二维数组转换成稀疏数组并还原的过程
二维数组
二维数组与一维数组相似,但是用法上要比一维数组复杂一点。后面的编程中,二维数组用得很少,因为二维数组的本质就是一维数组,只不过形式上是二维的。能用二维数组解决的问题用一维数组也能解决。但是在某些情况下,比如矩阵,对于程序员来说使用二维数组会更形象直观,但对于计算机而言与一维数组是一样的。
二维数组的创建
创建一个11*11的棋盘,黑棋为1,白棋为2,没有棋则为0:(如图)?
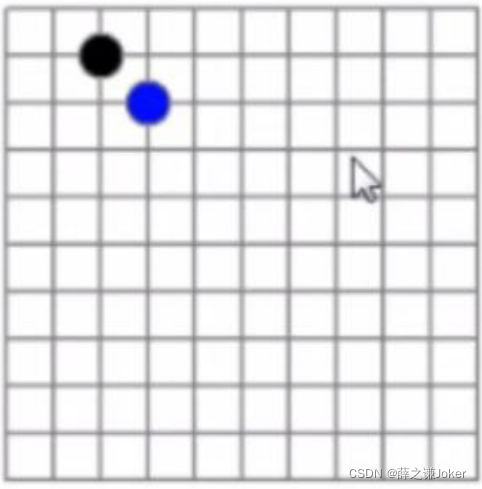
代码如下:
//1.创建11*11二维数组,0:没有棋子,1:黑棋,2:白棋
? ? ? ?int[][] array1 = new int[11][11];
? ? ? ?array1 [1][2]=1;
? ? ? ?array1 [2][3]=2;
//输出原数组
? ? ? ?System.out.println("原数组:");
? ? ? ?for (int i = 0; i < array1.length; i++) {
? ? ? ? ? ?for (int j = 0; j < array1[i].length; j++) {
? ? ? ? ? ? ? ?System.out.print(array1[i][j]+"\t");
? ? ? ? ? }
? ? ? ? ? ?System.out.println();
? ? ? }
System.out.println("============================================");
输出结果:
0 0 0 0 0 0 0 0 0 0 0
0 0 1 0 0 0 0 0 0 0 0
0 0 0 2 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
稀疏数组
稀疏数组可以看做是普通数组的压缩,但是这里说的普通数组是值无效数据量远大于有效数据量的数组
例如
原数组:
? ? ? ? ?0 0 0 0 0 0 0 0 0 0 0
? ? ? ? ?0 0 1 0 0 0 0 0 0 0 0
? ? ? ? ?0 0 0 0 2 0 0 0 0 0 0
? ? ? ? ?0 0 0 0 0 0 0 0 0 0 0
? ? ? ? ?0 0 0 0 0 0 0 0 0 0 0
? ? ? ? ?0 0 0 0 0 0 0 0 0 0 0
? ? ? ? ?0 0 0 0 0 0 0 0 0 0 0
? ? ? ? ?0 0 0 0 0 0 0 0 0 0 0
? ? ? ? ?0 0 0 0 0 0 0 0 0 0 0
? ? ? ? ?0 0 0 0 0 0 0 0 0 0 0
? ? ? ? ?0 0 0 0 0 0 0 0 0 0 0
稀疏数组:
? ? ? ? ?11 11 2
? ? ? ? ?1 ?2 ?1
? ? ? ? ?2 ?4 ?2
稀疏数组的意义
刚说到稀疏数组是一种压缩后的数组,为什么要进行压缩存储呢?
稀疏数组的创建
代码如下:
//创建稀疏函数
? ? ? ?int sum = 0;//原数组非零数的数量
? ? ? ?for (int i = 0; i < array1.length; i++) {
? ? ? ? ? ?for (int j = 0; j < array1[i].length; j++) {
? ? ? ? ? ? ? ?if (array1[i][j]!=0){
? ? ? ? ? ? ? ? ? ?sum++;
? ? ? ? ? ? ? }
? ? ? ? ? }
? ? ? }
? ? ? ?int[][] array2 = new int[sum+1][3];
? ? ? ?array2[0][0] = 11;//原数组行数
? ? ? ?array2[0][1] = 11;//原数组列数
? ? ? ?array2[0][2] = sum;//原数组非零数的数量
? ? ? ?int x = 1;//从第二行开始向稀疏素组写入数据
? ? ? ?for (int i = 0; i < array1.length; i++) { ? //循环原数组的行
? ? ? ? ? ?for (int j = 0; j < array1[i].length; j++) {//循环原数组的列
? ? ? ? ? ? ? ?if (array1[i][j]!=0){ ? ? ? ? ? ? ? //找到原数组非零的数据
? ? ? ? ? ? ? ? ? ?array2[x][0] = i; ? ? ? ? ? ? ? //向稀疏素组写入非零数据的行
? ? ? ? ? ? ? ? ? ?array2[x][1] = j; ? ? ? ? ? ? ? //向稀疏素组写入非零数据的列
? ? ? ? ? ? ? ? ? ?array2[x][2] = array1[i][j]; ? ?//向稀疏素组写入非零数据的值
? ? ? ? ? ? ? ? ? ?x++;//向下一行写入
? ? ? ? ? ? ? }
? ? ? ? ? }
? ? ? }
//输出稀疏素组
? ? ? ?System.out.println("稀疏素组:");
? ? ? ?for (int i = 0; i < array2.length; i++) {
? ? ? ? ? ?for (int j = 0; j < array2[i].length; j++) {
? ? ? ? ? ? ? ?System.out.print(array2[i][j]+"\t");
? ? ? ? ? }
? ? ? ? ? ?System.out.println();
? ? ? }
System.out.println("============================================");
输出结果:
稀疏素组:
11 11 2
1 2 1
2 3 2
稀疏数组还原成原二维数组
//还原
? ? ? ?int[][] array3 = new int[array2[0][0]][array2[0][1]]; ? //创建第三个数组,行为稀疏素组的[0][0],列为稀疏素组的[0][1]
? ? ? ?for (int i = 1; i < array2.length; i++) { ? ? ? ? ? ? ? //从第二行遍历稀疏素组
? ? ? ? ? ?array3[array2[i][0]][array2[i][1]]=array2[i][2]; ? ?//向数组3写入数据
? ? ? }
//输出
? ? ? ?System.out.println("还原结果:");
? ? ? ?for (int i = 0; i < array3.length; i++) {
