一、队列
- 队列是一个有序列表,可以用数组或是链表来实现。
- 遵循先入先出的原则。即:先存入队列的数据,要先取出。后存入的要后取出
1.1数组模拟队列
- 队列本身是有序列表,若使用数组的结构来存储队列的数据,则队列数组的声明如下图, 其中 maxSize 是该队 列的最大容量。
- 因为队列的输出、输入是分别从前后端来处理,因此需要两个变量 front 及 rear 分别记录队列前后端的下标, front
会随着数据输出而改变,而 rear 则是随着数据输入而改变,如图所示: 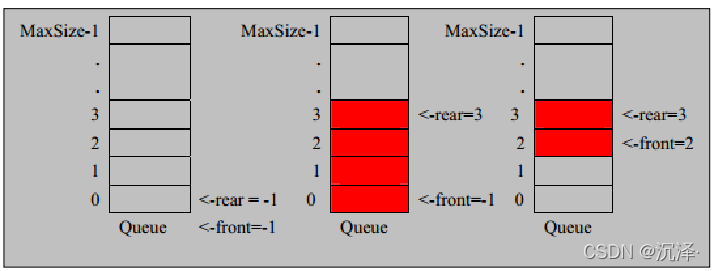 当我们将数据存入队列时称为”addQueue”,addQueue 的处理需要有两个步骤:思路分析
- 将尾指针往后移:rear+1 , 当 front == rear 时队列为空
- 若尾指针 rear 小于队列的最大下标 maxSize-1,则将数据存入 rear 所指的数组元素中;否则无法存入数据。即 rear == maxSize - 1队列满
代码实现:
class ArrayQueue {
private int maxSize;
private int front;
private int rear;
private int[] arr;
public ArrayQueue(int arrMaxSize) {
maxSize = arrMaxSize;
arr = new int[maxSize];
front = -1;
rear = -1;
}
public boolean isFull() {
return rear == maxSize - 1;
}
public boolean isEmpty() {
return rear == front;
}
public void addQueue(int n) {
if (isFull()) {
System.out.println("队列满,不能加入数据~");
return;
}
rear++;
arr[rear] = n;
}
public int getQueue() {
if (isEmpty()) {
throw new RuntimeException("队列空,不能取数据");
}
front++;
return arr[front];
}
public void showQueue() {
if (isEmpty()) {
System.out.println("队列空的,没有数据~~");
return;
}
for (int i = 0; i < arr.length; i++) {
System.out.printf("arr[%d]=%d\n", i, arr[i]);
}
}
public int headQueue() {
if (isEmpty()) {
throw new RuntimeException("队列空的,没有数据~~");
}
return arr[front + 1];
}
}
问题分析并优化
- 目前数组使用一次就不能用, 没有达到复用的效果
- 将这个数组使用算法,改进成一个环形的队列 取模:%
1.2数组模拟环形队列
对前面的数组模拟队列的优化,充分利用数组. 因此将数组看做是一个环形的。(通过取模的方式来实现即可)
分析说明:
- 尾索引的下一个为头索引时表示队列满,即将队列容量空出一个作为约定,这个在做判断队列满的 时候需要注意 (rear + 1) % maxSize == front [满]
- rear == front [空]
- 分析示意图:
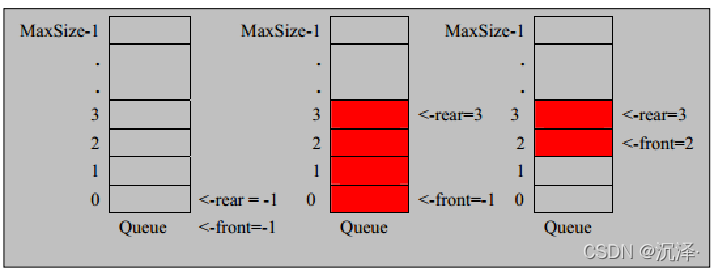
思路如下: 1.front变量的含义做一个调整: front就指向队列的第一个元素,也就是说arr[front]就是队列的第一个元素front的初始值=0 2. rear变量的含义做一个调整;rear指向队列的最后一个元素的后一个位置.因为希望空出一个空间做为约定.rear的初始值=0 3.当队列满时,条件是(rear+1) %maxsize = front【满】 4.对队列为空的条件,rear == front空 5.当我们这样分析,队列中有效的数据的个数(rear+ maxSize - front) % maxSize // rear=1 front =0 6.我们就可以在原来的队列上修改得到,一个环形队列
