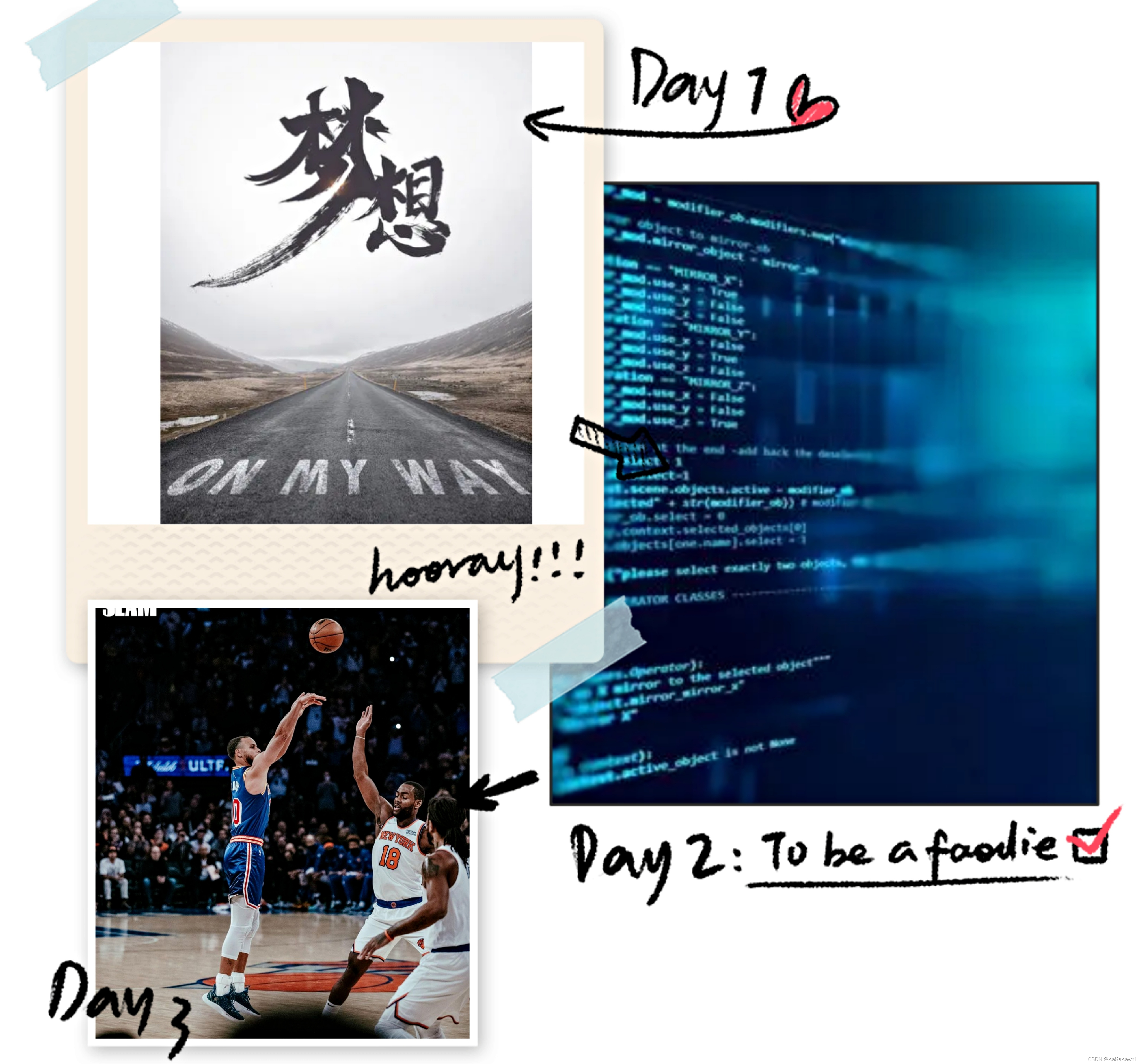
前言
本文总结学习单链表的各个接口实现。
链表是一种物理存储结构上非连续、非顺序的存储结构,数据元素的逻辑顺序是通过链表中的指针链接次序实现的。
一、链表头文件SListNode.h
本节主要包含:结构体类型创建,函数声明,头文件包含
#pragma once
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <errno.h>
#include <assert.h>
typedef int SLTDateType;
typedef struct SListNode
{
SLTDateType data;
struct SListNode* next;
}SListNode;
SListNode* BuySListNode(SLTDateType x);
void SListPrint(SListNode* plist);
SListNode* SListFind(SListNode* plist, SLTDateType x);
void SListPushBack(SListNode** pplist, SLTDateType x);
void SListPopBack(SListNode** pplist);
void SListPushFront(SListNode** pplist, SLTDateType x);
void SListPopFront(SListNode** pplist);
void SListInsertAfter(SListNode* pos, SLTDateType x);
void SListInsert(SListNode** pplist, SListNode* pos, SLTDateType x);
void SListEraseAfter(SListNode* pos);
void SListErase(SListNode** pplist, SListNode* pos);
void SListDestroy(SListNode** pplist);
二、链表各接口具体实现SListNode.c
本节主要包含:链表各个接口的实现方法
2.1 动态申请一个节点
SListNode* BuySListNode(SLTDateType x)
{
SListNode* newnode = (SListNode*)malloc(sizeof(SListNode));
if (NULL == newnode) {
printf("%s\n", strerror(errno));
exit(-1);
}
else {
newnode->data = x;
newnode->next = NULL;
}
return newnode;
}
2.2 单链表打印
void SListPrint(SListNode* plist)
{
if (NULL == plist) {
printf("头指针为NULL\n");
return;
}
else {
SListNode* cur = plist;
while (cur != NULL) {
printf("%d->", cur->data);
