水
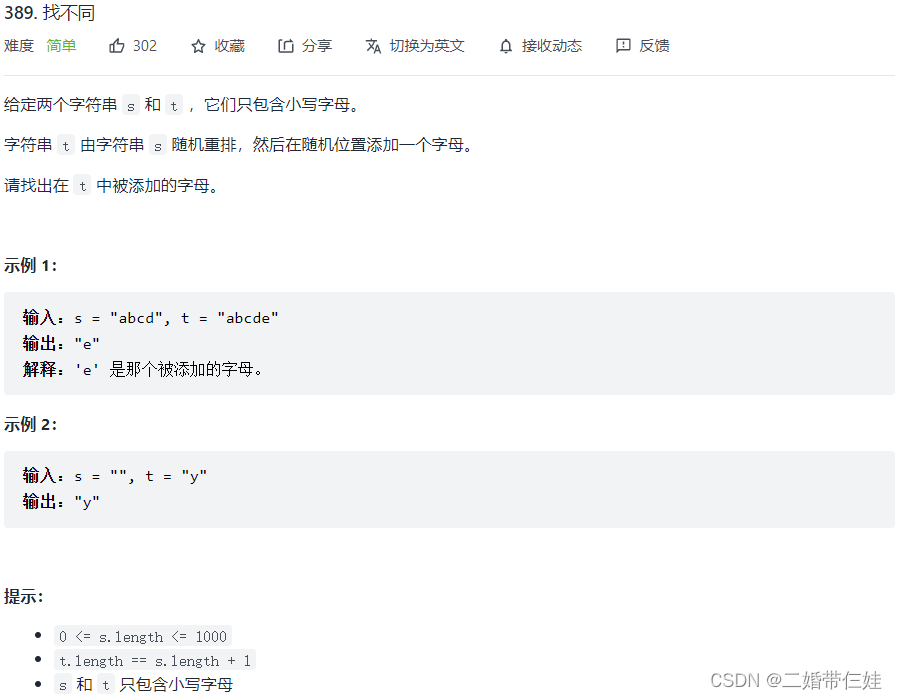
class Solution {
public:
char findTheDifference(string s, string t) {
unordered_map<char,int> cnt;
for(auto c:s) cnt[c]++;
for(auto c:t) cnt[c]--;
for(auto [a,b]:cnt){
if(b){
return a;
}
}
return 100;
}
};
dfs
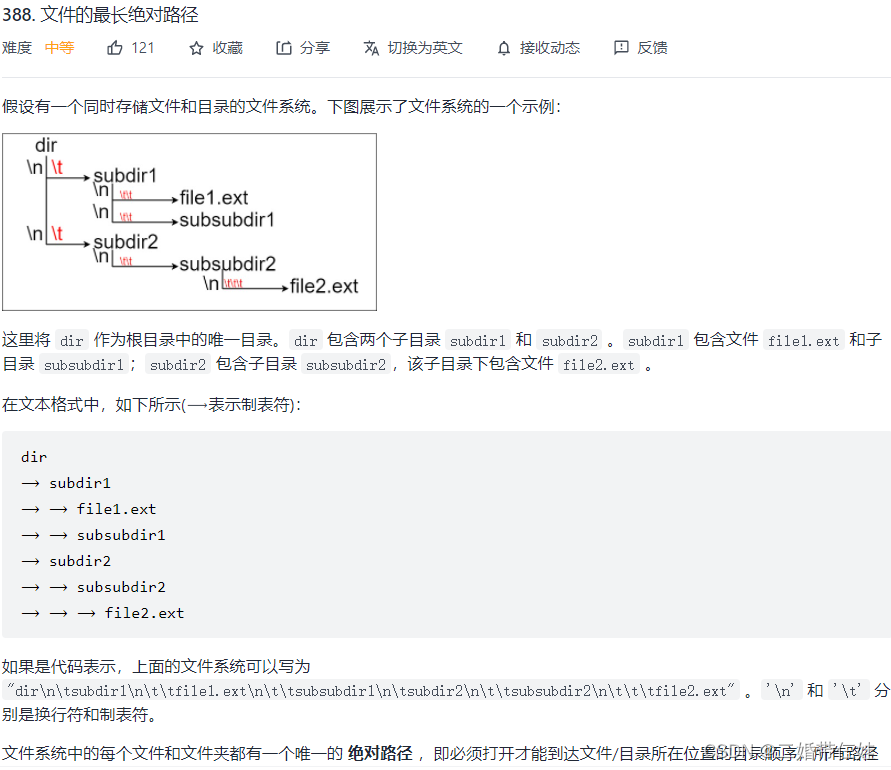 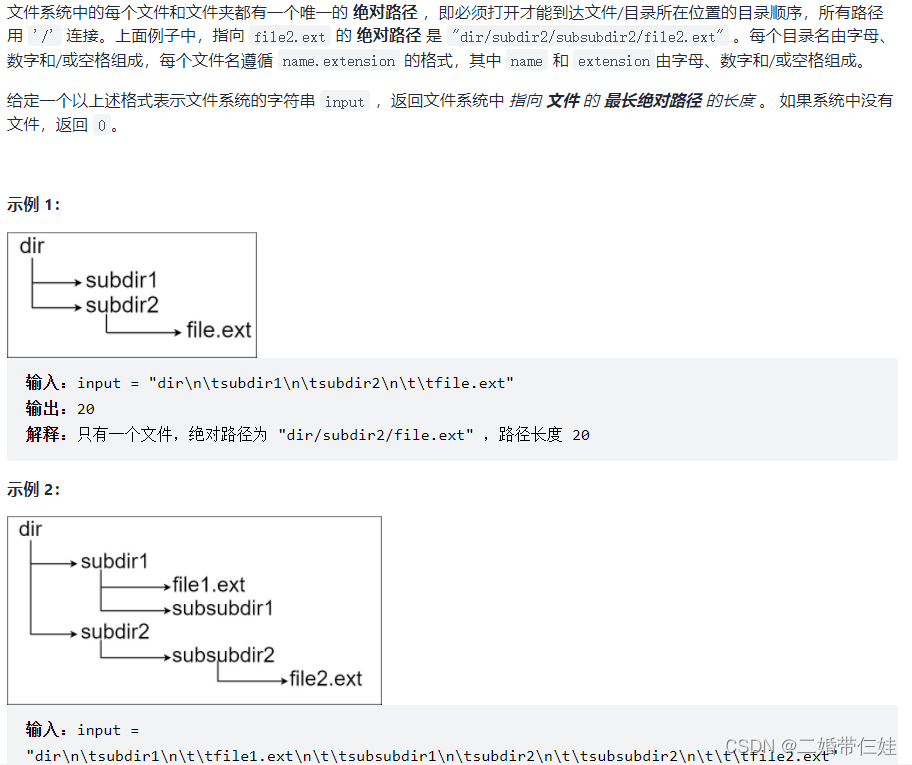 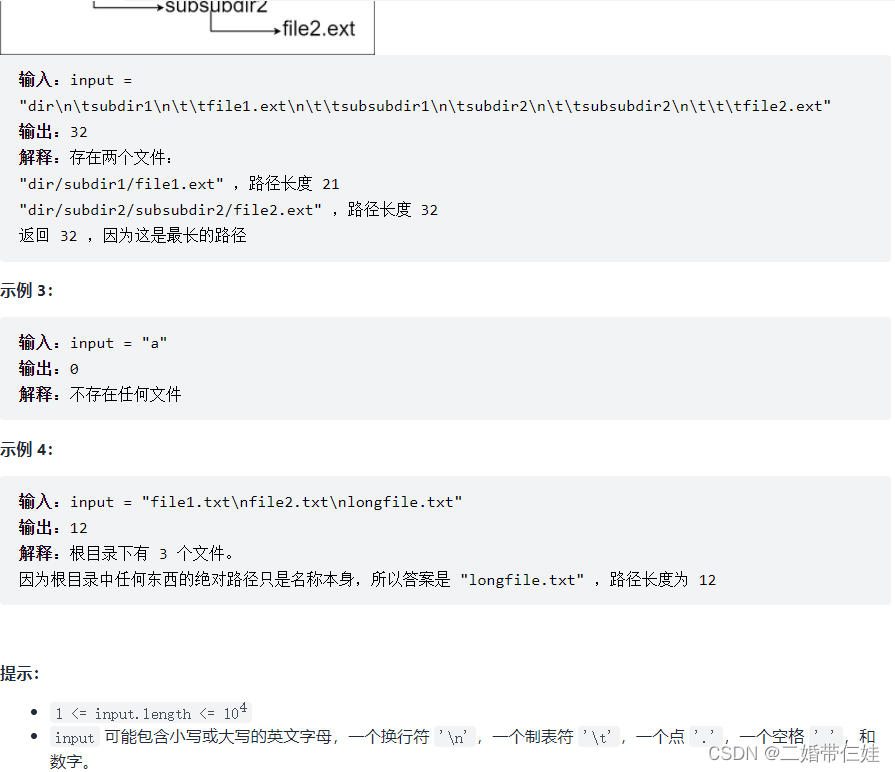
class Solution {
public:
int lengthLongestPath(string input) {
