视频链接:https://www.bilibili.com/video/BV1Rx411876f?p=1
视频范围P584 - P605
String字符串
1.String存储原理
- String表示字符串类型,属于引用数据类型,不属于基本数据类型
- 在java中随便使用双引号括起来的都是String对象,例如:“adc”,“def” 这是3个String对象
- 在java中规定,双引号括起来的字符串,是不可变的,也就是说“abc”自出生到最终死亡,不可变
- 在JDK当中双引号括起来的字符串,例如:“adc”,“def”都是直接存储在方法区的字符串常量池中的
(字符串在实际开发中使用太频繁,为了执行,所以把字符串接存储在方法区的字符串常量池中)
代码示例一:
package String;
public class StringTest01 {
public static void main(String[] args) {
String s1 = "abcdef";
String s2 = "abcdef" + "xy";
}
}
内存分析图:
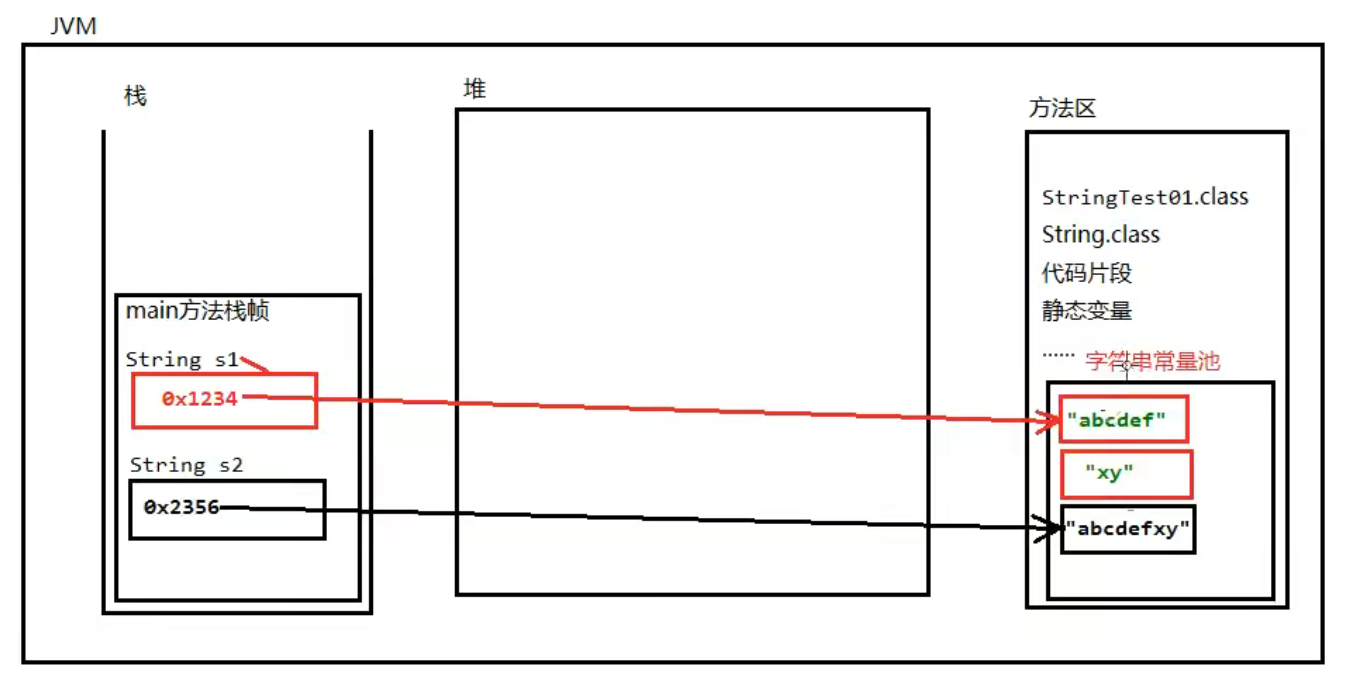 代码示例二:
package String;
public class StringTest01 {
public static void main(String[] args) {
String s3 = new String("xy");
}
}
内存分析图:
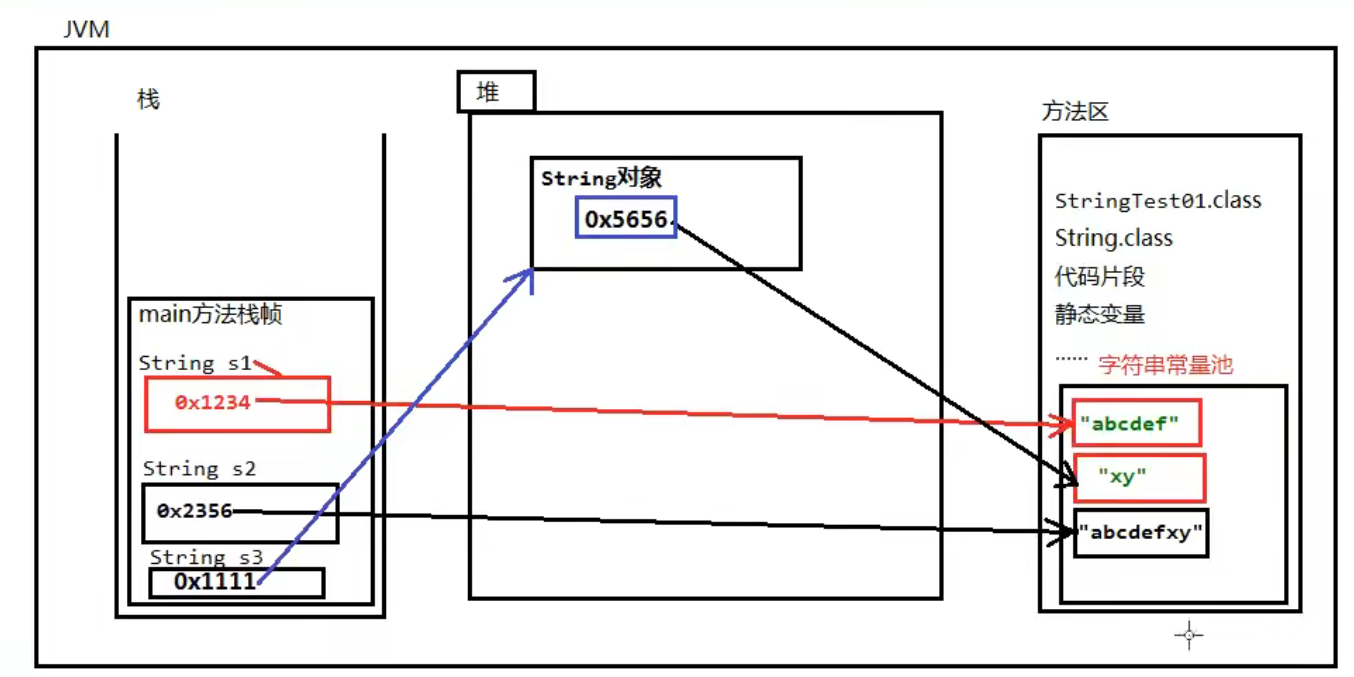
代码示例三:
用户类:
package String;
public class User {
private int id;
private String name;
public User() {
}
public User(int id, String name) {
this.id = id;
this.name = name;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
测试类:
package String;
public class Test {
public static void main(String[] args) {
User user = new User(110,"张三");
}
}
内存分析图: 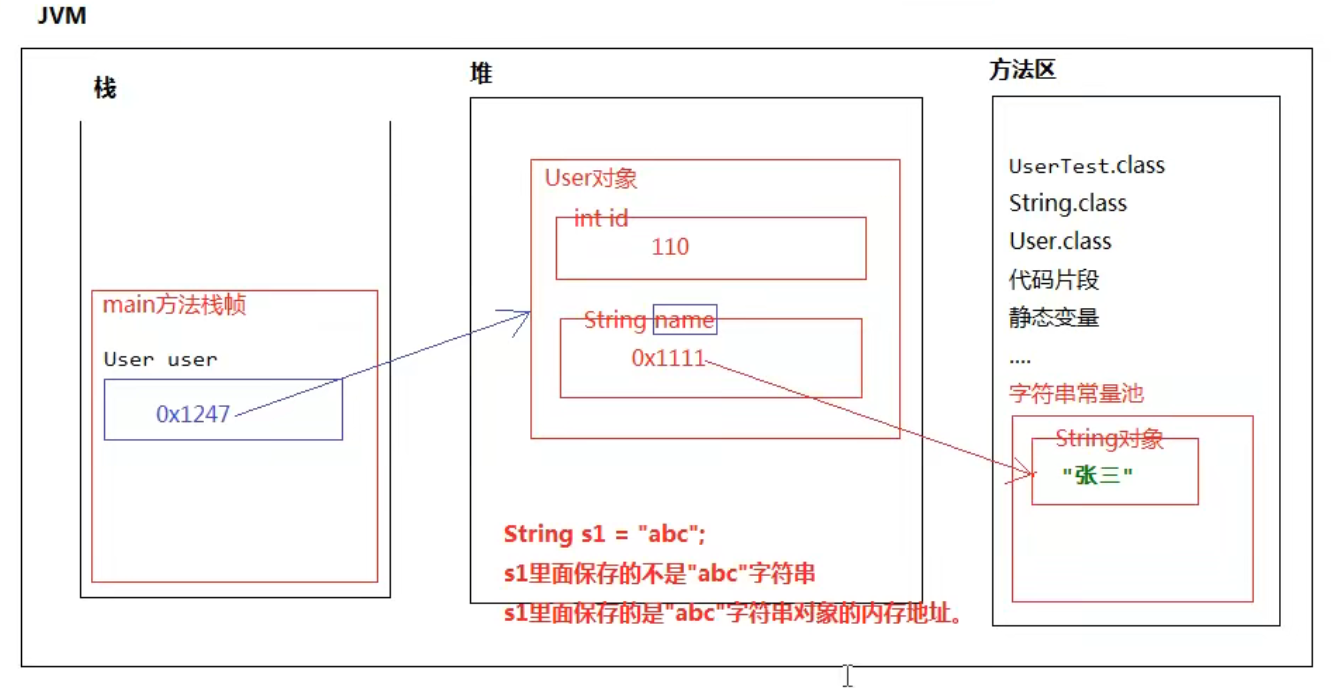 代码示例四:
package String;
public class StringTest02 {
public static void main(String[] args) {
String s1 = "hello";
String s2 = "hello";
System.out.println(s1 == s2);
}
}
内存分析图: 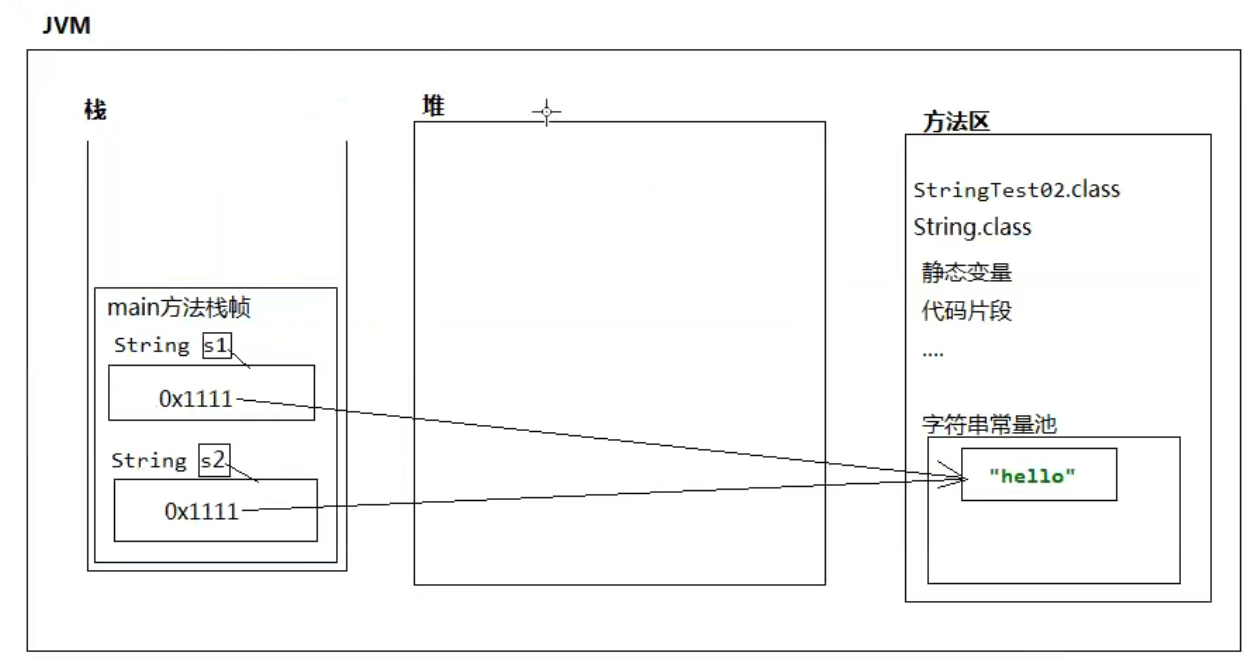 代码示例五:
package String;
