vector的模拟实现
类的设计
用三个指针分别指向开始位置,最后一个数据的下一个位置,空间分配的最大位置,通过指针运算来确定数据大小和容量
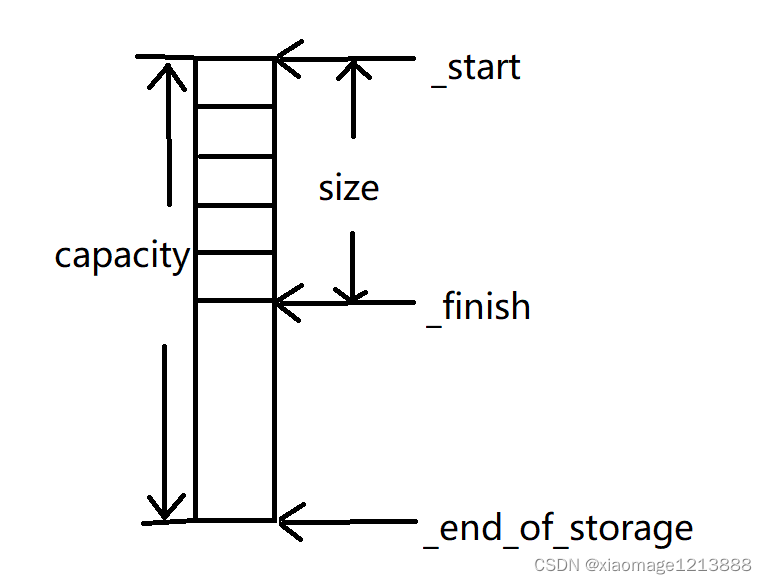
vector类的设计要用模板来实现,实现元素的可变性,把vector的变量参数设置成_Ty,
指针可以直接进行访问操作,所以vector的迭代器就可以用指针封重命名得到。
template<class _Ty>
class vector {
public:
typedef _Ty* iterator;
iterator begin() {
return _start;
}
iterator end() {
return _finish;
}
private:
iterator _start;
iterator _finish;
iterator _end_of_storage;
};
vector的构造和析构
vector()
:_start(nullptr)
, _finish(nullptr)
, _end_of_storage(nullptr)
{}
vector(int n, const _Ty& value = _Ty())
:_start(nullptr)
, _finish(nullptr)
, _end_of_storage(nullptr)
{
reserve(n);
while (n--) {
push_back(value);
}
}
vector(vector<_Ty>& v)
:_start(nullptr)
, _finish(nullptr)
, _end_of_storage(nullptr)
{
reserve(v.capacity());
for (int i = 0; i < v.size(); ++i) {
_finish[i] = v[i];
}
_finish = _start + v.size();
}
template<class InputIterator>
vector(InputIterator first, InputIterator last) {
reserve(last - first);
while (first != last) {
push_back(*first);
++first;
}
}
vector<_Ty>& operator=(vector<_Ty> t) {
swap(t);
return *this;
}
~vector() {
if (_start) {
delete[] _start;
_start = nullptr;
_finish = nullptr;
_end_of_storage = nullptr;
}
}
string容量操作
size_t size() const {
return _finish - _start;
}
size_t capacity() const {
return _end_of_storage - _start;
}
bool empty() const {
return size() == 0;
}
void reserve(size_t n) {
size_t oldSize = size();
if (n > capacity()) {
_Ty* new_start = new _Ty[n];
if (_start) {
for (int i = 0; i < oldSize; ++i) {
new_start[i] = _start[i];
}
}
delete[] _start;
_start = new_start;
_finish = _start + oldSize;
_end_of_storage = _start + n;
}
}
void resize(size_t n, const _Ty& value = _Ty()) {
if (n <= size()) {
_finish = _start + n;
return;
}
if (n > capacity()) {
reserve(n);
}
size_t offset = n - size();
while (offset--) {
*_finish = value;
++_finish;
}
}
vector访问操作
_Ty& operator[](size_t pos) {
return _start[pos];
}
const _Ty& operator[](size_t pos)const {
return _start[pos];
}
vector增删查改
void push_back(const _Ty& val) {
insert(end(), val);
}
void pop_back() {
erase((end() - 1));
}
iterator erase(iterator pos) {
iterator begin = pos;
while (begin != end()) {
*begin = *(begin + 1);
++begin;
}
--_finish;
return pos;
}
iterator insert(iterator pos, const _Ty& val) {
assert(pos >= _start && pos <= _finish);
if (_finish == _end_of_storage) {
size_t oldPos = pos - _start;
reserve(capacity() == 0 ? 1 : 2 * capacity());
pos = _start + oldPos;
}
iterator end = _finish - 1;
while (end >= pos) {
*(end + 1) = *end;
--end;
}
*pos = val;
++_finish;
return pos;
}
void swap(vector<_Ty>& v) {
std::swap(_start, v._start);
