BFS的模板
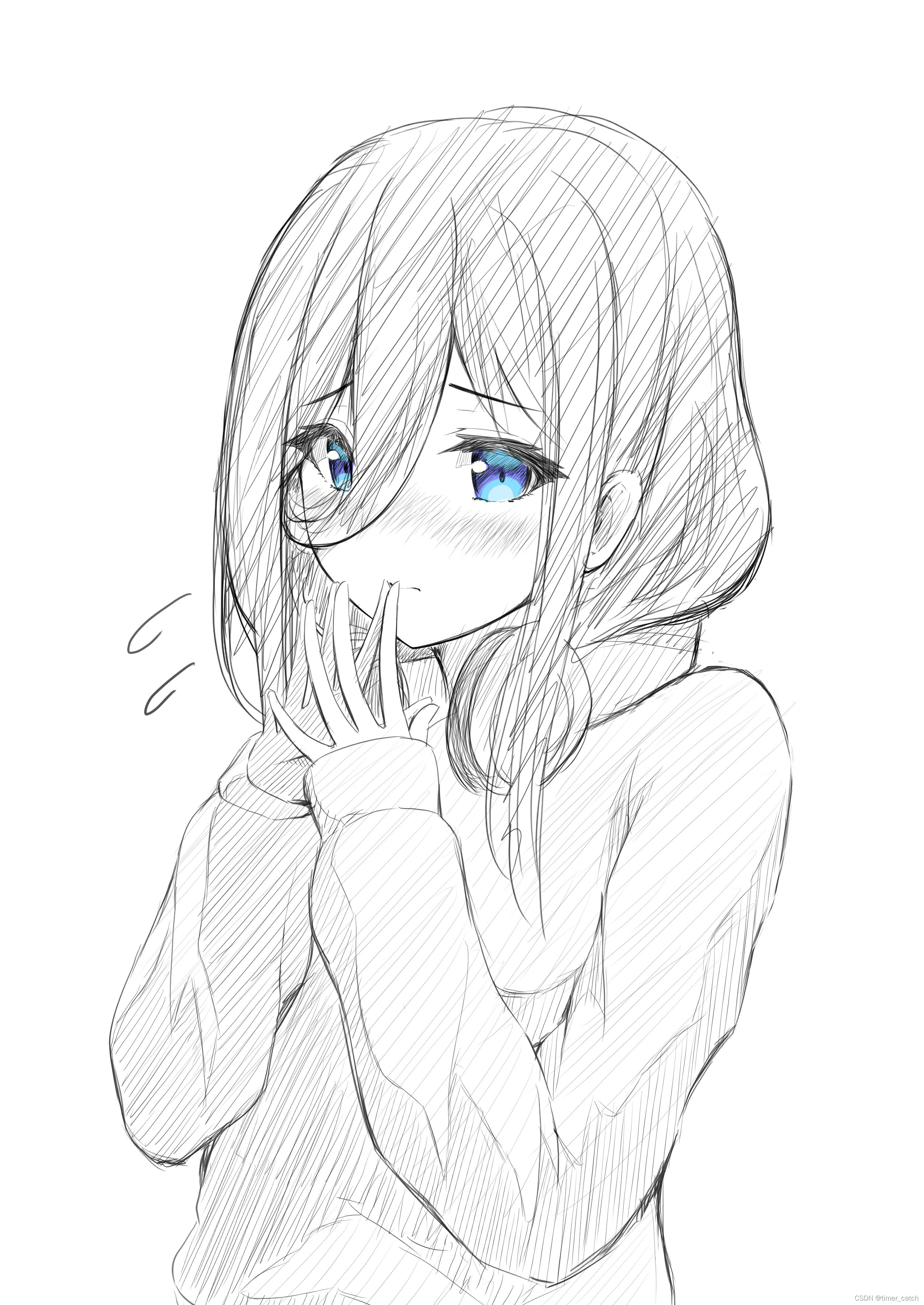
什么是bfs:
广度优先搜索,在搜索过程中由近及远,层层搜索。
从一个简单问题开始:
首先,看一个最简单的01迷宫:
1 1 1 0 0 1 0 1 1 0 1 0 1 1 0 1 1 0 1 0
起
点
:
(
1
,
1
)
{\color{red}起点:(1,1)}
起点:(1,1)
终
点
:
(
5
,
5
)
{\color{red}终点:(5,5)}
终点:(5,5)
要求我们输出从起点走到终点的最小步数。 不妨先给出代码,然后层层分析。
模板代码:
#include<iostream>
using namespace std;
const int MAXN = 200;
struct node{
int x;
int y;
int step;
}que[MAXN*MAXN];
int g[MAXN][MAXN];
int n,m,head,tail;
bool book[MAXN][MAXN];
int ne[4][2] = {{0,1},{1,0},{-1,0},{0,-1}};
void bfs()
{
head = 0,tail = -1;
que[++tail].x = 1;
que[tail].y = 1;
que[tail].step = 0;
book[1][1] = true;
while(tail>=head)
{
auto temp = que[head++];
if(temp.x==n&&temp.y==m)
{
cout<<temp.step;
return ;
}
for(int i = 0; i < 4 ; i++)
{
int tx = temp.x+ne[i][0];
int ty = temp.y+ne[i][1];
if(tx<1||ty<1||tx>n||ty>m||g[tx][ty]==1||book[tx][ty])
continue;
else
{
que[++tail].x = tx;
que[tail].y = ty;
que[tail].step = temp.step+1;
book[tx][ty] = true;
}
}
}
}
int main()
{
cin>>n>>m;
for(int i = 1 ; i <= n ; i++)
{
for(int j = 1; j <= m ; j++)
{
cin>>g[i][j];
}
}
bfs();
return 0;
}
代码的分析: 1.手写队列的使用:
tail >= head
tail++
que[tail] = (x,y);
node = que[head]
head++
2.从起点向四周拓展的方式:
int ne[4][2] = {{0,1},{1,0},{-1,0},{0,-1}};
for(int i = 0 ; i < 4 ; i++)
{
int tx = temp.x+ne[i][0];
int ty = temp.y+ne[i][1];
}
3.判断拓展出的点是否合法:
if(tx<1||ty<1||tx>n||ty>m||g[tx][ty]==1||book[tx][ty])continue;
4.避免重复地拓展相同的点:
book[tx][ty] = true;
5.判断是否走至终点并输出最小步数:
if(temp.x==n&&temp.y==m)
{
cout<<temp.step;
return;
}
|