public class StringTest02 {
public static void main(String[] args) {
String x = new String("xyz");
String y = new String("xyz");
System.out.println(x == y);
System.out.println(x.equals(y));
}
}
内存分析图: 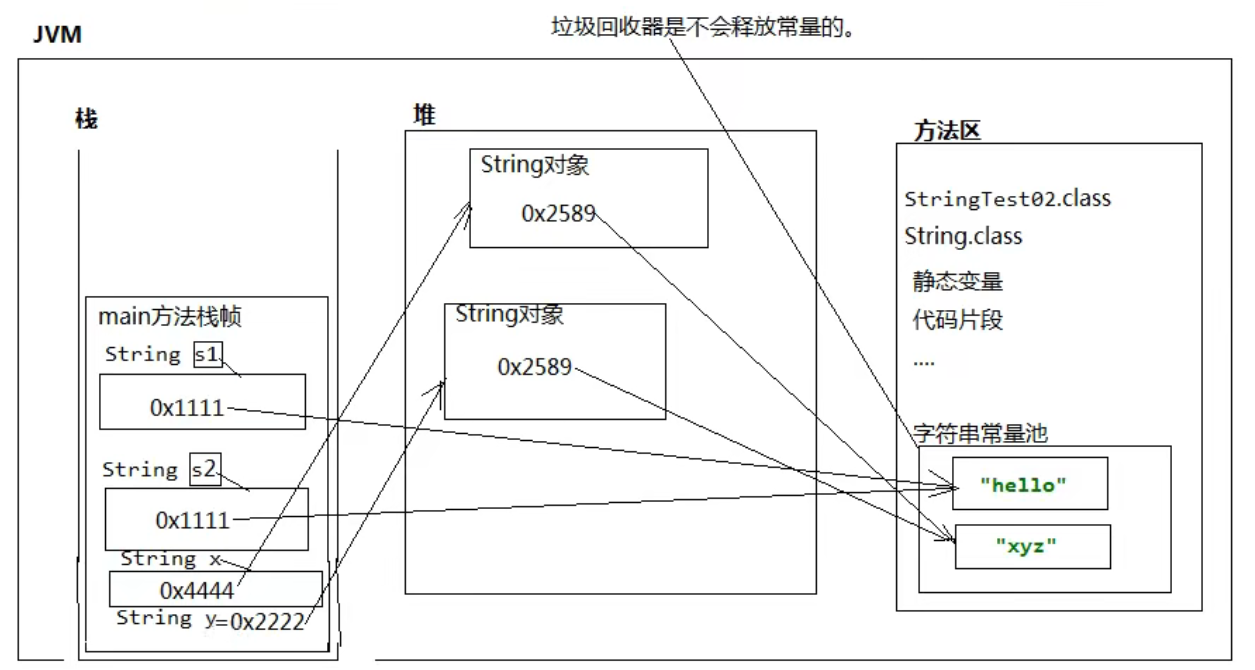 代码示例六:
package String;
public class StringTest02 {
public static void main(String[] args) {
String k = new String("test");
System.out.println("test".equals(k));
}
}
代码示例七:
package String;
public class StringTest02 {
public static void main(String[] args) {
int i = 100;
String s = "abc";
}
}
2.String面试题
2.1 题目一
分析下面程序,一共创建了几个对象:
package String;
public class StringTest02 {
public static void main(String[] args) {
String s1 = new String("hello");
String s2 = new String("hello");
}
}
2.2 题目二
题目:String为什么是不可变的? 答:我看过源代码,String类中有一个byte[]数组,这个byte[]数组采用了final修饰,因为数组一旦创建长度不可变。并且被final修饰的引用一旦指向某个对象之后,不可再指向其它对象,所以String是不可变的! 如:“abc”无法变成“abcd”
2.3 题目三
题目:StringBuilder/StringBuffer为什么是可变的呢? 答:我看过源代码,StringBuffer/StringBuilder内部实际上是一个byte[]数组,这个byte[]数组没有被final修饰,StringBuffer/StringBuilder的初始化容量我记得应该是16,当存满之后会进行扩容,底层调用了数组拷贝的方法System. arraycopy( ). …是这样扩容的。所以StringBuffer/StringBuilder适合于使用字符串的频繁拼接操作。
3.String构造方法
3.1 " "
package String;
public class StringTest03 {
public static void main(String[] args) {
String s1 = "hello world!";
System.out.println(s1);
}
}
3.2 String(" ")
package String;
public class StringTest03 {
