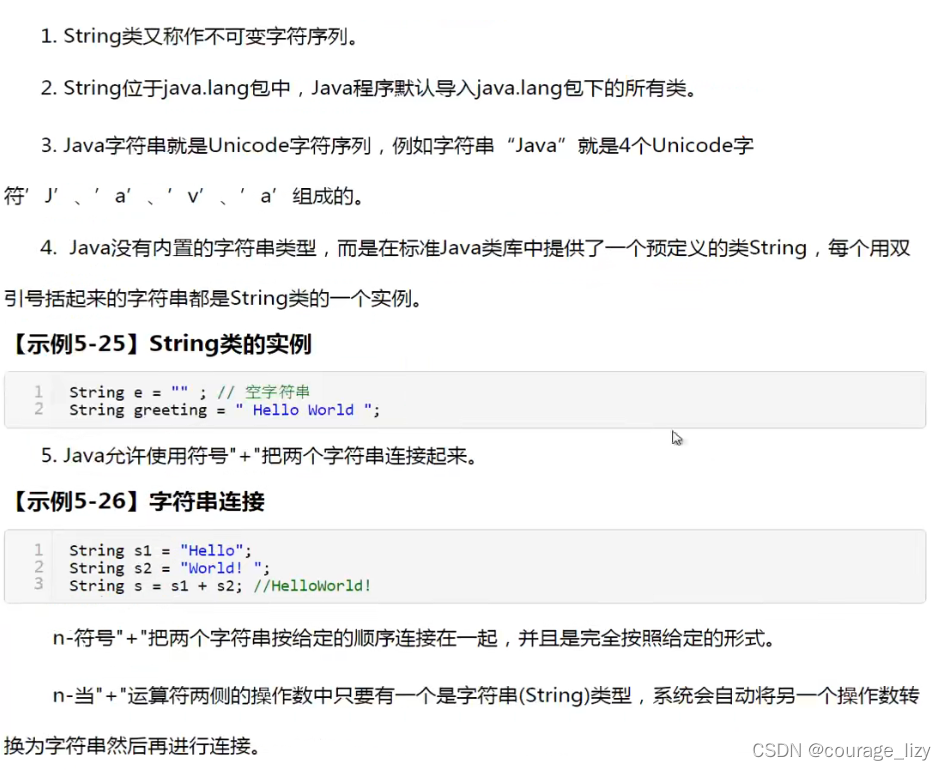
?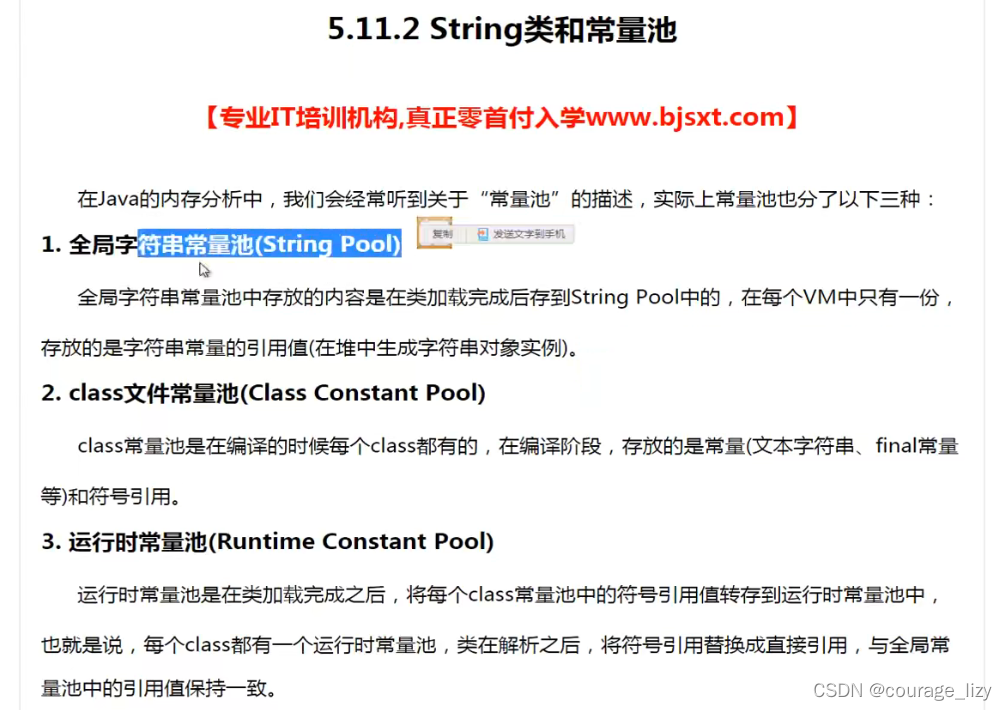
?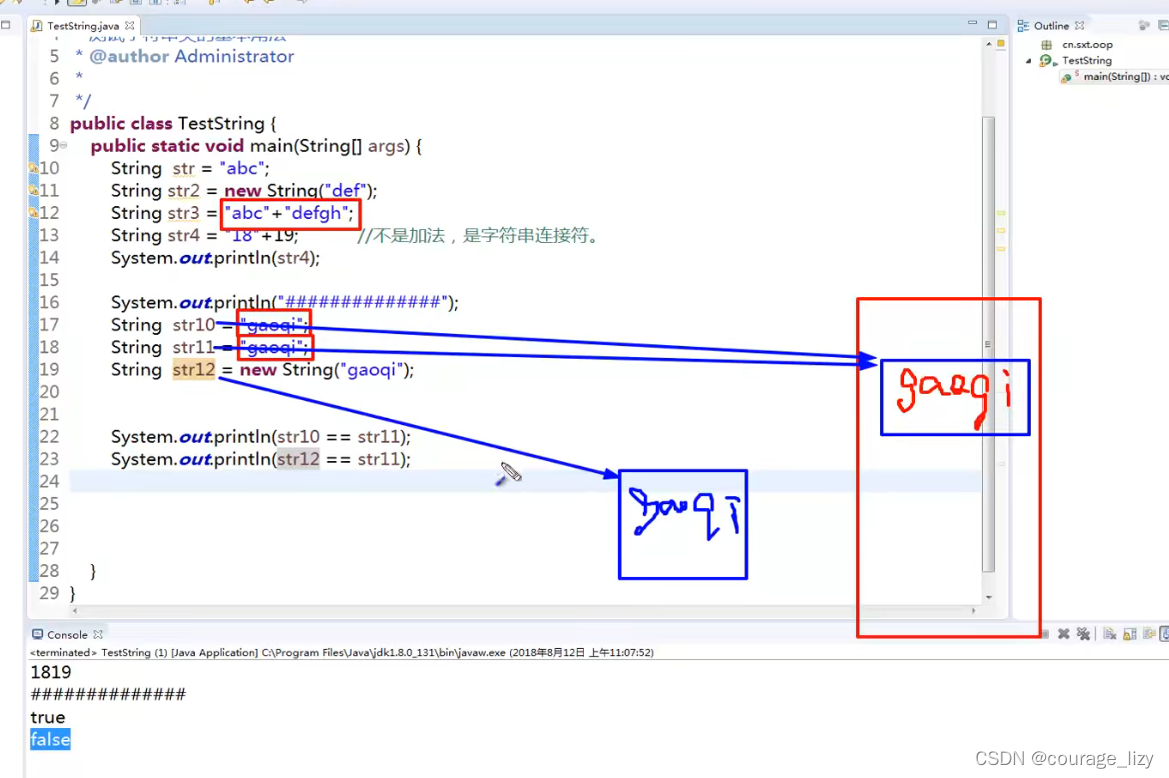
package JA;
public class TestString {
public static void main(String[] args) {
String str="abc";
String str2=new String("def");
String str3="abc"+"defgh";
String str4="18"+19;
System.out.println(str4);
String str10="gaoqi";
String str11="gaoqi";
String str12=new String("gaoqi");
System.out.println(str10==str11);
System.out.println(str12==str11);
//通常比较字符串时 使用equals
System.out.println(str12.equals(str11));
}
}
String类的常用方法
package JA;
import java.util.Locale;
//测试String的常用用法
public class TestString2 {
public static void main(String[] args) {
String s1="core Java";
String s2="Core Java";
System.out.println(s1.charAt(3));//提取下标为3的字符
System.out.println(s2.length());//字符串的长度
System.out.println(s1.equals(s2));//比较两个字符串是否相等
System.out.println(s1.equalsIgnoreCase(s2));//比较两个字符串(忽略大小写)
System.out.println(s1.indexOf("Java"));//字符串s1中是否包含Java 有的话返回J的位置 没有返回-1
System.out.println(s1.indexOf("apple"));//字符串s1中是否包含apple
String s=s1.replace(' ','&');//将s1中的空格替换成&
System.out.println("result:"+s);
String s3="";
String s5="How are you?";
System.out.println(s5.startsWith("How"));//是否以How开头
