力扣24 两两交换链表中的值
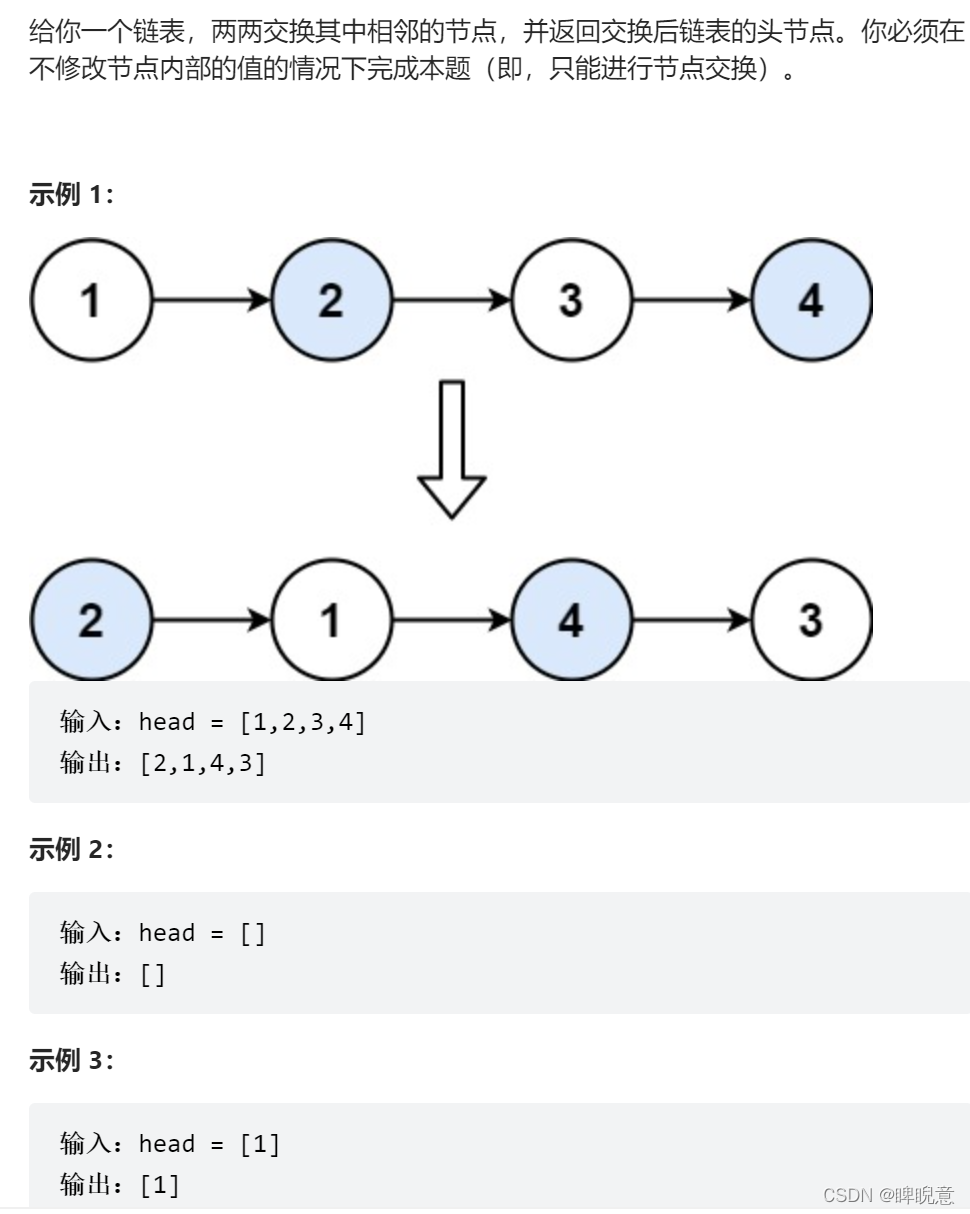
第一步,发现头结点需要改变 先创建一个虚拟头结点,称为X 在真实头结点A 之前,A的下一个节点称为B节点 对于虚拟头结点来说,第一轮需要交换的是 这个虚拟头结点的后节点一届后后节点,即需要交换A 和 B 第二步,先将当前节点的next指向后后节点 (将X的next指向B) 第三步,将后节点的next指向后后节点的next(将A的next 指向 B的next) 第四步,将后后节点的next指向后节点(将B 的next指向A) 第一轮交换完毕,想要进行下一轮交换就需要改变虚拟头结点的位置,然后重复操作。(将A视为虚拟节点,开始对接下来的部分进行交换)
class Solution {
public:
ListNode* swapPairs(ListNode* head) {
if(head == NULL || head -> next == NULL){
return head;
}
ListNode *p = new ListNode(0, head);
ListNode *now = p;
while(now -> next != NULL && now -> next -> next != NULL){
ListNode *l = now -> next;
ListNode *r = now -> next -> next;
now -> next = r;
l -> next = r -> next;
r -> next = l;
now = l;
}
return p->next;
}
};
递归解法
首先要了解swapPairs函数的作用就是 将传入的节点和下一个节点进行交换,然后返回交换后的头结点 进行交换, 交换两个链表中的节点需要三个步骤 (这里的A 指代需要交换的第一个节点 ,B指代需要交换的另一个节点 C指代还未发生交换的节点) A B C 初始的状态是 A的next指向B,B的next指向C 交换步骤 1:用指针记录B 的位置(因为一开始题目只会给A的位置,要确定B的位置才能进行交换) 2:将 A的next 指向C 3:将B的next指向A
class Solution {
public:
ListNode* swapPairs(ListNode* head) {
if(head == NULL || head -> next == NULL){
return head;
}
ListNode *B = head->next;
head -> next = swapPairs(B -> next);