cur = cur->next;
}
}
printf("NULL\n");
}
2.3 单链表查找
SListNode* SListFind(SListNode* plist, SLTDateType x)
{
if (NULL == plist) {
return NULL;
}
SListNode* cur = plist;
while (NULL != cur) {
if (x == (cur->data)) {
return cur;
}
else {
cur = cur->next;
}
}
return NULL;
}
2.4 单链表尾插(需要传二级指针->在于是否需要改变slist)
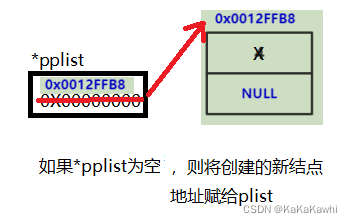 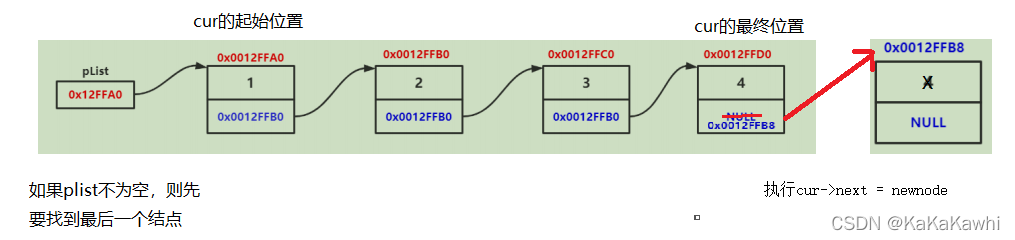
void SListPushBack(SListNode** pplist, SLTDateType x)
{
assert(pplist);
SListNode* newnode = BuySListNode(x);
if (NULL == *pplist) {
*pplist = newnode;
}
else {
SListNode* cur = *pplist;
while ((cur->next) != NULL) {
cur = cur->next;
}
cur->next = newnode;
}
}
2.5 单链表尾删(需要传二级指针->在于是否需要改变slist)
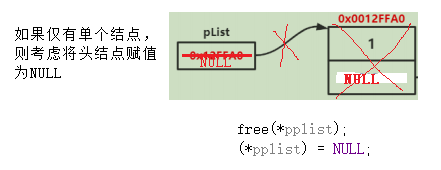 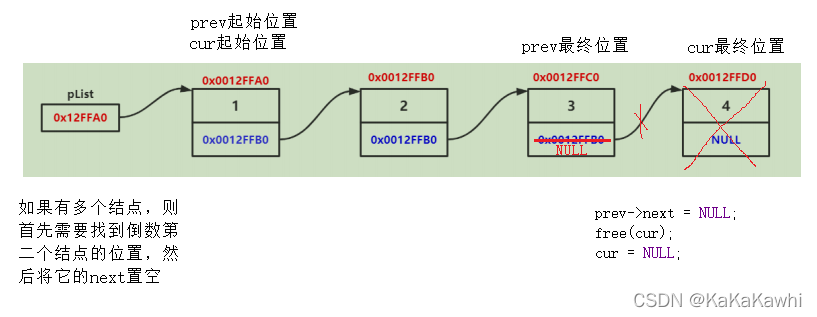
void SListPopBack(SListNode** pplist)
{
assert(pplist);
if (NULL == *pplist) {
printf("头指针为NULL\n");
return;
}
else if (NULL == ((*pplist)->next)) {
free(*pplist);
(*pplist) = NULL;
}
else {
SListNode* cur = *pplist;
SListNode* prev = NULL;
while ((cur->next) != NULL) {
prev = cur;
cur = cur->next;
}
prev->next = NULL;
free(cur);
cur = NULL;
}
}
2.6 单链表头插(需要传二级指针->在于是否需要改变slist)
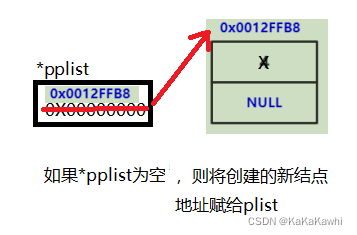 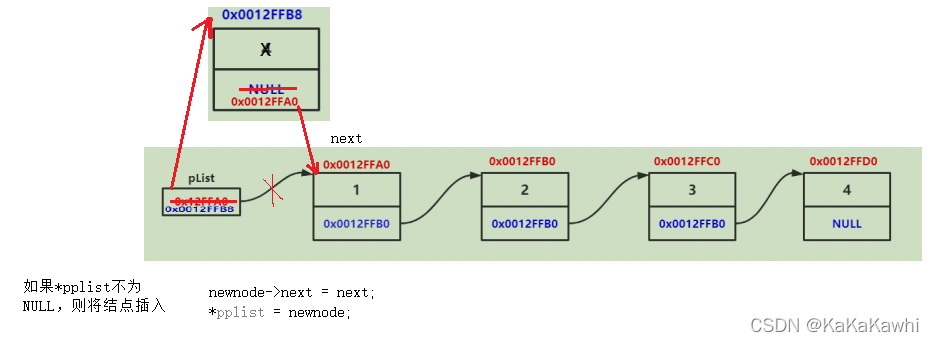
void SListPushFront(SListNode** pplist, SLTDateType x)
{
assert(pplist);
SListNode* newnode = BuySListNode(x);
if (NULL == *pplist) {
*pplist = newnode;
}
else {
SListNode* next = *pplist;