stack<int> stk;
int res=0;
for(int i=0,sum=0;i<input.size();i++){
int k=0;
while(i<input.size()&&input[i]=='\t') i++,k++;
while(stk.size()>k) sum-=stk.top(),stk.pop();
int j=i;
while(j<input.size()&&input[j]!='\n') j++;
int len=j-i;
stk.push(len),sum+=len;
if(input.substr(i,len).find('.')!=-1){
int res1=sum+stk.size()-1;
res=max(res,res1);
}
i=j;
}
return res;
}
};
水
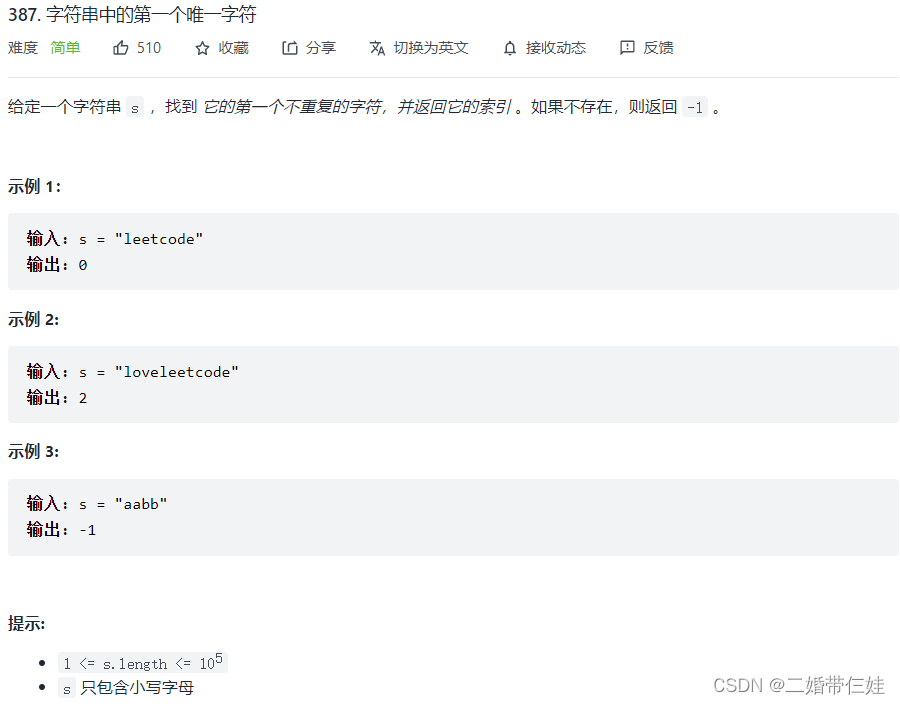
