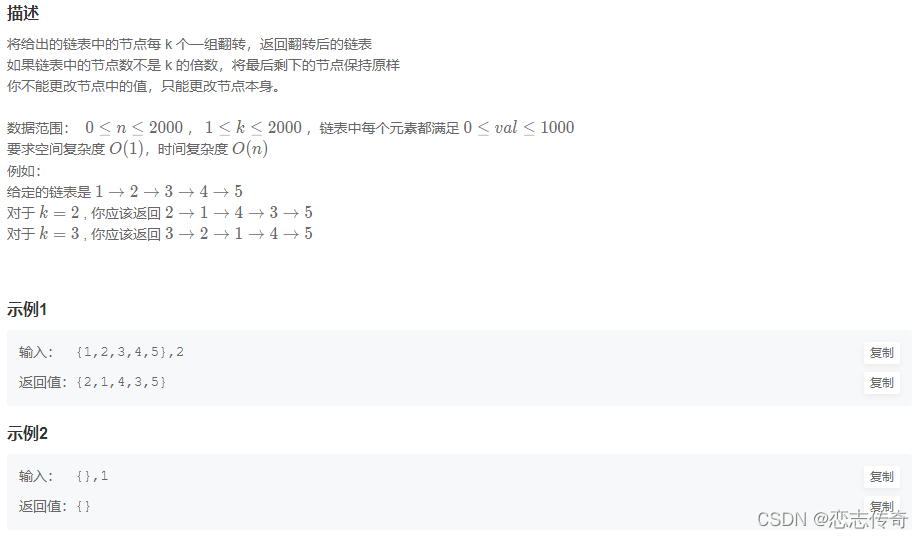
?
import java.util.*;
/*
* public class ListNode {
* int val;
* ListNode next = null;
* }
*/
public class Solution {
/**
*
* @param head ListNode类
* @param k int整型
* @return ListNode类
*/
public ListNode reverseKGroup (ListNode head, int k) {
// write code here
if(k <= 1 || head == null) return head;
Stack<ListNode> st = new Stack<ListNode>(); //模拟栈
ListNode result = new ListNode(0);// 声明一个新的节点,用于返回结果
ListNode now = result;
int cnt = 0; // 计数
while(true){
for(int i = 0; i < k; i ++){ //将当前链表前k个存入栈中
st.push(head);
head = head.next;
cnt ++;
if(head == null) break;
}
if(cnt == k){ //如果当前栈中有k个元素则一一取出存入链表
while(!st.isEmpty()){
now.next = st.pop();
now = now.next;
now.next = null;
}
}
if(head == null) break; //如果链表取完了跳出循环
cnt = 0;
}
ListNode end = null;
while(!st.isEmpty()){ //如果栈中还有剩下的就说明是最后的一块直接取栈底即可
end = st.pop();
}
now.next = end;
return result.next;
}
}
|