B -> next = head;
return B;
}
};
判断一个数字是否是回文串
int func(int a){
int temp = a;
int r = 0;
while(a){
r = r * 10 + a % 10;
a = a / 10;
}
return r == temp;
}
Sine之舞
#include<iostream>
using namespace std;
int A(int i, int n){
if(i == n){
cout << "sin(" << i << ")";
}
else{
if(i % 2 == 0) cout << "sin(" << i << "+";
else cout << "sin(" << i << "-";
A(i + 1, n);
cout << ")";
}
}
int S(int i, int n){
if(n == 1){
A(1, 1);
cout << "+" << i;
}
else{
cout << "(";
S(i + 1, n - 1);
cout << ")";
A(1, n);
cout << "+" << i;
}
}
int main()
{
int n;
cin >> n;
S(1, n);
return 0;
}
回形取数(经典dfs)
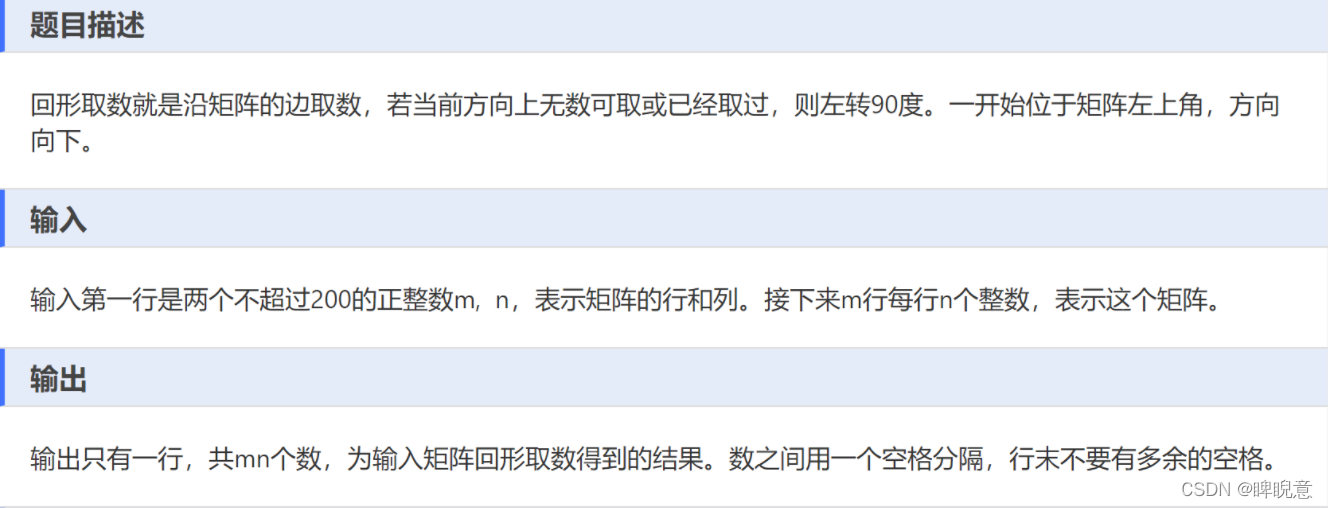
样例输入
3 3 1 2 3 4 5 6 7 8 9
样例输出
1 4 7 8 9 6 3 2 5
#include<iostream>
using namespace std;
const int maxn = 201;
int w[maxn][maxn];
int f[maxn][maxn] = {0};
int dir[4][2] = {1, 0, 0, 1, -1, 0, 0, -1};
int n, m;
bool check(int x, int y){
return (x >= 0 && y >= 0 && x < n && y < m && f[x][y] != 1);
}
void dfs(int x, int y, int i, int j){
if(f[x][y] == 0 && x == 0 && y == 0) cout << w[x][y];
else if(f[x][y] == 0) cout << " " << w[x][y];
f[x][y] = 1;
int xx = x + i;
int yy = y + j;
if(check(xx, yy)) dfs(xx, yy, i, j);
