一、单向链表
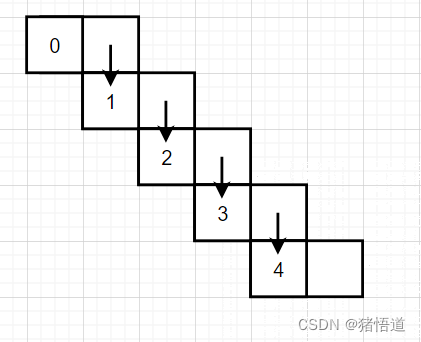
1.仅定义值域与指针域,构造方法
public class Test {
public static void main(String[] args) {
Node head = new Node(1);
Node temp = new Node(null);
for (int i = 0 ; i < 5 ; i++){
Node node = new Node(i);
while (true){
if (head.next == null){
head.next = node;
temp = node;
break;
}else{
if (temp.next == null){
temp.next = node;
break;
}else {
temp = temp.next;
}
}
}
}
System.out.println(0);
}
static class Node<T>{
private T val;
private Node next;
public Node(T val){
this(val,null);
}
public Node(T val,Node next){
this.val = val;
this.next = next;
}
}
}
2.增加一个插入方法
public class Test {
public static void main(String[] args) {
Node head = new Node(1);
for (int i = 0 ; i < 5 ; i++){
Node node = new Node(i);
head.addNext(node);
}
System.out.println(0);
}
static class Node<T>{
private T val;
private Node next;
public Node(T val){
this(val,null);
}
public Node(T val,Node next){
this.val = val;
this.next = next;
}
public void addNext(Node node){
if (null==this.next){
this.next=node;
}else{
this.next.addNext(node);
}
}
}
}
3.删除节点
1.根据任意一个待删除节点前的已知节点开始进行删除操作
Node delete = null;
Node start = null;
while (true){
if (null==start.next){
break;
}
if (delete==start.next){
start.next=delete.next;
}else {
start = start.next;
}
}
2.在链表定义时固定一个头、一个尾,增加节点时插入头尾之间,并将尾巴指向头,则任一节点开始的遍历呈现为环形,即可找到自己上一节点
二、双向链表
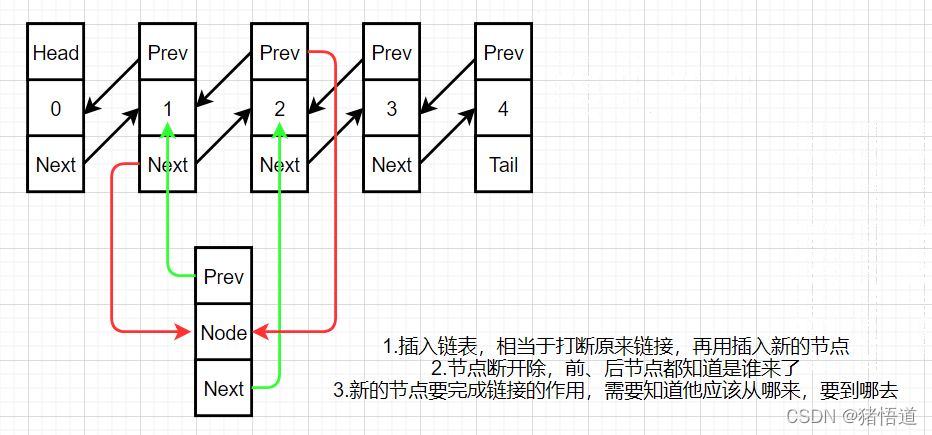
1.定义值域与指针域,构造方法,插入、删除方法
双向链表使用时注意节点增删时,上下节点衔接时交换顺序即可
public class Test {
public static void main(String[] args) {
DoubleLinkedList doubleLinkedListHead = new DoubleLinkedList<>(0);
DoubleLinkedList doubleLinkedListTail = new DoubleLinkedList<>(0);
for (int i=0;i<5;i++){
doubleLinkedListHead.addFromHead(new Node(i));
doubleLinkedListTail.addFromTail(new Node(i));
}
System.out.println();
}
static class DoubleLinkedList<T>{
Node head;
Node tail;
public DoubleLinkedList(T val){
this.head = new Node(val);
this.tail = new Node(val);
head.next = tail;
tail.prev = head;
}
public void addFromHead(Node node){
node.next = head.next;
node.prev = head;
head.next.prev = node;
head.next = node;
}
public void addFromTail(Node node){
node.prev = tail.prev;
node.next = tail;
tail.prev.next = node;
tail.prev = node;
}
public void addAfterOneNode(Node current,Node node){
node.next = current.next;
node.prev = current;
current.next.prev = node;
current.next = node;
}
public void addBeforeOneNode(Node current,Node node){
node.prev = current.prev;
node.next = current;
current.prev.next = node;
current.prev = node;
}
public void delete(Node node){
node.next.prev = node.prev;
node.prev.next = node.next;
}
public void deleteLast(){
if (head.next.equals(tail)){
return;
}
delete(tail.prev);
}
}
static class Node<T>{
private T val;
private Node prev;
private Node next;
public Node(T val){
this(val,null,null);
}
public Node(T val,Node prev,Node next){
this.val = val;
this.prev = prev;
this.next = next;
}
}
}
|