代码实现:
class CircleArray {
private int maxSize;
private int front;
private int rear;
private int[] arr;
public CircleArray(int arrMaxSize) {
maxSize = arrMaxSize;
arr = new int[maxSize];
}
public boolean isFull() {
return (rear + 1) % maxSize == front;
}
public boolean isEmpty() {
return rear == front;
}
public void addQueue(int n) {
if (isFull()) {
System.out.println("队列满,不能加入数据~");
return;
}
arr[rear] = n;
rear = (rear + 1) % maxSize;
}
public int getQueue() {
if (isEmpty()) {
throw new RuntimeException("队列空,不能取数据");
}
int value = arr[front];
front = (front + 1) % maxSize;
return value;
}
public void showQueue() {
if (isEmpty()) {
System.out.println("队列空的,没有数据~~");
return;
}
for (int i = front; i < front + size() ; i++) {
System.out.printf("arr[%d]=%d\n", i % maxSize, arr[i % maxSize]);
}
}
public int size() {
return (rear + maxSize - front) % maxSize;
}
public int headQueue() {
if (isEmpty()) {
throw new RuntimeException("队列空的,没有数据~~");
}
return arr[front];
}
}
二、链表
链表是有序的列表,但是它在内存中是存储如下: 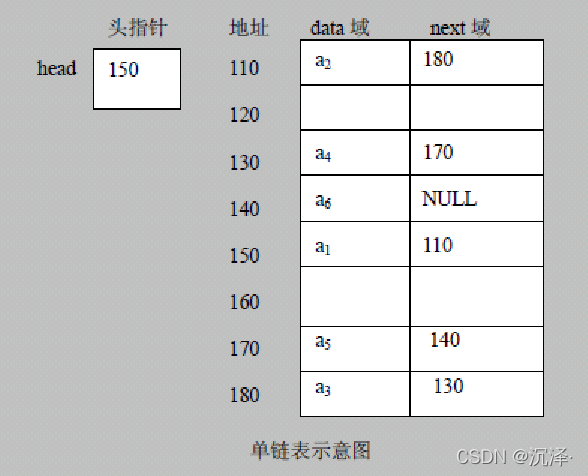 小结上图:
- 链表是以节点的方式来存储,是链式存储
- 每个节点包含 data 域, next 域:指向下一个节点.
- 如图:发现链表的各个节点不一定是连续存储.
- 链表分带头节点的链表和没有头节点的链表,根据实际的需求来确定
2.1 单链表
单链表(带头结点) 逻辑结构示意图如下 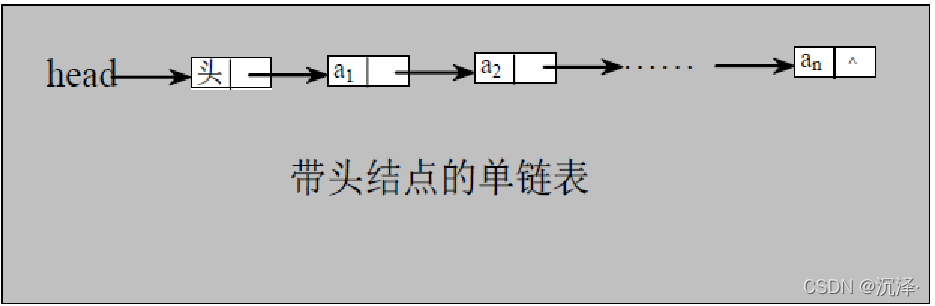 示例:使用带 head 头的单向链表实现 –水浒英雄排行榜管理完成对英雄人物的增删改查操作, 注:新增、 删除和修改,查找
1、第一种方法在添加英雄时,直接添加到链表的尾部: 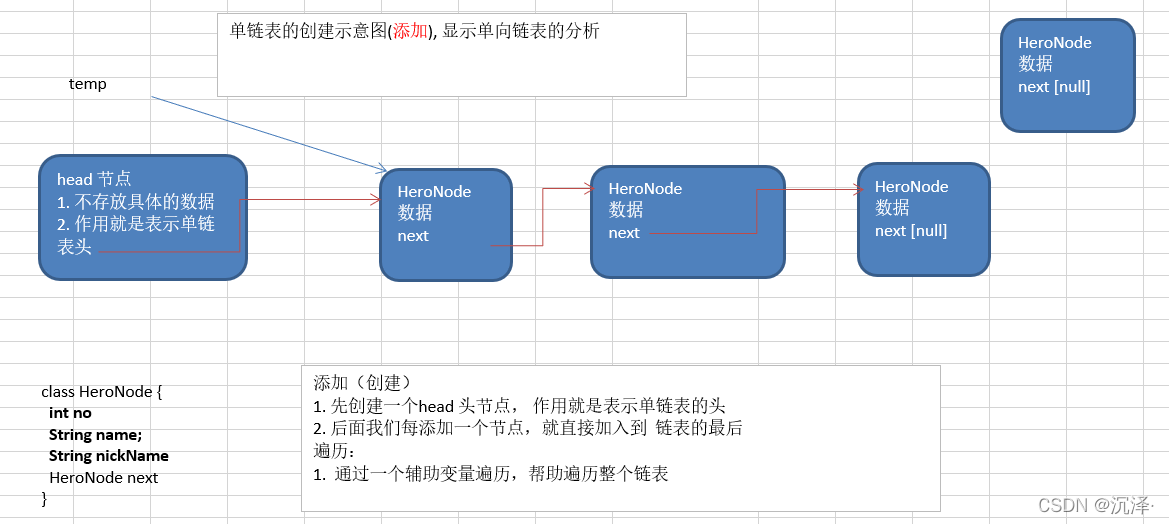 2、第二种方式在添加英雄时,根据排名将英雄插入到指定位置(如果有这个排名,则添加失败,并给出提示) 思路的分析示意图: 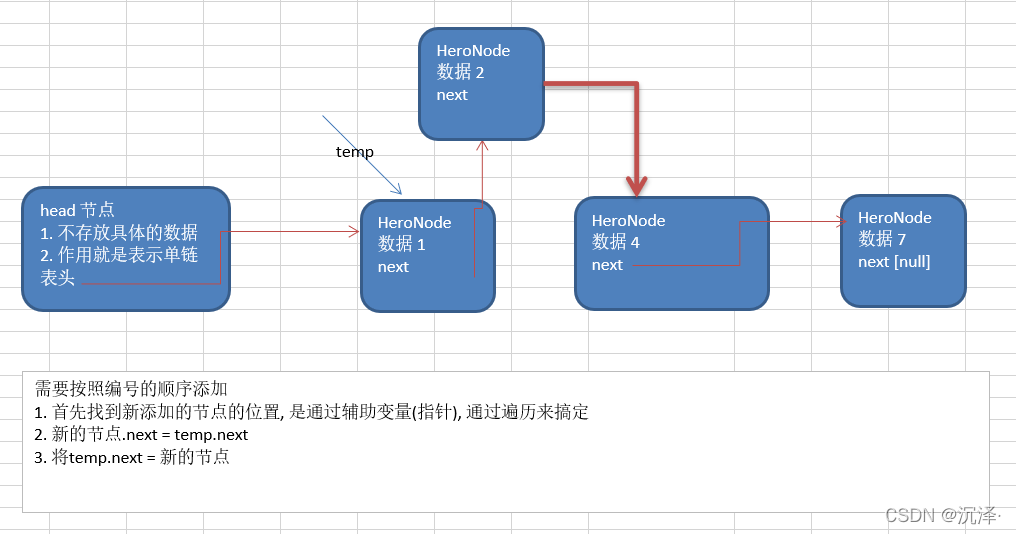 3、 修改节点功能 思路 先找到该节点,通过遍历 temp.name = newHeroNode.name ; temp.nickname= newHeroNode.nickname
4、删除节点 思路分析的示意图 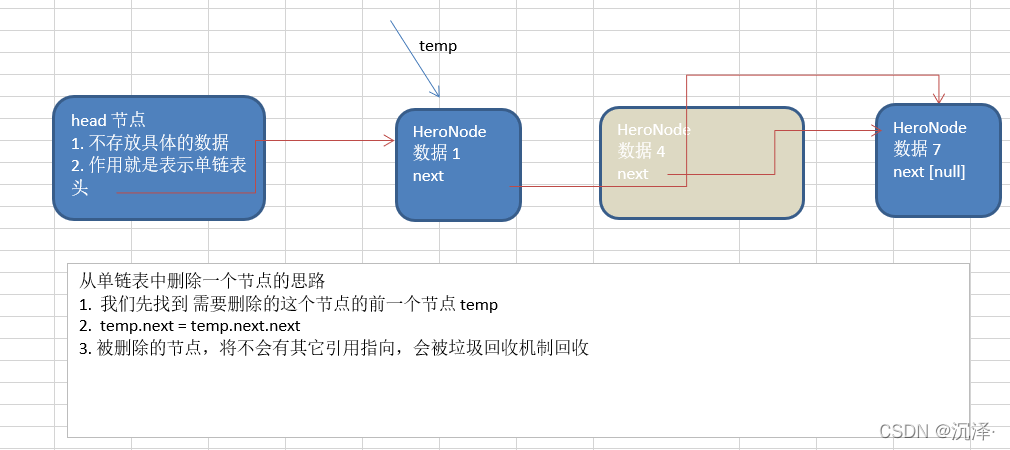
