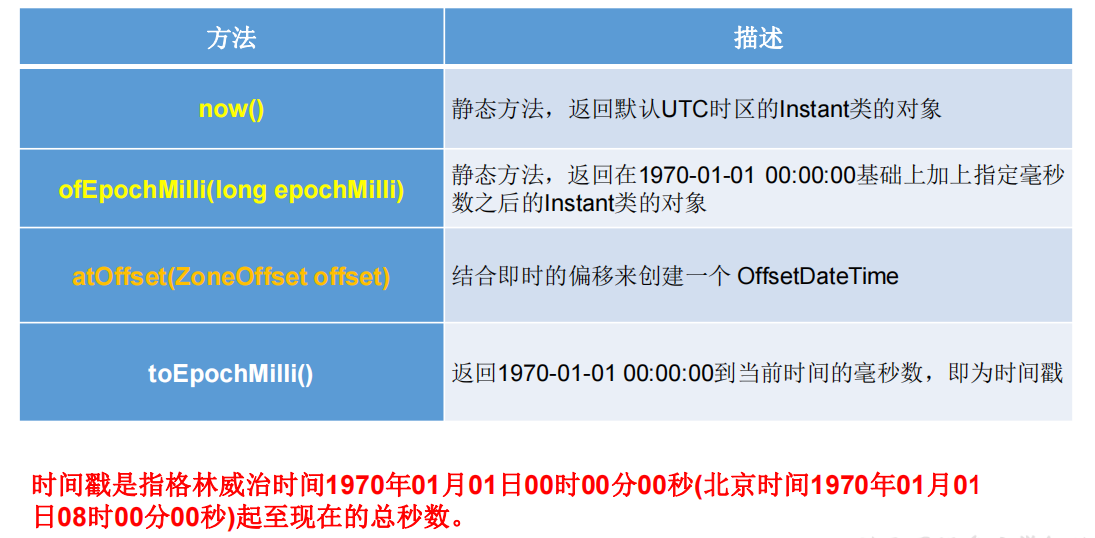
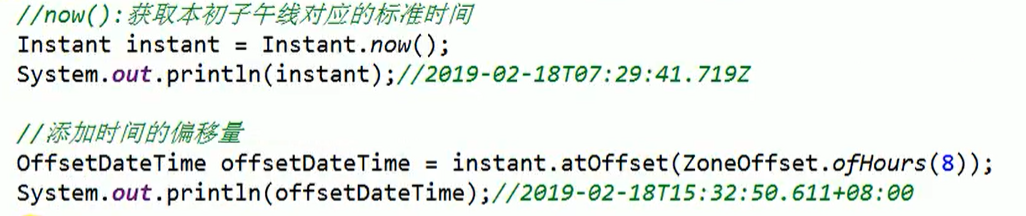
?
格式化与解析日期或时间
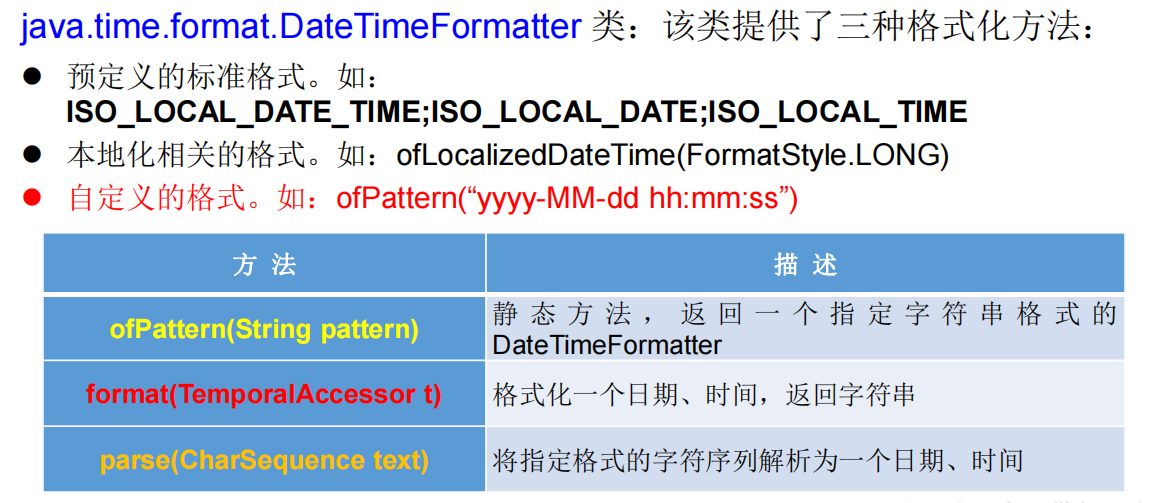

?