System.out.println(s5.endsWith("you"));//是否以you结尾
s1=s5.substring(4);//提取字符串 从下标为4的开始到字符串结束为止
System.out.println(s1);
s1=s5.substring(4,7);//提取子字符串: 下标[4,7)不包含7
System.out.println(s1);
s1=s5.toLowerCase();//转小写
System.out.println(s1);
s1=s5.toUpperCase();//转大写
System.out.println(s1);
String s6=" How old are you ";
s=s6.trim();//去除字符串首尾的空格 注意:中间的空格不能去除
System.out.println(s6);
System.out.println(s);
}
}
数组的拷贝插入和删除
package JA;
public class TestArrayCopy {
public static void main(String[] args) {
String[] str={"阿里","京东","尚学堂","百度","亚马逊"};
removeElement(str,1);
str=extendRange(str);
String[] s1={"aa","bb","cc","dd","ee"};
String[] s2=new String[10];
System.arraycopy(s1,2,s2,6,3);
for(int i=0;i<s2.length;i++){
System.out.println(i+"--"+s2[i]);
}
}
public static String[] removeElement(String[] s,int index){
System.arraycopy(s,index+1,s,index,s.length-index-1);
s[s.length-1]=null;
for(int i=0;i<s.length;i++){
System.out.println(i+"--"+s[i]);
}
return s;
}
//数组的扩容
public static String[] extendRange(String[] s1){
String[] s2=new String[s1.length+10];
System.arraycopy(s1,0,s2,0,s1.length);//将s1中所有元素拷贝到s2
for(String temp:s2){
System.out.println(temp);
}
return s2;
}
}
|