newnode->next = next;
*pplist = newnode;
}
}
2.7 单链表头删(需要传二级指针->在于是否需要改变slist)
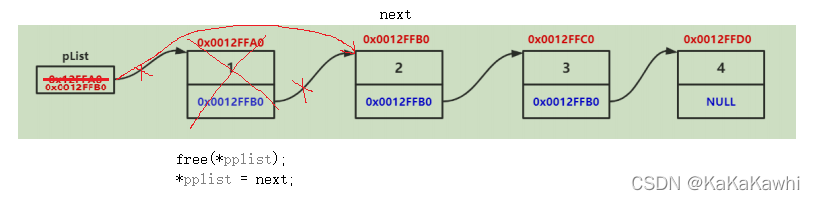
void SListPopFront(SListNode** pplist)
{
assert(pplist);
if (NULL == *pplist) {
printf("头指针为NULL\n");
return;
}
else {
SListNode* next = (*pplist)->next;
free(*pplist);
*pplist = next;
}
}
2.8 单链表在pos位置之后插入x
void SListInsertAfter(SListNode* pos, SLTDateType x)
{
assert(pos);
SListNode* newnode = BuySListNode(x);
SListNode* next = pos->next;
pos->next = newnode;
newnode->next = next;
}
2.9 单链表在pos位置插入x
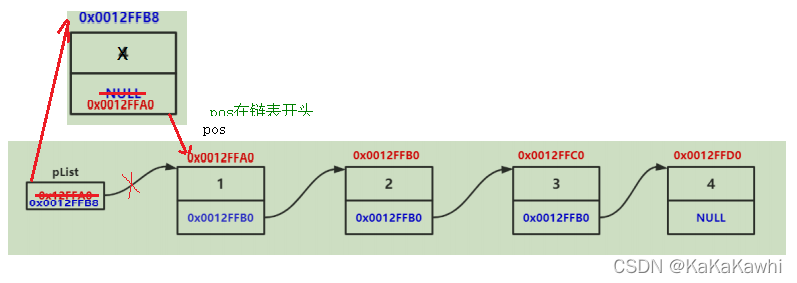 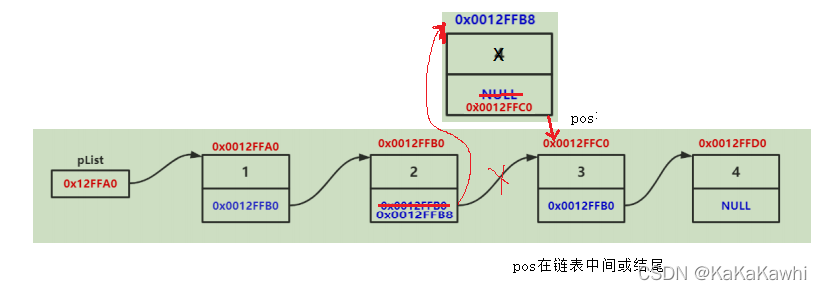
void SListInsert(SListNode** pplist, SListNode* pos, SLTDateType x)
{
assert(pplist);
assert(pos);
if (NULL == *pplist) {
printf("头指针为NULL\n");
return;
}
else if (*pplist == pos) {
SListPushFront(pplist, x);
}
else {
SListNode* newnode = BuySListNode(x);
SListNode* cur = *pplist;
while (pos != (cur->next)) {
cur = cur->next;
}
cur->next = newnode;
newnode->next = pos;
}
}
2.10 单链表删除pos位置之后的值
void SListEraseAfter(SListNode* pos)
{
assert(pos);
SListNode* next = pos->next;
if (next) {
pos->next = next->next;
free(next);
next = NULL;
}
else {
printf("pos位置之后为NULL,无法删除\n");
return;
}
}
2.12 单链表删除pos位置的值
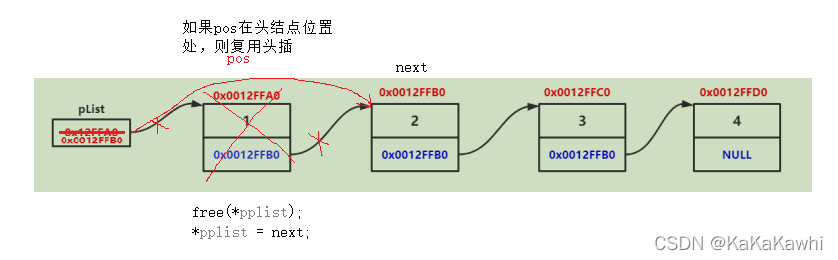 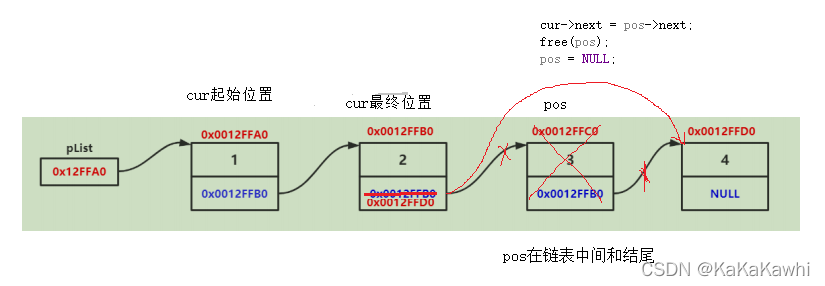
void SListErase(SListNode** pplist, SListNode* pos)
{
assert(pplist);
assert(pos);
if (NULL == *pplist) {
printf("NULL\n");