public static void main(String[] args) {
String s = new String("我爱你!");
System.out.println(s);
}
}
3.3 String(byte[ ] bytes)
package String;
public class StringTest03 {
public static void main(String[] args) {
String s1 = "hello world!";
System.out.println(s1);
byte[] bytes = {97,98,99};
String s2 = new String(bytes);
System.out.println(s2);
System.out.println(s2.toString());
}
}
3.4 String(byte[ ] bytes, int offset, int length)
String(字节数组,数组元素下标的起始位置,长度) 将byte数组中的一部分转换成字符串
package String;
public class StringTest03 {
public static void main(String[] args) {
byte[] bytes = {97,98,99};
String s3 = new String(bytes,1,2);
System.out.println(s3);
}
}
3.5 String(char[ ] value)
将char数组全部转换成字符串
package String;
public class StringTest03 {
public static void main(String[] args) {
char[] chars = {'我','爱','你'};
String s4 = new String(chars);
System.out.println(s4);
}
}
3.6 String(char[ ] value, int offset, int count)
将char数组一部分转换成字符串
package String;
public class StringTest03 {
public static void main(String[] args) {
char[] chars = {'我','爱','你'};
String s5 = new String(chars,1,2);
System.out.println(s5);
}
}
4.String中的方法
4.1 charAt方法
package String;
public class StringTest03 {
public static void main(String[] args) {
char c = "中国人".charAt(1);
System.out.println(c);
}
}
4.2 compareTo方法
- 拿着字符串第一个字母和后面字符串的第一个字母比较,能分胜负不再比较了