class SingleLinkedList {
private HeroNode head = new HeroNode(0, "", "");
public HeroNode getHead() {
return head;
}
public void add(HeroNode heroNode) {
HeroNode temp = head;
while(true) {
if(temp.next == null) {
break;
}
temp = temp.next;
}
temp.next = heroNode;
}
public void addByOrder(HeroNode heroNode) {
HeroNode temp = head;
boolean flag = false;
while(true) {
if(temp.next == null) {
break;
}
if(temp.next.no > heroNode.no) {
break;
} else if (temp.next.no == heroNode.no) {
flag = true;
break;
}
temp = temp.next;
}
if(flag) {
System.out.printf("准备插入的英雄的编号 %d 已经存在了, 不能加入\n", heroNode.no);
} else {
heroNode.next = temp.next;
temp.next = heroNode;
}
}
public void update(HeroNode newHeroNode) {
if(head.next == null) {
System.out.println("链表为空~");
return;
}
HeroNode temp = head.next;
boolean flag = false;
while(true) {
if (temp == null) {
break;
}
if(temp.no == newHeroNode.no) {
flag = true;
break;
}
temp = temp.next;
}
if(flag) {
temp.name = newHeroNode.name;
temp.nickname = newHeroNode.nickname;
} else {
System.out.printf("没有找到 编号 %d 的节点,不能修改\n", newHeroNode.no);
}
}
public void del(int no) {
HeroNode temp = head;
boolean flag = false;
while(true) {
if(temp.next == null) {
break;
}
if(temp.next.no == no) {
flag = true;
break;
}
temp = temp.next;
}
if(flag) {
temp.next = temp.next.next;
}else {
System.out.printf("要删除的 %d 节点不存在\n", no);
}
}
public void list() {
if(head.next == null) {
System.out.println("链表为空");
return;
}
HeroNode temp = head.next;
while(true) {
if(temp == null) {
break;
}
System.out.println(temp);
temp = temp.next;
}
}
}
class HeroNode {
public int no;
public String name;
public String nickname;
public HeroNode next;
public HeroNode(int no, String name, String nickname) {
this.no = no;
this.name = name;
