线性表
双向链表
定义
双向链表(双链表),多个结点,每个节点=一个数据域+两个指针域,数据域存数据,指针域分前后,前一个指针域指向前驱结点,后一个指针域指向后继结点。头结点数据域不存储数据,头结点指向前驱结点的指针域的值为null,指向后继结点的指针域指向第一个真正存储数据的结点;尾结点指向后继结点的指针域为null。
图示
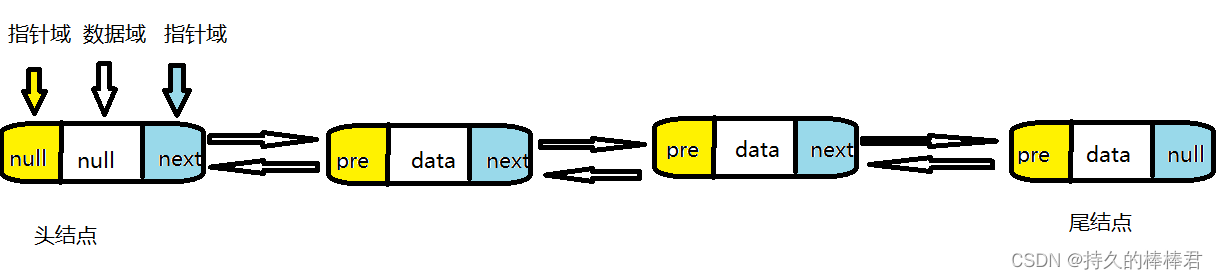
代码实现
按照面向对象的思想,设计一个类,描述结点,结点属于链表,所有把结点类作为链表类的内部类来实现
链表类
public class DoublyLinkedList<T> implements Iterable{
private Node head;
private Node last;
private int N;
private class Node{
public T item;
public Node pre;
public Node next;
public Node( Node pre,T item, Node next) {
this.pre = pre;
this.item = item;
this.next = next;
}
}
public DoublyLinkedList(){
this.head = new Node(null,null,null);
this.last = null;
this.N = 0;
}
public void clear(){
this.head.pre = null;
this.head.item = null;
this.head.next = null;
this.last = null;
this.N = 0;
}
public int length(){
return N;
}
public boolean isEmpty(){
return N == 0;
}
public T getFirst(){
if(isEmpty()){
return null;
}
return head.next.item;
}
public T getLast(){
if(isEmpty()){
return null;
}
return last.item;
}
public void add(T t){
if(isEmpty()){
Node newNode = new Node(head, t,null);
last = newNode;
head.next = last;
}else{
Node oldLast = last;
Node newNode = new Node(oldLast, t, null);
last.next = newNode;
last = newNode;
}
N++;
}
public void insert(int i, T t){
Node preNode = head;
for(int index = 0; index<i; index++){
preNode = preNode.next;
}
Node currNode = preNode.next;
Node newNode = new Node(preNode,t,currNode);
preNode.next = newNode;
currNode.pre = newNode;
N++;
}
public T get(int i){
Node n = head.next;
for(int index = 0; index<i; index++){
n = n.next;
}
return n.item;
}
public int indexOf(T t){
Node n = head;
for(int i = 0; n.next != null; i++){
n = n.next;
if(n.item.equals(t)){
return i;
}
}
return -1;
}
public T remove(int i){
Node pre = head;
for(int index = 0; index<i; index++){
pre = pre.next;
}
Node currNode = pre.next;
Node nextNode = currNode.next;
pre.next = nextNode;
nextNode.pre = pre;
N--;
return currNode.item;
}
@Override
public Iterator iterator() {
return new DIterator();
}
private class DIterator implements Iterator{
private Node n;
public DIterator(){
this.n = head;
}
@Override
public boolean hasNext() {
return n.next != null;
}
@Override
public Object next() {
n = n.next;
return n.item;
}
}
}
测试类
public class DoublyLinkedListTest {
public static void main(String[] args) {
DoublyLinkedList dll = new DoublyLinkedList();
dll.add("姚明");
dll.add("科比");
dll.add("乔丹");
System.out.println("----------------测试遍历--------------------");
for(Object obj : dll){
System.out.println(obj);
}
System.out.println("----------------测试插入--------------------");
dll.insert(1,"杜兰特");
dll.insert(2,"奥尼尔");
for(Object obj : dll){
System.out.println(obj);
}
System.out.println("---------获取第一个和最后一个结点的值------------");
System.out.println(dll.getFirst());;
System.out.println(dll.getLast());
System.out.println("---------获取指定元素第一次出现的位置-------------");
System.out.println(dll.indexOf("科比"));
System.out.println("--------------测试删除----------------------");
System.out.println(dll.remove(2));
System.out.println("--------------清空链表----------------------");
dll.clear();
for(Object obj : dll){
System.out.println(obj);
}
}
}
结果
----------------测试遍历-------------------- 姚明 科比 乔丹 ----------------测试插入-------------------- 姚明 杜兰特 奥尼尔 科比 乔丹 ---------获取第一个和最后一个结点的值------------ 姚明 乔丹 ---------获取指定元素第一次出现的位置------------- 3 --------------测试删除---------------------- 奥尼尔 --------------清空链表----------------------
|