- 字符串之间比较大小不能直接使用><,需要使用compareTo方法
package String;
public class StringTest03 {
public static void main(String[] args) {
int result = "abc".compareTo("abc");
System.out.println(result);
int result1 = "abcd".compareTo("abce");
System.out.println(result1);
int result2 = "abce".compareTo("abcd");
System.out.println(result2);
}
}
4.3 contains方法
判断前面的字符串中是否包含后面的子字符串
package String;
public class StringTest03 {
public static void main(String[] args) {
System.out.println("HelloWorld.java".contains(".java"));
System.out.println("HelloWorld.java".contains(".javae"));
}
}
4.4 endsWith方法
判断当前字符串是否以某个字符串结尾
package String;
public class StringTest03 {
public static void main(String[] args) {
System.out.println("HelloWorld.java".endsWith(".java"));
System.out.println("HelloWorld.java".endsWith(".javae"));
}
}
4.5 compareTo方法和equals方法
- 比较两个字符串必须使用equals方法,不能使用“==”
- JDK13中equals方法没有调用compareTo()方法
- equals方法只能看出相等不相等
- compareTo方法可以看出是否相等,并且同时还可以看出谁大谁小
package String;
public class StringTest03 {
public static void main(String[] args) {
System.out.println("abc".equals("abc"));
}
}
4.6 equalsIgnoreCase方法
判断两个字符串是否相等, 并且同时忽略大小写
package String;
public class StringTest03 {
public static void main(String[] args) {
System.out.println("ABC".equalsIgnoreCase("abc"));
}
}
4.7 getBytes方法
将字符串对象转换成字节数组
package String;
public class StringTest03 {