? ? ? ? ? ?for (int j = 0; j < array3[i].length; j++) {
? ? ? ? ? ? ? ?System.out.print(array3[i][j]+"\t");
? ? ? ? ? }
? ? ? ? ? ?System.out.println();
? ? ? }
输出结果:
还原结果:
0 0 0 0 0 0 0 0 0 0 0
0 0 1 0 0 0 0 0 0 0 0
0 0 0 2 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
总结
全部代码:
public static void main(String[] args) {
? ? ? ?//1.创建11*11二维数组,0:没有棋子,1:黑棋,2:白棋
? ? ? ?int[][] array1 = new int[11][11];
? ? ? ?array1 [1][2]=1;
? ? ? ?array1 [2][3]=2;
? ? ? ?//输出原数组
? ? ? ?System.out.println("原数组:");
? ? ? ?for (int i = 0; i < array1.length; i++) {
? ? ? ? ? ?for (int j = 0; j < array1[i].length; j++) {
? ? ? ? ? ? ? ?System.out.print(array1[i][j]+"\t");
? ? ? ? ? }
? ? ? ? ? ?System.out.println();
? ? ? }
?
? ? ? ?System.out.println("============================================");
? ? ? ?//创建稀疏函数
? ? ? ?int sum = 0;//原数组非零数的数量
? ? ? ?for (int i = 0; i < array1.length; i++) {
? ? ? ? ? ?for (int j = 0; j < array1[i].length; j++) {
? ? ? ? ? ? ? ?if (array1[i][j]!=0){
? ? ? ? ? ? ? ? ? ?sum++;
? ? ? ? ? ? ? }
? ? ? ? ? }
? ? ? }
? ? ? ?int[][] array2 = new int[sum+1][3];
? ? ? ?array2[0][0] = 11;//原数组行数
? ? ? ?array2[0][1] = 11;//原数组列数
? ? ? ?array2[0][2] = sum;//原数组非零数的数量
? ? ? ?int x = 1;//从第二行开始向稀疏素组写入数据
? ? ? ?for (int i = 0; i < array1.length; i++) { ? //循环原数组的行
? ? ? ? ? ?for (int j = 0; j < array1[i].length; j++) {//循环原数组的列
? ? ? ? ? ? ? ?if (array1[i][j]!=0){ ? ? ? ? ? ? ? //找到原数组非零的数据
? ? ? ? ? ? ? ? ? ?array2[x][0] = i; ? ? ? ? ? ? ? //向稀疏素组写入非零数据的行
? ? ? ? ? ? ? ? ? ?array2[x][1] = j; ? ? ? ? ? ? ? //向稀疏素组写入非零数据的列
? ? ? ? ? ? ? ? ? ?array2[x][2] = array1[i][j]; ? ?//向稀疏素组写入非零数据的值
? ? ? ? ? ? ? ? ? ?x++;//向下一行写入
? ? ? ? ? ? ? }
? ? ? ? ? }
? ? ? }
? ? ? ?//输出稀疏素组
? ? ? ?System.out.println("稀疏素组:");
? ? ? ?for (int i = 0; i < array2.length; i++) {
? ? ? ? ? ?for (int j = 0; j < array2[i].length; j++) {
? ? ? ? ? ? ? ?System.out.print(array2[i][j]+"\t");
? ? ? ? ? }
? ? ? ? ? ?System.out.println();
? ? ? }
? ? ? ?System.out.println("============================================");
? ? ? ?//还原
? ? ? ?int[][] array3 = new int[array2[0][0]][array2[0][1]]; ? //创建第三个数组,行为稀疏素组的[0][0],列为稀疏素组的[0][1]
? ? ? ?for (int i = 1; i < array2.length; i++) { ? ? ? ? ? ? ? //从第二行遍历稀疏素组
? ? ? ? ? ?array3[array2[i][0]][array2[i][1]]=array2[i][2]; ? ?//向数组3写入数据
? ? ? }
? ? ? ?//输出
? ? ? ?System.out.println("还原结果:");
? ? ? ?for (int i = 0; i < array3.length; i++) {
? ? ? ? ? ?for (int j = 0; j < array3[i].length; j++) {
? ? ? ? ? ? ? ?System.out.print(array3[i][j]+"\t");
? ? ? ? ? }
? ? ? ? ? ?System.out.println();
? ? ? }
? }
结果:
原数组:
0 0 0 0 0 0 0 0 0 0 0
0 0 1 0 0 0 0 0 0 0 0
0 0 0 2 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
============================================
稀疏素组:
11 11 2
1 2 1
2 3 2
============================================
还原结果:
0 0 0 0 0 0 0 0 0 0 0
0 0 1 0 0 0 0 0 0 0 0
0 0 0 2 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
?
Process finished with exit code 0
|