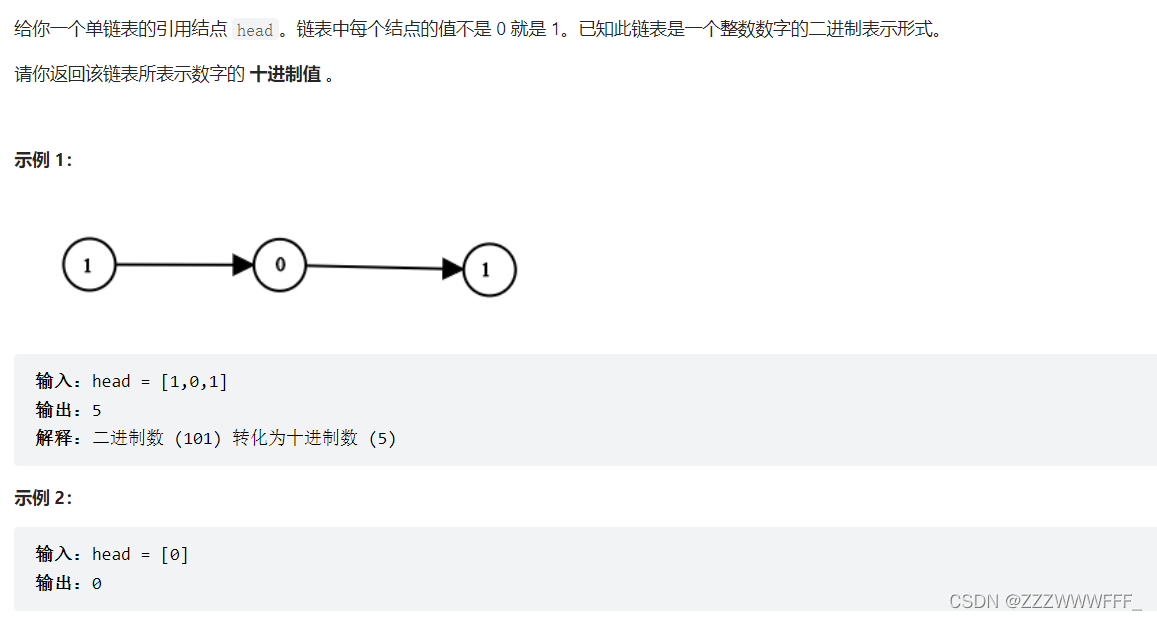
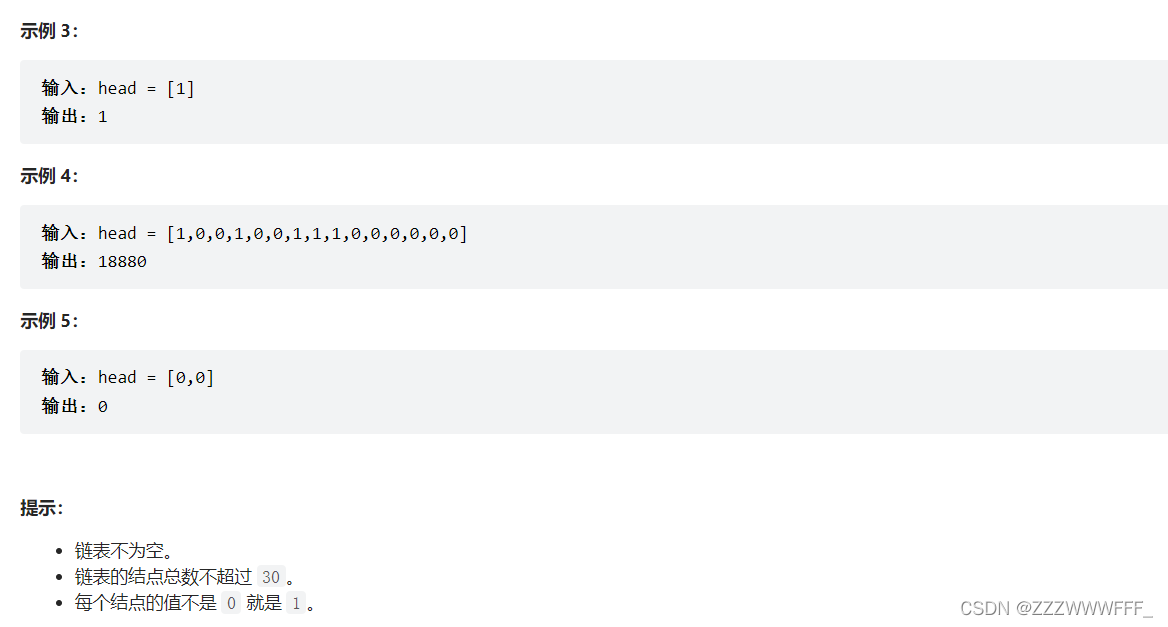
?该题最容易想到的思路就是直接模拟我们在遇到一个二进制数将它转换成十进制数的过程
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* ListNode *next;
* ListNode() : val(0), next(nullptr) {}
* ListNode(int x) : val(x), next(nullptr) {}
* ListNode(int x, ListNode *next) : val(x), next(next) {}
* };
*/
class Solution {
public:
int getDecimalValue(ListNode* head) {
int cn=0,num=0;
for(ListNode *p=head;p;p=p->next)
{
cn++;//计算有多少个结点
}
cn-=1;//由于最后一个结点是乘以2^0,所以--
for(ListNode *p=head;p;p=p->next)
{
if(p->val==1)
num+=pow(2,cn);
cn--;
}
return num;
}
};
栈
该思路与上面的思路如出一辙,只是多利用了栈的“先入后出”的特点
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* ListNode *next;
* ListNode() : val(0), next(nullptr) {}
* ListNode(int x) : val(x), next(nullptr) {}
* ListNode(int x, ListNode *next) : val(x), next(next) {}
* };
*/
class Solution {
public:
int getDecimalValue(ListNode* head) {
stack<int>num;
ListNode *p=head;
int n=0,ans=0;
while(p!=nullptr)
{
num.push(p->val);//每个结点的值都入栈
p=p->next;
}
while(!num.empty())
{
if(num.top()==1)
ans+=pow(2,n);
n++;
num.pop();
}
return ans;
}
};
位运算?
该思路就是像我们计算一个十进制数的模样去模拟,比如
1234=((((0*10+1)*10+2)*10+3)*10+4),对于二进制而言我们将10换成2即可
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* ListNode *next;
* ListNode() : val(0), next(nullptr) {}
* ListNode(int x) : val(x), next(nullptr) {}
* ListNode(int x, ListNode *next) : val(x), next(next) {}
* };
*/
class Solution {//位运算
public:
int getDecimalValue(ListNode* head) {
int ans=0;
ListNode *p=head;
while(p!=nullptr)
{
ans=(ans<<1)+p->val;
p=p->next;
}
delete p;
return ans;
}
};
|