class Solution {
public:
int firstUniqChar(string s) {
unordered_map<char,int> hash;
for(auto c:s) hash[c]++;
for(int i=0;i<s.size();i++){
if(hash[s[i]]==1){
return i;
}
}
return -1;
}
};
dfs
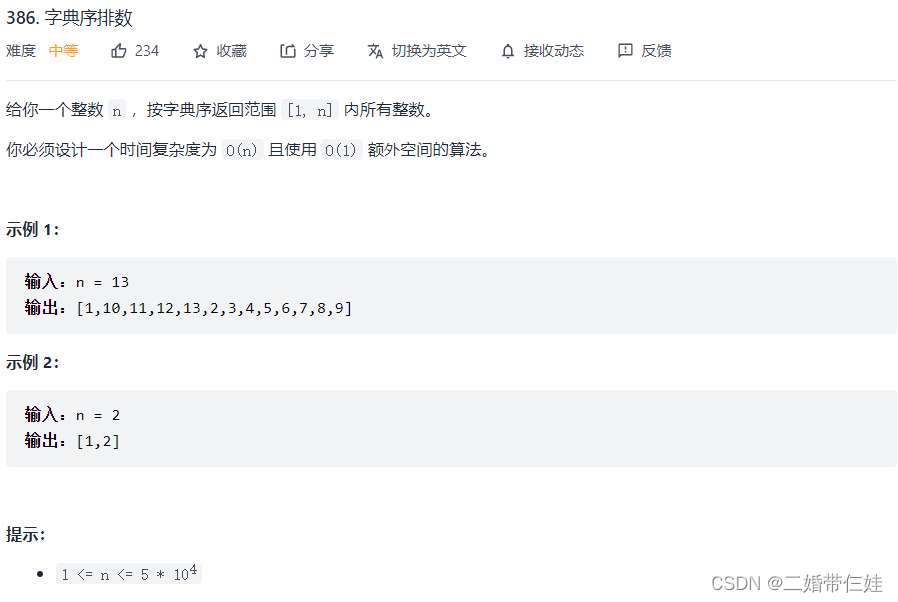
class Solution {
public:
vector<int> res;
vector<int> lexicalOrder(int n) {
for(int i=1;i<=9;i++){
dfs(i,n);
}
return res;
}
void dfs(int x,int y){
if(x<=y) res.push_back(x);
