题目
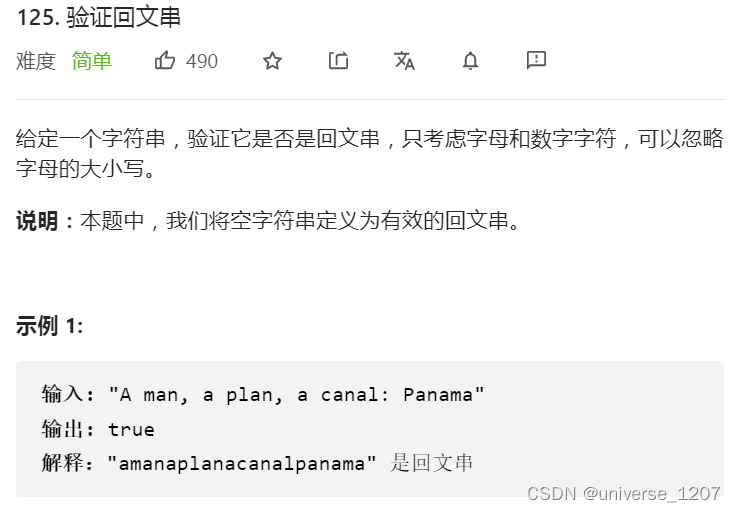
我的方法
- 思路:新建一个列表,储存所有有效字符的Ascii码,并统一大小写,再用双指针遍历一遍列表,看是否回文
class Solution:
def isPalindrome(self, s: str) -> bool:
asc = []
for i in range(len(s)):
a = ord(s[i])
if a>=ord('A') and a<=ord('Z'):
asc.append(a+ord('a')-ord('A'))
elif a>=ord('a') and a<=ord('z'):
asc.append(a)
elif a>=ord('0') and a<=ord('9'):
asc.append(a)
end = len(asc)-1
for i in range(int(len(asc)/2)):
if asc[i] == asc[end]:
end -= 1
else:
return False
return True
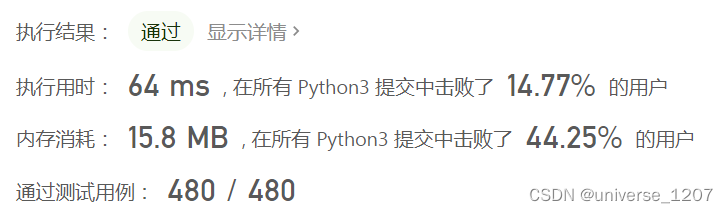
升级版
- 其实python中有很多内置函数可以好好利用
- 比如
lower() 函数是统一大小写的 - 还有
isalnum() 函数是判断char是否是数字和字母的,如果不是则返回0,是则返回不为0的数字 - 上面思路的一个步骤:预处理字符,使其只有数字和字母且字母要统一大小写,一行代码就出来了
sgood = "".join(ch.lower() for ch in s if ch.isalnum())
- 关于这个
join() 就和list.append() 一样 - 如果不采用双指针的方法,可以直接将新的字符串反转,看反转后的字符串与原字符串是否相等
- 这个方法完整的代码只有两行,哭,太简单了
class Solution:
def isPalindrome(self, s: str) -> bool:
sgood = "".join(ch.lower() for ch in s if ch.isalnum())
return sgood == sgood[::-1]
还有最省内存的方法
- 只在原s上判断即可
- 就是得每次找到真的能比较的位置有点麻烦
class Solution:
def isPalindrome(self, s: str) -> bool:
n = len(s)
left, right = 0, n - 1
while left < right:
while left < right and not s[left].isalnum():
left += 1
while left < right and not s[right].isalnum():
right -= 1
if left < right:
if s[left].lower() != s[right].lower():
return False
left, right = left + 1, right - 1
return True
- 我当时也想过这个办法,但是因为不知道统一大小写的内置函数,觉得每次都要比较ascii码很麻烦,就放弃了
|