public static void main(String[] args) {
byte[] bytes = "abcdef".getBytes();
for (int i = 0; i < bytes.length; i++) {
System.out.println(bytes[i]);
}
}
}
运行结果:
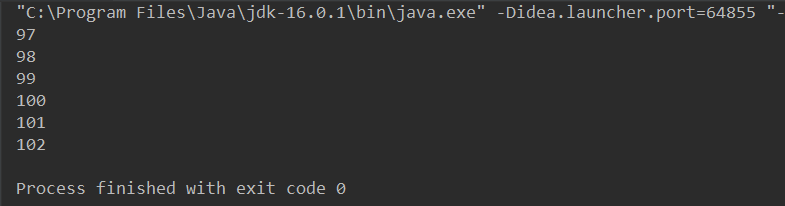
4.8 indexOf方法
判断某个子字符串在当前字符串中第一次出现处的索引
package String;
public class StringTest03 {
public static void main(String[] args) {
System.out.println("helloworld".indexOf("ow"));
}
}
4.9 isEmpty方法
判断某个字符串是否为空字符串
package String;
public class StringTest03 {
public static void main(String[] args) {
String s1 = "";
String s2 = "a";
System.out.println(s1.isEmpty());
System.out.println(s2.isEmpty());
}
}
面试题:判断数组长度和判断字符串长度不一样
- 判断数组长度是length属性
- 判断字符串长度是length()方法
package String;
public class StringTest03 {
public static void main(String[] args) {
System.out.println("abc".length());
System.out.println(new int[] {1,2,3,4}.length);
}
}
4.10 lastIndexOf方法
判断某个子字符串在当前字符串中最后一次出现的索引(下标)
package String;
public class StringTest03 {
public static void main(String[] args) {
System.out.println("abcdefbcfkbc".lastIndexOf("bc"));
}
}
4.11 replace方法
String replace(CharSequence target, CharSequence replacement)
- target The sequence of char values to be replaced
- replacement The replacement sequence of char values
String的父接口就是:CharSequence
package String;
public class StringTest03 {
public static void main(String[] args) {
System.out.println("abcdefbcfkbc".replace("bc","SS"));