else return;
for(int i=0;i<=9;i++){
dfs(x*10+i,y);
}
}
};
dfs
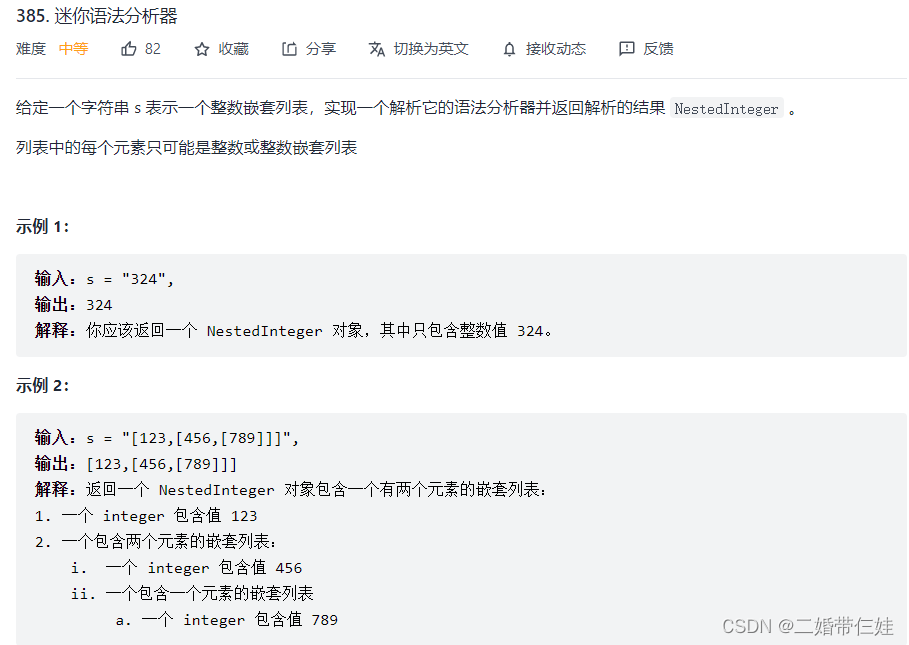 
class Solution {
public:
NestedInteger deserialize(string s) {
int u=0;
return dfs(s,u);
}
NestedInteger dfs(string& s,int& u){
NestedInteger res;
if(s[u]=='['){
u++;
while(s[u]!=']') res.add(dfs(s,u));
u++;
if(u<s.size()&&s[u]==',') u++;
}else{
int k=u;
while(k<s.size()&&s[k]!=','&&s[k]!=']') k++;
res.setInteger(stoi(s.substr(u,k-u)));