实例化的三种方式

 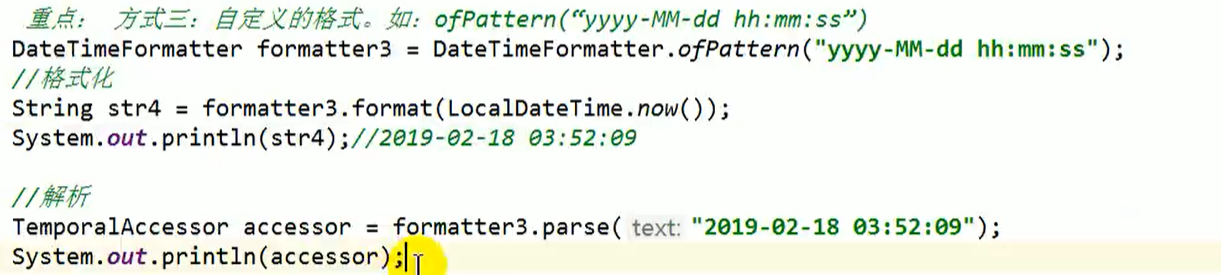
Java比较器
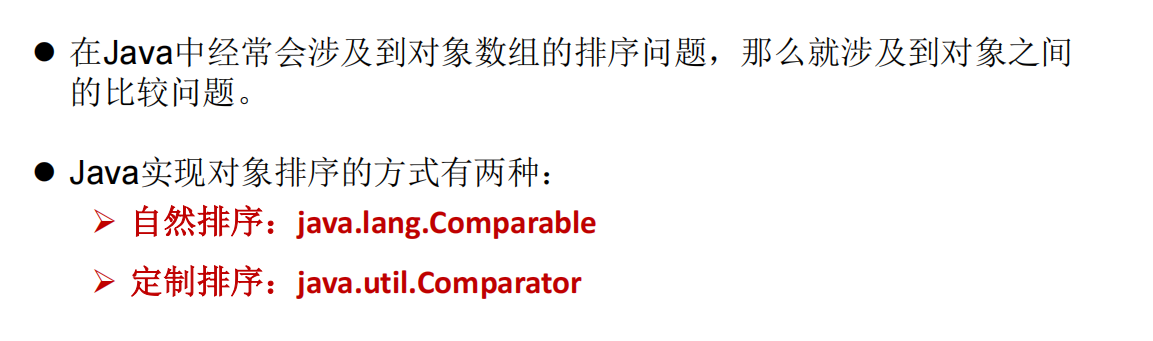 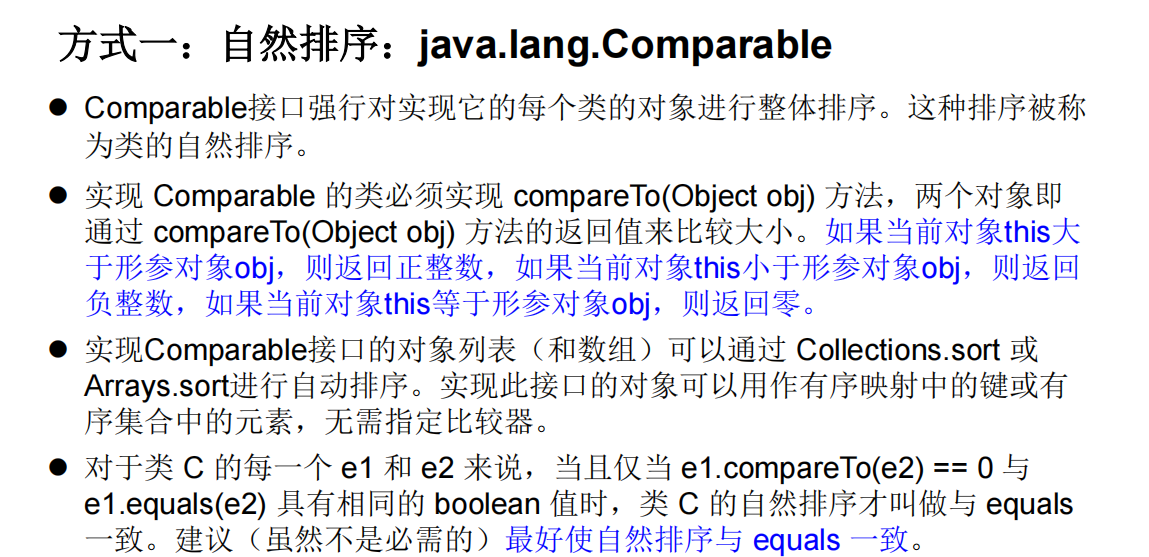
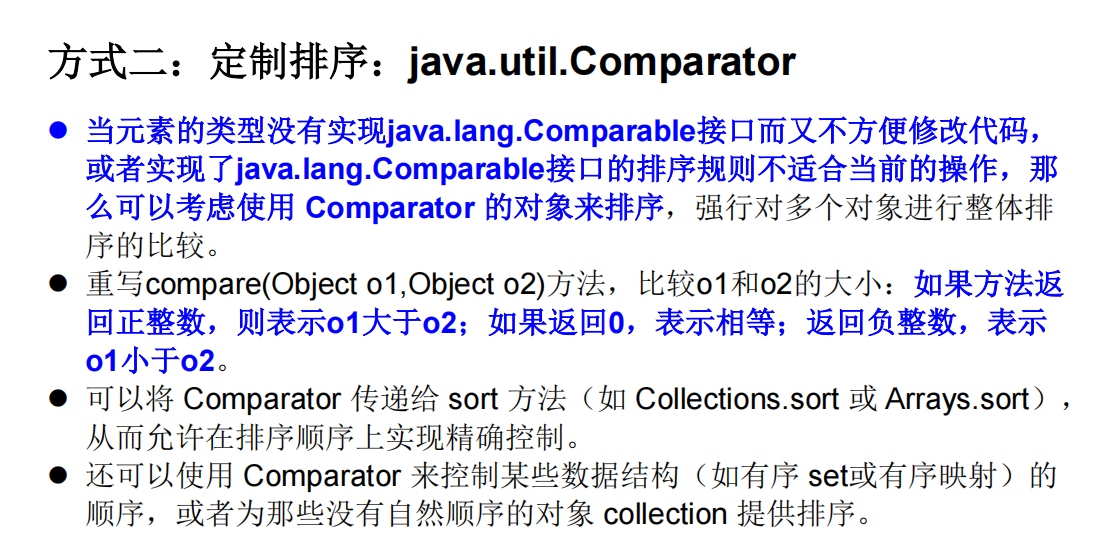
?
?
Comparable接口
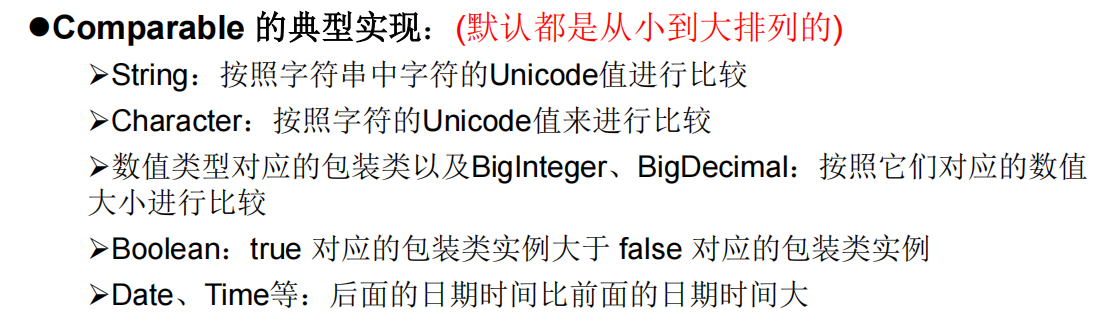

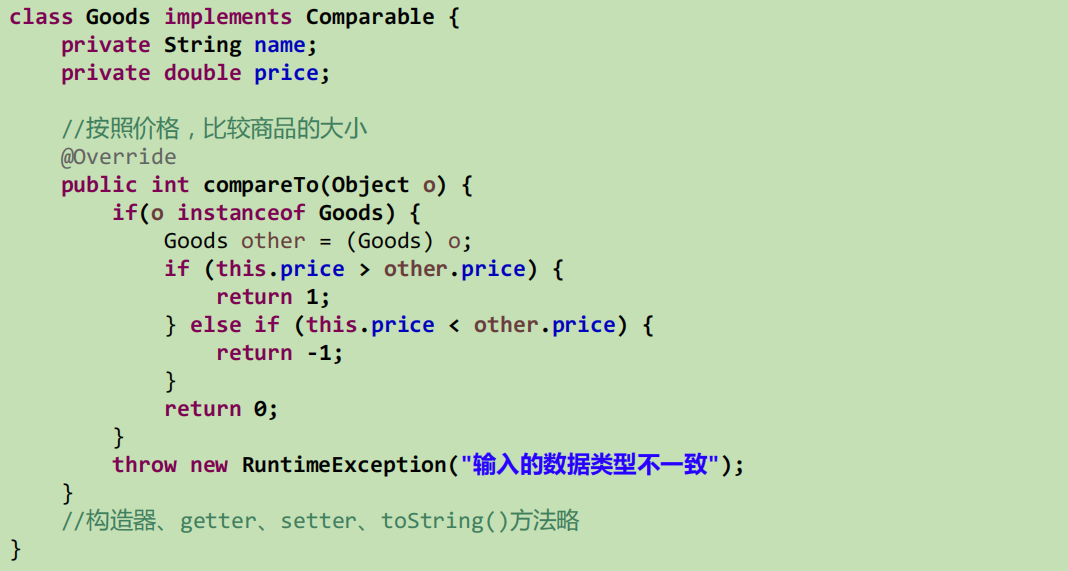
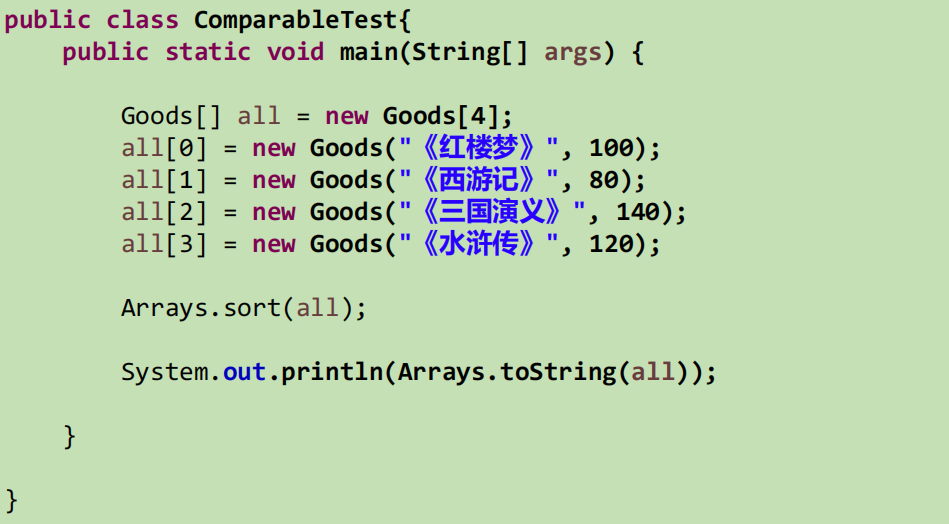
?
package com.ykx.java;
import org.junit.Test;
import java.util.Arrays;
/**
* @author: yangkx
* @Title: CmpTest
* @ProjectName: JavaSenior
* @Description:
* @date: 2022/2/9 14:22
* comparable排序的实现
*/
public class CmpTest {
@Test
public void test() {
Goods[] gs = new Goods[4];
gs[0] = new Goods(12,1);
gs[1] = new Goods(50,2);
gs[2] = new Goods(50,3);
gs[3] = new Goods(6,4);
Arrays.sort(gs);
System.out.println(Arrays.toString(gs));
}
}
class Goods implements Comparable{
int price;
int num;
Goods(){
}
public Goods(int price, int num) {
this.price = price;
this.num = num;
}
@Override
public int compareTo(Object o) {
Goods g = (Goods) o;
if(this.price != g.price){
return -(this.price - g.price);//从大到小
}else{
return (this.num - g.num);//从小到大
}
}
@Override
public String toString() {
return "Goods{" +
"price=" + price +
", num=" + num +
'}';
}
}
?
