注意标准形式
下面两个方法约束规划的一般标准形式为: 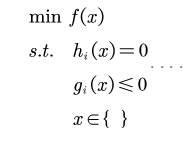
利用scikit-opt的遗传算法求解约束规划问题
先放上链接:scikit-opt网址 主要四个步骤: 下面依照此题多约束为例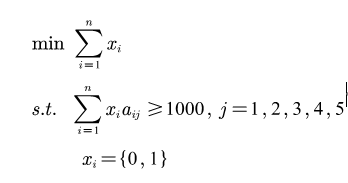
可知该题有5个不等式约束,且决策变量为01整数,后面将具体讲解如何将目标函数的约束条件加入GA模型中
一:import scikit-opt库
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
from sko.GA import GA
二:定义目标函数
看线面的代码我们发现里面有个参数p,这个参数我理解就是GA中的某一个种群,真正调用这个函数的时候我们不需要写参数和括号
def schaffer(p):
'''
目标函数: min(xi)求和
决策变量维度:n
决策变量类型:0 1 整数
:param p:
:return:
'''
x=np.array(p)
return sum(x)
三:定义约束
注意这里遗传算法中的约束应该默认为≤0的形式,而且不管有多少个约束,最终将约束的值及你给过一个max函数累加后返回,同样这里也需要决策变量,这里也是参数P,我们真正调用时,不需要写括号和参数
def constraint_yueshu(p):
x=np.array(p)
he = 0
for j in range(5):
tem=0
for i in range(n):
tem+=x[i]*a[i][j]
he+=max(0,tem)
print(he)
return he
constraint= [constraint_yueshu]
四:代入模板函数,设定相关参数
n=10
ga = GA(func=schaffer, n_dim=n, size_pop=50, max_iter=800, prob_mut=0.001, lb=[0]*n, ub=[1]*n, precision=[1]*n,constraint_ueq=constraint)
best_x, best_f = ga.run()
print('best_x:', best_x, '\n', 'best_y:', best_f)
参数说明:(官方文档有) 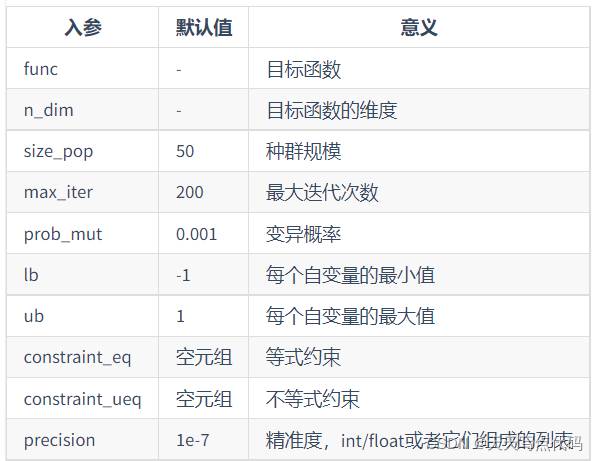
五:结果可视化
Y_history = pd.DataFrame(ga.all_history_Y)
fig, ax = plt.subplots(2, 1)
ax[0].plot(Y_history.index, Y_history.values, '.', color='red')
Y_history.min(axis=1).cummin().plot(kind='line')
plt.show()
六:完整代码:
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
from sko.GA import GA
def schaffer(p):
'''
目标函数: min(xi)求和
决策变量维度:n
决策变量类型:0 1 整数
:param p:
:return:
'''
x=np.array(p)
return sum(x)
def constraint_yueshu(p):
x=np.array(p)
he = 0
for j in range(5):
tem=0
for i in range(n):
tem+=x[i]*a[i][j]
he+=max(0,tem)
print(he)
return he
constraint= [constraint_yueshu]
n=10
ga = GA(func=schaffer, n_dim=n, size_pop=50, max_iter=800, prob_mut=0.001, lb=[0]*n, ub=[1]*n, precision=[1]*n,constraint_ueq=constraint)
best_x, best_f = ga.run()
print('best_x:', best_x, '\n', 'best_y:', best_f)
Y_history = pd.DataFrame(ga.all_history_Y)
fig, ax = plt.subplots(2, 1)
ax[0].plot(Y_history.index, Y_history.values, '.', color='red')
Y_history.min(axis=1).cummin().plot(kind='line')
plt.show()
利用scipy.optimize求解
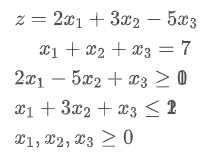
详细见代码 参考自:代码来源
'''参考自:https://blog.csdn.net/qq_39241986/article/details/104832513'''
import numpy as np
from scipy.optimize import minimize
def objective(x):
return x[0]**2+x[1]**2+x[2]**2+8
def constraint1(x):
return x[0]**2-x[1]+x[2]**2
def constraint2(x):
return -(x[0]+x[1]**2+x[2]**2-20)
def constraint3(x):
return -x[0]-x[1]**2+2
def constraint4(x):
return x[1]+2*x[2]**2-3
con1 = {'type': 'ineq', 'fun': constraint1}