if(k<s.size()&&s[k]==',') k++;
u=k;
}
return res;
}
};
洗牌算法
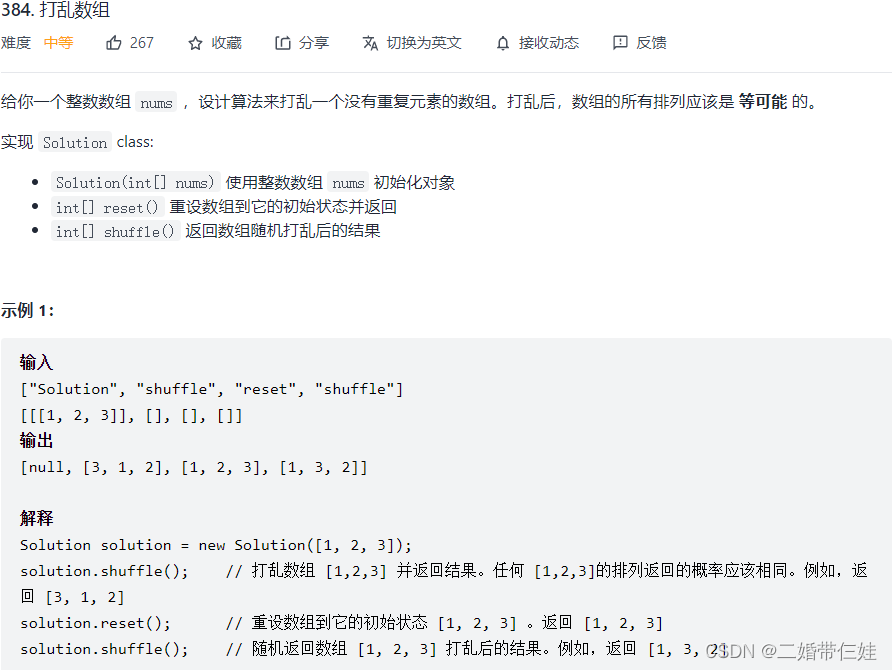 
class Solution {
public:
vector<int> a;
Solution(vector<int>& nums) {
a=nums;
}
vector<int> reset() {
return a;
}
vector<int> shuffle() {
auto b=a;
int n=a.size();
for(int i=0;i<n;i++){
swap(b[i],b[i+rand()%(n-i)]);
}
return b;
}
};
水
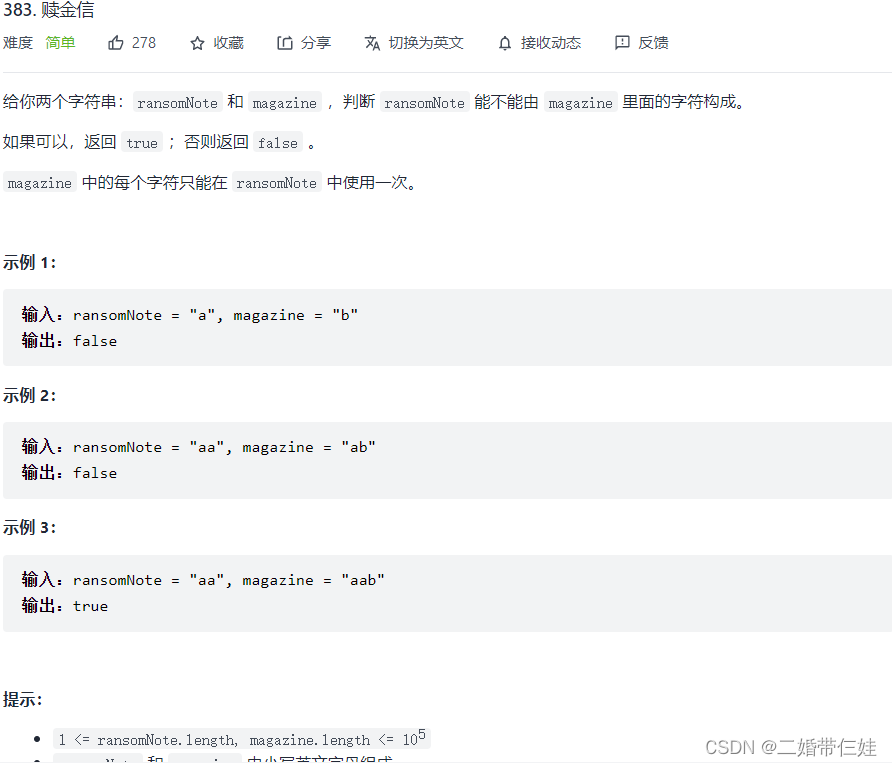
class Solution {
public:
bool canConstruct(string ransomNote, string magazine) {
unordered_map<char,int> cnt;
for(auto c:magazine) cnt[c]++;
for(auto c:ransomNote){
if(!cnt[c]) return false;
cnt[c]--;
}
return true;
}
};
上台表演算法
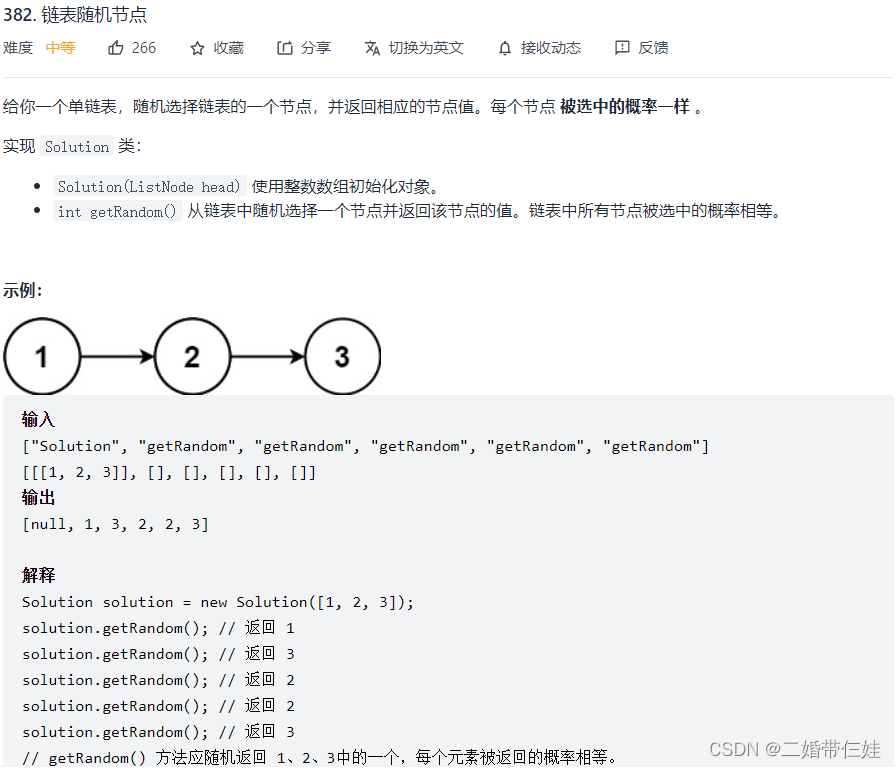 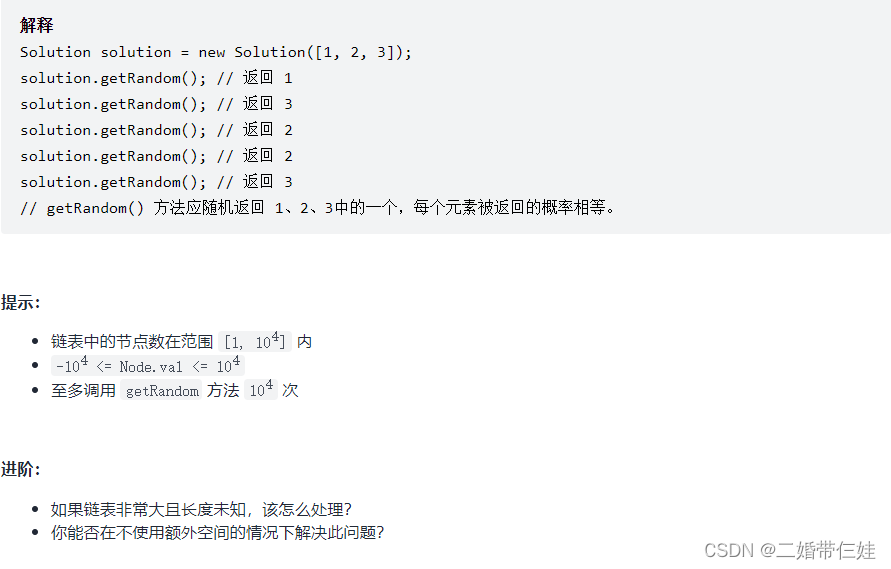
class Solution {
public:
ListNode* h;
Solution(ListNode* head) {
h=head;
}
int getRandom() {
int c=-1,n=0;
for(auto i=h;i;i=i->next){
n++;