Comparator排序
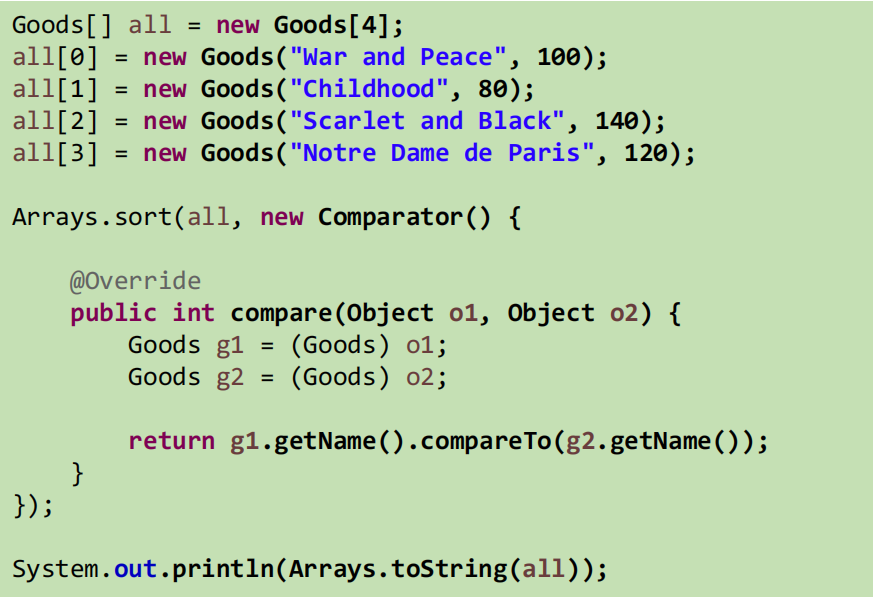
实际在写算法题时用到的排序方法
class Solution {
// 区间调度问题
public int findMinArrowShots(int[][] intvs) {
if (intvs.length == 0) return 0;
// 按 end 升序排序
Arrays.sort(intvs, new Comparator<int[]>() {