return;
}
else if(*pplist == pos){
SListPopFront(pplist);
}
else {
SListNode* cur = *pplist;
while (pos != (cur->next)) {
cur = cur->next;
}
cur->next = pos->next;
free(pos);
pos = NULL;
}
}
2.11 单链表销毁
void SListDestroy(SListNode** pplist)
{
assert(pplist);
SListNode* cur = *pplist;
while (NULL != cur) {
SListNode* next = cur->next;
free(cur);
cur = next;
}
*pplist = NULL;
}
三、链表接口测试test.c
#define _CRT_SECURE_NO_WARNINGS 1
#include "SListNode.h"
int main()
{
SListNode* slist = NULL;
SListNode* n1 = BuySListNode(1);
SListNode* n2 = BuySListNode(2);
SListNode* n3 = BuySListNode(3);
SListNode* n4 = BuySListNode(4);
SListNode* n5 = BuySListNode(5);
slist = n1;
n1->next = n2;
n2->next = n3;
n3->next = n4;
n4->next = n5;
n5->next = NULL;
SListPrint(slist);
SListPushBack(&slist, 100);
SListPrint(slist);
SListPopBack(&slist);
SListPrint(slist);
SListPushFront(&slist, 123);
SListPrint(slist);
SListPopFront(&slist);
SListPrint(slist);
SListInsertAfter(n4, 88);
SListPrint(slist);
SListInsert(&slist, n4, 99);
SListPrint(slist);
SListEraseAfter(n4);
SListPrint(slist);
SListErase(&slist, n4);
SListPrint(slist);
SListNode* ret = SListFind(slist, 2);
if (ret) {
ret->data = 77;
printf("%d\n", ret->data);
}
SListDestroy(&slist);
return 0;
}
总结
这里对文章进行总结: 以上就是今天总结的内容,本文包括了C语言实现单链表各个接口,分享给大家。 真💙欢迎各位给予我更好的建议,欢迎访问!!!小编创作不易,觉得有用可以一键三连哦,感谢大家。peace 希望大家一起坚持学习,共同进步。梦想一旦被付诸行动,就会变得神圣。
欢迎各位大佬批评建议,分享更好的方法!!!🙊🙊🙊
|