}
}
4.12 split方法
拆分字符串
package String;
public class StringTest03 {
public static void main(String[] args) {
String[] ymd = "1980-10-11".split("-");
for (int i = 0; i < ymd.length; i++) {
System.out.println(ymd[i]);
}
}
}
运行结果:

4.13 startsWith方法
判断某个字符串是否以某个子字符串开始
package String;
public class StringTest03 {
public static void main(String[] args) {
System.out.println("abcdefbcfkbc".startsWith("bc"));
System.out.println("abcdefbcfkbc".startsWith("ab"));
}
}
4.14 substring方法
String substring(int beginIndex) 截取字符串,参数为起始下标
package String;
public class StringTest03 {
public static void main(String[] args) {
System.out.println("abcdefbcfkbc".substring(3));
}
}
String substring(int beginIndex, int endIndex) 截取字符串,参数为起始坐标和结束坐标:[起始坐标,结束坐标)
package String;
public class StringTest03 {
public static void main(String[] args) {
System.out.println("abcdefbcfkbc".substring(3,6));
}
}
4.15 toCharArray方法
将字符串转换为char数组
package String;
public class StringTest03 {
public static void main(String[] args) {
char[] chars = "我是中国人".toCharArray();
for (int i = 0; i < chars.length; i++) {
System.out.println(chars[i]);
}
}
}
运行结果: 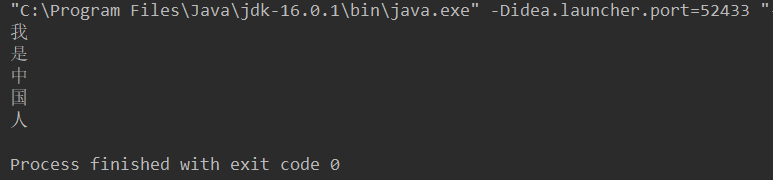
4.16 toLowerCase方法
转换为小写
package String;
public class StringTest03 {
public static void main(String[] args) {
System.out.println("SFDSAds".toLowerCase());