public int compare(int[] a, int[] b) {
return a[1] - b[1];
}
});
// 至少有一个区间不相交
int count = 1;
// 排序后,第一个区间就是 x
int x_end = intvs[0][1];
for (int[] interval : intvs) {
int start = interval[0];
// 把 >= 改成 > 就行了
if (start > x_end) {
count++;
x_end = interval[1];
}
}
return count;
}
}
System类
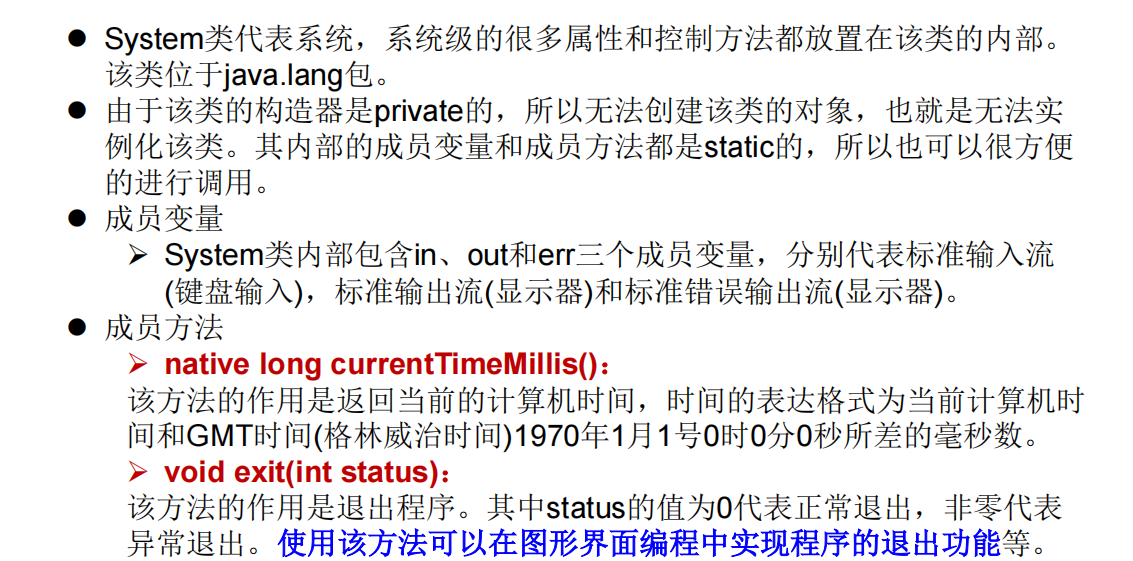
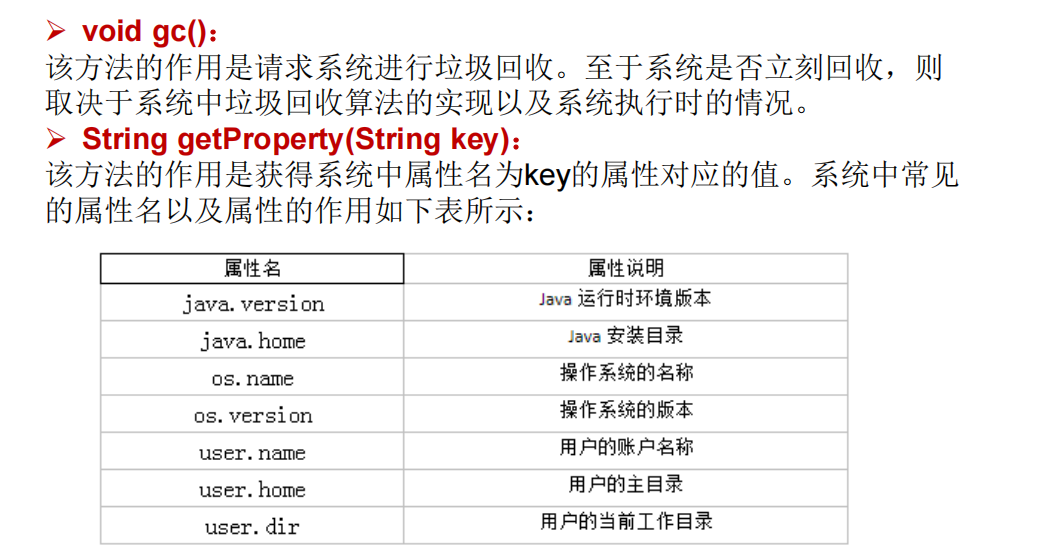
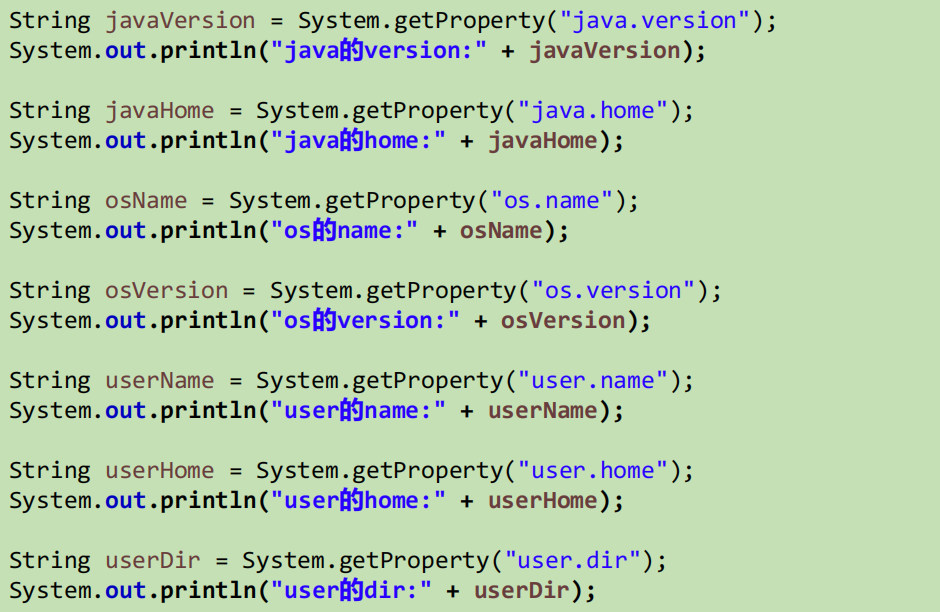
Math类