std::swap(_finish, v._finish);
std::swap(_end_of_storage, v._end_of_storage);
}
完整代码实现
#include<iostream>
#include<vector>
#include<assert.h>
namespace xiaomage {
template<class _Ty>
class vector {
public:
typedef _Ty* iterator;
typedef const _Ty* const_iterator;
iterator begin() {
return _start;
}
iterator end() {
return _finish;
}
vector()
:_start(nullptr)
,_finish(nullptr)
,_end_of_storage(nullptr)
{}
vector(int n, const _Ty& value = _Ty())
:_start(nullptr)
, _finish(nullptr)
, _end_of_storage(nullptr)
{
reserve(n);
while (n--) {
push_back(value);
}
}
vector(vector<_Ty>& v)
:_start(nullptr)
, _finish(nullptr)
, _end_of_storage(nullptr)
{
reserve(v.capacity());
for (int i = 0; i < v.size(); ++i) {
_finish[i] = v[i];
}
_finish = _start + v.size();
}
template<class InputIterator>
vector(InputIterator first, InputIterator last) {
reserve(last - first);
while (first != last) {
push_back(*first);
++first;
}
}
vector<_Ty>& operator=(vector<_Ty> t) {
swap(t);
return *this;
}
~vector() {
if (_start) {
delete[] _start;
_start = nullptr;
_finish = nullptr;
_end_of_storage = nullptr;
}
}
_Ty& operator[](size_t pos) {
return _start[pos];
}
const _Ty& operator[](size_t pos)const {
return _start[pos];
}
size_t size() const{
return _finish - _start;
}
size_t capacity() const {
return _end_of_storage - _start;
}
bool empty() const {
return size() == 0;
}
void reserve(size_t n) {
size_t oldSize = size();
if (n > capacity()) {
_Ty* new_start = new _Ty[n];
if (_start) {
for (int i = 0; i < oldSize; ++i) {
new_start[i] = _start[i];
}
}
delete[] _start;
_start = new_start;
_finish = _start + oldSize;
_end_of_storage = _start + n;
}
}
void resize(size_t n, const _Ty& value = _Ty()) {
if (n <= size()) {
_finish = _start + n;
return;
}
if (n > capacity()) {
reserve(n);
}
size_t offset = n - size();
while (offset--) {
*_finish = value;
++_finish;
}
}
void push_back(const _Ty& val) {
insert(end(), val);
}
void pop_back() {
erase((end() - 1));
}
iterator erase(iterator pos) {
iterator begin = pos;
while (begin!=end()) {
*begin = *(begin + 1);
++begin;
}
--_finish;
return pos;
}
iterator insert(iterator pos, const _Ty& val) {
assert(pos >= _start && pos <= _finish);
if (_finish == _end_of_storage) {
size_t oldPos = pos - _start;
reserve(capacity() == 0 ? 1 : 2 * capacity());
pos = _start + oldPos;
}
iterator end = _finish-1;
while (end>=pos) {
*(end + 1) = *end;
--end;
}
*pos = val;
++_finish;
return pos;
}
void swap(vector<_Ty>& v) {
std::swap(_start, v._start);
std::swap(_finish, v._finish);
std::swap(_end_of_storage, v._end_of_storage);
}
private:
iterator _start;
iterator _finish;
iterator _end_of_storage;
};
}
|