}
}
4.17 toUpperCase方法
转换为大写
package String;
public class StringTest03 {
public static void main(String[] args) {
System.out.println("SFDSAds".toUpperCase());
}
}
4.18 trim方法
去除字符串前后空白
package String;
public class StringTest03 {
public static void main(String[] args) {
System.out.println(" SF DS Ads ".trim());
}
}
4.19 valueOf方法
- valueOf:String中只有一个方法是静态的,不需要new对象
- 作用:将非字符串转换为字符串
package String;
public class StringTest03 {
public static void main(String[] args) {
String s1 = String.valueOf(true);
String s2 = String.valueOf(100);
System.out.println(s1);
System.out.println(s2);
}
}
参数是一个对象的时候,会自动调用该对象的toString()方法
package String;
public class StringTest03 {
public static void main(String[] args) {
String s1 = String.valueOf(new Customer());
System.out.println(s1);
}
}
class Customer{
}
重写toString()方法
package String;
public class StringTest03 {
public static void main(String[] args) {
String s1 = String.valueOf(new Customer());
System.out.println(s1);
}
}
class Customer{
public String toString(){
return "我爱你!!!";
}
}
研究println()方法的源码 为什么打印输出一个引用的时候会调用toString()方法 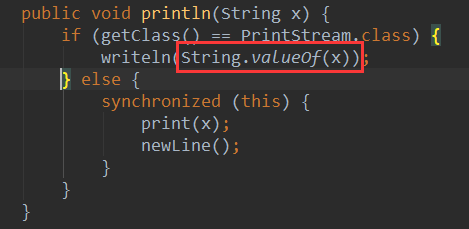 
5.String字符串的拼接
疑问:在实际开发中,如果需要进行字符串的频繁拼接,会出现什么问题? 答:因为java中的字符串是不可变的,每一次拼接都会产生新的字符串,这样会占用大量的方法区内存,造成内存空间的浪费
代码举例:
package StringBuffer;
public class StringBufferTest01 {
public static void main(String[] args) {
String s = "";
