- 多项式求和规则建议百度
- 我使用的是顺序存储的线性表,代码比链式存储相对简单许多,较易懂,建议直接看代码中的Add方法即可
#include <iostream>
#define MAXSIZE 100
using namespace std;
typedef struct {
double x;
int y;
}P;
typedef struct {
P* elem;
int length;
}SqList;
int InitList(SqList& L)
{
L.elem = new P[MAXSIZE];
if (!L.elem) {
return 0;
}
L.length = 0;
return 1;
}
int ListInsert(SqList& L, int i, P &e)
{
if (L.length == MAXSIZE || i < 1 || i > L.length + 1) {
return 0;
}
for (int j = L.length - 1; j >= i - 1; j--) {
L.elem[j + 1] = L.elem[j];
}
L.elem[i - 1] = e;
L.length++;
return 1;
}
int ListDelete(SqList& L, int i)
{
if (i < 1 || i > L.length) {
return 0;
}
for (int j = i; j <= L.length - 1; j++) {
L.elem[j - 1] = L.elem[j];
}
--L.length;
return 1;
}
void DisplaySqlist(SqList& L)
{
for (int i = 0; i < L.length; i++) {
if (L.elem[i].x == 0)
continue;
if(i != 0)
cout << " + ";
cout << L.elem[i].x << "x^" << L.elem[i].y;
}
cout << endl;
}
void CreateDuo(SqList& L, P a[], int n)
{
for (int i = 0; i < n; i++) {
ListInsert(L, i + 1, a[i]);
}
}
void Swap(P& a, P& b) {
P t = a;
a = b;
b = t;
}
void BubbleSort(SqList& L) {
for (int i = L.length - 1; i >= 0; i--) {
for (int j = 0; j < i; j++) {
if (L.elem[j].y < L.elem[j + 1].y) {
Swap(L.elem[j], L.elem[j + 1]);
}
}
}
}
void Add(SqList& L1, SqList& L2, SqList& L3) {
int i = 0, j = 0, z = 1;
while (i < L1.length && j < L2.length) {
if (L1.elem[i].y == L2.elem[j].y) {
P p = { L1.elem[i].x + L2.elem[j].x, L1.elem[i].y };
ListInsert(L3, z++, p);
i++;
j++;
}
else if (L1.elem[i].y > L2.elem[j].y) {
ListInsert(L3, z++, L1.elem[i]);
i++;
}
else{
ListInsert(L3, z++, L2.elem[j]);
j++;
}
}
while (i < L1.length) {
ListInsert(L3, z++, L1.elem[i++]);
}
while (j < L2.length) {
ListInsert(L3, z++, L2.elem[j++]);
}
}
int main()
{
SqList list1;
SqList list2;
SqList list3;
InitList(list1);
InitList(list2);
InitList(list3);
P a[] = { {3.2, 0}, {2.5, 1},{2.2, 2},{-2.5, 3} };
P b[] = { {-1.2, 0},{2.5, 1},{3.2, 2},{2.5, 3},{5.4, 4} };
CreateDuo(list1, a, sizeof(a) / sizeof(P));
CreateDuo(list2, b, sizeof(b) / sizeof(P));
cout << "多项式1:\t";
DisplaySqlist(list1);
cout << "多项式2:\t";
DisplaySqlist(list2);
BubbleSort(list1);
BubbleSort(list2);
cout << "有序多项式1:\t";
DisplaySqlist(list1);
cout << "有序多项式2:\t";
DisplaySqlist(list2);
Add(list1, list2, list3);
cout << "多项式相加:\t";
DisplaySqlist(list3);
return 0;
}
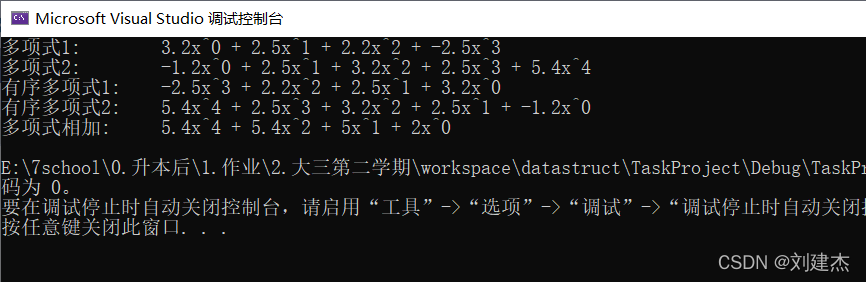
|