if(rand()%n==0) c=i->val;
}
return c;
}
};
设计
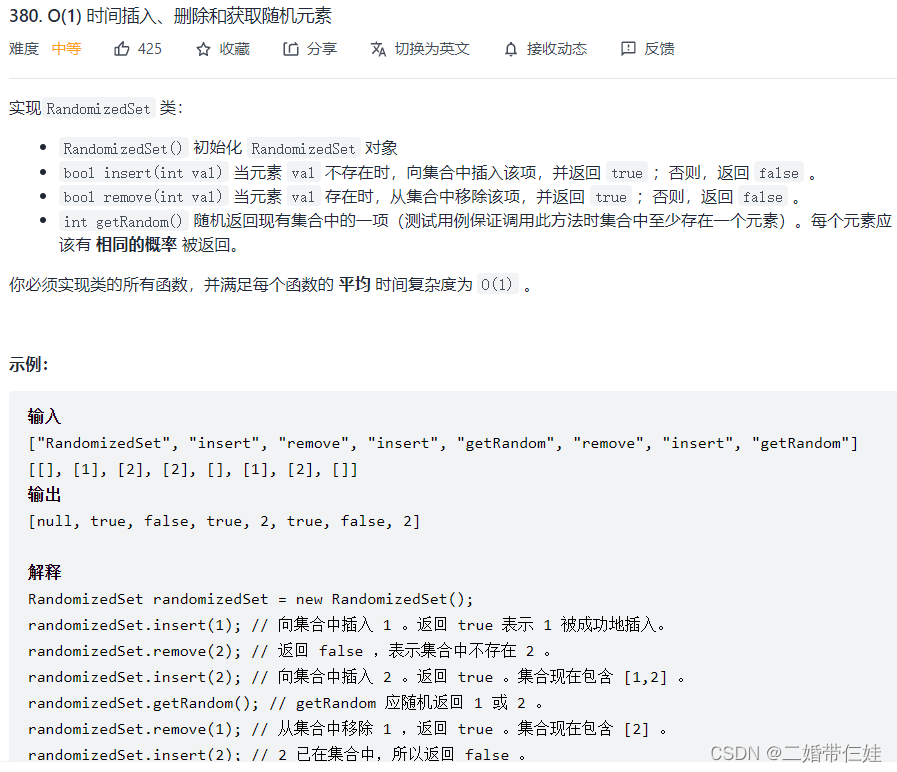 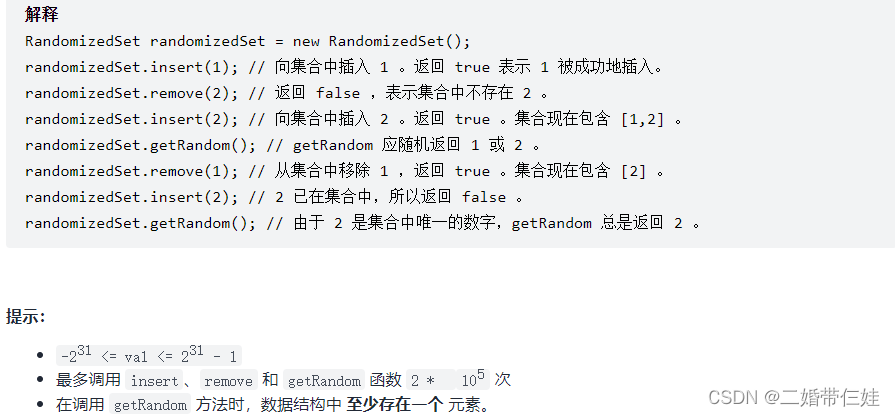
class RandomizedSet {
public:
unordered_map<int,int> hash;
vector<int> nums;
RandomizedSet() {
}
bool insert(int val) {
if(hash.count(val)==0){
nums.push_back(val);
hash[val]=nums.size()-1;
return true;
}
return false;
}
bool remove(int val) {
if(hash.count(val)){
int y=nums.back();
