原创首发于CSDN,转载请注明出处,谢谢!https://blog.csdn.net/weixin_46959681/article/details/123631398
头插法
演示代码:insertHead.c
#include <stdio.h>
#include <stdlib.h>
struct Test{
int data;
struct Test *next;
};
struct Test *insertHead(struct Test *head,struct Test *new)
{
if(head == NULL){
head = new;
}else{
new->next = head;
head = new;
}
return head;
}
struct Test* createLink(struct Test *head)
{
struct Test *new;
while(1){
new = (struct Test *)malloc(sizeof(struct Test));
printf("Please enter a number as the new node: ");
scanf("%d",&(new->data));
if(new->data == 0){
printf("0 Quit.\n");
free(new);
return head;
}
head = insertHead(head,new);
}
}
void printLink(struct Test *head);
int main()
{
struct Test *head = NULL;
head = createLink(head);
printLink(head);
return 0;
}
void printLink(struct Test *head){
struct Test *p = NULL;
p = head;
while(p != NULL){
printf("%d ",p->data);
p = p->next;
}
putchar('\n');
}
运行结果:
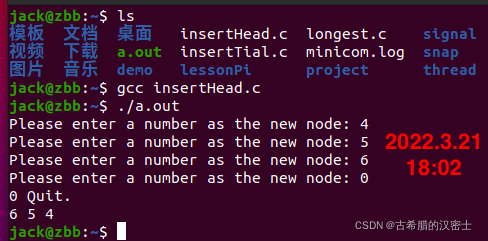
尾插法
演示代码:insertTial.c
#include <stdio.h>
#include <stdlib.h>
struct Test{
int data;
struct Test *next;
};
struct Test *insertTial(struct Test *head,struct Test *new)
{
struct Test *p = NULL;
p = head;
if(p == NULL){
head = new;
return head;
}
while(p->next != NULL){
p = p->next;
}
p->next = new;
return head;
}
struct Test* createLink(struct Test *head)
{
struct Test *new;
while(1){
new = (struct Test *)malloc(sizeof(struct Test));
printf("Please enter a number as the new node: ");
scanf("%d",&(new->data));
if(new->data == 0){
printf("0 Quit.\n");
free(new);
return head;
}
head = insertTial(head,new);
}
}
void printLink(struct Test *head);
int main(){
struct Test *head = NULL;
head = createLink(head);
printLink(head);
return 0;
}
void printLink(struct Test *head){
struct Test *p = NULL;
p = head;
while(p != NULL){
printf("%d ",p->data);
p = p->next;
}
putchar('\n');
}
运行结果:
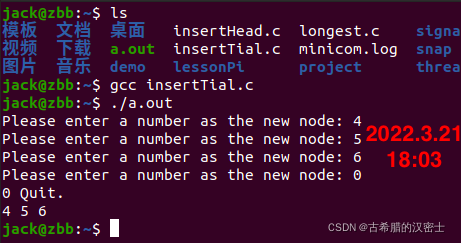
参考资料
|