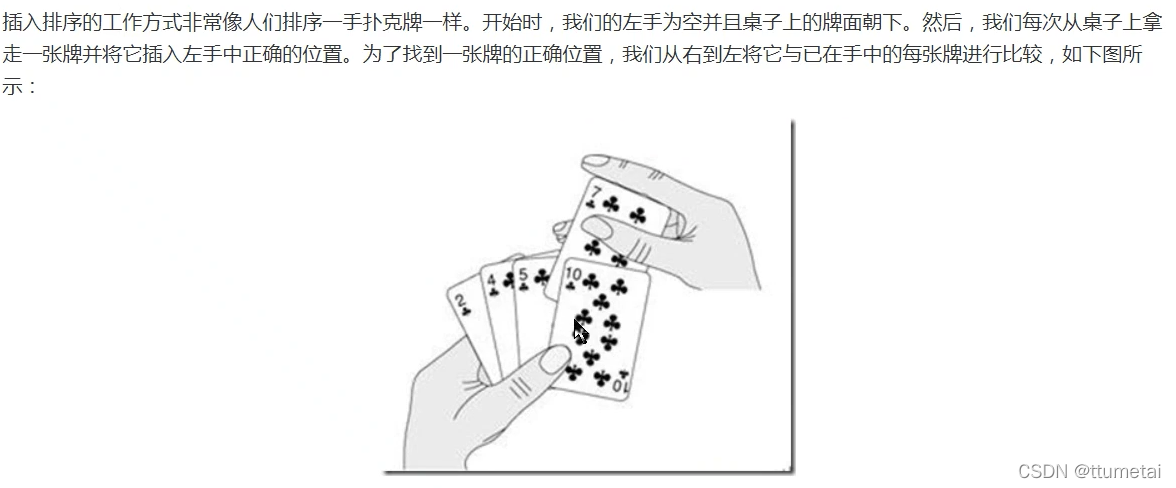 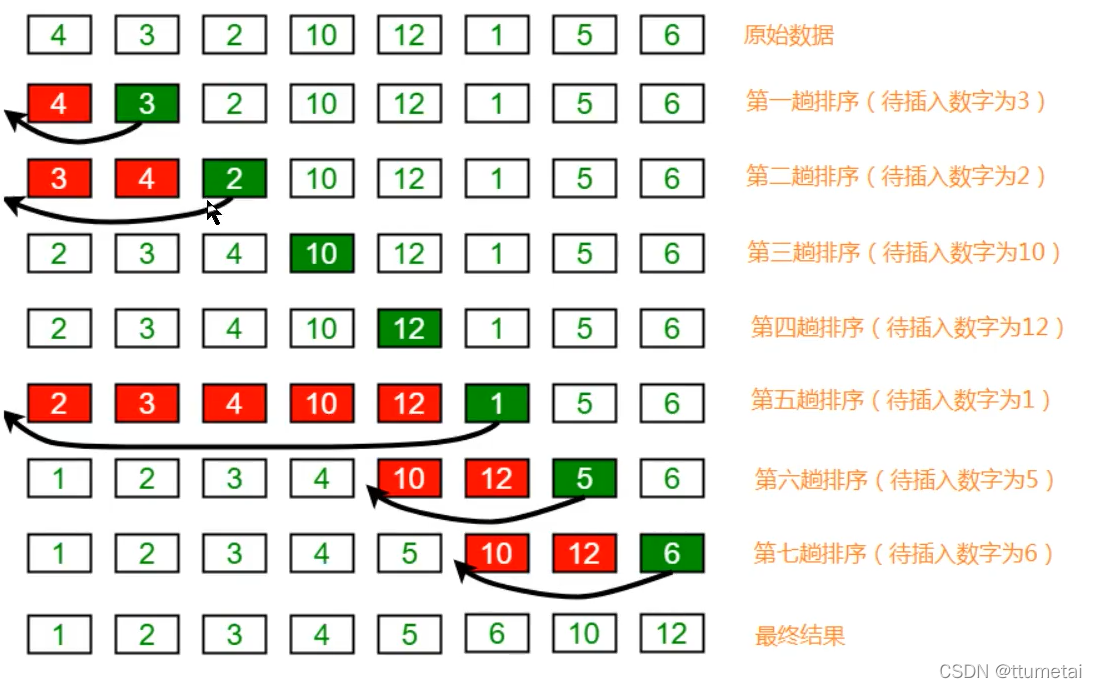
package algorithm.sort;
public class Insertion {
public static void sort(Comparable[] a){
for (int i = 1 ; i < a.length ; i ++){
for (int j = i - 1 ; j > -1 ; j --){
if (greater(a[j],a[i])){
exchange(a,j,i);
i--;
}else{
break;
}
}
}
}
public static boolean greater(Comparable v,Comparable w){
return v.compareTo(w) > 0;
}
public static void exchange(Comparable[] a,int i,int j){
Comparable temp = a[i];
a[i] = a[j];
a[j] = temp;
}
}
package algorithm.test;
import algorithm.sort.Insertion;
import java.util.Arrays;
public class InsertionTest {
public static void main(String[] args) {
Integer[] array = {5,3,4,1,2};
Insertion.sort(array);
System.out.println(Arrays.toString(array));
}
}
得到结果
[1, 2, 3, 4, 5]
|