this.nickname = nickname;
}
@Override
public String toString() {
return "HeroNode [no=" + no + ", name=" + name + ", nickname=" + nickname + "]";
}
}
2.1.1 求单链表中有效节点的个数
方法:获取到单链表的节点的个数(如果是带头结点的链表,需求不统计头节点)
public static int getLength(HeroNode head) {
if(head.next == null) {
return 0;
}
int length = 0;
HeroNode cur = head.next;
while(cur != null) {
length++;
cur = cur.next;
}
return length;
}
2.1.2 查找单链表中的倒数第 k 个结点
思路: //1. 编写一个方法,接收head节点,同时接收一个index //2. index 表示是倒数第index个节点 //3. 先把链表从头到尾遍历,得到链表的总的长度 getLength //4. 得到size 后,我们从链表的第一个开始遍历 (size-index)个,就可以得到 //5. 如果找到了,则返回该节点,否则返回nulll
public static HeroNode findLastIndexNode(HeroNode head, int index) {
if(head.next == null) {
return null;
}
int size = getLength(head);
if(index <=0 || index > size) {
return null;
}
HeroNode cur = head.next;
for(int i =0; i< size - index; i++) {
cur = cur.next;
}
return cur;
}
2.1.3 单链表的反转
思路分析图解 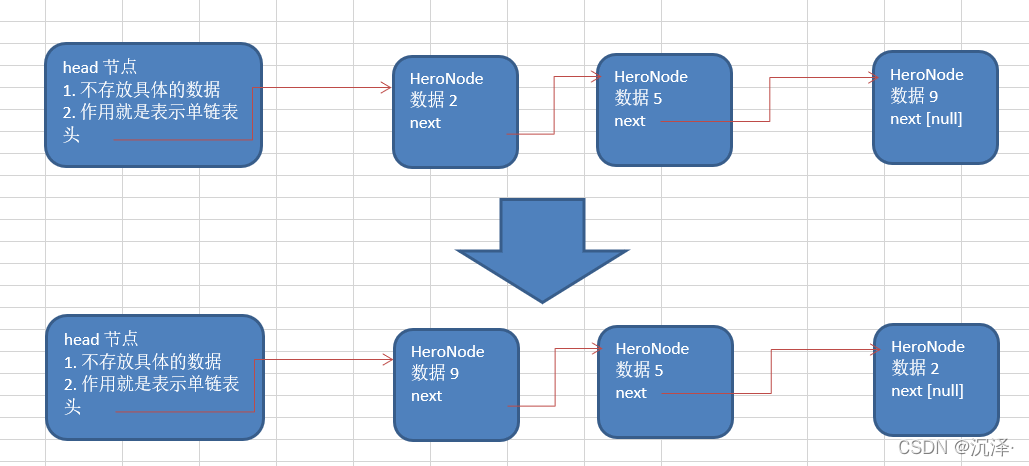
public static void reversetList(HeroNode head) {
if(head.next == null || head.next.next == null) {
return ;
}
HeroNode cur = head.next;
HeroNode next = null;
HeroNode reverseHead = new HeroNode(0, "", "");
while(cur != null) {
next = cur.next;
cur.next = reverseHead.next;
reverseHead.next = cur;
cur = next;
}
head.next = reverseHead.next;
}
2.1.4 从尾到头打印单链表
思路
- 方式1:先将单链表进行反转操作,然后再逾预今这样的做的问题是会破坏原来的单链表的结构,不建议
- 方式2:可以利用找这个数据结构,将各个节点压火到栈中,然后利用栈的先进后出的特点,就实现了逆序打印的效果.
举例演示柱的使用stack:
public class TestStack {
public static void main(String[] args) {
Stack<String> stack = new Stack();
stack.add("jack");
stack.add("tom");
stack.add("smith");
while (stack.size() > 0) {
System.out.println(stack.pop());
}
}
}
单链表的逆序打印代码:
public static void reversePrint(HeroNode head) {
if(head.next == null) {
return;
}
