符号表
1、概述
符号表和map集合很相像,由键值对组合而成,符号表中键也具有唯一性,通常也是根据键来找到键对应的值。 如图 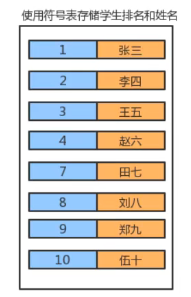
2、分类
1、底层是数组实现的符号表 2、底层是链表的符号表,又分为无序和有序
这里主要讲底层是链表实现的符号表。。。
无序符号表
每次表中元素的添加都是直接从表的头部插入的,无论插入值的大小是啥,都直接插入,可能会导致表中元素无顺序,这就是无序符号表 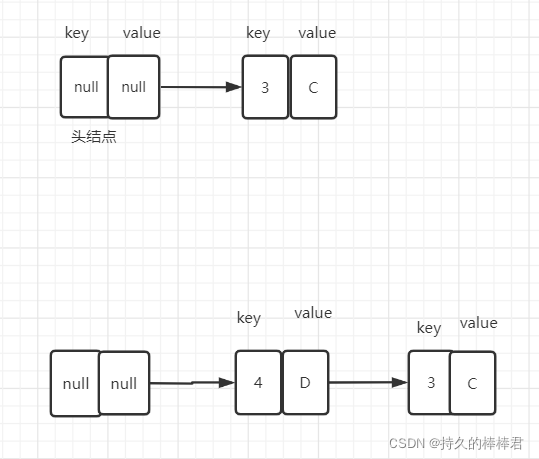
public class SymbolTable<Key,Value> {
private Node head;
private int Num;
private class Node{
public Key key;
public Value value;
public Node next;
public Node(Key key, Value value, Node next) {
this.key = key;
this.value = value;
this.next = next;
}
}
public SymbolTable(){
this.head = new Node(null,null,null);
this.Num = 0;
}
public int size(){
return Num;
}
public void put(Key key, Value value){
Node n = head;
while(n.next != null){
n = n.next;
if(n.key.equals(key)){
n.value = value;
return ;
}
}
Node newNode = new Node(key, value, null);
Node oldFirst = head.next;
newNode.next = oldFirst;
head.next = newNode;
Num++;
}
public void delete(Key key){
Node n = head;
while(n.next != null){
if(n.next.key.equals(key)){
n.next = n.next.next;
Num--;
return ;
}
n = n.next;
}
}
public Value get(Key key){
Node n = head;
while(n.next != null){
n = n.next;
if(n.key.equals(key)){
return n.value;
}
}
return null;
}
}
,那么如果要使表有顺序,该如何做到呢,其实对put()方法进行一些改变就行,这就有了有序符号表,如下
有序符号表
其他方法后无序符号表的一样,我们要改变的就是put()方法,使得添加元素后表有序 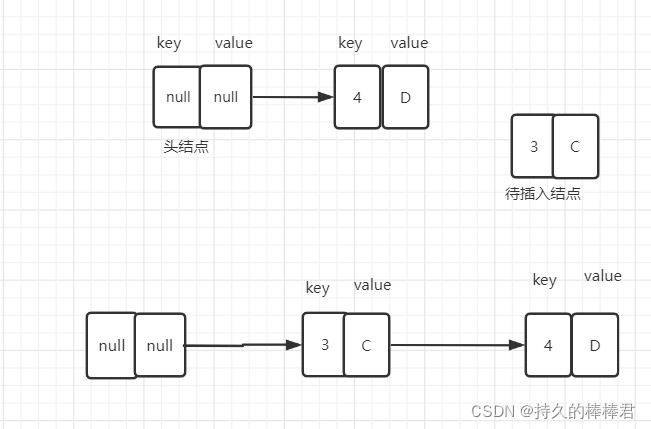
思路,让泛型Key继承Comparable接口
代码如下
public class SymbolTable<Key extends Comparable<Key>,Value> {
private Node head;
private int Num;
private class Node{
public Key key;
public Value value;
public Node next;
public Node(Key key, Value value, Node next) {
this.key = key;
this.value = value;
this.next = next;
}
}
public SymbolTable(){
this.head = new Node(null,null,null);
this.Num = 0;
}
public int size(){
return Num;
}
public void put(Key key, Value value){
Node curr = head.next;
Node pre = head;
while(curr != null && key.compareTo(curr.key)>0) {
pre = curr;
curr = curr.next;
}
if(curr != null && key.compareTo(curr.key)==0){
curr.value = value;
return ;
}
Node newNode = new Node(key, value, curr);
pre.next = newNode;
Num++;
}
public void delete(Key key){
Node n = head;
while(n.next != null){
if(n.next.key.equals(key)){
n.next = n.next.next;
Num--;
return ;
}
n = n.next;
}
}
public Value get(Key key){
Node n = head;
while(n.next != null){
n = n.next;
if(n.key.equals(key)){
return n.value;
}
}
return null;
}
}
测试代码
public class SymbolTableTest {
public static void main(String[] args) {
SymbolTable<Integer, String> st = new SymbolTable<>();
st.put(3,"C");
st.put(5,"F");
st.put(2,"B");
st.put(4,"D");
System.out.println(st.size());
}
}
通过debug的方式可以看到每次插入后都会将键值对按key的顺序排列好 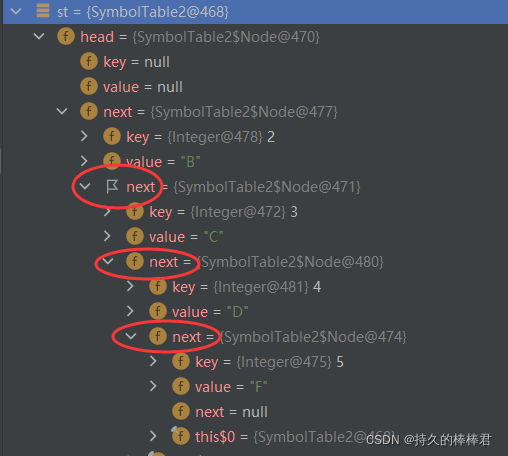
|