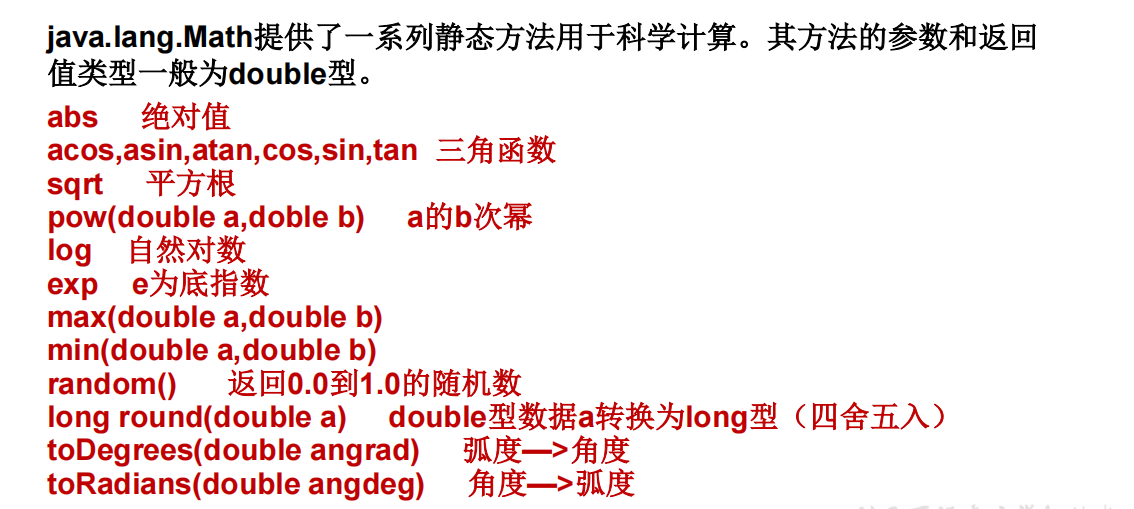
?
BigInteger类
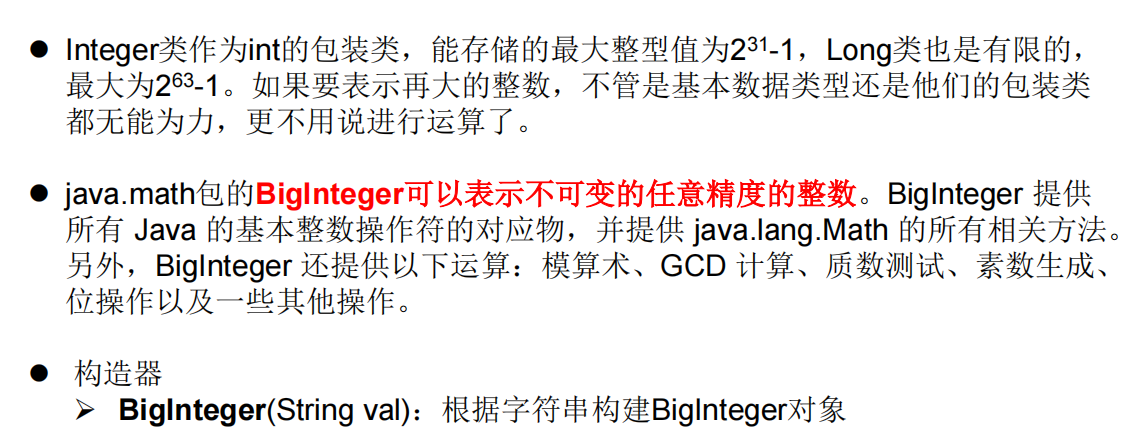
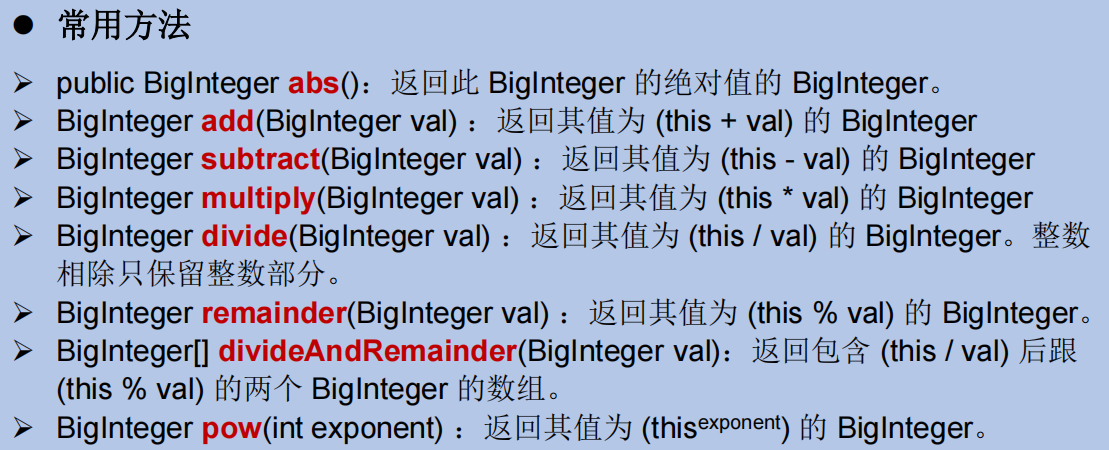
?
BigDecimal类
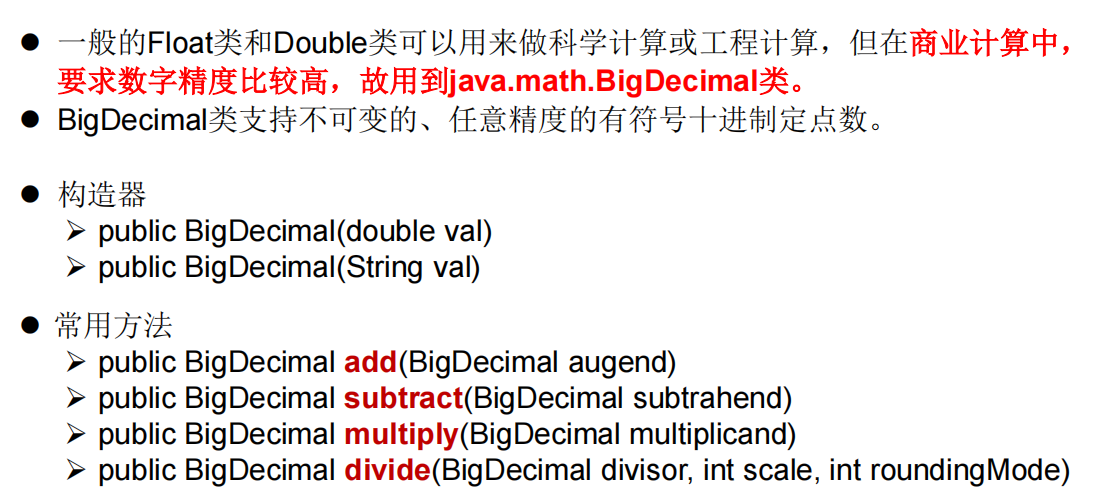
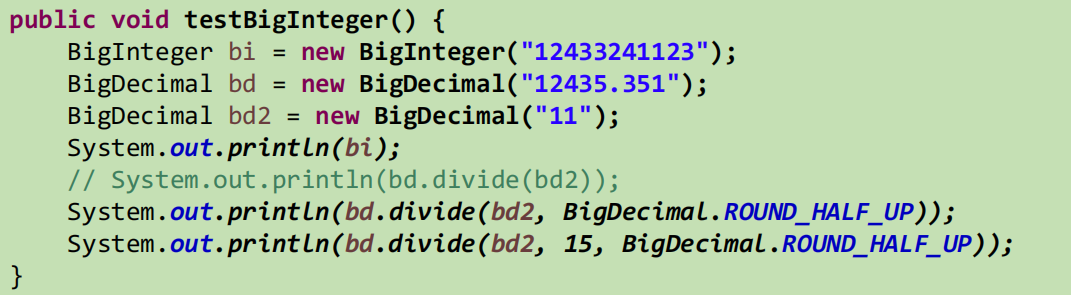
?
|