Stack<HeroNode> stack = new Stack<HeroNode>();
HeroNode cur = head.next;
while(cur != null) {
stack.push(cur);
cur = cur.next;
}
while (stack.size() > 0) {
System.out.println(stack.pop());
}
}
2.1.5 合并两个有序的单链表,合并之后的链表依然有序
package com.atguigu.test;
public class Test {
public static void main(String[] args) {
System.out.println("************");
Node link1 = new Node(null, "");
Node a1 = new Node(1, "a1");
Node a3 = new Node(3, "a3");
Node a5 = new Node(5, "a5");
link1.next = a1;
a1.next = a3;
a3.next = a5;
Node link2 = new Node(null, "");
Node a2 = new Node(2, "a2");
Node a4 = new Node(4, "a4");
Node a6 = new Node(6, "a6");
Node a8 = new Node(8, "a8");
link2.next = a2;
a2.next = a4;
a4.next = a6;
a6.next = a8;
Node node = mergeLink(link1, link2);
}
public static Node mergeLink(Node link1, Node link2) {
if (link1 == null || link1.next == null) {
return link2;
}
if (link2 == null || link2.next == null) {
return link1;
}
Node newLink = new Node(null, "");
Node cur1 = link1.next;
Node cur2 = link2.next;
while (cur1 != null && cur2 != null) {
if (cur1.no <= cur2.no) {
Node temp = new Node(cur1.no, cur1.name);
temp.next = newLink.next;
newLink.next = temp;
cur1 = cur1.next;
} else {
Node temp = new Node(cur2.no, cur2.name);
temp.next = newLink.next;
newLink.next = temp;
cur2 = cur2.next;
}
}
while (cur2 != null){
Node temp = new Node(cur2.no, cur2.name);
temp.next = newLink.next;
newLink.next = temp;
cur2 = cur2.next;
}
while (cur1 != null){
Node temp = new Node(cur1.no, cur1.name);
temp.next = newLink.next;
newLink.next = temp;
cur1 = cur1.next;
}
return newLink;
}
}
class Node {
Integer no;
String name;
Node next;
public Node(Integer no, String name) {
this.no = no;
this.name = name;
}
}
合并成新的有序链表,一般要创建新的节点,想通过改变原有链表节点指向实现,相对较复杂。
