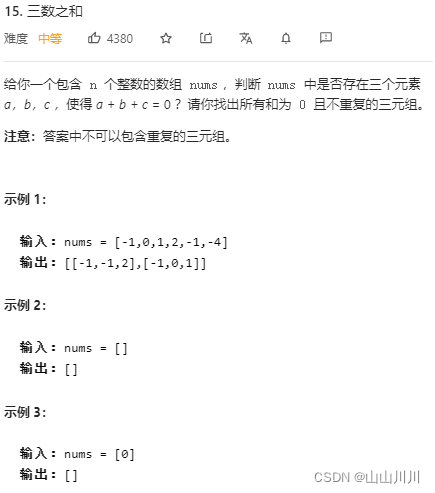
public List<List<Integer>> threeSum(int[] nums) {
List<List<Integer>> LLI = new ArrayList<>();
int right = 0;
int left = 0;
Arrays.sort(nums);
for(int i = 0; i< nums.length; i++){
right = i + 1;
left = nums.length -1;
while(i > 0 && nums[i-1] == nums[i])
i++;
while(right < left){
if (nums[i] > 0) {
return LLI;
}
if( nums[i] + nums[right] + nums[left] == 0 ){
List<Integer> LI = new ArrayList<Integer>();
Collections.addAll(LI,nums[i],nums[right],nums[left]);
LLI.add(LI);
while(right < left && nums[right] == nums[right+1])
right++;
while(right < left && nums[left] == nums[left-1])
left--;
left--;
right++;
}else if (nums[i] + nums[right] + nums[left] < 0 ){
right++;
}else {
left--;
}
}
}
return LLI;
}
看起来像三指针,最难的就是去重的问题,每改一点都是不停测试测出来的,一开始没有思考去重问题,注意代码中标志的注释部分,需要循环来跳过数组中相同的值以防止添加相同的元组进入List.
思路流程如下(来自代码随想录),思路不难但是代码写起来可能很难一次bugfree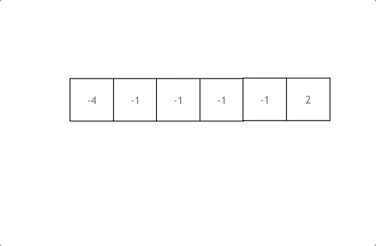 再多理解一下吧
就这样
|