int px=hash[val],py=hash[y];
swap(nums[px],nums[py]);
nums.pop_back();
swap(hash[val],hash[y]);
hash.erase(val);
return true;
}
return false;
}
int getRandom() {
return nums[rand()%nums.size()];
}
};
水
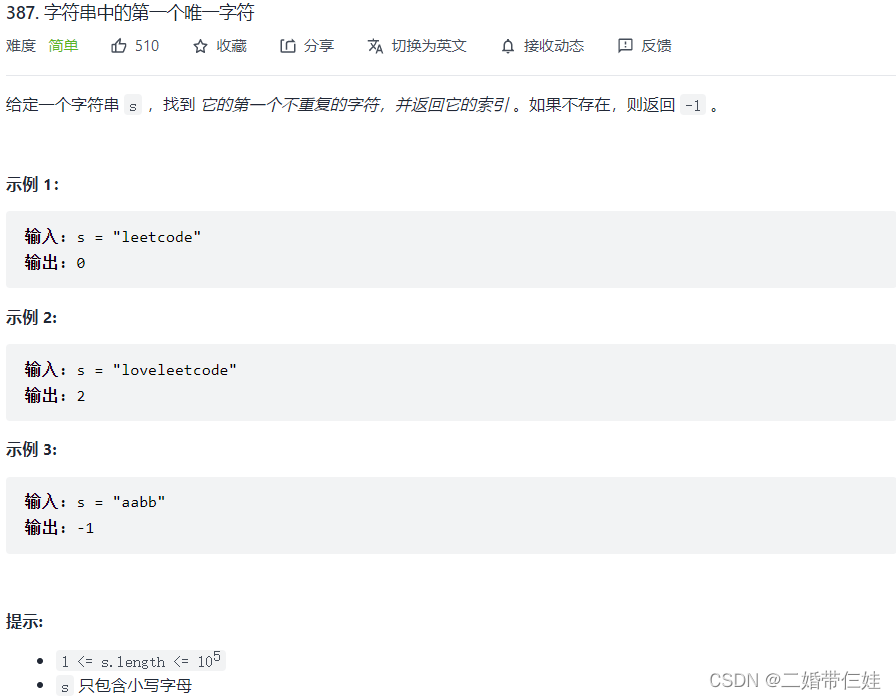
class Solution {
public:
int firstUniqChar(string s) {
unordered_map<char,int> hash;
for(auto c:s) hash[c]++;
for(int i=0;i<s.size();i++){
if(hash[s[i]]==1){
return i;
}
}
return -1;
}
};
|