2.2 双向链表
管理单向链表的缺点分析:
- 单向链表,查找的方向只能是一个方向,而双向链表可以向前或者向后查找。
- 单向链表不能自我删除,需要靠辅助节点 ,而双向链表,则可以自我删除,所以前面我们单链表删除 时节点,总是找到 temp,temp 是待删除节点的前一个节点(认真体会).
使用带 head 头的双向链表实现 –水浒英雄排行榜,分析双向链表如何完成遍历,添加,修改和删除的思路 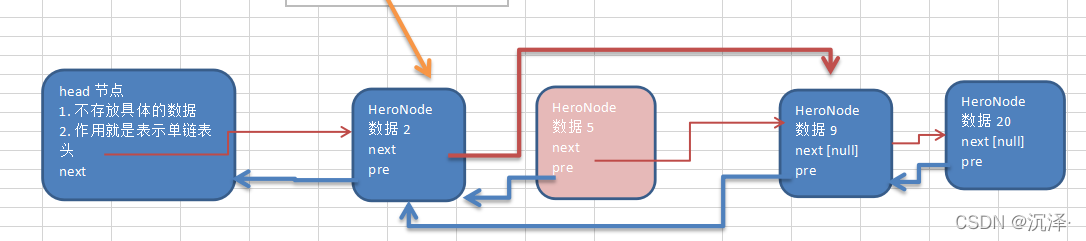 对上图的说明: 分析 双向链表的遍历,添加,修改,删除的操作思路===》代码实现 1、 遍历 方和 单链表一样,只是可以向前,也可以向后查找 2、 添加 (默认添加到双向链表的最后)
(1) 先找到双向链表的最后这个节点 (2) temp.next = newHeroNode (3) newHeroNode.pre = temp;
3、 修改 思路和 原来的单向链表一样. 4、 删除
(1) 因为是双向链表,因此,我们可以实现自我删除某个节点 (2) 直接找到要删除的这个节点,比如 temp (3) temp.pre.next = temp.next (4) temp.next.pre = temp.pre;
代码实现:
class DoubleLinkedList {
private HeroNode2 head = new HeroNode2(0, "", "");
public HeroNode2 getHead() {
return head;
}
public void list() {
if (head.next == null) {
System.out.println("链表为空");
return;
}
HeroNode2 temp = head.next;
while (true) {
if (temp == null) {
break;
}
System.out.println(temp);
temp = temp.next;
}
}
public void add(HeroNode2 heroNode) {
HeroNode2 temp = head;
while (true) {
if (temp.next == null) {
break;
}
temp = temp.next;
}
temp.next = heroNode;
heroNode.pre = temp;
}
public void addByOrder(int no,HeroNode2 heroNode) {
HeroNode2 temp = head;
boolean flag = false;
while (true) {
if(temp.next == null) {
break;
}
if(temp.next.no > heroNode.no) {
break;
} else if (temp.next.no == heroNode.no) {
flag = true;
break;
}
temp = temp.next;
}
if (flag){
System.out.printf("没有找到 编号 %d 的节点,不能修改\n", no);
}else {
heroNode.next = temp.next;
temp.next.pre = heroNode;
temp.next = heroNode;
heroNode.pre = temp;
}
}
public void update(HeroNode2 newHeroNode) {
if (head.next == null) {
System.out.println("链表为空~");
return;
}
HeroNode2 temp = head.next;
boolean flag = false;
while (true) {
if (temp == null) {
break;
}
if (temp.no == newHeroNode.no) {
flag = true;
break;
}
