package main
import (
"fmt"
"sort"
)
func main() {
s := []int{2, 4, 1, 3}
sort.Ints(s)
fmt.Printf("s: %v\n", s)
f := []float64{1.1, 4.4, 5.5, 3.3, 2.2}
sort.Float64s(f)
fmt.Printf("f: %v\n", f)
str := []string{"Go", "Bravo", "Gopher", "Alpha", "Grin", "Delta"}
sort.Strings(str)
fmt.Println(str)
hz := sort.StringSlice{
"我",
"叫",
"王",
"帆",
"啊",
}
fmt.Printf("hz: %v\n", hz)
sort.Strings(hz)
fmt.Printf("hz: %v\n", hz)
for _, v := range hz {
fmt.Println(v, []byte(v))
}
}
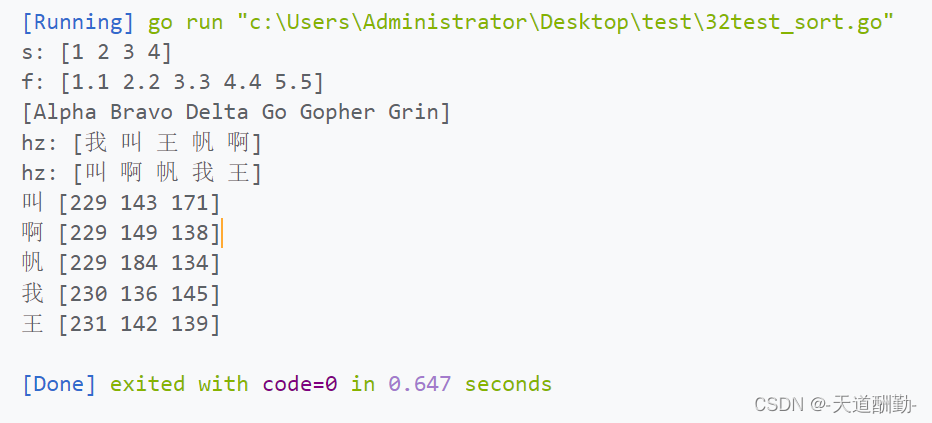
less规则自己写!!!
map根据键排序 []map[string]float64
package main
import (
"fmt"
"sort"
)
type testSlice []map[string]float64
func (l testSlice) Len() int {
return len(l)
}
func (l testSlice) Swap(i, j int) {
l[i], l[j] = l[j], l[i]
}
func (l testSlice) Less(i, j int) bool {
return l[i]["a"] < l[j]["a"]
}
func main() {
ls := testSlice{
{"a": 4, "b": 12},
{"a": 3, "b": 11},
{"a": 5, "b": 10},
}
fmt.Printf("ls: %v\n", ls)
sort.Sort(ls)
fmt.Printf("ls: %v\n", ls)
}
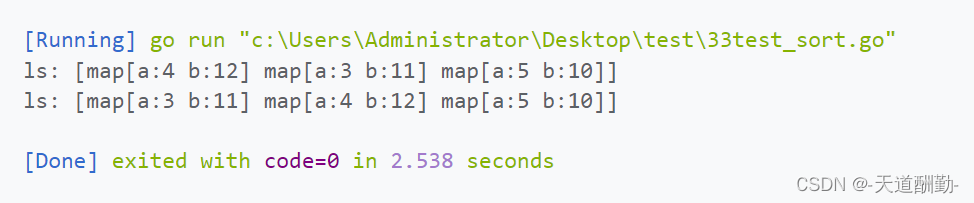
复杂结构[][]int
package main
import (
"fmt"
"sort"
)
type testSlice [][]int
func (l testSlice) Len() int {
return len(l)
}
func (l testSlice) Swap(i, j int) {
l[i], l[j] = l[j], l[i]
}
func (l testSlice) Less(i, j int) bool {
return l[i][1] < l[j][1]
}
func main() {
ls := testSlice{
{1, 4},
{9, 3},
{7, 5},
}
fmt.Printf("ls: %v\n", ls)
sort.Sort(ls)
fmt.Printf("ls: %v\n", ls)
}
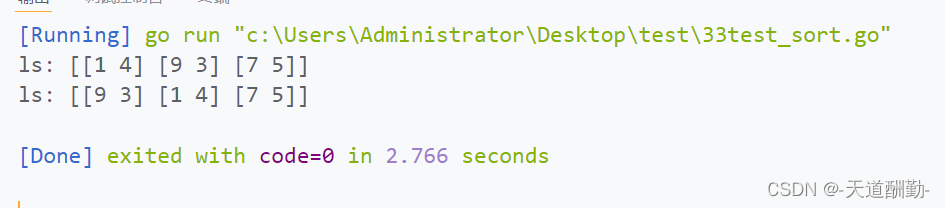
复杂结构体[]struct
package main
import (
"fmt"
"sort"
)
type People struct {
Name string
Age int
}
type testSlice []People
func (l testSlice) Len() int {
return len(l)
}
func (l testSlice) Swap(i, j int) {
l[i], l[j] = l[j], l[i]
}
func (l testSlice) Less(i, j int) bool {
return l[i].Age < l[j].Age
}
func main() {
ls := testSlice{
{Name: "n1", Age: 12},
{Name: "n2", Age: 11},
{Name: "n3", Age: 10},
}
fmt.Printf("ls: %v\n", ls)
sort.Sort(ls)
fmt.Printf("ls: %v\n", ls)
}

|