稀疏数组
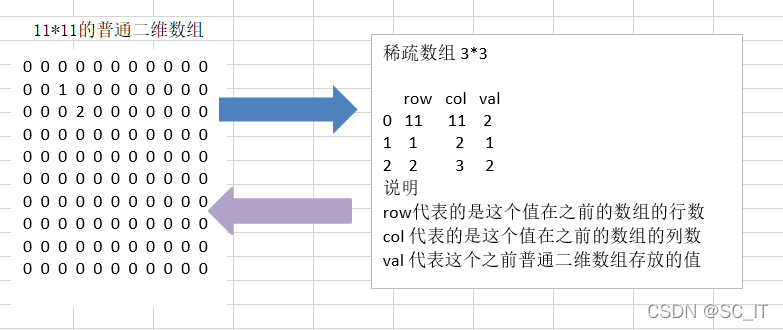
稀疏数组的实战(五子棋的编写 并保存到文件当中)
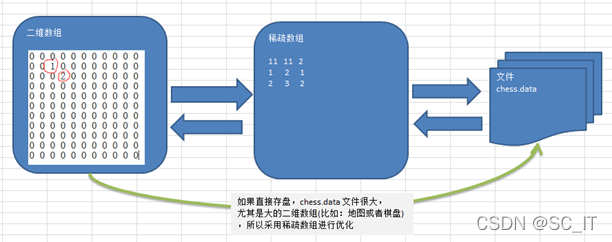
package com.song.sparse;
import java.io.File;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
public class SparseArray {
public static void main(String[] args) {
int[][] chessArr = new int[11][11];
chessArr[1][2] = 1;
chessArr[2][3] = 2;
int num = 0;
for (int[] row : chessArr) {
for (int data : row) {
if (data != 0) {
num++;
}
System.out.printf("%d\t", data);
}
System.out.println();
}
int[][] sparseArr = new int[num + 1][3];
sparseArr[0][0] = 11;
sparseArr[0][1] = 11;
sparseArr[0][2] = num;
int count = 0;
for (int i = 0; i < 11; i++) {
for (int j = 0; j < 11; j++) {
if (chessArr[i][j] != 0) {
count++;
sparseArr[count][0] = i;
sparseArr[count][1] = j;
sparseArr[count][2] = chessArr[i][j];
}
}
}
for (int i = 0; i < sparseArr.length; i++) {
System.out.printf("%d\t%d\t%d\n", sparseArr[i][0], sparseArr[i][1],sparseArr[i][2]);
}
String s = "";
for (int[] ints : sparseArr) {
for (int anInt : ints) {
s += anInt+"\t";
}
s+="\n";
}
File file = new File("Mydata");
FileWriter fileWriter = null;
try {
fileWriter = new FileWriter(file, false);
fileWriter.write(s);
} catch (IOException e) {
e.printStackTrace();
} finally {
if (fileWriter != null) {
try {
fileWriter.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
System.out.println("===================文件读取 转换为 字符串 然后将字符串转换为稀疏数组 再转为二维数组===================");
String s1 = "";
FileReader fr = null;
try {
fr = new FileReader(file);
char[] cbuf = new char[1024];
int len;
while ((len = fr.read(cbuf)) != -1) {
s1 =new String(cbuf, 0, len);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if (fr != null) {
try {
fr.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
String[] split = s1.split("\n");
System.out.println("从Mydata文件当中读取到数据---》稀疏数组");
int sparseArr2[][] = new int[split.length][3];
for(int i= 0 ;i<split.length;i++){
String[] split1 = split[i].split("\t");
for(int j=0 ;j<3;j++){
sparseArr2[i][j] = Integer.parseInt(split1[j]);
}
}
for (int i = 0; i < sparseArr2.length; i++) {
System.out.printf("%d\t%d\t%d\n", sparseArr2[i][0], sparseArr2[i][1], sparseArr2[i][2]);
}
int chessArr2 [][] = new int[sparseArr2[0][0]][sparseArr2[0][1]];
for (int i=1;i<sparseArr2.length;i++){
chessArr2[sparseArr2[i][0]][sparseArr2[i][1]] = sparseArr2[i][2];
}
System.out.println("稀疏数组恢复成二维数组");
for (int[] ints : chessArr2) {
for (int anInt : ints) {
System.out.printf("%d\t",anInt);
}
System.out.println();
}
}
}
#控制台打印的结果
0 0 0 0 0 0 0 0 0 0 0
0 0 1 0 0 0 0 0 0 0 0
0 0 0 2 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
11 11 2
1 2 1
2 3 2
===================文件读取 转换为 字符串 然后将字符串转换为稀疏数组 再转为二维数组===================
从Mydata文件当中读取到数据---》稀疏数组
11 11 2
1 2 1
2 3 2
稀疏数组恢复成二维数组
0 0 0 0 0 0 0 0 0 0 0
0 0 1 0 0 0 0 0 0 0 0
0 0 0 2 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0
Process finished with exit code 0
可以换成BufferedWriter/BufferedRead流来进行文件的存储 都其余差不多
package com.rg.java;
import java.io.*;
public class SparseTest {
public static void main(String[] args) {
int Array1[][] = new int[11][11];
Array1[1][2] = 1;
Array1[2][3] = 2;
Array1[5][6] = 9;
int index = 0;
for (int i = 0; i < 11; i++) {
for (int j = 0; j < 11; j++) {
if (Array1[i][j] != 0) {
index++;
}
System.out.print(Array1[i][j] + "\t");
}
System.out.println();
}
int sparseArray[][] = new int[index + 1][3];
int sum = 0;
sparseArray[0][0] = Array1.length;
sparseArray[0][1] = Array1.length;
sparseArray[0][2] = index;
for (int i = 0; i < 11; i++) {
for (int j = 0; j < 11; j++) {
if (Array1[i][j] != 0) {
sum++;
sparseArray[sum][0] = i;
sparseArray[sum][1] = j;
sparseArray[sum][2] = Array1[i][j];
}
}
}
System.out.println();
for (int i = 0; i < sparseArray.length; i++) {
for (int j = 0; j < 3; j++) {
System.out.print(sparseArray[i][j] + "\t");
}
System.out.println();
}
BufferedWriter butw = null;
try {
butw = new BufferedWriter(new FileWriter("map.data"));
for (int i = 0; i < sparseArray.length; i++) {
for (int j = 0; j < 3; j++) {
butw.write((sparseArray[i][j] + "\t"));
}
butw.write("\n");
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
butw.close();
} catch (IOException e) {
e.printStackTrace();
}
}
System.out.println();
BufferedReader bufferedReader = null;
int arr2[][] = new int[index + 1][3];
int getNum = 0;
String line;
try {
bufferedReader = new BufferedReader(new FileReader("map.data"));
while ((line=bufferedReader.readLine())!=null){
String[] temp = line.split("\t");
for (int j = 0; j < temp.length; j++) {
arr2[getNum][j]=Integer.parseInt(temp[j]);
}
getNum++;
}
} catch (IOException ex) {
ex.printStackTrace();
} finally {
try {
bufferedReader.close();
} catch (IOException ex) {
ex.printStackTrace();
}
}
int[][] Array4 = new int[arr2[0][0]][arr2[0][1]];
for(int i=1;i<arr2.length;i++){
for(int j=0;j<3;j++){
Array4[arr2[i][0]][arr2[i][1]] = arr2[i][2];
}
}
System.out.println();
for(int i=0;i<11;i++){
for(int j=0;j<11;j++){
System.out.print(Array4[i][j]+"\t");
}
System.out.println();
}
}
}
|