else{
for(int i = 0; i < 4; i++){
int dx = x + dir[i][0];
int dy = y + dir[i][1];
if(check(dx, dy)){
dfs(dx, dy, dir[i][0], dir[i][1]);
break;
}
}
}
}
int main()
{
cin >> n >> m;
for(int i = 0; i < n; i++){
for(int j = 0; j < m; j++){
cin >> w[i][j];
}
}
dfs(0, 0, 1, 0);
return 0;
}
危险系数
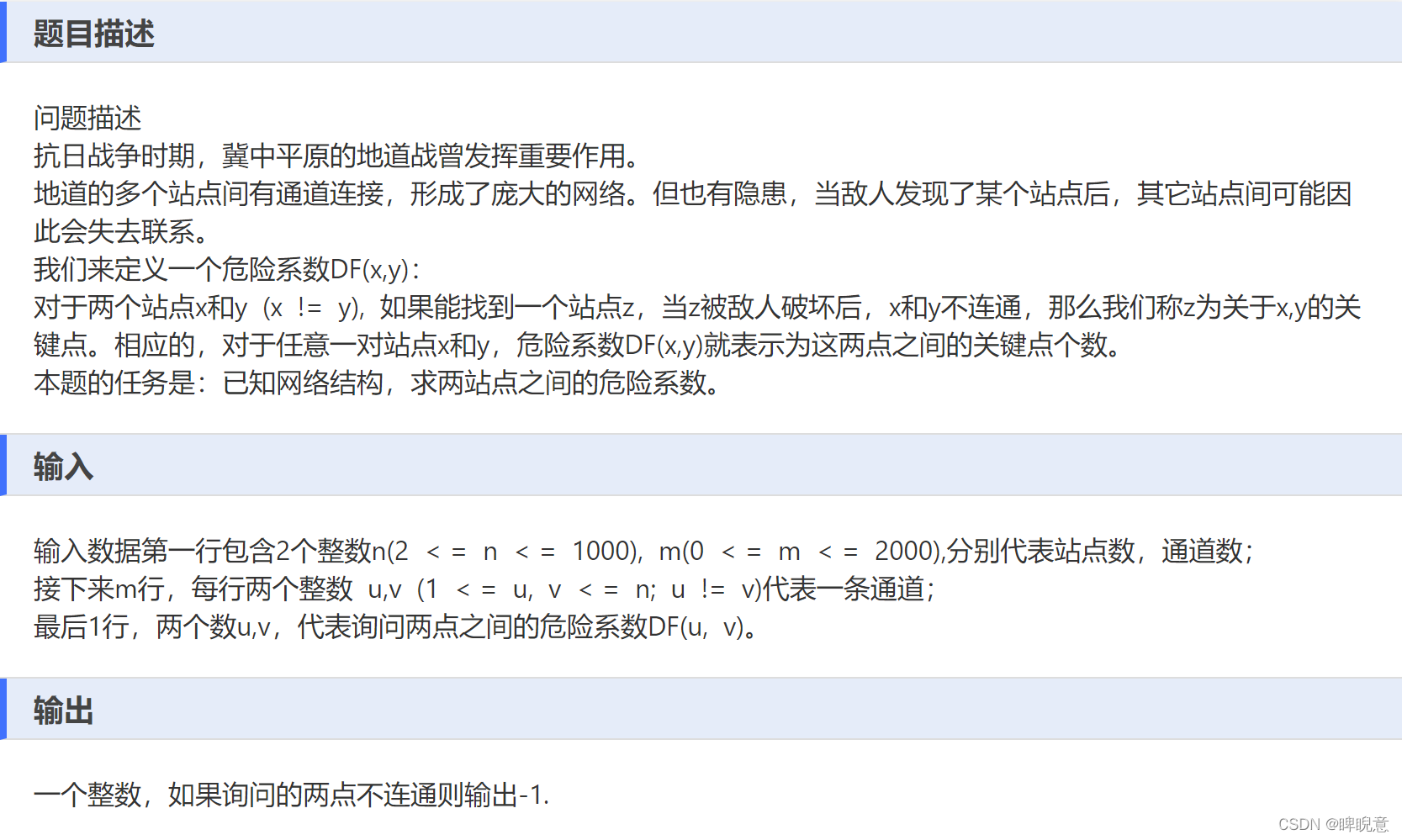
样例输入
7 6 1 3 2 3 3 4 3 5 4 5 5 6 1 6
样例输出
2
#include<iostream>
#include<vector>
using namespace std;
const int maxn = 1001;
vector<int> v[maxn];
int f[maxn] = {0};
int count = 0;
int success_load = 0;
int pass_by[maxn];
int n, m;
int e, c;
void dfs(int now){
f[now] = 1;
if(now == c){
success_load++;
for(int i = 1; i <= n; i++){
if(f[i] == 1) pass_by[i]++;
}
f[now] = 0;
return ;
}
for(int i = 0; i < v[now].size(); i++){
if(f[ v[now][i] ] == 0){
dfs(v[now][i]);
}
}
f[now] = 0;
}
int main(){
cin >> n >> m;
int a, b;
for(int i = 0; i < m; i++){
cin >> a >> b;
v[a].push_back(b);
v[b].push_back(a);
}
cin >> e >> c;
dfs(e);
for(int i = 1; i <= n; i++){
if(pass_by[i] == success_load && i != e && i != c){
count++;
}
}
if(success_load == 0) cout << "-1";
else cout << count;
return 0;
}
|