temp = temp.next;
}
if (flag) {
temp.name = newHeroNode.name;
temp.nickname = newHeroNode.nickname;
} else {
System.out.printf("没有找到 编号 %d 的节点,不能修改\n", newHeroNode.no);
}
}
public void del(int no) {
if (head.next == null) {
System.out.println("链表为空,无法删除");
return;
}
HeroNode2 temp = head.next;
boolean flag = false;
while (true) {
if (temp == null) {
break;
}
if (temp.no == no) {
flag = true;
break;
}
temp = temp.next;
}
if (flag) {
temp.pre.next = temp.next;
if (temp.next != null) {
temp.next.pre = temp.pre;
}
} else {
System.out.printf("要删除的 %d 节点不存在\n", no);
}
}
}
class HeroNode2 {
public int no;
public String name;
public String nickname;
public HeroNode2 next;
public HeroNode2 pre;
public HeroNode2(int no, String name, String nickname) {
this.no = no;
this.name = name;
this.nickname = nickname;
}
@Override
public String toString() {
return "HeroNode [no=" + no + ", name=" + name + ", nickname=" + nickname + "]";
}
}
2.3 单向环形链表
2.3.1 约瑟夫环
Josephu(约瑟夫、约瑟夫环) 问题 Josephu 问题为:设编号为 1,2,… n 的 n 个人围坐一圈,约定编号为 k(1<=k<=n)的人从 1 开始报数,数 到 m 的那个人出列,它的下一位又从 1 开始报数,数到 m 的那个人又出列,依次类推,直到所有人出列为止,由 此产生一个出队编号的序列。
提示:用一个不带头结点的循环链表来处理 Josephu 问题:先构成一个有 n 个结点的单循环链表,然后由 k 结 点起从 1 开始计数,计到 m 时,对应结点从链表中删除,然后再从被删除结点的下一个结点又从 1 开始计数,直 到最后一个结点从链表中删除算法结束。 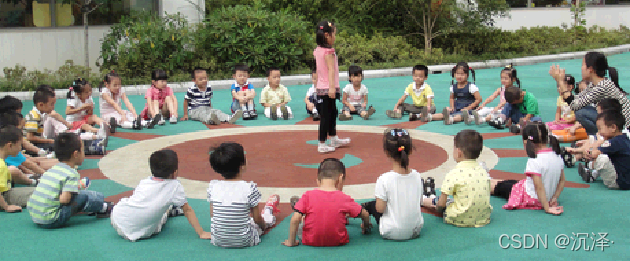
2.3.2 单向环形链表介绍
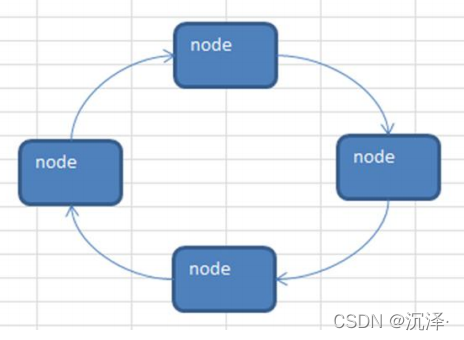
2.3.3 Josephu 问题解决
Josephu 问题为:设编号为1,2,… n的n个人围坐一圈,约定编号为k(1<=k<=n)的人从1开始报数,数到m 的那个人出列,它的下一位又从1开始报数,数到m的那个人又出列,依次类推,直到所有人出列为止,由此产生一个出队编号的序列。
假设:有五人围坐在一起,从第一个人报数,每数两下就有一人出列。 n = 5 , 即有5个人 k = 1, 从第一个人开始报数 m = 2, 数2下
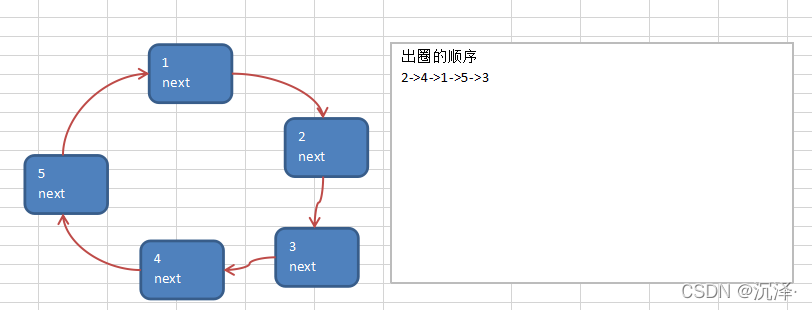 约瑟夫问题-创建环形链表的思路图解: 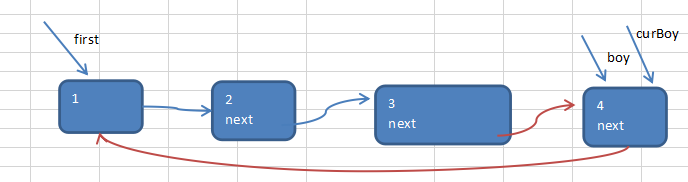
构建一个单向的环形链表思路
- 先创建第一个节点, 让 first 指向该节点,并形成环形,即first.next=first
- 后面当我们每创建一个新的节点,就把该节点,加入到已有的环形链表中即可.