con2 = {'type': 'ineq', 'fun': constraint2}
con3 = {'type': 'eq', 'fun': constraint3}
con4 = {'type': 'eq', 'fun': constraint4}
cons=([con1,con2,con3,con4])
b=(0,None)
bnds=(b,b,b)
x=np.array([0,0,0])
solution=minimize(objective,x,method='SLSQP',bounds=bnds,constraints=cons)
x=solution.x
print('目标值: ' + str(objective(x)))
print('最优解为')
print('x1 = ' + str(round(x[0],2)))
print('x2 = ' + str(round(x[1],2)))
print('x3 = ' + str(round(x[2],2)))
print(solution)
利用geatpy
注意,其实仔细看geatpy官方文档是比较好改的。需要改的也只有自定义的目标函数了,虽然看着后面很多代码很复杂,但是其实都不用动。 可以看官方文档中的《数据结构》和《快速入门》来改函数。 看数据结构方便自己自定义目标函数的约束条件。 文档网址
import numpy
import pip
import pandas as pd
import numpy as np
import geatpy as ea
weidu=402
path='E:\桌面\gyx.xlsx'
dataframe=pd.read_excel(path,'供')
data=dataframe.iloc[0:402,1:dataframe.shape[1]].values
class MyProblem(ea.Problem):
def __init__(self):
name = 'MyProblem'
M = 1
maxormins = [1]
Dim = weidu
varTypes = [1] * Dim
lb = [0]*Dim
ub = [1]*Dim
lbin = [1]*Dim
ubin = [1]*Dim
ea.Problem.__init__(self, name, M, maxormins, Dim, varTypes, lb,ub, lbin, ubin)
def aimFunc(self, pop):
Vars = pop.Phen
x=[[] for i in range(weidu)]
for i in range(weidu):
x[i]=Vars[:,[i]]
pop.ObjV=x[0]
for i in range(1,weidu):
pop.ObjV +=x[i]
list = []
for i in range(24):
tem = 0
for j in range(weidu):
if data[j][0] == 'A':
tem += data[j][i + 1] * x[j] / 0.6
elif data[j][0] == 'B':
tem += data[j][i + 1] * x[j] / 0.66
else:
tem += data[j][i + 1] * x[j] / 0.72
list.append(2*1e4-tem)
pop.CV = np.hstack(list)
"""============================实例化问题对象========================"""
problem = MyProblem()
"""==============================种群设置==========================="""
Encoding = 'RI'
NIND = 100
Field = ea.crtfld(Encoding, problem.varTypes, problem.ranges,
problem.borders)
population = ea.Population(Encoding, Field, NIND)
"""===========================算法参数设置=========================="""
myAlgorithm = ea.soea_DE_best_1_L_templet(problem, population)
myAlgorithm.MAXGEN = 1500
myAlgorithm.mutOper.F = 0.6
myAlgorithm.recOper.XOVR = 0.8
myAlgorithm.logTras = 100
myAlgorithm.verbose = True
myAlgorithm.drawing = 1
"""==========================调用算法模板进行种群进化==============="""
[BestIndi, population] = myAlgorithm.run()
BestIndi.save()
"""=================================输出结果======================="""
print('评价次数:%s' % myAlgorithm.evalsNum)
print('时间已过 %s 秒' % myAlgorithm.passTime)
if BestIndi.sizes != 0:
print('最优的目标函数值为:%s' % BestIndi.ObjV[0][0])
print('最优的控制变量值为:')
for i in range(BestIndi.Phen.shape[1]):
print(BestIndi.Phen[0, i])
else:
print('没找到可行解。')
注:此题的例子是2021数学建模国赛的C题066论文约束规划问题的简化
|