LeetCode算法入门(第二十六天)
二叉树
226.翻转二叉树
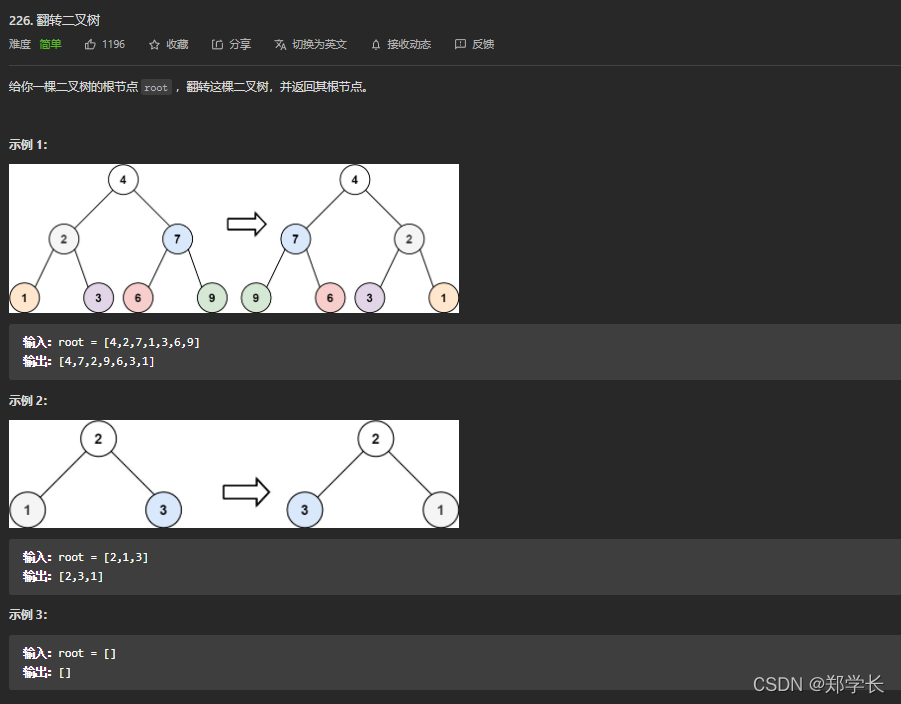
交换左右子树,直到叶子节点,叶子节点为没有子节点的节点。
class Solution {
public:
TreeNode* invertTree(TreeNode* root) {
if(root == NULL)
return root;
swap(root->left, root->right);
invertTree(root->left);
invertTree(root->right);
return root;
}
};
112.路径总和
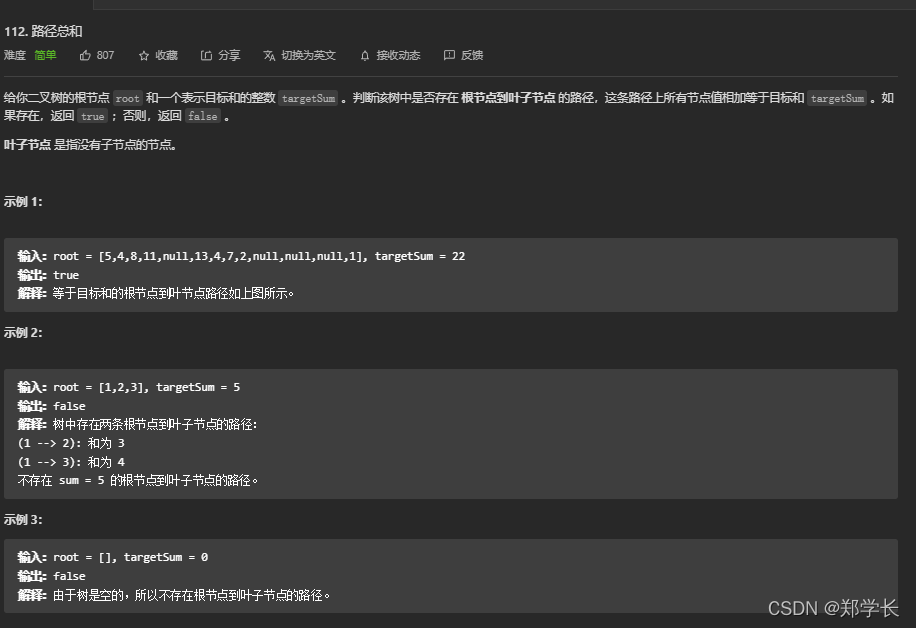
class Solution {
public:
bool traversal(TreeNode* cur, int count){
if(!cur->left && !cur->right && count == 0)
return true;
if(!cur->left && !cur->right)
return false;
if(cur->left){
count -= cur->left->val;
if(traversal(cur->left, count))
return true;
count += cur->left->val;
}
if(cur->right){
count -= cur->right->val;
if(traversal(cur->right, count))
return true;
count += cur->right->val;
}
return false;
}
bool hasPathSum(TreeNode* root, int targetSum) {
if(root == NULL)
return false;
return traversal(root, targetSum - root->val);
}
};
|