意思就是构建一个单链表的类。 包装一下。 【以下是类的基本结构】
struct Node {
int data;
Node *next;
};
class LinkList {
private:
Node *head;
public:
LinkList();
void Insert(const int &);
bool Delete(const int &);
bool Search(const int &);
void Show();
friend ostream &operator<<(ostream &out, const LinkList &L);
~LinkList();
};
【具体实现】
LinkList::LinkList() {
head = new Node;
head->data = 0;
head->next = NULL;
}
void LinkList::Insert(const int &elem) {
Node *p = new Node;
p->data = elem;
p->next = head->next;
head->next = p;
}
bool LinkList::Delete(const int &elem) {
Node *p = head->next;
Node *q = head;
while (p) {
if (p->data == elem) {
q->next = p->next;
delete p;
return 1;
}
p = p->next;
q = q->next;
}
return 0;
}
void LinkList::Show() {
Node *p = head->next;
while (p) {
cout << p->data << " ";
p = p->next;
}
cout << endl;
}
bool LinkList::Search(const int &add) {
Node *p = head->next;
int n = 1;
while (p && n <= add) {
if (add == n) {
cout << p->data;
return true;
}
p = p->next;
n++;
}
return false;
}
ostream &operator<<(ostream &out, const LinkList &L) {
Node *p = L.head->next;
while (p) {
out << p->data << " ";
p = p->next;
}
out << endl;
return out;
}
LinkList::~LinkList() {
while (head) {
Node *q = head;
head = head->next;
delete q;
}
}
【测试代码】
void menu() {
cout << "测试单链表:" << endl
<< "1. 插入指定元素" << endl
<< "2. 删除指定元素" << endl
<< "3. 查找指定位置的元素" << endl
<< "4. 展示链表内部元素-通过Show方法" << endl
<< "5. 展示链表内部元素-通过<<输出流" << endl
<< "6. 离开" << endl
<< "你的选择是:";
}
int main () {
LinkList *L = new LinkList;
int n, elem;
menu();
cin >> n;
while (n) {
if (n == 1) {
cout << "插入元素是:";
cin >> elem;
L->Insert(elem);
} else if (n == 2) {
cout << "删除元素是:";
cin >> elem;
if (L->Delete(elem)) cout << "【成功删除】" << endl;
else cout << "【未找到该元素,不能删除】" << endl;
} else if (n == 3) {
cout << "查找元素是:";
cin >> elem;
if (L->Search(elem)) cout << "【成功找到】" << endl;
else cout << "【未找到该元素】" << endl;
}else if (n == 4) L->Show();
else if (n == 5) cout << *L;
else break;
menu();
cin >> n;
}
delete L;
return 0;
}
【部分测试结果】 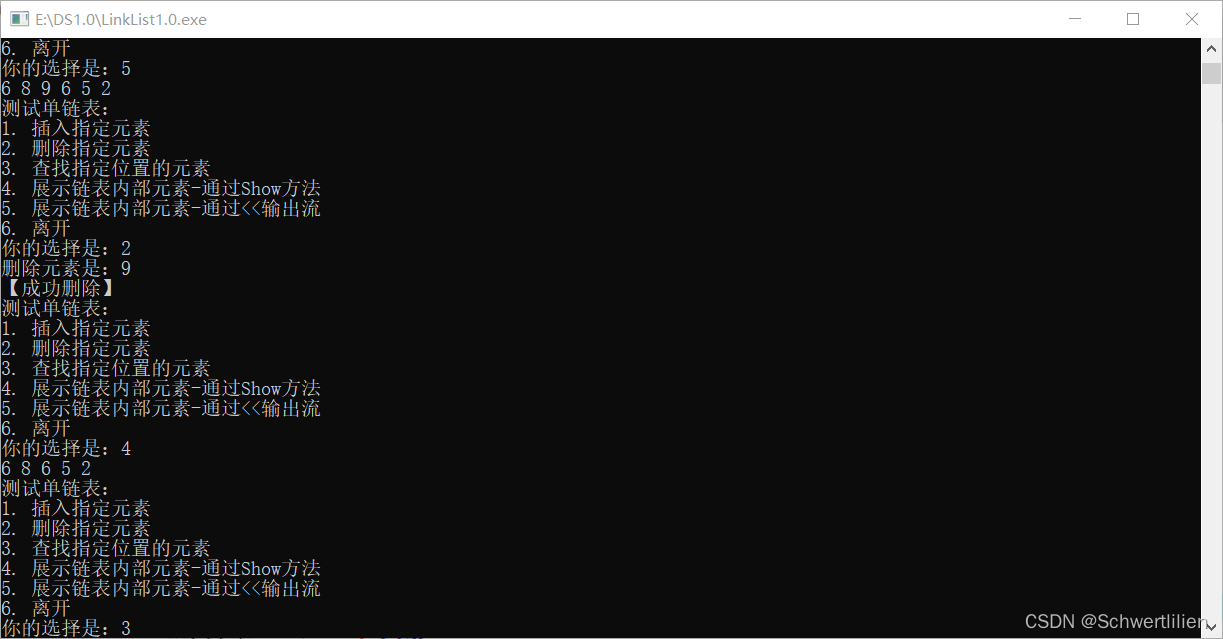 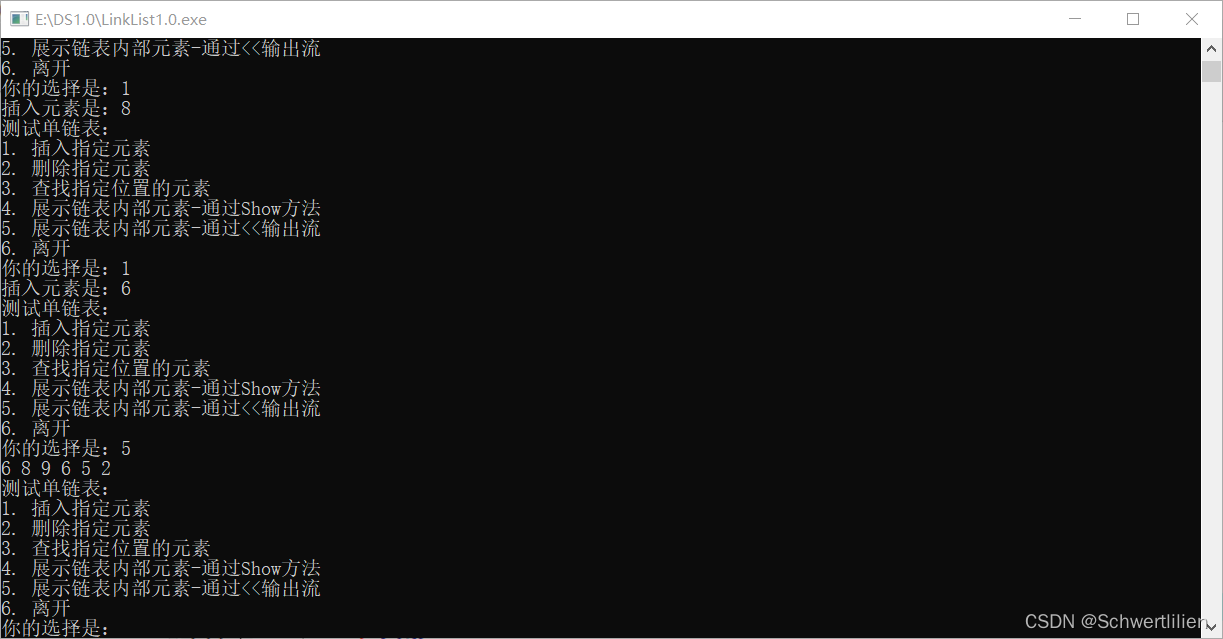
|