for (int i = 0; i < 100; i++) {
s = s + i;
System.out.println(s);
}
}
}
运行结果:
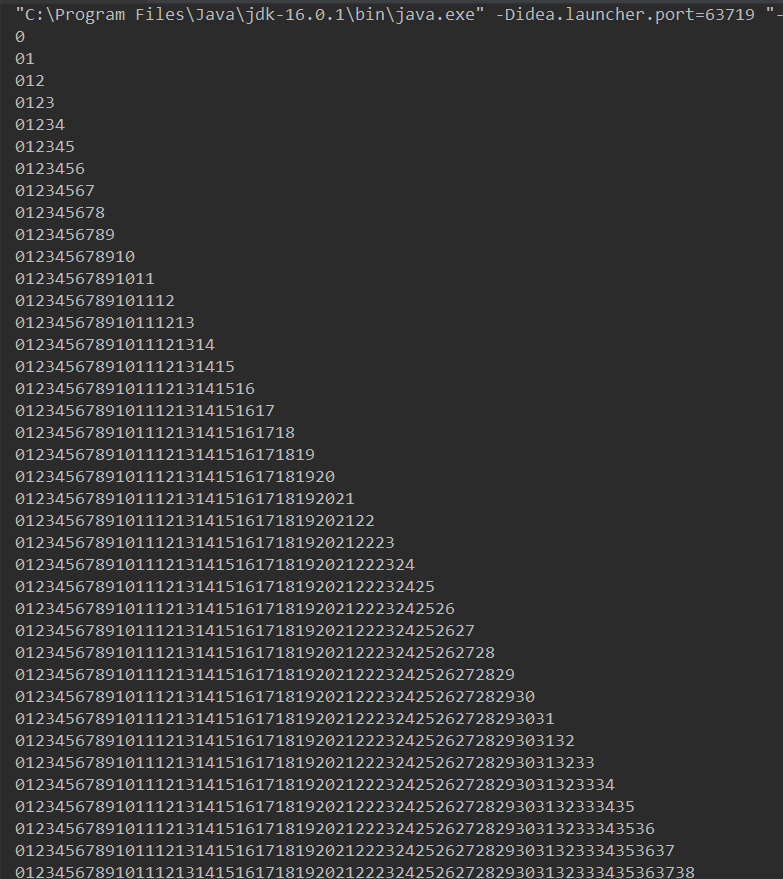
方法内存图:
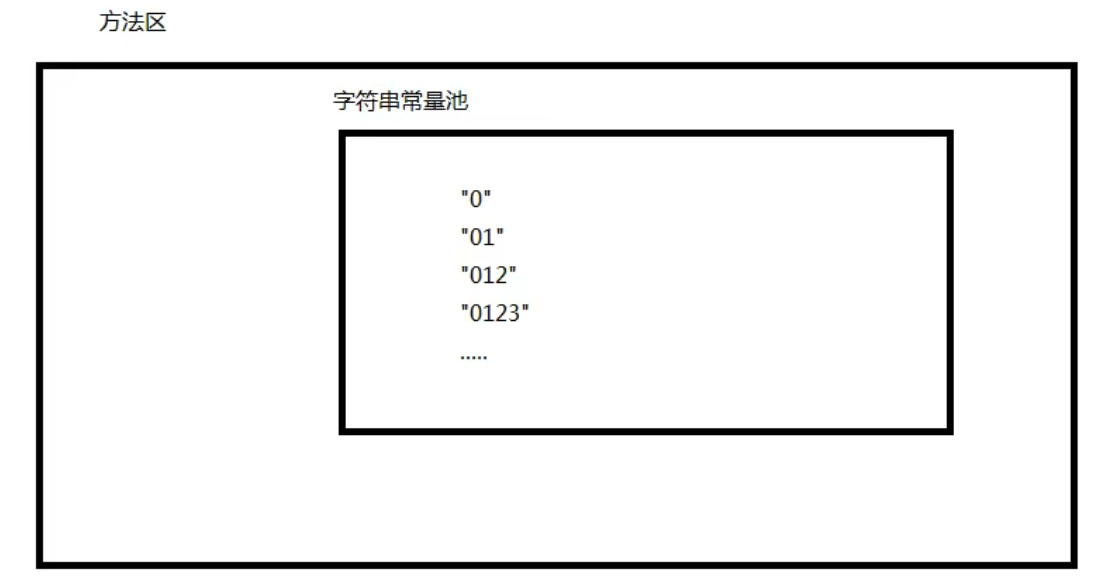 总结:以后需要进行大量字符串的拼接操作,建议使用JDK中自带的
- java.lang.StringBuffer
- java.lang.StringBuilder
5.1 StringBuffer
- 拼接字符串,以后拼接字符串统一调用append()方法,append是追加的意思
- append方法底层在进行追加的时候,如果byte数组满了,会自动扩容
- 如何优化StringBuffer的性能?
答:在创建StringBuffer的时候尽可能给定一个初始化容量,最好减少底层数组的扩容次数,预估一下,给个大一些的初始化容量 - 给一个合适的初始化容量,可以提高程序的执行效率
package StringBuffer;
public class StringBufferTest01 {
public static void main(String[] args) {
StringBuffer stringBuffer = new StringBuffer();
stringBuffer.append("a");
stringBuffer.append("b");
stringBuffer.append("c");
System.out.println(stringBuffer);
StringBuffer sb = new StringBuffer(100);
sb.append("d");
sb.append("e");
sb.append("f");
System.out.println(sb);
}
}
5.2 StringBuilder
package StringBuffer;
public class StringBuilderTest01 {
public static void main(String[] args) {
StringBuilder stringBuilder = new StringBuilder();
stringBuilder.append("我");
stringBuilder.append("爱");
stringBuilder.append("你");
System.out.println(stringBuilder);
}
}
5.3 StringBuffer和StringBuilder的区别
- StringBuffer中的方法都有:synchronized关键字修饰,表示StringBuffer在多线程环境下运行是安全的
- StringBuilder中的方法都没有:synchronized关键字修饰,表示StringBuilder在多线程环境下运行是不安全的
- StringBuffer是线程安全的
- StringBuilder是非线程安全的
|