遍历环形链表
- 先让一个辅助指针(变量) curBoy,指向first节点
- 然后通过一个while循环遍历 该环形链表即可 curBoy.next == first 结束
约瑟夫问题-小孩出圈的思路分析图: 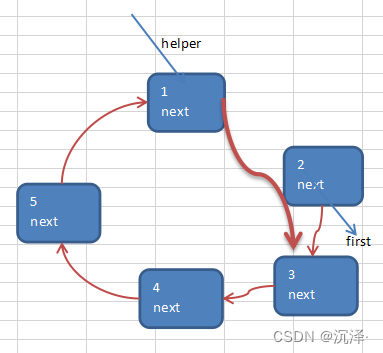
根据用户的输入,生成一个小孩出圈的顺序 n = 5 , 即有5个人 k = 1, 从第一个人开始报数 m = 2, 数2下
- 需求创建一个辅助指针(变量) helper , 事先应该指向环形链表的最后这个节点. 补充: 小孩报数前,先让 first 和 helper 移动 k - 1次
- 当小孩报数时,让first 和 helper 指针同时 的移动 m - 1 次
- 这时就可以将first 指向的小孩节点 出圈 first = first .next helper.next = first;原来first 指向的节点就没有任何引用,就会被回收
出圈的顺序 2->4->1->5->3
class CircleSingleLinkedList {
private Boy first = null;
public void addBoy(int nums) {
if (nums < 1) {
System.out.println("nums的值不正确");
return;
}
Boy curBoy = null;
for (int i = 1; i <= nums; i++) {
Boy boy = new Boy(i);
if (i == 1) {
first = boy;
first.setNext(first);
curBoy = first;
} else {
curBoy.setNext(boy);
boy.setNext(first);
curBoy = boy;
}
}
}
public void showBoy() {
if (first == null) {
System.out.println("没有任何小孩~~");
return;
}
Boy curBoy = first;
while (true) {
System.out.printf("小孩的编号 %d \n", curBoy.getNo());
if (curBoy.getNext() == first) {
break;
}
curBoy = curBoy.getNext();
}
}
public void countBoy(int startNo, int countNum, int nums) {
if (first == null || startNo < 1 || startNo > nums) {
System.out.println("参数输入有误, 请重新输入");
return;
}
Boy helper = first;
while (true) {
if (helper.getNext() == first) {
break;
}
helper = helper.getNext();
}
for(int j = 0; j < startNo - 1; j++) {
first = first.getNext();
helper = helper.getNext();
}
while(true) {
if(helper == first) {
break;
}
for(int j = 0; j < countNum - 1; j++) {
first = first.getNext();
helper = helper.getNext();
}
System.out.printf("小孩%d出圈\n", first.getNo());
first = first.getNext();
helper.setNext(first);
}
System.out.printf("最后留在圈中的小孩编号%d \n", first.getNo());
}
}
class Boy {
private int no;
private Boy next;
public Boy(int no) {
this.no = no;
}
public int getNo() {
return no;
}
public void setNo(int no) {
this.no = no;
}
public Boy getNext() {
return next;
}
public void setNext(Boy next) {
this.next = next;
}
}
|